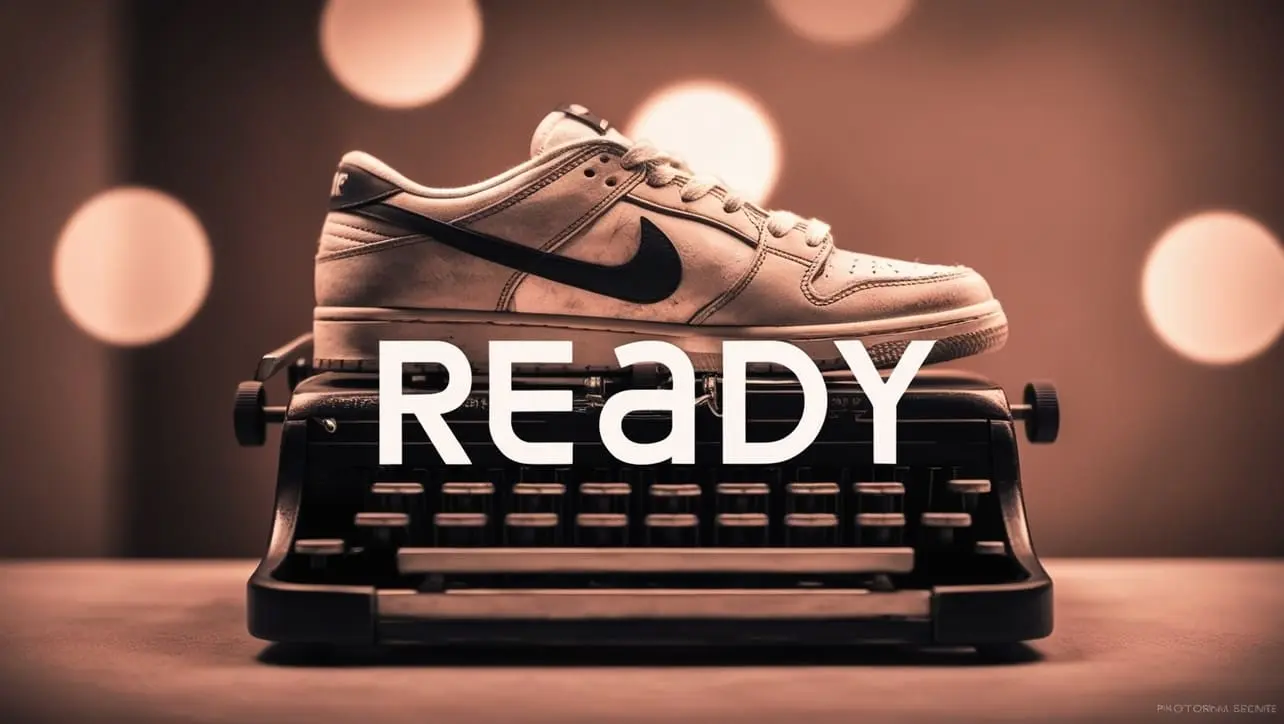
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .hide() Method
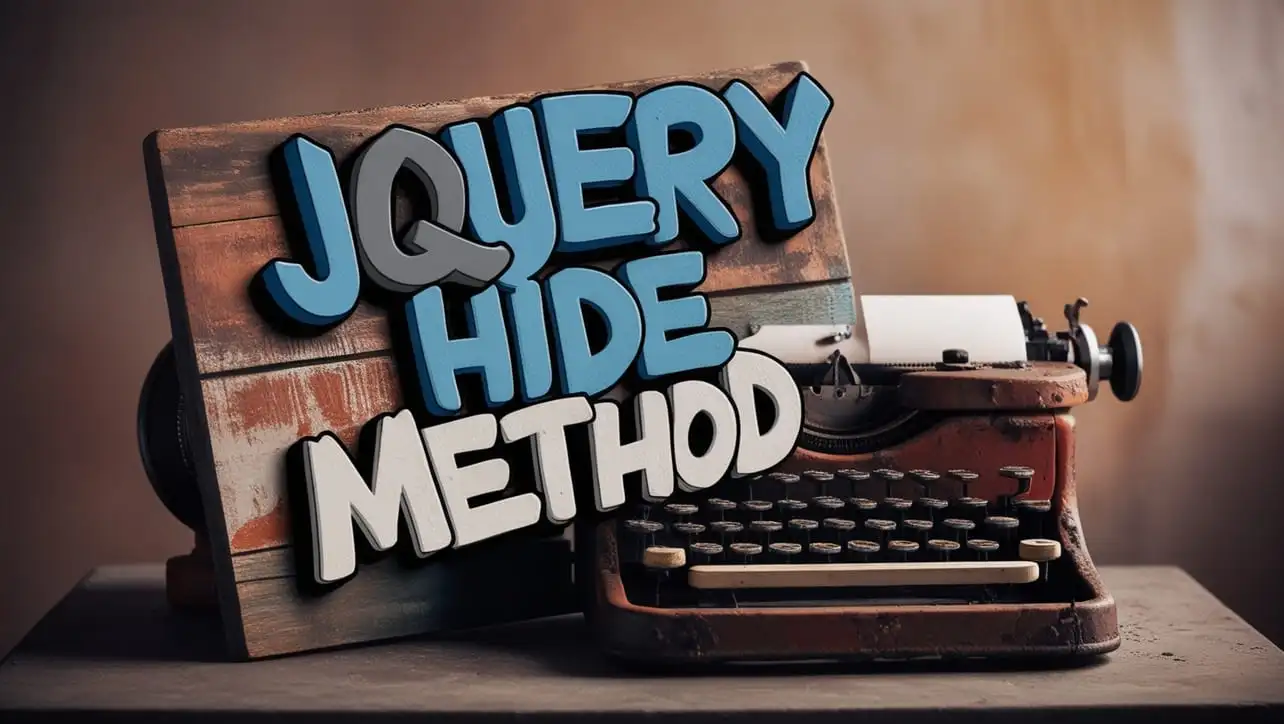
Photo Credit to CodeToFun
Introduction
jQuery is renowned for its simplicity and effectiveness in manipulating HTML elements and enhancing interactivity on web pages. Among its arsenal of methods, the .hide()
method stands out as a straightforward solution for hiding elements dynamically.
In this comprehensive guide, we'll delve into the details of the jQuery .hide()
method, providing clear examples and practical applications to help you harness its power effectively.
Understanding .hide() Method
The .hide()
method in jQuery is used to hide selected elements by adjusting their display property. It offers a quick and convenient way to conceal elements on a web page, making them invisible while still occupying space in the layout.
Syntax
The syntax for the .hide()
method is straightforward:
$(selector).hide(speed, easing, callback);
Parameters:
- speed (optional): Specifies the speed of the hiding animation in milliseconds or predefined strings like "slow" or "fast".
- easing (optional): Specifies the easing function to use for the animation.
- callback (optional): A function to execute once the hiding animation is complete.
Example
Hiding Elements Instantly:
To hide an element instantly without any animation, simply call the
.hide()
method without specifying any parameters:example.jsCopied$("#elementToHide").hide();
Adding Animation Effects:
You can add animation effects to the hiding process by specifying the speed parameter. For instance, to hide an element with a slow animation:
example.jsCopied$("#elementToHide").hide("slow");
This will gradually fade out the element over a period of time.
Custom Easing Function:
jQuery allows you to define custom easing functions for animations. Here's an example of hiding an element with a custom easing function named "easeInOutExpo":
example.jsCopied$("#elementToHide").hide(1000, "easeInOutExpo");
Callback Function:
You can execute a function once the hiding animation is complete by passing a callback function. For example, to display an alert after hiding an element:
example.jsCopied$("#elementToHide").hide(500, function() { alert("Element is now hidden!"); });
Creating Toggle Effects:
The
.hide()
method is often used in combination with .show() or .toggle() to create toggle effects, allowing users to show or hide elements with a single click. For instance:example.jsCopied$("#toggleButton").click(function() { $("#elementToToggle").toggle(); });
Preparing Elements for Dynamic Display:
In dynamic web applications, elements may need to be hidden initially and then displayed based on user interactions or application logic. The
.hide()
method facilitates this by hiding elements during the page load or initialization phase.
Conclusion
The jQuery .hide()
method provides a simple yet powerful way to hide elements on a web page, whether for aesthetic purposes, user interactions, or dynamic content management. With its versatile parameters and seamless integration with other jQuery methods, .hide()
empowers developers to create engaging and interactive web experiences effortlessly.
By mastering its usage and exploring its various applications, you can elevate the quality and functionality of your web projects significantly.
Join our Community:
Author
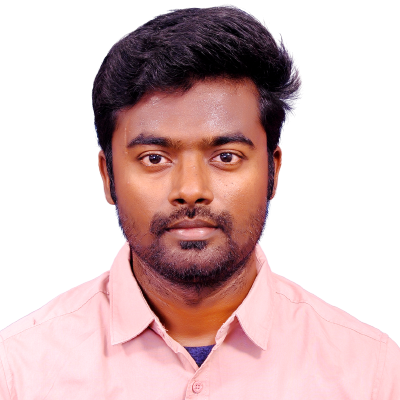
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .hide() Method), please comment here. I will help you immediately.