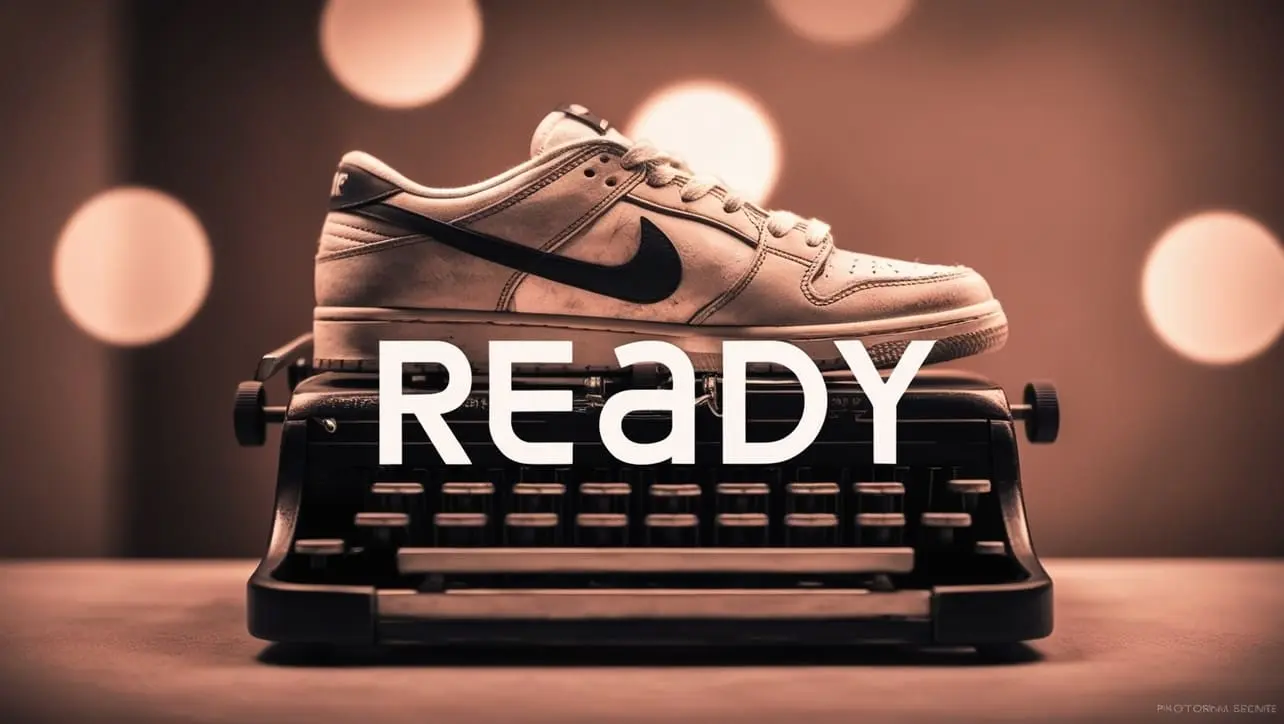
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .find() Method
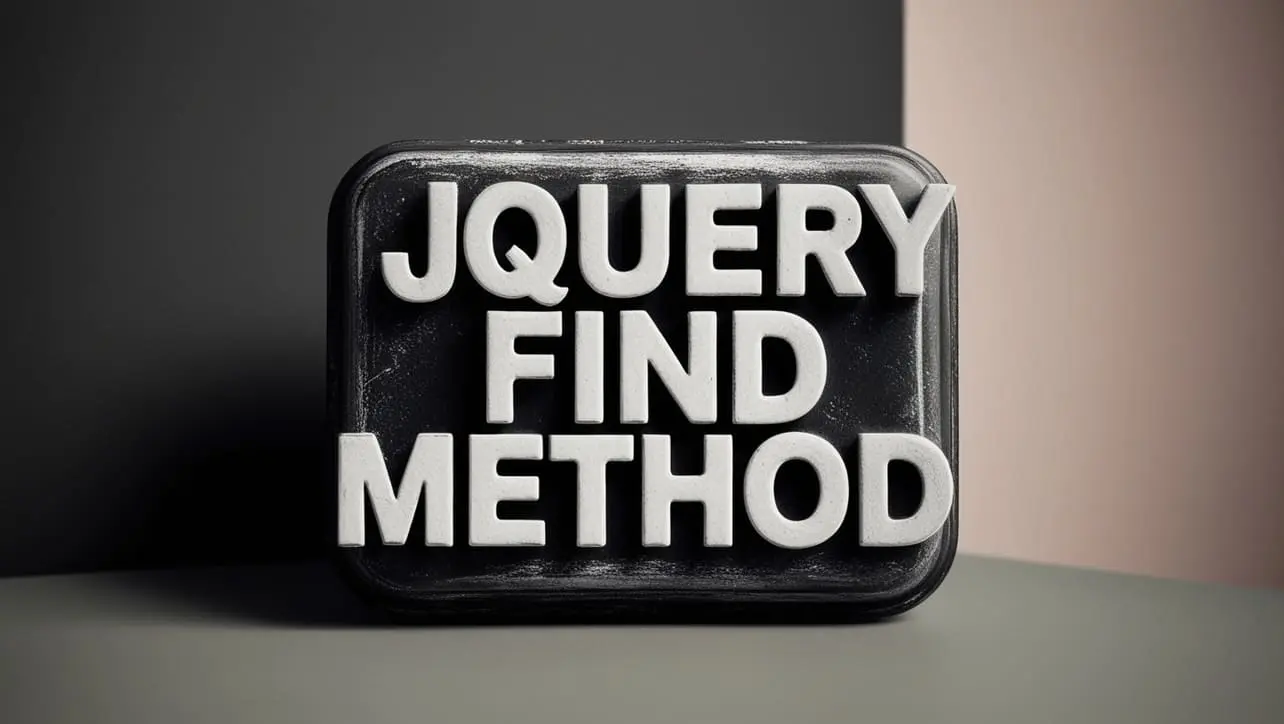
Photo Credit to CodeToFun
🙋 Introduction
In jQuery, the .find()
method is a versatile tool that allows you to search for descendant elements within a selected set of elements. Whether you're navigating through a complex DOM structure or targeting specific elements within a container, mastering the .find()
method can greatly streamline your jQuery code.
In this guide, we'll dive into the functionality of the .find()
method with practical examples to help you harness its power effectively.
🧠 Understanding .find() Method
The .find()
method in jQuery is used to search for descendant elements that match a specified selector within the selected elements. It traverses down the DOM tree, starting from the selected elements, and retrieves all matching descendants.
💡 Syntax
The syntax for the .find()
method is straightforward:
$(selector).find(filter)
📝 Example
Basic Usage:
Suppose you have an HTML structure with nested elements, and you want to find all <span> elements within a <div> with the class .container. You can achieve this using the
.find()
method as follows:index.htmlCopied<div class="container"> <p>This is a paragraph.</p> <span>This is a span.</span> </div>
example.jsCopied$(".container").find("span").css("color", "blue");
This will change the text color of the <span> element within the .container <div> to blue.
Chaining Methods:
The
.find()
method can be chained with other jQuery methods to perform more complex operations. For instance, let's find all <a> elements within a <div> and add a click event handler to them:index.htmlCopied<div id="container"> <a href="#">Link 1</a> <a href="#">Link 2</a> </div>
example.jsCopied$("#container").find("a").click(function() { alert("Link clicked!"); });
This will display an alert message when any <a> element within the #container <div> is clicked.
Searching for Specific Descendants:
You can use more specific selectors with the
.find()
method to narrow down your search. For example, let's find all <input> elements with the class .checkbox within a <form>:index.htmlCopied<form id="myForm"> <input type="text" name="username"> <input type="checkbox" class="checkbox"> </form>
example.jsCopied$("#myForm").find("input.checkbox").prop("checked", true);
This will check the checkbox within the #myForm <form>.
Contextual Searching:
You can limit the search scope of the
.find()
method by providing a context. This allows you to search for descendants within a specific element rather than the entire document. For example:example.jsCopiedvar $container = $(".container"); $("span", $container).css("font-weight", "bold");
This will make all <span> elements within the $container element bold.
🎉 Conclusion
The jQuery .find()
method is a powerful tool for searching and manipulating descendant elements within a selected set. Whether you're navigating through complex DOM structures, chaining methods for advanced operations, or searching for specific descendants, the .find()
method provides a convenient and efficient solution.
By incorporating it into your jQuery workflow, you can enhance the functionality and readability of your code significantly.
👨💻 Join our Community:
Author
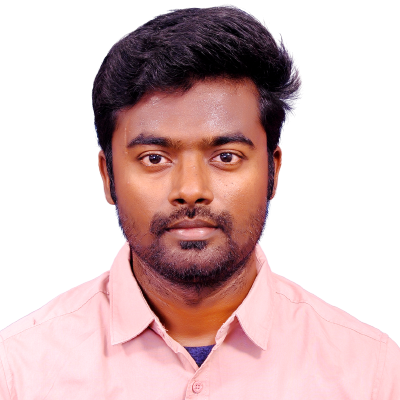
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .find() Method), please comment here. I will help you immediately.