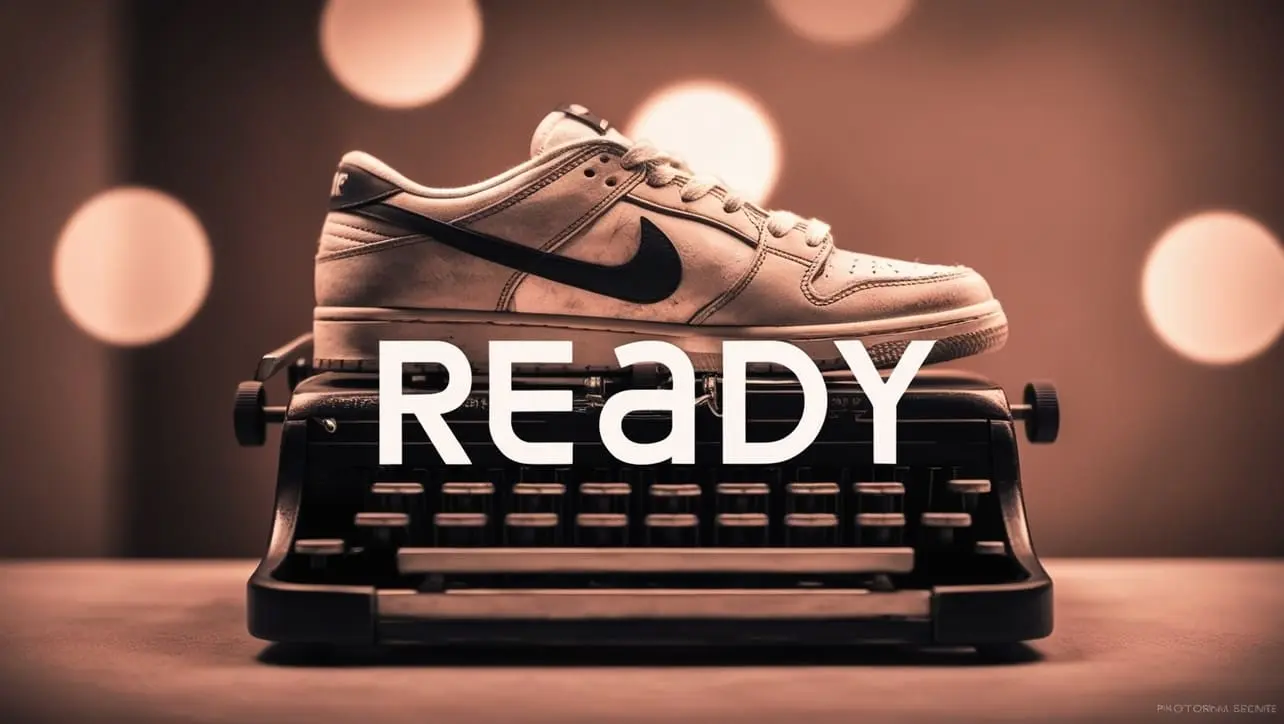
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery load Event
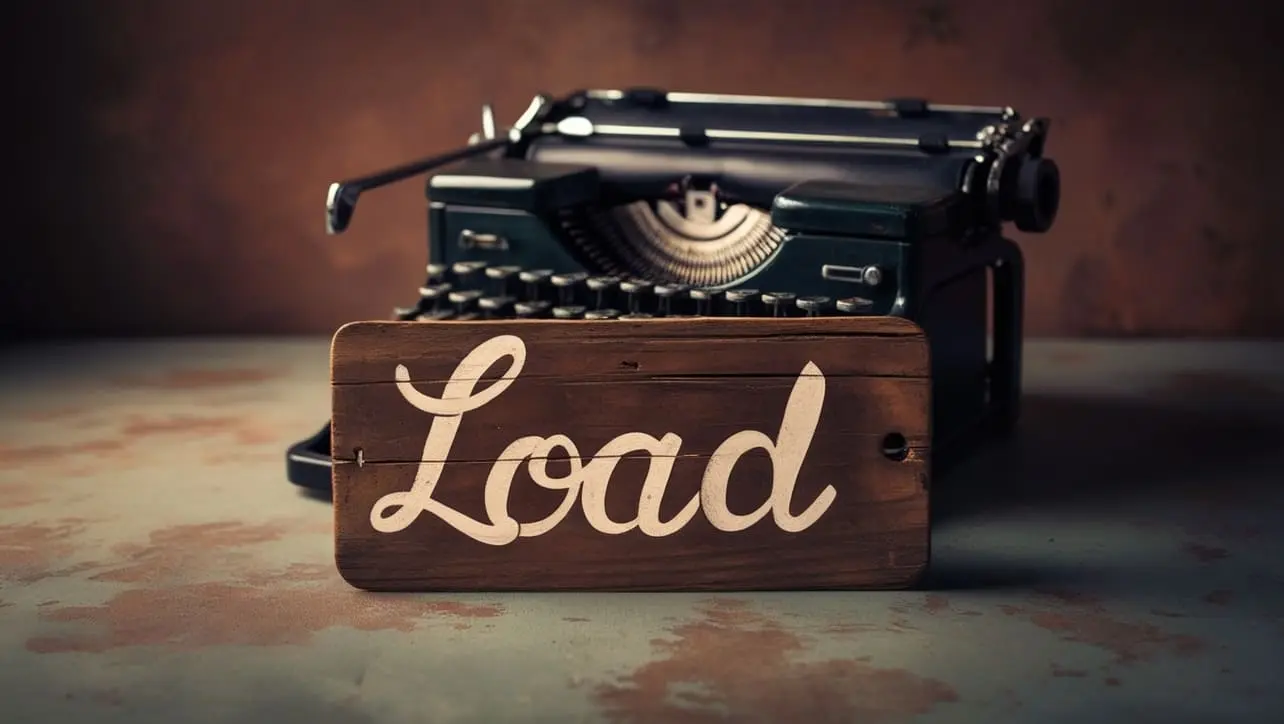
Photo Credit to CodeToFun
🙋 Introduction
In jQuery, the load
event was traditionally used to execute JavaScript code when a specific element or the entire document finishes loading. However, as of jQuery version 3.0, the .load() method has been deprecated in favor of the more versatile .on() method. Understanding how to use the .on() method with the load
event is essential for modern web development.
In this guide, we'll explore the usage of the jQuery load
event with the .on() method, providing clear examples to help you grasp its functionality effectively.
🧠 Understanding load Event
The .on() method in jQuery is a powerful event handling function that allows you to attach one or more event handlers to selected elements. It provides a flexible way to bind event handlers to elements, including handling the load
event.
💡 Syntax
The syntax for the load
event is straightforward:
.on( "load", [, eventData ], handler )
📝 Example
Handling Document Load Event:
You can use the .on() method to execute code when the entire document has finished loading. Here's how you can do it:
example.jsCopied$(document).on("load", function() { console.log("Document is fully loaded"); });
This code will log Document is fully loaded to the console when the document is loaded.
Binding Load Event to Specific Element:
If you want to execute code when a specific element has finished loading, you can target that element using its selector. For example:
example.jsCopied$("#image").on("load", function() { console.log("Image is loaded"); });
This code will log "Image is loaded" to the console when the element with ID image finishes loading, such as an <img> element.
Using Event Data:
You can also pass additional data to the event handler using the eventData parameter. This data will be available within the handler function. Here's an example:
example.jsCopied$(window).on("load", { message: "Window is loaded" }, function(event) { console.log(event.data.message); });
This code will log Window is loaded to the console when the window finishes loading.
Ensuring Event Binding After DOM Manipulation:
Since the .on() method allows event delegation, it's useful for dynamically added elements or elements that might not exist when the page initially loads. Ensure that you bind the event after any DOM manipulation to ensure proper functionality.
🎉 Conclusion
The jQuery load
event, when used with the .on() method, provides a robust mechanism for executing code when elements or the entire document finishes loading. Whether you need to handle the document load
event, bind load
events to specific elements, or pass additional data to event handlers, the .on() method offers a flexible and efficient solution.
By mastering its usage, you can create more responsive and interactive web applications.
👨💻 Join our Community:
Author
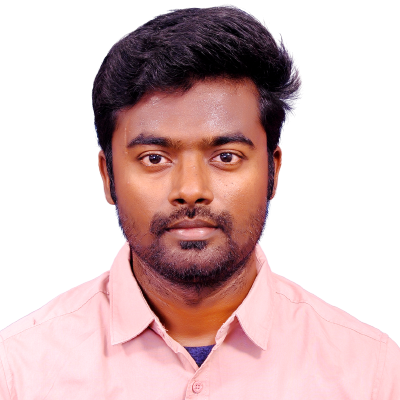
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery load Event), please comment here. I will help you immediately.