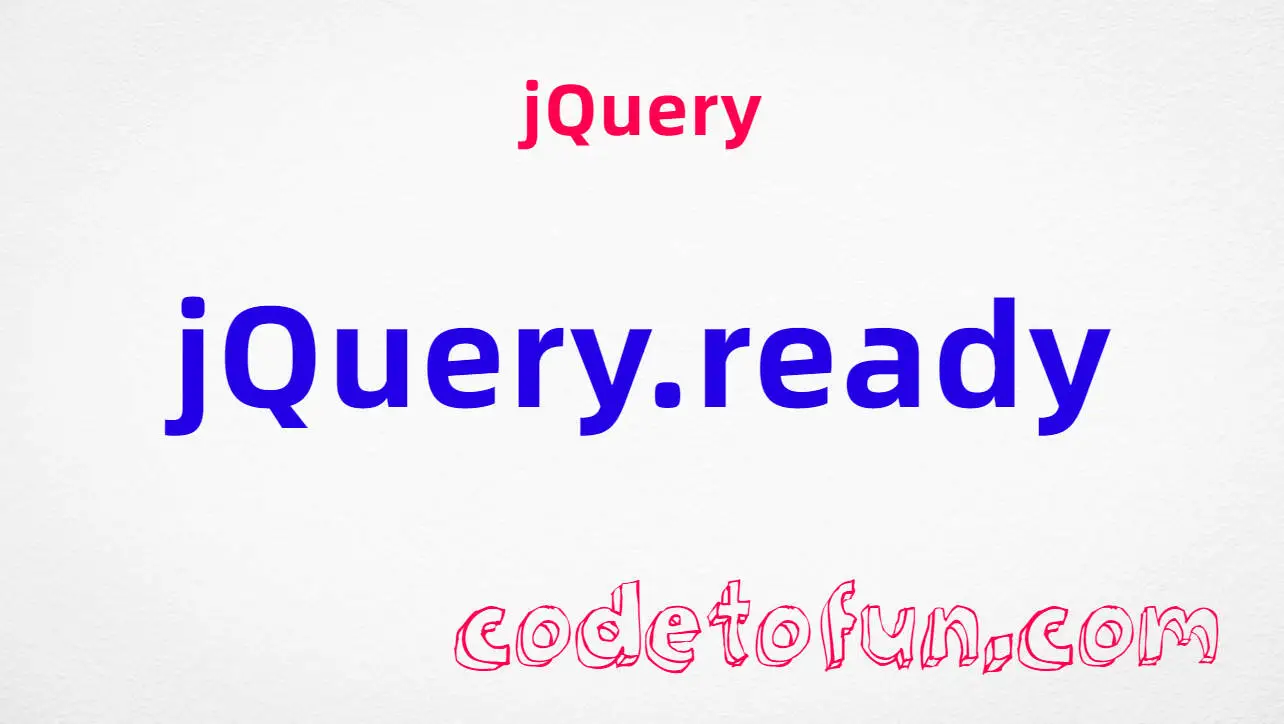
jQuery Basic
jQuery Ajax Events
- jQuery ajaxComplete
- jQuery ajaxError
- jQuery ajaxSend
- jQuery ajaxStart
- jQuery ajaxStop
- jQuery ajaxSuccess
jQuery Ajax Methods
- jQuery .ajaxComplete()
- jQuery .ajaxError()
- jQuery .ajaxSend()
- jQuery .ajaxStart()
- jQuery .ajaxStop()
- jQuery .ajaxSuccess()
- jQuery jQuery.ajax()
- jQuery jQuery.ajaxPrefilter()
- jQuery jQuery.ajaxSetup()
- jQuery jQuery.ajaxTransport()
- jQuery .get()
- jQuery jQuery.getJSON()
- jQuery jQuery.param()
- jQuery jQuery.post()
- jQuery .load()
- jQuery .serialize()
- jQuery .serializeArray()
jQuery Keyboard Events
jQuery Keyboard Methods
jQuery Form Events
jQuery Form Methods
- jQuery .blur()
- jQuery .change()
- jQuery .focus()
- jQuery .focusin()
- jQuery .focusout()
- jQuery .select()
- jQuery .submit()
jQuery Mouse Event
- jQuery click
- jQuery contextmenu
- jQuery dblclick
- jQuery mousedown
- jQuery mouseenter
- jQuery mouseleave
- jQuery mousemove
- jQuery mouseout
- jQuery mouseover
- jQuery mouseup
jQuery Mouse Methods
- jQuery .click()
- jQuery .contextmenu()
- jQuery .dblclick()
- jQuery .hover()
- jQuery .mousedown()
- jQuery .mouseenter()
- jQuery .mouseleave()
- jQuery .mousemove()
- jQuery .mouseout()
- jQuery .mouseover()
- jQuery .mouseup()
- jQuery .toggle()
jQuery Event Object
- jQuery .bind()
- jQuery currentTarget
- jQuery data
- jQuery .delegate()
- jQuery delegateTarget
- jQuery .die()
- jQuery error
- jQuery .live()
- jQuery .off()
- jQuery .on()
- jQuery .one()
- jQuery isDefaultPrevented()
- jQuery isImmediatePropagationStopped()
- jQuery isPropagationStopped()
- jQuery metakey
- jQuery namespace
- jQuery pageX
- jQuery pageY
- jQuery preventDefault()
- jQuery relatedTarget
- jQuery resize
- jQuery result
- jQuery scroll()
- jQuery stopImmediatePropagation()
- jQuery stopPropagation()
- jQuery target
- jQuery timeStamp
- jQuery .trigger()
- jQuery .triggerHandler()
- jQuery type
- jQuery .unbind()
- jQuery .undelegate()
- jQuery which
jQuery Fading
jQuery Document Loading
- jQuery jQuery.error()
- jQuery .getScript()
- jQuery jQuery.holdReady()
- jQuery jQuery.ready
- jQuery load
- jQuery .ready()
- jQuery unload
- jQuery .unload()
jQuery Traversing
- jQuery .add()
- jQuery .addBack()
- jQuery .andSelf()
- jQuery .children()
- jQuery .closest()
- jQuery .contents()
- jQuery .each()
- jQuery .end()
- jQuery .eq()
- jQuery .even()
- jQuery .filter()
- jQuery .find()
- jQuery .first()
- jQuery .has()
- jQuery .is()
- jQuery .last()
- jQuery .map()
- jQuery .next()
- jQuery .nextAll()
- jQuery .nextUntil()
- jQuery .not()
- jQuery .odd()
- jQuery .offsetParent()
- jQuery .parent()
- jQuery .parents()
- jQuery .parentsUntil()
- jQuery .prev()
- jQuery .prevAll()
- jQuery .prevUntil()
- jQuery .siblings()
- jQuery .slice()
jQuery Utilities
- jQuery .clearQueue()
- jQuery .dequeue()
- jQuery jQuery.contains()
- jQuery jQuery.data()
- jQuery jQuery.dequeue()
- jQuery jQuery.each()
- jQuery jQuery.extend()
- jQuery jQuery.fn.extend()
- jQuery jQuery.globalEval()
- jQuery jQuery.grep()
- jQuery jQuery.inArray()
- jQuery jQuery.isArray()
- jQuery jQuery.isEmptyObject()
- jQuery jQuery.isFunction()
- jQuery jQuery.isNumeric()
- jQuery jQuery.isPlainObject()
- jQuery jQuery.isWindow()
- jQuery jQuery.isXMLDoc()
- jQuery jQuery.makeArray()
- jQuery jQuery.map()
- jQuery jQuery.merge()
- jQuery jQuery.noop()
- jQuery jQuery.now()
- jQuery jQuery.parseHTML()
- jQuery jQuery.parseJSON()
- jQuery jQuery.parseXML()
- jQuery jQuery.proxy()
- jQuery jQuery.queue()
- jQuery jQuery.removeData()
- jQuery jQuery.support
- jQuery jQuery.trim()
- jQuery jQuery.type()
- jQuery jQuery.unique()
- jQuery jQuery.uniqueSort()
- jQuery .queue()
- jQuery .uniqueSort()
jQuery Property
jQuery HTML
- jQuery .after()
- jQuery .append()
- jQuery .appendTo()
- jQuery .attr()
- jQuery .before()
- jQuery .clone()
- jQuery .data()
- jQuery .detach()
- jQuery .empty()
- jQuery .hasData()
- jQuery .html()
- jQuery .htmlPrefilter()
- jQuery .index()
- jQuery .insertAfter()
- jQuery .insertBefore()
- jQuery .prepend()
- jQuery .prependTo()
- jQuery .prop()
- jQuery .pushStack()
- jQuery .remove()
- jQuery .removeAttr()
- jQuery .removeData()
- jQuery .removeProp()
- jQuery .replaceAll()
- jQuery .replaceWith()
- jQuery .size()
- jQuery .text()
- jQuery .toArray()
- jQuery .unwrap()
- jQuery .val()
- jQuery .wrap()
- jQuery .wrapAll()
- jQuery .wrapInner()
jQuery CSS
- jQuery .addClass()
- jQuery .animate()
- jQuery .css()
- jQuery .delay()
- jQuery .finish()
- jQuery .hasClass()
- jQuery .height()
- jQuery .hide()
- jQuery .innerHeight()
- jQuery .innerWidth()
- jQuery jQuery.cssHooks
- jQuery jQuery.cssNumber
- jQuery jQuery.escapeSelector()
- jQuery .offset()
- jQuery .outerHeight()
- jQuery .outerWidth()
- jQuery .position()
- jQuery .removeClass()
- jQuery .resize()
- jQuery .scroll()
- jQuery .scrollLeft()
- jQuery .scrollTop()
- jQuery .show()
- jQuery .slideDown()
- jQuery .slideToggle()
- jQuery .slideUp()
- jQuery .stop()
- jQuery .sub()
- jQuery .toggleClass()
- jQuery .width()
jQuery Miscellaneous
jQuery jQuery.post() Method

Photo Credit to CodeToFun
🙋 Introduction
jQuery provides a variety of methods to facilitate AJAX requests, making it easier to handle asynchronous data exchange between the client and server. One of the most commonly used methods for making HTTP POST requests is jQuery.post()
. This method simplifies the process of sending data to the server and handling the response, which is crucial for creating dynamic, data-driven web applications.
In this guide, we will explore the jQuery.post()
method in detail, with examples to illustrate its usage.
🧠 Understanding jQuery.post() Method
The jQuery.post()
method is a shorthand version of the jQuery.ajax() method, designed specifically for making HTTP POST requests. It sends data to the server and retrieves the server's response in a more concise and readable way.
💡 Syntax
The syntax for the jQuery.post()
method is straightforward:
$.post(url, data, success, dataType)
Parameters:
- url: The URL to which the request is sent.
- data: A plain object or string that is sent to the server with the request.
- success: A callback function that is executed if the request succeeds.
- dataType (optional): The type of data expected from the server (e.g., "json", "xml", "html").
📝 Example
Basic Usage:
Let's start with a basic example where we send a POST request to a server endpoint and handle the response.
index.htmlCopied<button id="submitBtn">Submit</button> <div id="response"></div>
example.jsCopied$("#submitBtn").click(function() { $.post("https://example.com/api/submit", { name: "John", age: 30 }, function(response) { $("#response").html("Server Response: " + response); }); });
In this example, when the button is clicked, a POST request is sent to https://example.com/api/submit with the data { name: "John", age: 30 }. The server's response is then displayed in the #response div.
Handling JSON Responses:
Often, servers respond with JSON data. Here's how to handle JSON responses using
jQuery.post()
.index.htmlCopied<button id="fetchDataBtn">Fetch Data</button> <div id="userData"></div>
example.jsCopied$("#fetchDataBtn").click(function() { $.post("https://example.com/api/user", { userId: 1 }, function(data) { $("#userData").html("User Name: " + data.name + "<br>Age: " + data.age); }, "json"); });
In this example, the server's JSON response is automatically parsed, and the user data is displayed in the #userData div.
Error Handling:
It's essential to handle errors that may occur during the AJAX request. You can achieve this by chaining the .fail() method.
index.htmlCopied<button id="errorHandlingBtn">Test Error Handling</button> <div id="errorMessage"></div>
example.jsCopied$("#errorHandlingBtn").click(function() { $.post("https://example.com/api/test", { test: "data" }) .done(function(response) { $("#errorMessage").html("Success: " + response); }) .fail(function(xhr, status, error) { $("#errorMessage").html("Error: " + xhr.status + " - " + error); }); });
Here, if the request fails, an error message is displayed in the #errorMessage div.
Sending Form Data:
The
jQuery.post()
method can also be used to send form data. This example demonstrates how to serialize form data and send it via a POST request.index.htmlCopied<form id="userForm"> Name: <input type="text" name="name"><br> Age: <input type="text" name="age"><br> <input type="submit" value="Submit"> </form> <div id="formResponse"></div>
example.jsCopied$("#userForm").submit(function(event) { event.preventDefault(); $.post("https://example.com/api/formSubmit", $(this).serialize(), function(response) { $("#formResponse").html("Server Response: " + response); }); });
In this example, the form data is serialized and sent to the server when the form is submitted, and the server's response is displayed in the #formResponse div.
🎉 Conclusion
The jQuery.post()
method is a convenient and powerful tool for making HTTP POST requests. Whether you are sending simple data, handling JSON responses, managing errors, or submitting form data, this method simplifies the process and enhances your ability to create dynamic web applications.
By mastering jQuery.post()
, you can improve the interactivity and responsiveness of your web pages.
👨💻 Join our Community:
Author
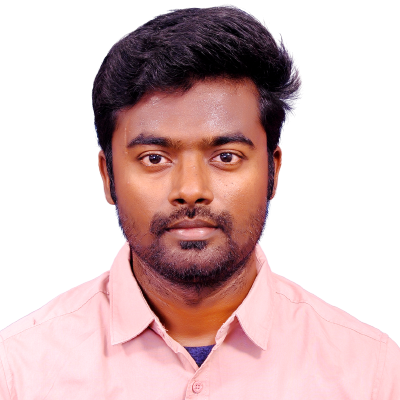
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.post() Method), please comment here. I will help you immediately.