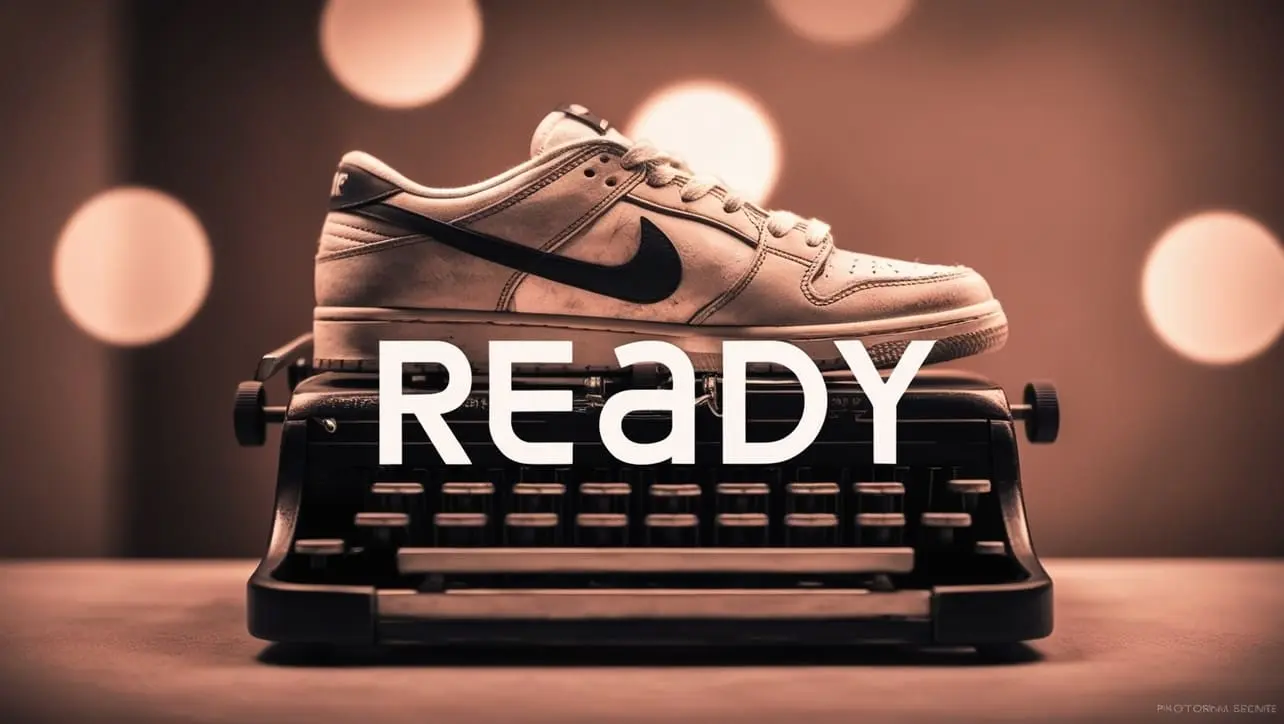
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery event.stopPropagation() Method
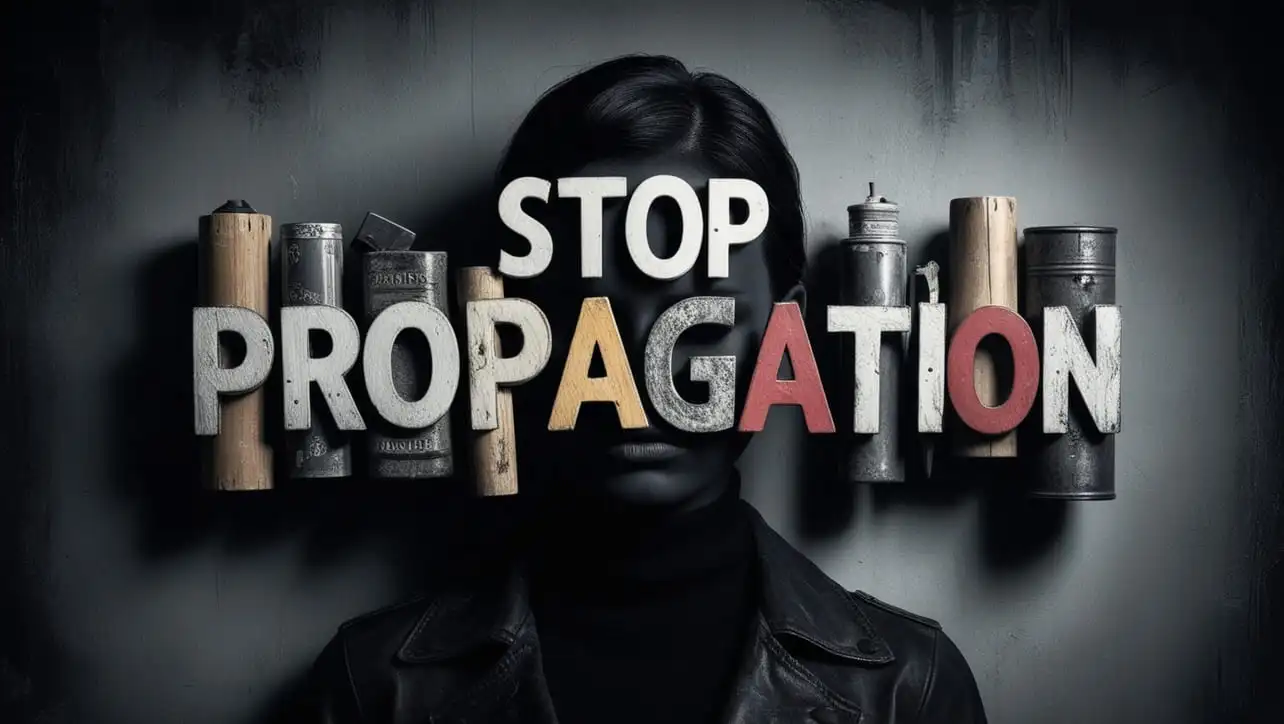
Photo Credit to CodeToFun
Introduction
In jQuery, handling events efficiently is crucial for creating dynamic and interactive web applications. One of the essential methods in event handling is event.stopPropagation()
. This method plays a vital role in controlling the event flow, ensuring that events do not bubble up to parent elements unnecessarily.
In this guide, we'll explore the event.stopPropagation()
method with clear examples to help you understand its functionality and use cases.
Understanding event.stopPropagation() Method
The event.stopPropagation()
method is used to prevent the event from propagating (or bubbling) up the DOM tree. When an event occurs on an element, it normally bubbles up to all its ancestors. By using this method, you can stop this behavior and ensure that the event is handled only by the target element.
Syntax
The syntax for the event.stopPropagation()
method is straightforward:
event.stopPropagation()
Example
Basic Example: Stopping Event Propagation:
Consider a scenario where you have nested elements, and you want to prevent a click event from bubbling up from a child element to its parent:
index.htmlCopied<div id="parent"> Parent Div <button id="child">Child Button</button> </div>
example.jsCopied$("#child").click(function(event) { alert("Child button clicked."); event.stopPropagation(); }); $("#parent").click(function() { alert("Parent div clicked."); });
In this example, clicking the child button will alert "Child button clicked." but will not trigger the parent div's click event, thanks to
event.stopPropagation()
.Practical Use Case: Handling Specific Events:
This method is particularly useful in complex UIs where multiple layers of elements might have event listeners. For instance, in a dropdown menu, you might want to handle clicks on menu items without triggering the dropdown's parent container events:
index.htmlCopied<div id="dropdown"> <ul> <li class="menu-item">Item 1</li> <li class="menu-item">Item 2</li> <li class="menu-item">Item 3</li> </ul> </div>
example.jsCopied$(".menu-item").click(function(event) { alert($(this).text() + " clicked."); event.stopPropagation(); }); $("#dropdown").click(function() { alert("Dropdown container clicked."); });
Clicking on any menu item will show an alert with the item text, but it won't trigger the alert for the dropdown container.
Combining with Other Event Methods:
You can use
event.stopPropagation()
in conjunction with other event methods such as event.preventDefault() to enhance control over event behavior:index.htmlCopied<a href="https://example.com" id="exampleLink">Example Link</a>
example.jsCopied$("#exampleLink").click(function(event) { alert("Link clicked but default action prevented."); event.preventDefault(); event.stopPropagation(); });
This will prevent the default action of navigating to the link and stop the event from bubbling up.
Conclusion
The jQuery event.stopPropagation()
method is a powerful tool for controlling event flow in your web applications. By preventing events from bubbling up the DOM tree, you can ensure that your event handlers execute only where needed, enhancing the precision and performance of your code.
Understanding and utilizing this method effectively allows you to create more responsive and well-behaved web interfaces.
Join our Community:
Author
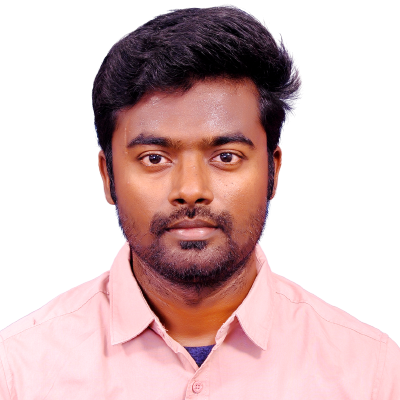
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery event.stopPropagation() Method), please comment here. I will help you immediately.