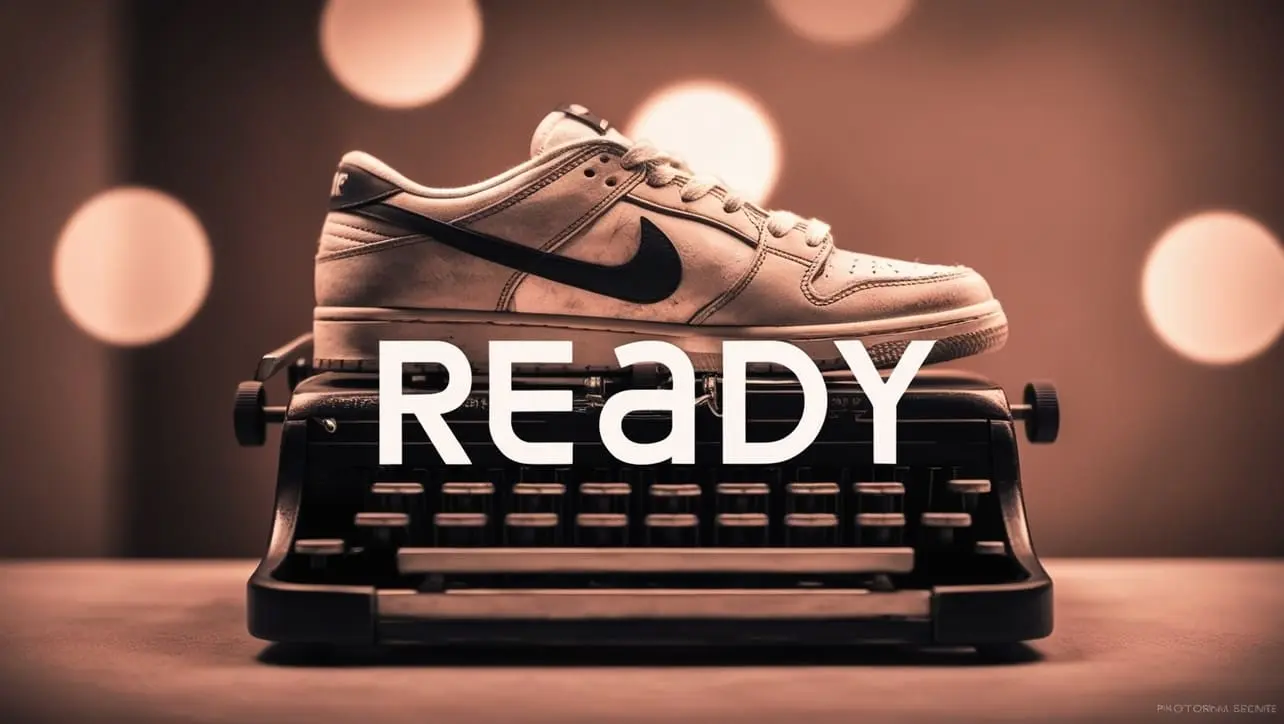
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery ajaxStop Event
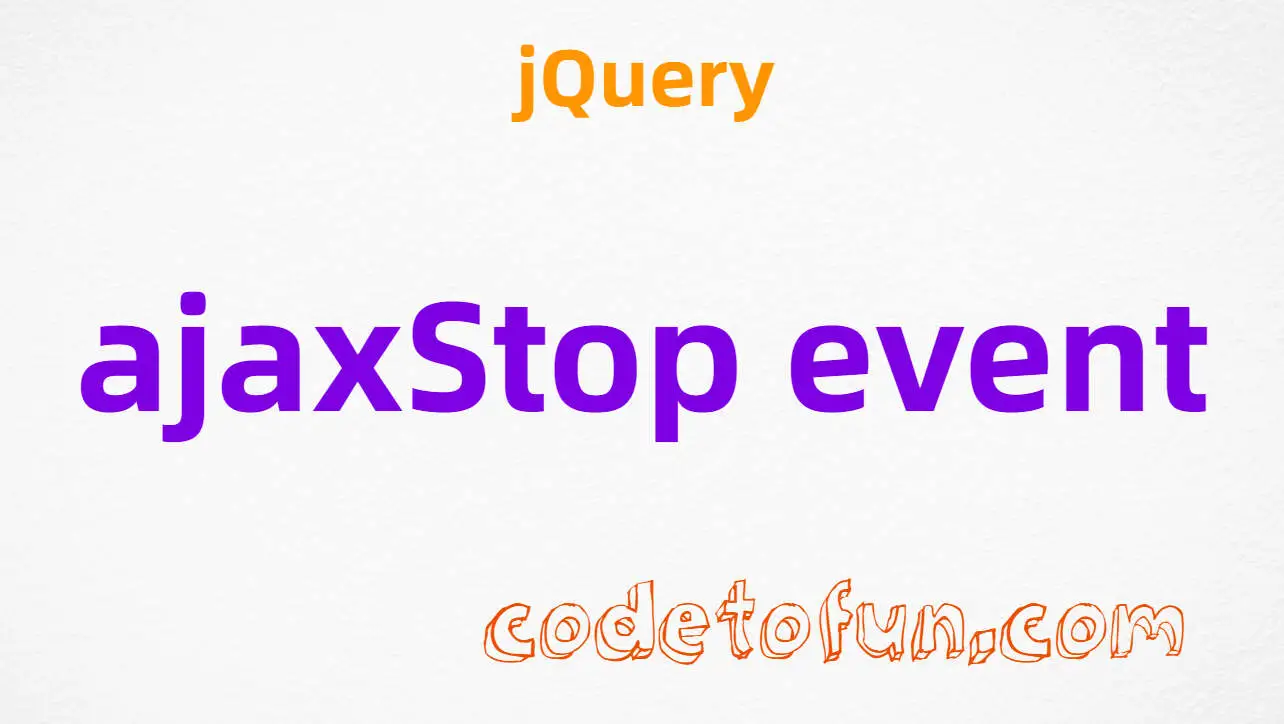
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a multitude of events to facilitate seamless handling of AJAX requests and responses. Among these, the ajaxStop
event plays a pivotal role, signaling the completion of all AJAX requests within a page. While the conventional .ajaxStop() method is now deprecated, jQuery recommends using the .on() method for event binding instead.
In this comprehensive guide, we'll explore the ajaxStop
event and demonstrate its usage with the .on() method, empowering you to streamline your AJAX event handling effectively.
🧠 Understanding ajaxStop Event
The ajaxStop
event is triggered when all AJAX requests initiated by jQuery have completed. This event is particularly useful for executing tasks that depend on the completion of AJAX operations, such as updating UI elements or performing post-request processing.
💡 Syntax
The syntax for the ajaxStop
event is straightforward:
$(document).on("ajaxStop", handler);
📝 Example
Handling the ajaxStop Event:
Let's consider a scenario where you want to display a message when all AJAX requests on a page have finished. You can achieve this by binding a handler to the
ajaxStop
event using the .on() method:example.jsCopied$(document).on("ajaxStop", function() { $("#status").text("All AJAX requests have completed."); });
In this example, the text content of an element with the ID status will be updated to indicate the completion of AJAX requests.
Performing Cleanup Operations:
You can also utilize the
ajaxStop
event to perform cleanup operations after all AJAX requests have finished. For instance, let's hide a loading spinner when all AJAX operations are complete:example.jsCopied$(document).on("ajaxStop", function() { $("#loadingSpinner").hide(); });
Here, the loading spinner with the ID loadingSpinner will be hidden once all AJAX requests are done.
Chaining Handlers:
The .on() method allows you to chain multiple event handlers for the same event. This enables you to execute multiple tasks sequentially when the
ajaxStop
event is triggered. For example:example.jsCopied$(document).on("ajaxStop", function() { console.log("All AJAX requests have completed."); }) .on("ajaxStop", function() { // Perform additional tasks here });
You can chain as many handlers as needed to handle various aspects of the event.
🎉 Conclusion
The ajaxStop
event, although deprecated in its traditional form, remains a crucial component in jQuery's AJAX event ecosystem. By leveraging the .on() method for event binding, you can seamlessly integrate ajaxStop
event handling into your web applications.
Whether it's updating UI elements, performing cleanup operations, or executing custom logic upon completion of AJAX requests, mastering the ajaxStop
event empowers you to create responsive and efficient web experiences.
👨💻 Join our Community:
Author
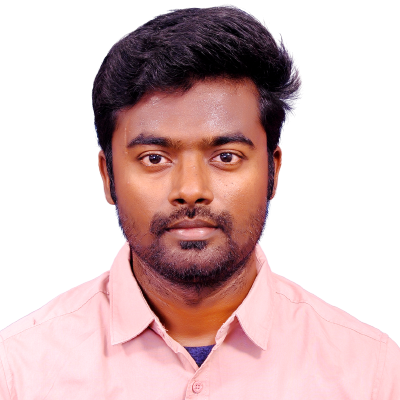
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery ajaxStop Event), please comment here. I will help you immediately.