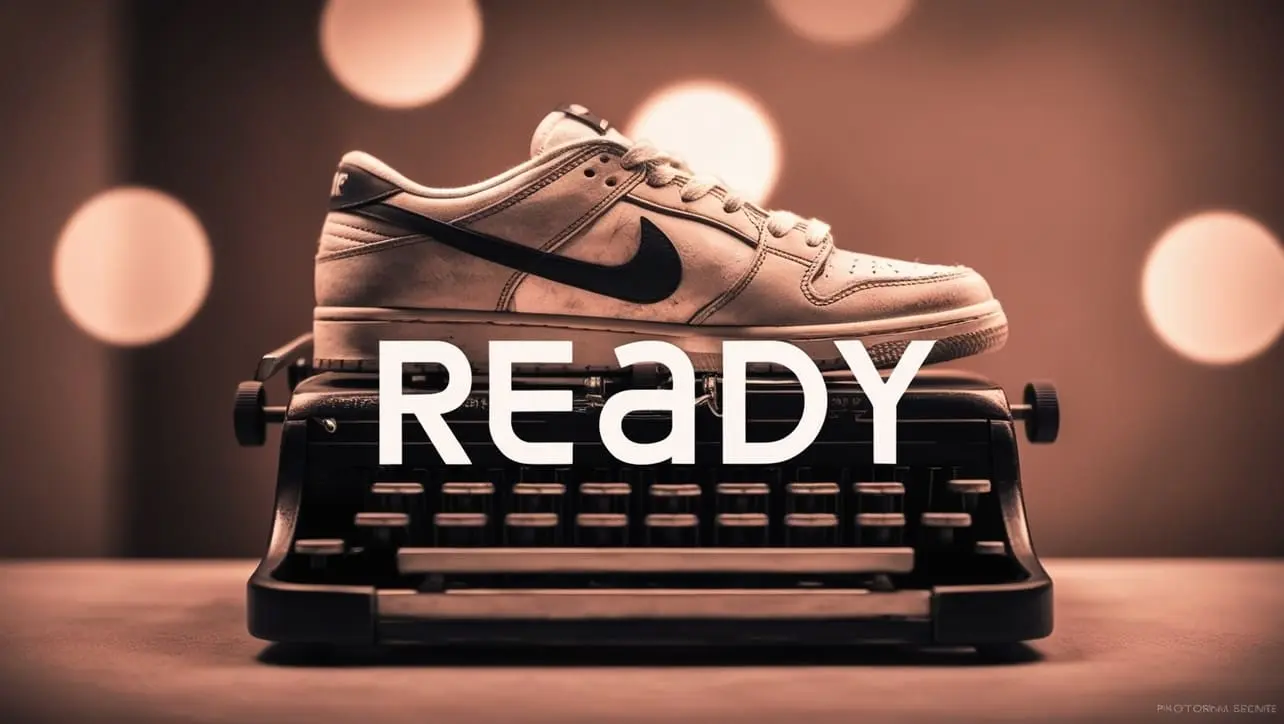
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery mouseover Event
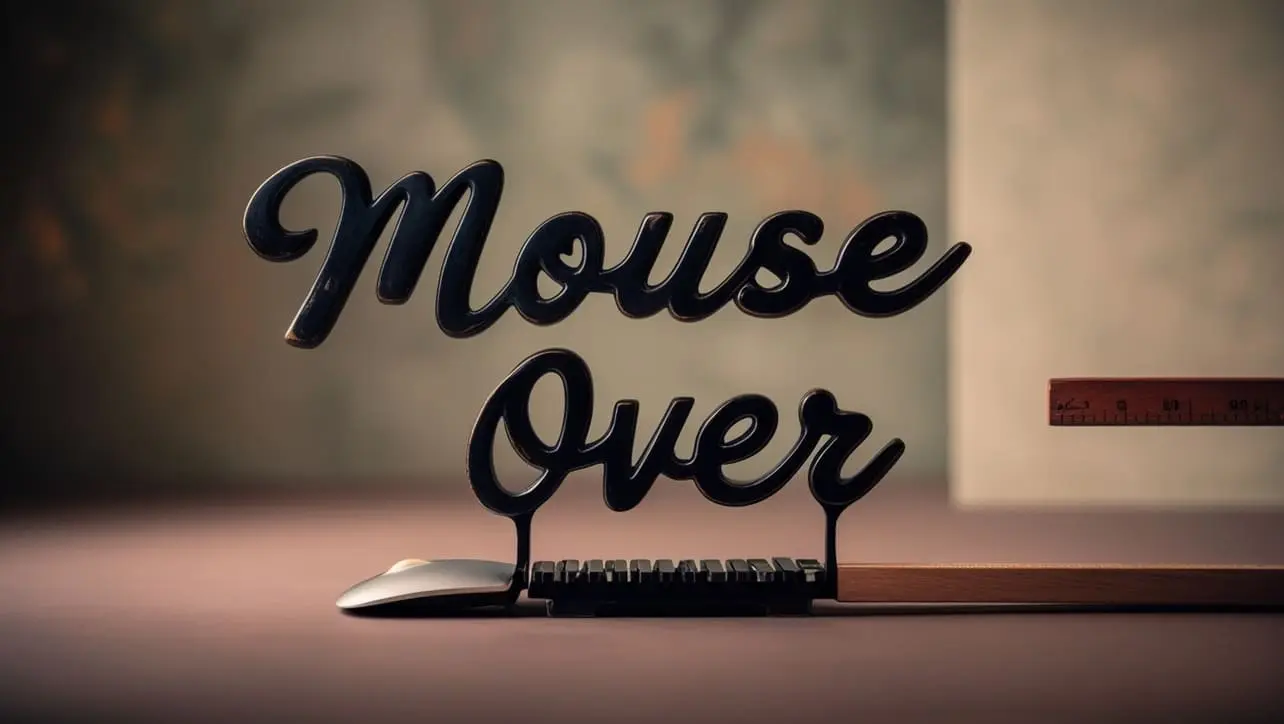
Photo Credit to CodeToFun
🙋 Introduction
jQuery simplifies web development by providing powerful tools for handling events and creating interactive user experiences. One such event is the mouseover
event, which occurs when the mouse pointer enters the boundaries of an element. In this guide, we'll explore how to effectively use the jQuery mouseover
event to enhance your web pages.
It's worth noting that the .mouseover() method has been deprecated in favor of .on(), which offers more flexibility and consistency.
🧠 Understanding mouseover Event
The jQuery mouseover
event is triggered when the mouse pointer enters the boundaries of an element. It is commonly used to implement hover effects, tooltips, and interactive elements on web pages.
💡 Syntax
The syntax for the mouseover
event is straightforward:
$(selector).on("mouseover", [eventData], handler)
Parameters:
- selector: The selector for the element(s) to which the event handler will be attached.
- eventData (optional): Additional data to pass to the event handler.
- handler: The function to execute when the
mouseover
event is triggered.
📝 Example
Implementing Hover Effects:
You can use the
mouseover
event to add hover effects to elements. For example, let's change the background color of a <div> when the mouse is hovered over it:index.htmlCopied<div id="hoverDiv" style="width: 200px; height: 100px; background-color: #f0f0f0;"></div>
example.jsCopied$("#hoverDiv").on("mouseover", function() { $(this).css("background-color", "#ffcc00"); });
This will change the background color of the <div> to yellow when the mouse is hovered over it.
Creating Tooltips:
You can also use the
mouseover
event to display tooltips when the mouse hovers over specific elements. For instance, let's display a tooltip when hovering over a button:index.htmlCopied<button id="tooltipBtn">Hover Me</button> <div id="tooltip" style="display: none;">This is a tooltip!</div>
example.jsCopied$("#tooltipBtn").on("mouseover", function() { $("#tooltip").show(); }).on("mouseout", function() { $("#tooltip").hide(); });
This will show a tooltip with the text "This is a tooltip!" when hovering over the button and hide it when the mouse moves away.
Handling Dynamic Elements:
If you're working with dynamically added elements, you can still attach
mouseover
event handlers using event delegation. For example:example.jsCopied$("body").on("mouseover", ".dynamicElement", function() { // Handle mouseover event for dynamically added elements with class "dynamicElement" });
🎉 Conclusion
The jQuery mouseover
event is a versatile tool for creating interactive and engaging web pages. Whether you're implementing hover effects, tooltips, or other interactive elements, understanding how to effectively use the mouseover
event can greatly enhance user experience.
By utilizing the .on() method to attach event handlers, you ensure compatibility with modern jQuery practices while maintaining flexibility in handling mouseover
events.
👨💻 Join our Community:
Author
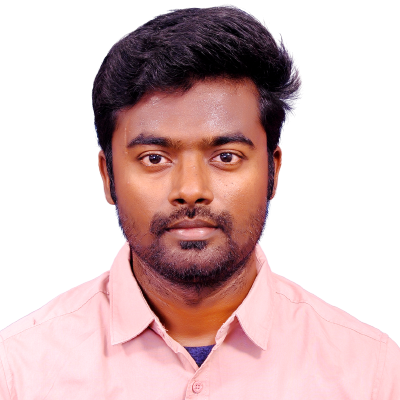
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery mouseover Event), please comment here. I will help you immediately.