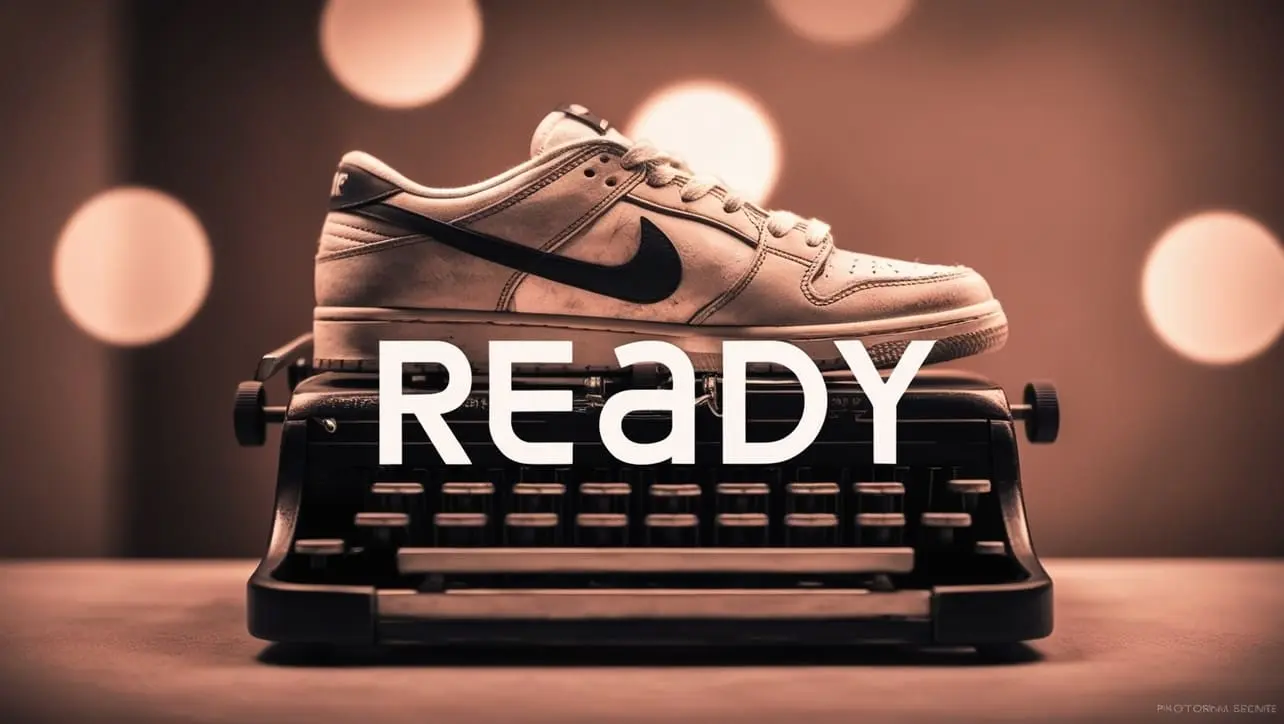
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery jQuery.removeData() Method
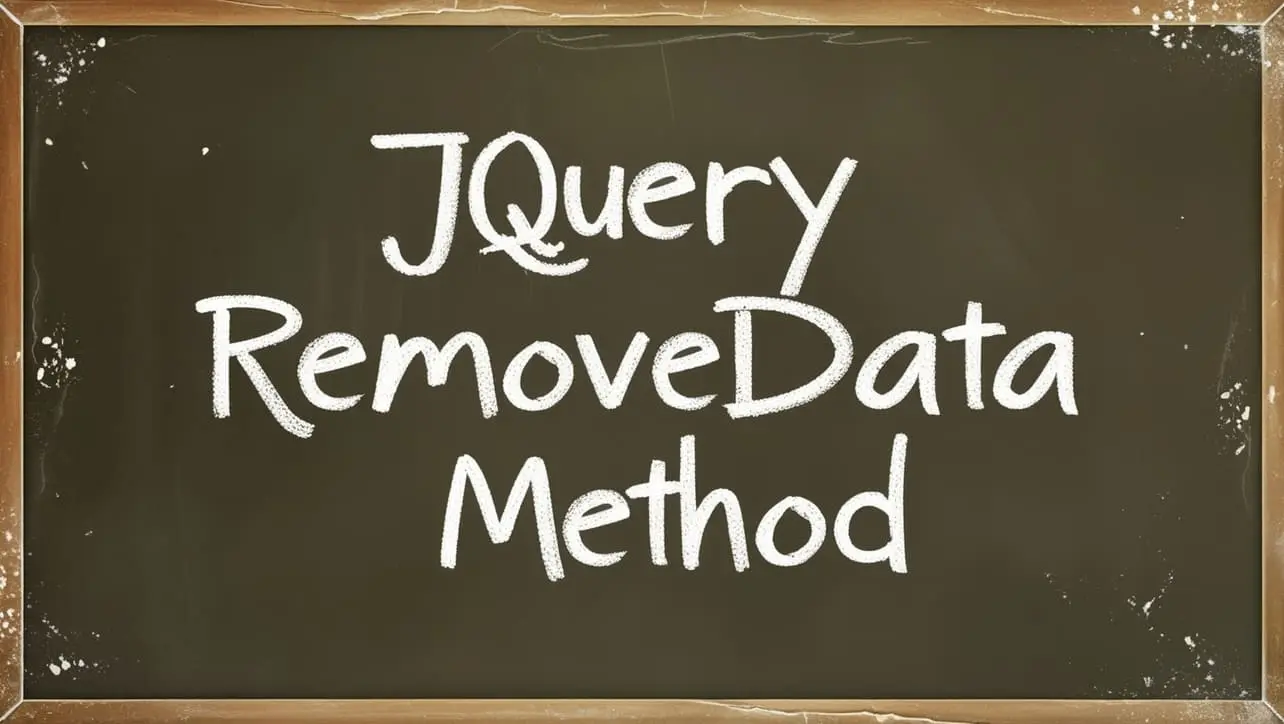
Photo Credit to CodeToFun
Introduction
In web development, managing data associated with HTML elements is crucial for creating dynamic and interactive user experiences. jQuery offers a robust method for handling this: .removeData()
. This method allows you to remove data that was previously set on elements using jQuery.
In this guide, we will explore the .removeData()
method, its syntax, and practical examples to help you understand how to use it effectively.
Understanding jQuery.removeData() Method
The .removeData()
method in jQuery is used to remove data that has been associated with DOM elements. This data is usually set using the .data() method and can be crucial for various operations such as storing state information, caching, and more.
Syntax
The syntax for the jQuery.removeData()
method is straightforward:
$(element).removeData(name);
Parameters:
- element: The target DOM element(s) from which the data should be removed.
- name (optional): A string naming the piece of data to remove. If omitted, all data associated with the elements will be removed.
Example
Removing Specific Data:
You can remove specific data associated with an element by passing the name of the data as an argument.
index.htmlCopied<div id="myDiv" data-info="example"></div>
example.jsCopied// Setting data $("#myDiv").data("info", "example"); // Removing specific data $("#myDiv").removeData("info");
After calling removeData("info"), the info data will be removed from the #myDiv element.
Removing All Data:
If you want to remove all data associated with an element, simply call
.removeData()
without any arguments.index.htmlCopied<div id="myDiv" data-info="example" data-detail="details"></div>
example.jsCopied// Setting multiple data points $("#myDiv").data("info", "example"); $("#myDiv").data("detail", "details"); // Removing all data $("#myDiv").removeData();
This will remove both info and detail data from the #myDiv element.
Cleaning Up Data After AJAX Requests:
When working with AJAX, you might store temporary data on elements. Once the AJAX operation is complete, you can clean up this data to free up memory.
example.jsCopied$("#myButton").on("click", function() { var $this = $(this); // Storing temporary data $this.data("requestPending", true); $.ajax({ url: "example.com/api", success: function() { // Handling successful response // Removing the temporary data $this.removeData("requestPending"); } }); });
Managing State in Complex Interfaces:
In complex UIs, you might need to manage the state of various components. Using
.removeData()
, you can reset the state when necessary.example.jsCopied$("#myDiv").data("state", "active"); // Resetting state $("#myDiv").removeData("state");
Conclusion
The jQuery .removeData()
method is a powerful tool for managing data associated with DOM elements. Whether you need to remove specific pieces of data or clear all data from an element, .removeData()
provides a simple and efficient way to handle these operations.
By understanding and utilizing this method, you can ensure your web applications remain clean, efficient, and easy to maintain.
Join our Community:
Author
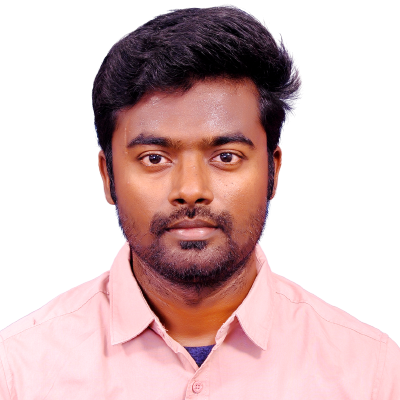
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.removeData() Method), please comment here. I will help you immediately.