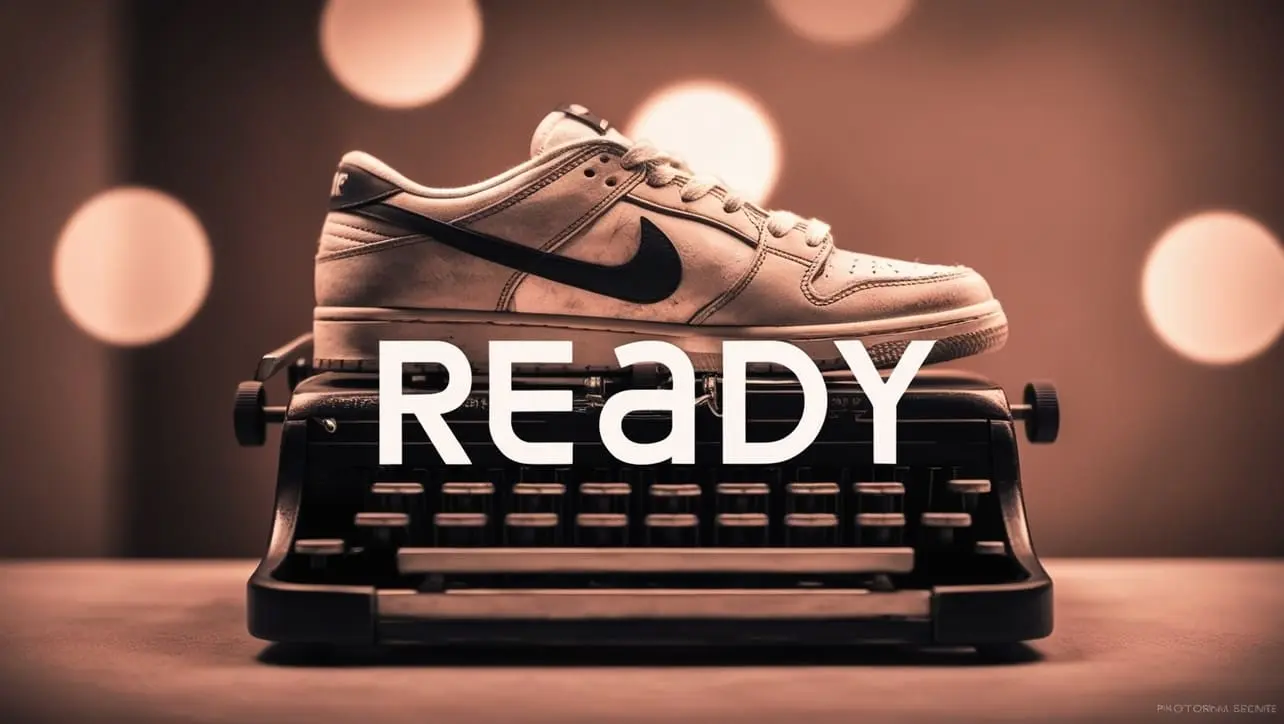
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery error Event
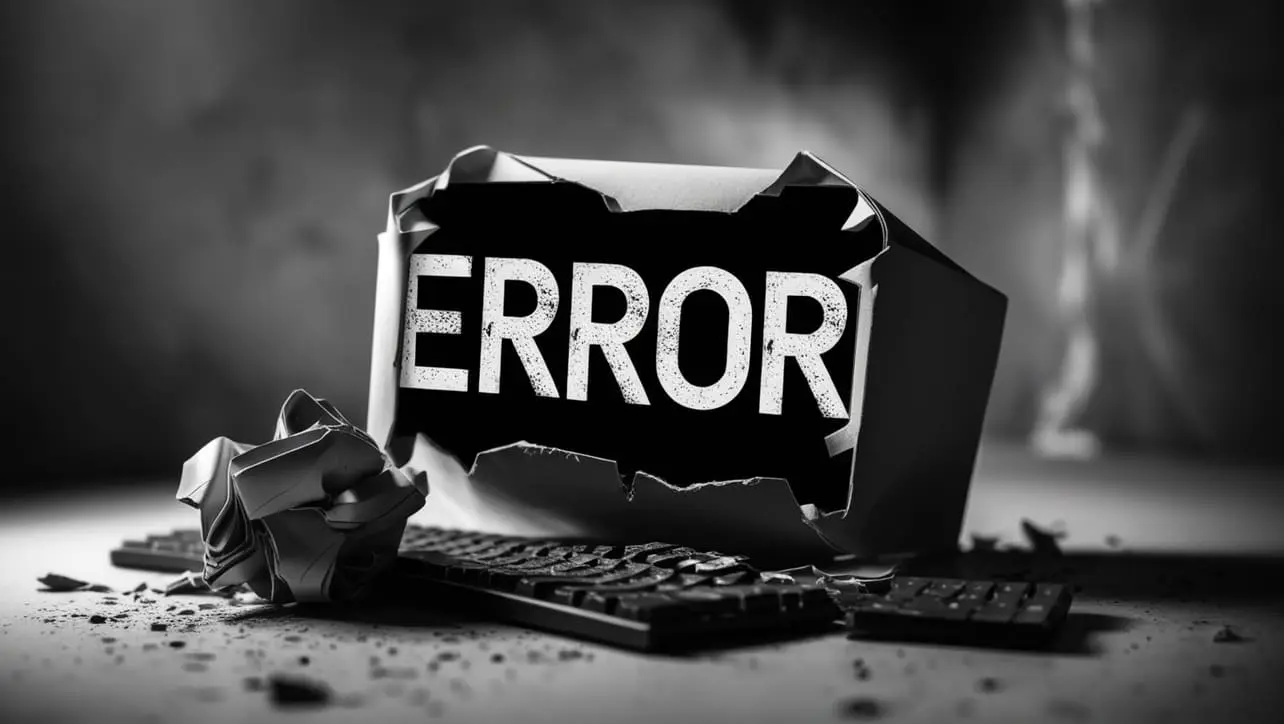
Photo Credit to CodeToFun
🙋 Introduction
In web development, errors are inevitable, and handling them gracefully is crucial for providing a seamless user experience. jQuery provides a mechanism to handle errors through the error
event. However, it's important to note that the .error() method has been deprecated, and the recommended approach is to use .on() instead.
In this guide, we'll explore the jQuery error
event, its syntax using .on(), and how you can effectively manage errors in your web applications.
🧠 Understanding error Event
The error
event in jQuery is triggered when an error occurs during the loading of an external resource, such as an image, script, or stylesheet. This event allows you to execute custom code to handle the error appropriately, whether it's displaying a fallback content or logging the error for debugging purposes.
💡 Syntax
The syntax for the error
event is straightforward:
$(selector).on("error", function(event) {
// Handle error
});
📝 Example
Handling Image Loading Errors:
Suppose you have an <img> element with a specific ID, and you want to handle any errors that occur during its loading:
index.htmlCopied<img src="invalid_path.jpg" id="image" alt="Image">
example.jsCopied$("#image").on("error", function() { $(this).attr("src", "fallback_image.jpg"); });
In this example, if the image fails to load due to an invalid path, the
error
event will be triggered, and the image source will be replaced with a fallback image.Logging Script Loading Errors:
You can also log errors that occur during the loading of external scripts for debugging purposes:
index.htmlCopied<script src="invalid_script.js"></script>
example.jsCopied$("script").on("error", function(event) { console.error("Script loading error:", event.target.src); });
This code snippet logs an error message along with the URL of the script that failed to load.
Handling CSS Loading Errors:
Similarly, you can handle errors that occur when loading external CSS stylesheets:
index.htmlCopied<link rel="stylesheet" href="invalid_styles.css">
example.jsCopied$("link[rel='stylesheet']").on("error", function(event) { console.error("Stylesheet loading error:", event.target.href); });
Here, any errors during the loading of CSS stylesheets will be logged to the console.
🎉 Conclusion
The jQuery error
event, although deprecated in favor of .on(), remains a valuable tool for handling errors in web development. By using .on() to bind event handlers, you can effectively manage errors occurring during the loading of external resources and ensure a smoother user experience.
Whether it's displaying fallback content, logging errors for debugging, or implementing alternative strategies, leveraging the error
event empowers you to create more robust and resilient web applications.
👨💻 Join our Community:
Author
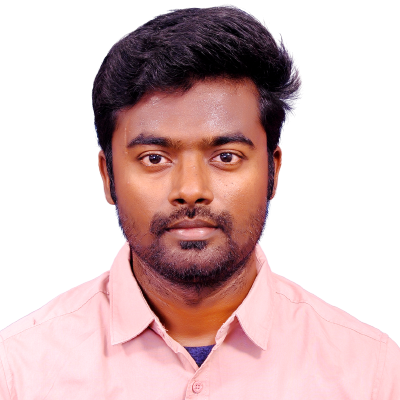
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery error Event), please comment here. I will help you immediately.