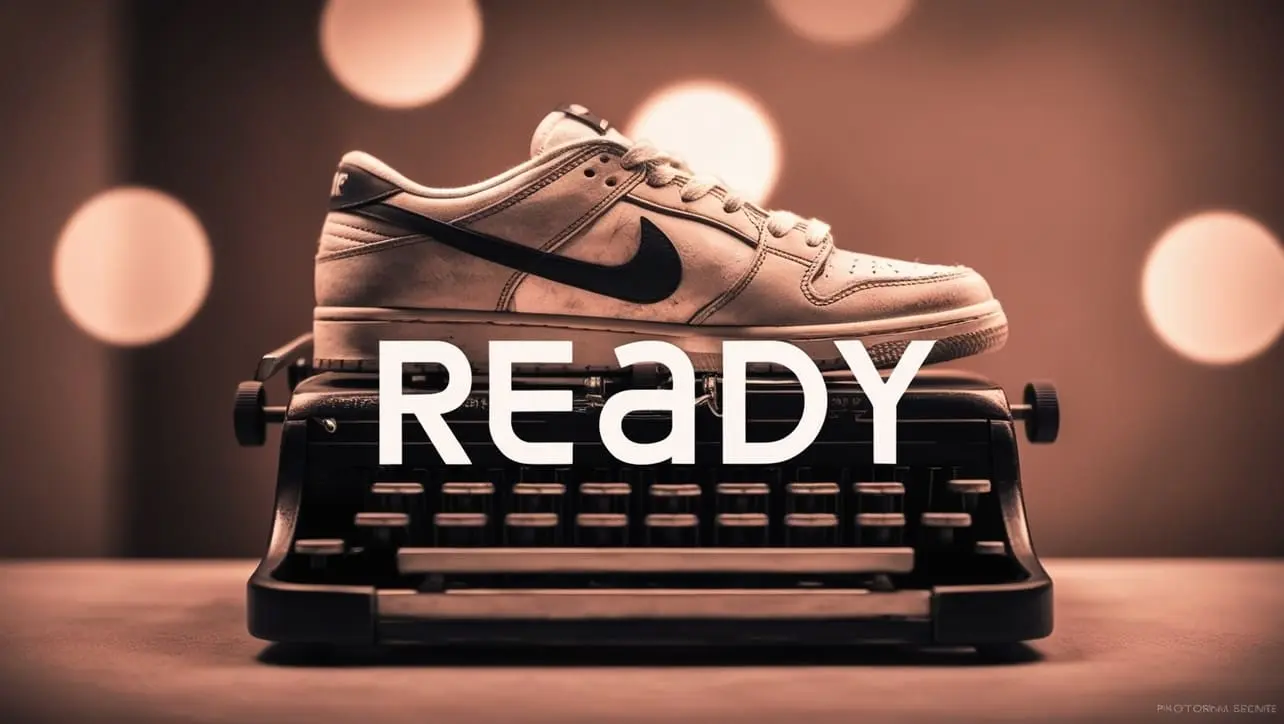
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery jQuery.ready Object
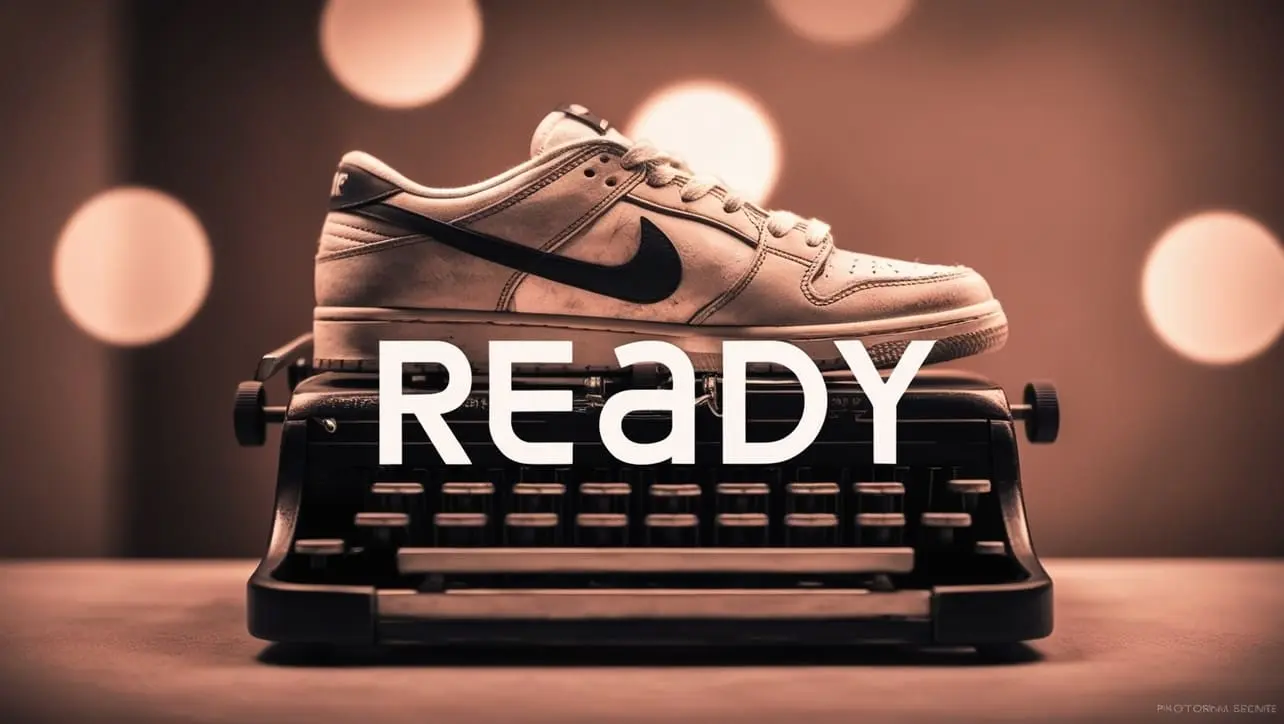
Photo Credit to CodeToFun
🙋 Introduction
jQuery makes it easy to handle the document ready event, which ensures that your code runs only after the DOM is fully loaded. This is crucial for interacting with elements that need to be present and fully rendered before any JavaScript manipulations occur.
In this guide, we will explore the jQuery.ready
method and how to use it effectively with examples.
🧠 Understanding jQuery.ready
The jQuery.ready
event is triggered when the DOM is fully loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. It ensures that your JavaScript code is executed as soon as the document is ready.
💡 Basic Syntax:
The traditional way to use the document ready event in jQuery is as follows:
$(document).ready(function() {
// Your code here
});
💡 Alternatively, the shorthand version is:
$(function() {
// Your code here
});
📝 Example
Basic Document Ready Usage:
This example shows the simplest way to use the document ready event to ensure the DOM is fully loaded before executing the code:
index.htmlCopied<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document Ready Example</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(document).ready(function() { console.log("DOM is fully loaded"); }); </script> </head> <body> <p>Hello, world!</p> </body> </html>
In this example, the message DOM is fully loaded will be logged to the console once the DOM is ready.
Using $.when() with $.ready:
The $.when() method can be used in conjunction with $.ready to perform actions once the document is ready. This approach is particularly useful for more complex scenarios involving multiple asynchronous operations:
index.htmlCopied<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>$.when with $.ready Example</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $.when($.ready).then(function() { console.log("Document is ready using $.when()"); }); </script> </head> <body> <p>Welcome to the $.when and $.ready example!</p> </body> </html>
In this example, the message Document is ready using $.when() will be logged once the document is fully loaded.
Document Ready with Multiple Handlers:
You can attach multiple handlers to the document ready event. Each handler will be executed in the order they were added:
index.htmlCopied<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Multiple Document Ready Handlers</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script> $(function() { console.log("First handler"); }); $(function() { console.log("Second handler"); }); </script> </head> <body> <p>Check the console for multiple handlers example.</p> </body> </html>
Both First handler and Second handler messages will be logged to the console in sequence.
Running Code After the DOM is Fully Loaded:
It's a common requirement to ensure that certain scripts run after all DOM elements are fully loaded. Using
jQuery.ready
guarantees that your code executes only when the DOM is ready:example.jsCopied$(document).ready(function() { // Your DOM manipulation code here });
This ensures that your script interacts with elements that are already rendered in the document.
🎉 Conclusion
The jQuery.ready
method is an essential tool for ensuring your JavaScript code runs only after the DOM is fully loaded. Whether you use the traditional $(document).ready() syntax or the more versatile $.when($.ready), mastering this technique will help you create more reliable and interactive web pages.
By using multiple handlers or combining ready events with other asynchronous operations, you can build complex, responsive web applications with ease.
👨💻 Join our Community:
Author
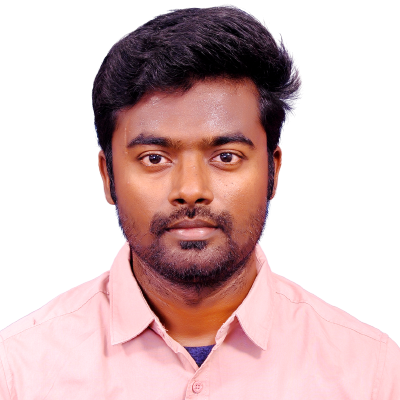
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.ready Object), please comment here. I will help you immediately.