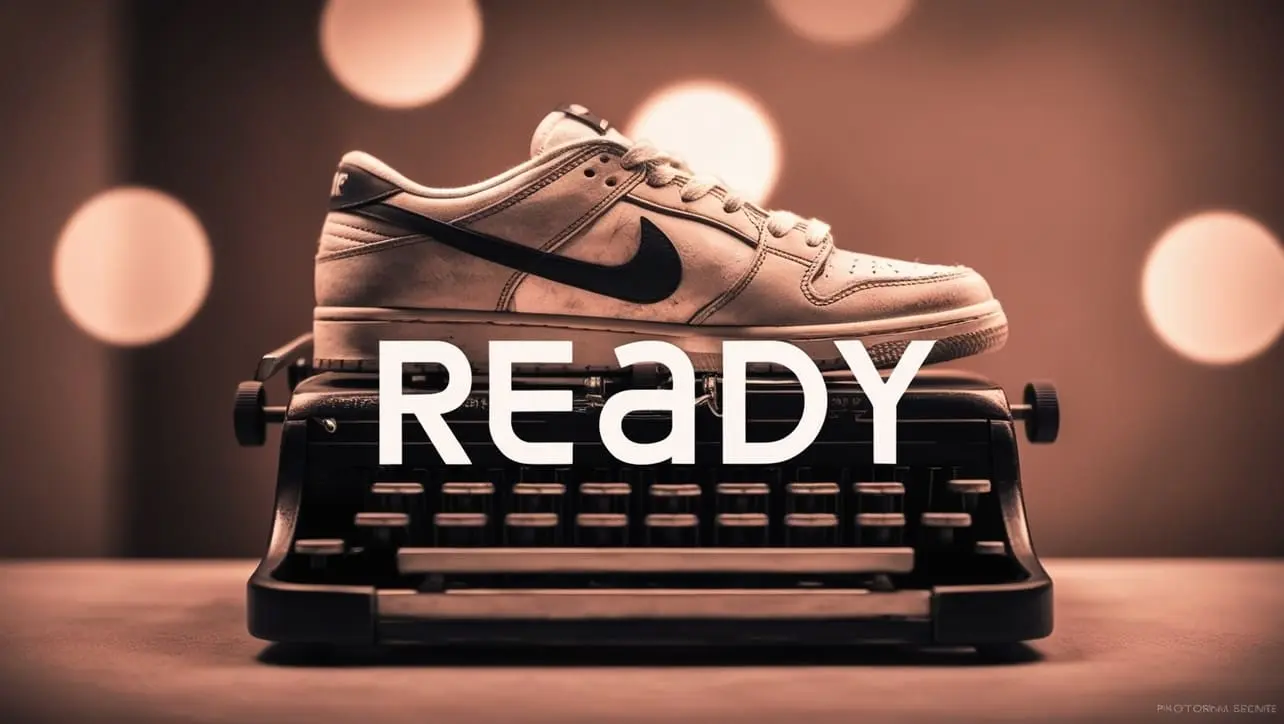
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery event.isImmediatePropagationStopped() Method
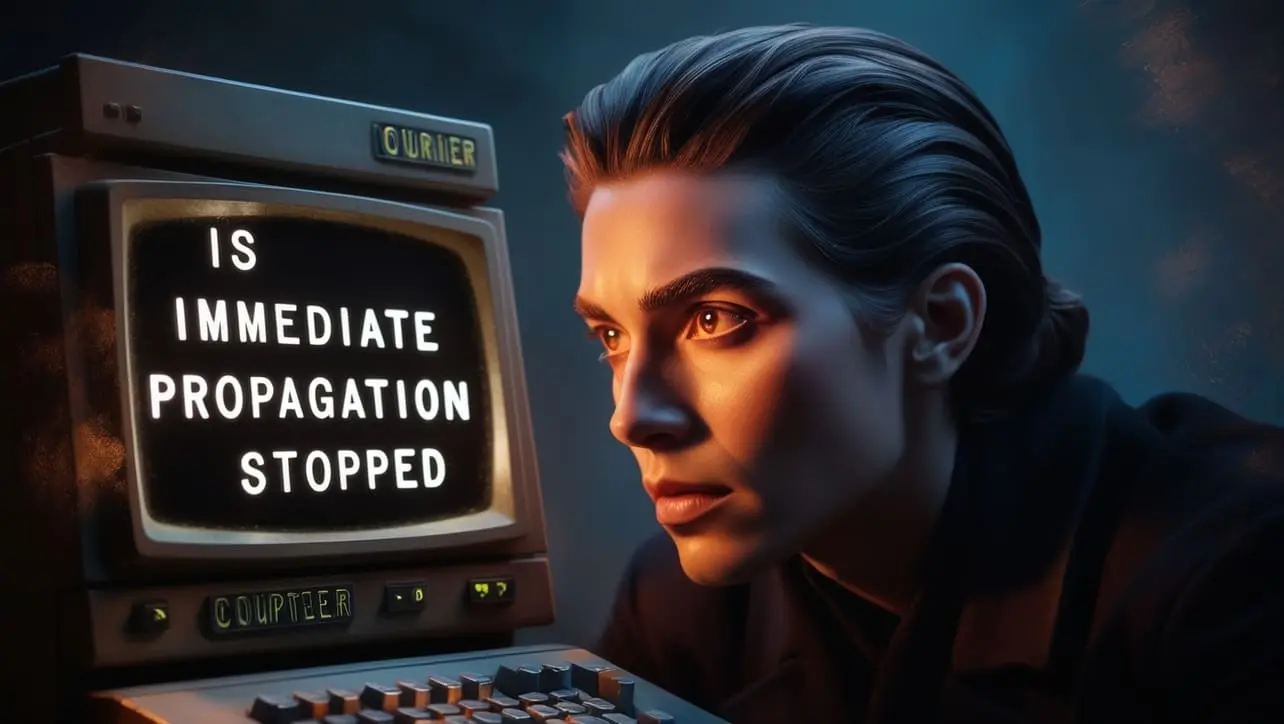
Photo Credit to CodeToFun
π Introduction
jQuery provides a rich set of methods for handling events, allowing developers to create responsive and interactive web applications. One such method is event.isImmediatePropagationStopped()
. This method is crucial for understanding event propagation in the context of event handling.
In this guide, weβll explore the event.isImmediatePropagationStopped()
method, its usage, and practical examples to demonstrate its functionality.
π§ Understanding event.isImmediatePropagationStopped() Method
The event.isImmediatePropagationStopped()
method in jQuery is used to determine whether the event.stopImmediatePropagation() method was called on an event. When stopImmediatePropagation() is invoked, it prevents other handlers from executing on the same element and also stops the event from propagating to other elements.
π‘ Syntax
The syntax for the event.isImmediatePropagationStopped()
method is straightforward:
event.isImmediatePropagationStopped()
This method returns a boolean value:
- true: if stopImmediatePropagation() was called.
- false: otherwise.
π Example
Basic Usage:
Let's understand the basic usage of
event.isImmediatePropagationStopped()
with an example where multiple event handlers are attached to a button:index.htmlCopied<button id="myButton">Click Me</button>
example.jsCopied$("#myButton").on("click", function(event) { alert("First handler"); event.stopImmediatePropagation(); }); $("#myButton").on("click", function(event) { if(event.isImmediatePropagationStopped()) { alert("Immediate propagation stopped!"); } else { alert("Second handler"); } });
In this example, when the button is clicked, only the first alert ("First handler") will be shown, followed by the second alert ("Immediate propagation stopped!") because stopImmediatePropagation() stops the second handler from executing normally.
Conditional Event Handling:
You can use
event.isImmediatePropagationStopped()
to conditionally execute code based on whether stopImmediatePropagation() has been called:index.htmlCopied<button id="conditionalButton">Click Me</button>
example.jsCopied$("#conditionalButton").on("click", function(event) { event.stopImmediatePropagation(); }); $("#conditionalButton").on("click", function(event) { if(event.isImmediatePropagationStopped()) { console.log("Propagation has been stopped."); } else { console.log("Propagation continues."); } });
In this case, clicking the button will log "Propagation has been stopped." to the console, confirming that stopImmediatePropagation() was called.
Multiple Event Handlers on Different Elements:
The method is also useful when dealing with event propagation between different elements:
index.htmlCopied<div id="parentDiv"> <button id="childButton">Click Me</button> </div>
example.jsCopied$("#childButton").on("click", function(event) { event.stopImmediatePropagation(); alert("Child button clicked"); }); $("#parentDiv").on("click", function(event) { if(event.isImmediatePropagationStopped()) { alert("Immediate propagation stopped at child"); } else { alert("Parent div clicked"); } });
Here, clicking the button will trigger the alert "Child button clicked" and stop the propagation, preventing the parent div's click handler from executing normally. It will show "Immediate propagation stopped at child" instead.
π Conclusion
The event.isImmediatePropagationStopped()
method is an essential part of jQueryβs event handling suite, providing developers with fine-grained control over event propagation. By understanding and utilizing this method, you can create more robust and predictable event-driven behaviors in your web applications.
Whether you need to manage multiple event handlers on the same element or control the propagation of events across different elements, mastering this method is key to effective event management in jQuery.
π¨βπ» Join our Community:
Author
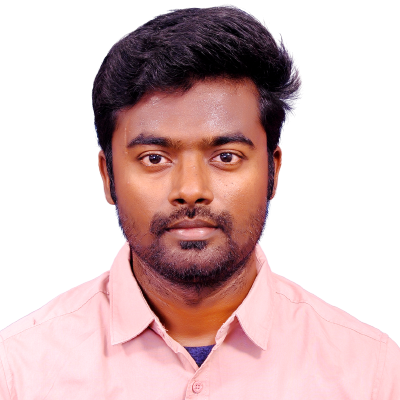
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery event.isImmediatePropagationStopped() Method), please comment here. I will help you immediately.