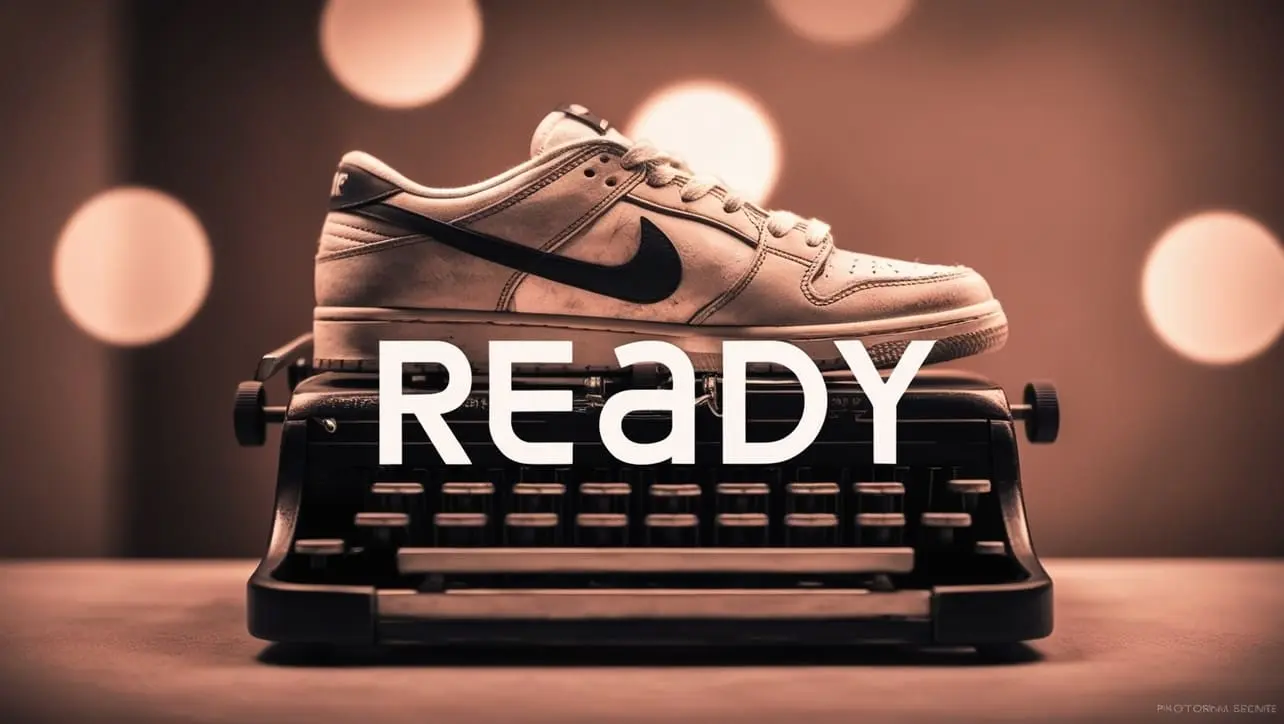
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery mousemove Event
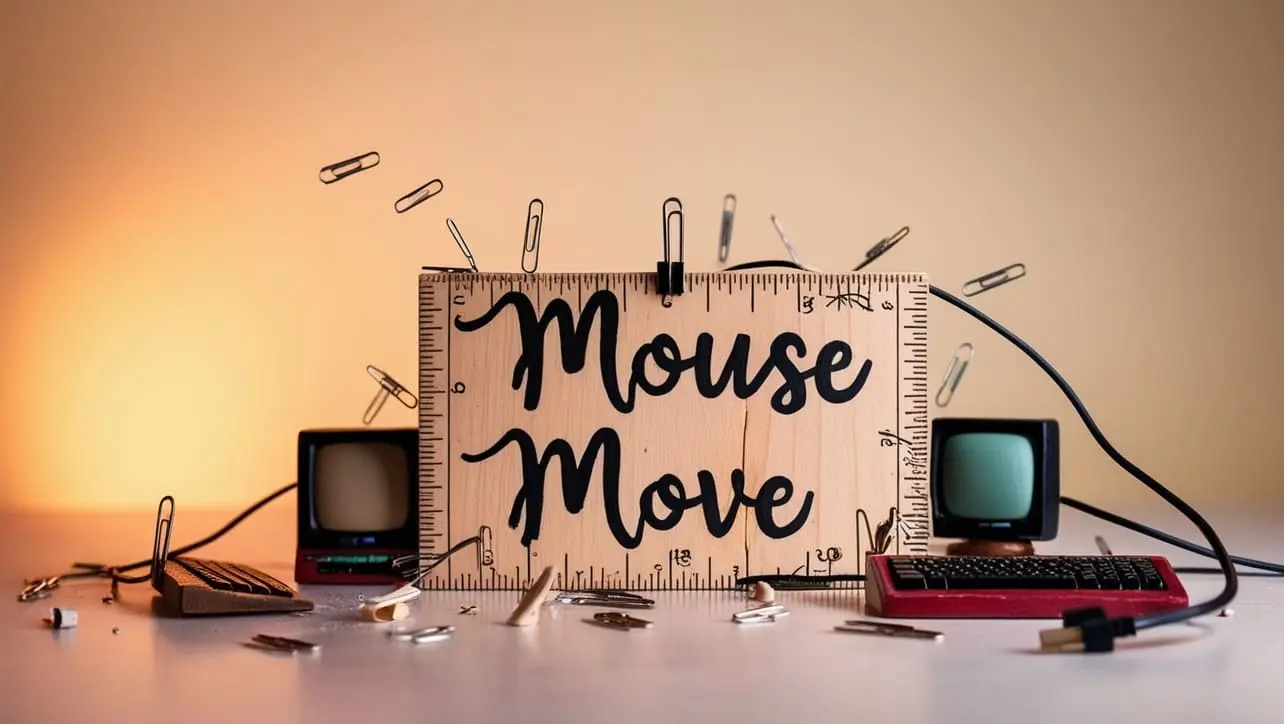
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a plethora of event handling functions to make web development more interactive and responsive. One such event is mousemove
, which is triggered when the mouse pointer is moved over an element. Although the .mousemove() method has been deprecated, you can achieve the same functionality using the .on() method.
In this guide, we'll explore the jQuery mousemove event, its syntax using .on(), and provide examples to demonstrate its practical usage.
🧠 Understanding mousemove Event
The mousemove
event occurs when the mouse pointer is moved over an element. It's commonly used to track the movement of the mouse and trigger actions accordingly. With jQuery's event handling capabilities, you can easily attach functions to this event and create dynamic interactions on your web page.
💡 Syntax
The syntax for the mousemove
event is straightforward:
$(selector).on("mousemove", handler);
or with optional event data:
$(selector).on("mousemove", eventData, handler);
📝 Example
Tracking Mouse Movement:
Suppose you want to track the mouse movement within a specific element and display the coordinates. You can achieve this by binding a
mousemove
event handler to that element:index.htmlCopied<div id="tracker" style="width: 300px; height: 200px; background-color: lightgray;"></div>
example.jsCopied$("#tracker").on("mousemove", function(event) { $("#coordinates").text("X: " + event.pageX + ", Y: " + event.pageY); });
This will display the current coordinates of the mouse pointer within the #tracker element.
Creating Interactive Effects:
You can use the
mousemove
event to create interactive effects, such as changing the background color of an element based on the mouse position:index.htmlCopied<div id="interactive" style="width: 300px; height: 200px; background-color: lightblue;"></div>
example.jsCopied$("#interactive").on("mousemove", function(event) { var x = event.pageX / $(this).width(); var y = event.pageY / $(this).height(); $(this).css("background-color", "rgb(" + (x * 255) + "," + (y * 255) + ", 100)"); });
This will change the background color of the #interactive element based on the mouse position, creating a dynamic color effect.
Managing Event Data:
You can pass additional data to the event handler using the .on() method. This can be useful for customizing behavior based on specific conditions or contexts.
🎉 Conclusion
The jQuery mousemove
event, although deprecated in its method form, remains a fundamental tool for creating interactive and dynamic web experiences. By leveraging the .on() method, you can easily attach event handlers to elements and respond to mouse movement with precision.
Whether you're tracking coordinates, creating interactive effects, or implementing custom behaviors, mastering the mousemove
event opens up a world of possibilities for enhancing user interaction on your web pages.
👨💻 Join our Community:
Author
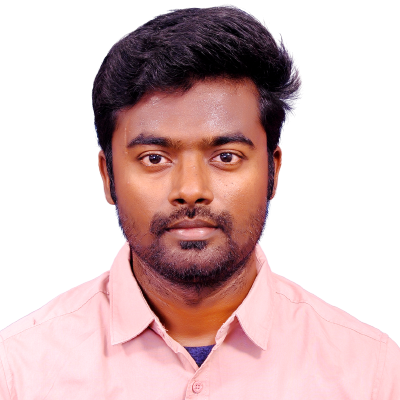
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery mousemove Event), please comment here. I will help you immediately.