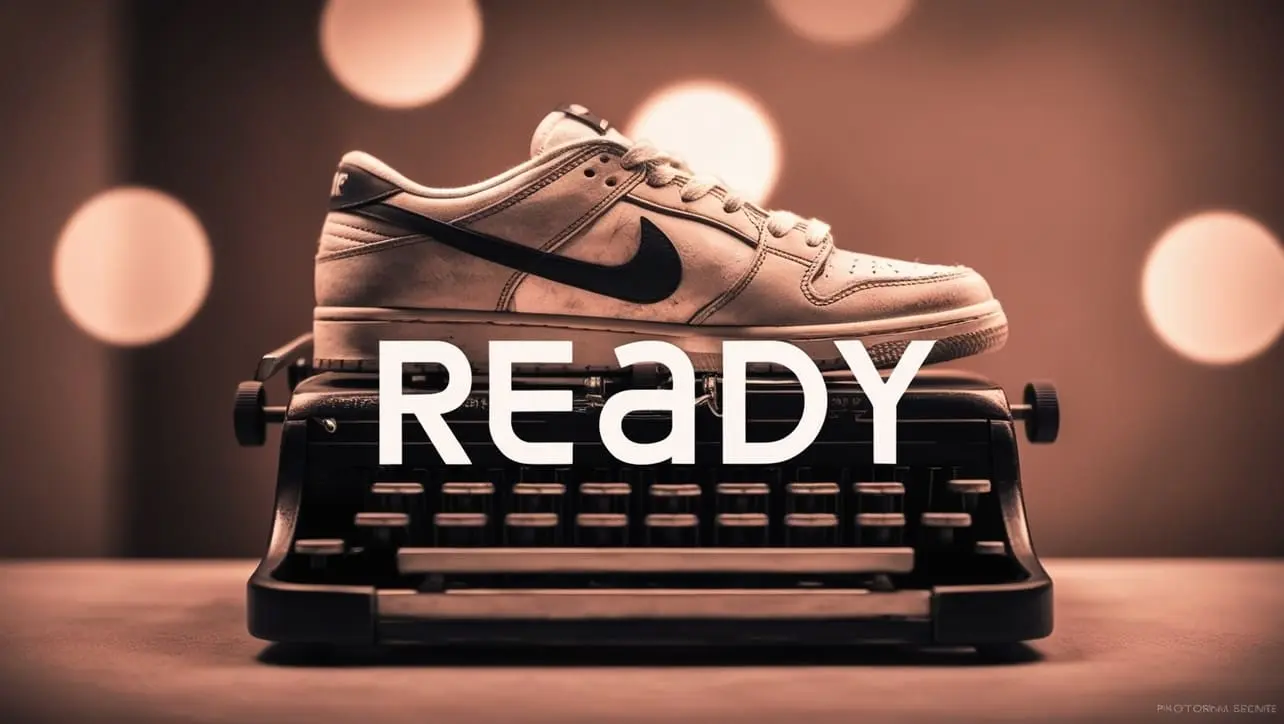
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .html() Method
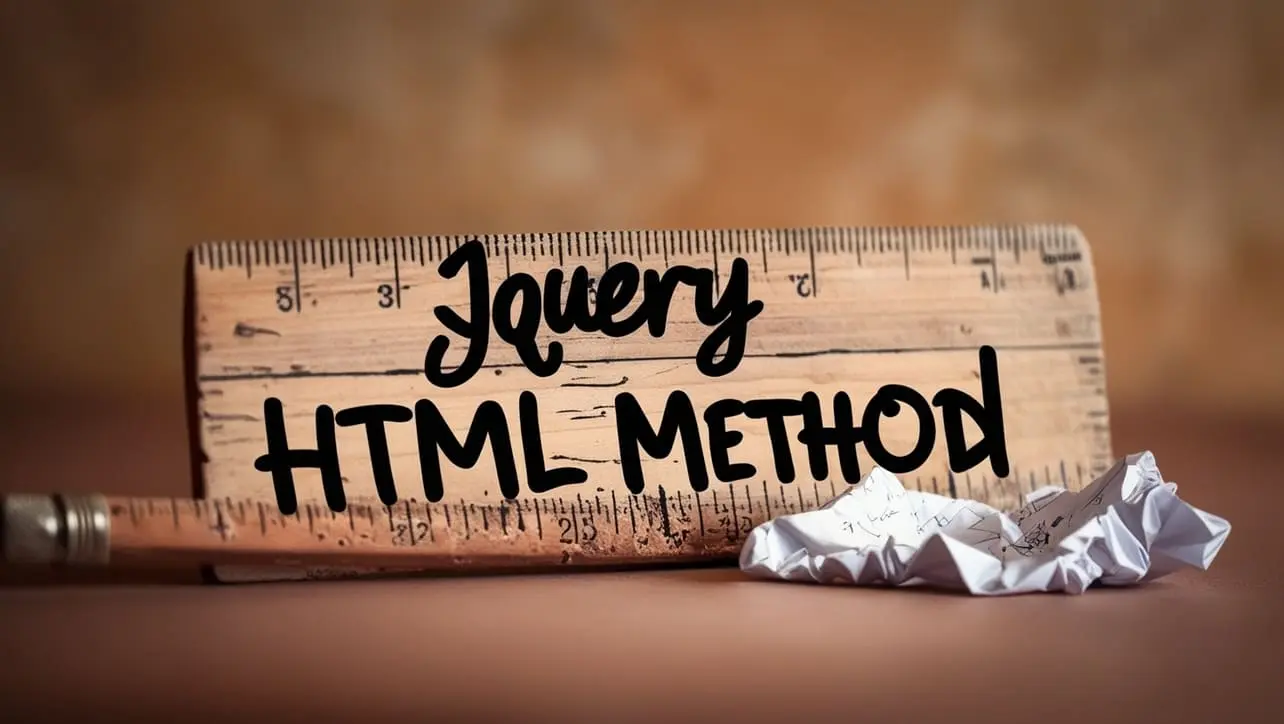
Photo Credit to CodeToFun
🙋 Introduction
In web development, manipulating HTML content dynamically is a common task. jQuery simplifies this process with its .html()
method, allowing you to get or set the HTML content of elements effortlessly. Understanding and mastering this method can greatly enhance your ability to create dynamic and interactive web pages.
In this guide, we'll explore the usage of the jQuery .html()
method with clear examples to help you grasp its full potential.
🧠 Understanding .html() Method
The .html()
method in jQuery is used to get or set the HTML content of an element. It can be applied to any HTML element, including divs, paragraphs, spans, and more. This method is particularly useful when you need to dynamically update or retrieve HTML content within your web page.
💡 Syntax
The syntax for the .html()
method is straightforward:
// Get HTML content
$(selector).html()
// Set HTML content
$(selector).html(htmlContent)
📝 Example
Getting HTML Content:
To retrieve the HTML content of an element, simply use the
.html()
method without passing any parameters. For example:index.htmlCopied<div id="content"> <p>This is some <strong>bold</strong> text.</p> </div>
example.jsCopiedvar htmlContent = $("#content").html(); console.log(htmlContent);
This will log the HTML content of the <div> element to the console.
Setting HTML Content:
To set the HTML content of an element, pass the desired HTML content as a parameter to the
.html()
method. For example:index.htmlCopied<div id="content"> <p>This is some <strong>bold</strong> text.</p> </div>
example.jsCopied$("#content").html("<p>New HTML content</p>");
This will replace the existing HTML content of the <div> element with the specified content.
Dynamic Content Generation:
The
.html()
method is often used to generate dynamic content based on user interactions or data from an external source. For instance:index.htmlCopied<div id="dynamic-content"></div>
example.jsCopiedvar dynamicContent = "<ul>"; for (var i = 1; i <= 5; i++) { dynamicContent += "<li>Item " + i + "</li>"; } dynamicContent += "</ul>"; $("#dynamic-content").html(dynamicContent);
This will generate a list of items dynamically and insert it into the <div> element with the ID dynamic-content.
Escaping HTML Entities:
When setting HTML content dynamically, be cautious of potential security vulnerabilities such as cross-site scripting (XSS) attacks. Use proper escaping techniques or consider using other jQuery methods like .text() if HTML formatting is not required.
🎉 Conclusion
The jQuery .html()
method is a powerful tool for dynamically updating and retrieving HTML content within web pages. Whether you need to retrieve content, set content dynamically, or generate HTML dynamically based on user interactions or data, this method provides a convenient and efficient solution.
By mastering its usage, you can create more dynamic and interactive web pages with ease.
👨💻 Join our Community:
Author
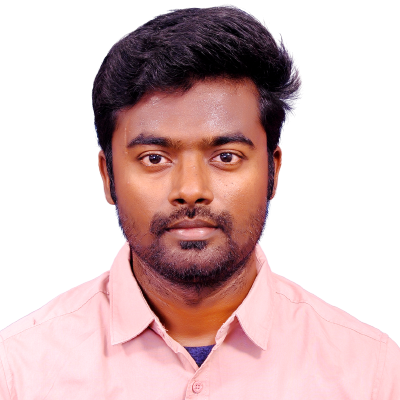
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .html() Method), please comment here. I will help you immediately.