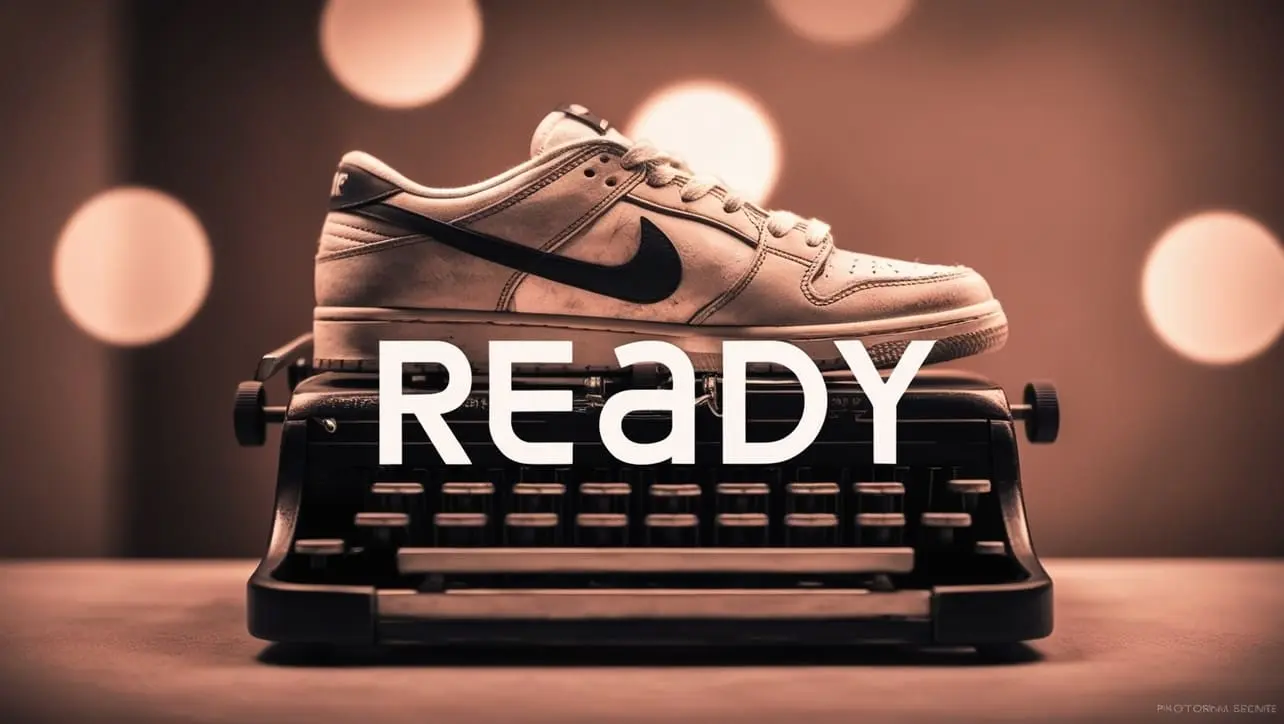
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery deferred.rejectWith() Method
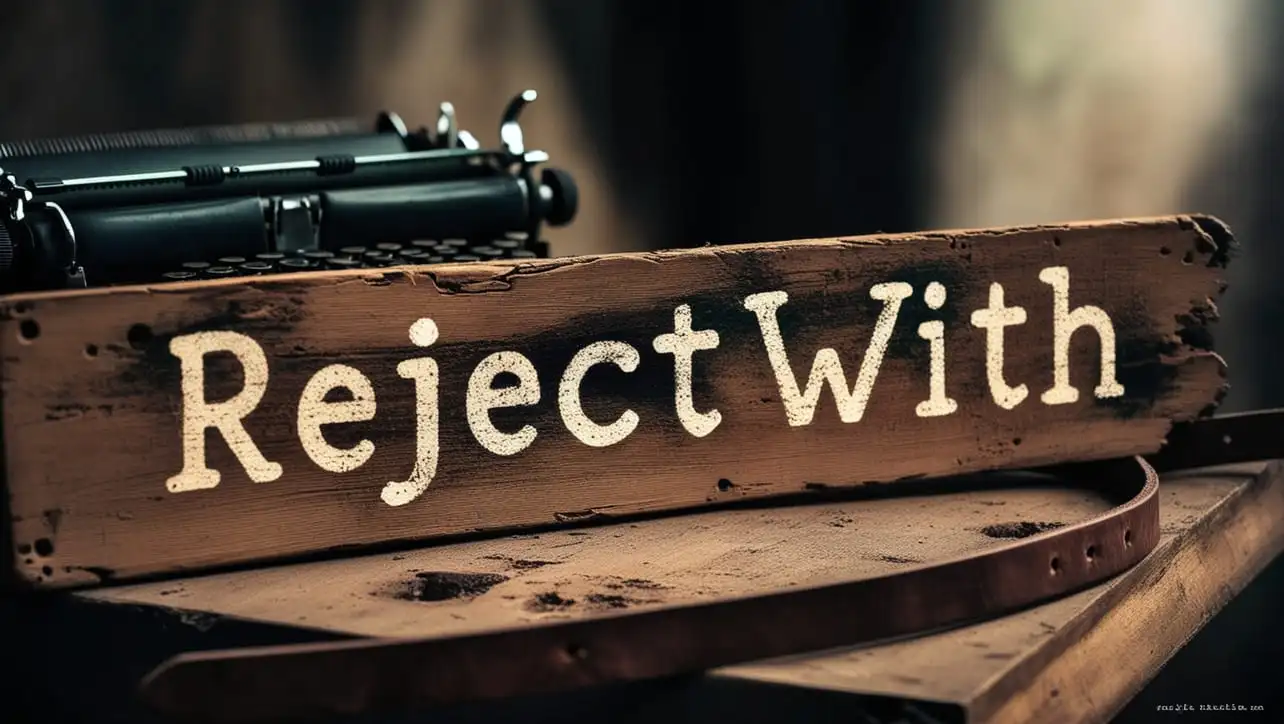
Photo Credit to CodeToFun
Introduction
In the realm of asynchronous programming, error handling plays a pivotal role in ensuring the reliability and stability of applications. jQuery offers a suite of tools for managing asynchronous operations, and one such tool is the deferred.rejectWith()
method. This method provides developers with a mechanism to explicitly reject deferred objects with custom contexts and arguments.
In this comprehensive guide, we'll explore the deferred.rejectWith()
method, examining its syntax, practical applications, and best practices for effective error management.
Understanding deferred.rejectWith() Method
The deferred.rejectWith()
method is part of jQuery's Deferred Object API, designed to handle asynchronous operations such as AJAX requests, animations, and deferred promises. Unlike deferred.reject(), which simply rejects a deferred object with a specified context and arguments, deferred.rejectWith()
allows for finer control over the rejection process by accepting a custom context and arguments.
Syntax
The syntax for the deferred.rejectWith()
method is straightforward:
deferred.rejectWith( context, [args] )
- context: The context in which the error-handling function (fail callback) will be executed.
- args (Optional): An array or array-like object containing arguments to be passed to the error-handling function.
Example
Let's dive into a simple example to illustrate the usage of the deferred.rejectWith()
method:
var deferred = $.Deferred();
deferred.rejectWith(document, ["error"]);
deferred.fail(function(error) {
console.error("Error occurred:", error);
});
Best Practices
When working with the deferred.rejectWith()
method, consider the following best practices:
Contextual Rejections:
Select appropriate contexts for rejection to ensure error-handling functions execute in the desired environment.
Parameterization:
Pass relevant arguments to the error-handling function to provide additional context or diagnostic information about the error.
Error Reporting:
Implement robust error reporting mechanisms to capture and log rejected deferred objects, aiding in troubleshooting and issue resolution.
Consistent Error Handling:
Establish consistent error-handling conventions across your codebase to streamline development and maintenance efforts.
Error Recovery:
Consider implementing fallback mechanisms or alternative strategies to recover gracefully from rejected deferred objects and maintain application functionality.
Use Cases
Custom Error Handling:
Tailor error handling logic to specific contexts or scenarios, allowing for more granular control over error management.
Deferred Chaining:
Integrate
deferred.rejectWith()
seamlessly into chains of asynchronous operations to handle errors at various stages of execution.AJAX Requests:
Reject AJAX requests with custom error contexts and arguments to provide detailed feedback to the user or log pertinent information for debugging purposes.
Deferred Promises:
Employ
deferred.rejectWith()
in conjunction with promises to gracefully handle errors in asynchronous JavaScript operations.
Conclusion
The deferred.rejectWith()
method in jQuery empowers developers to manage asynchronous errors with precision and flexibility, enhancing the robustness and reliability of their applications.
By mastering its syntax, exploring practical applications, and adhering to best practices for error management, you can effectively handle rejections in asynchronous operations with confidence. Incorporate deferred.rejectWith()
into your toolkit to build resilient web applications that gracefully handle errors and deliver exceptional user experiences.
Join our Community:
Author
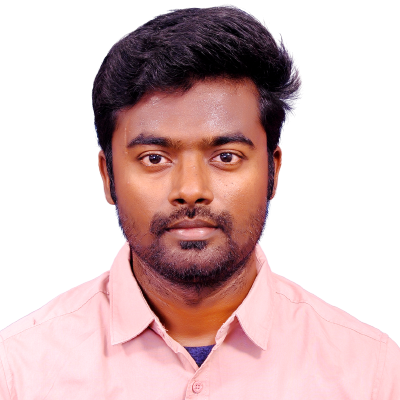
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.rejectWith() Method), please comment here. I will help you immediately.