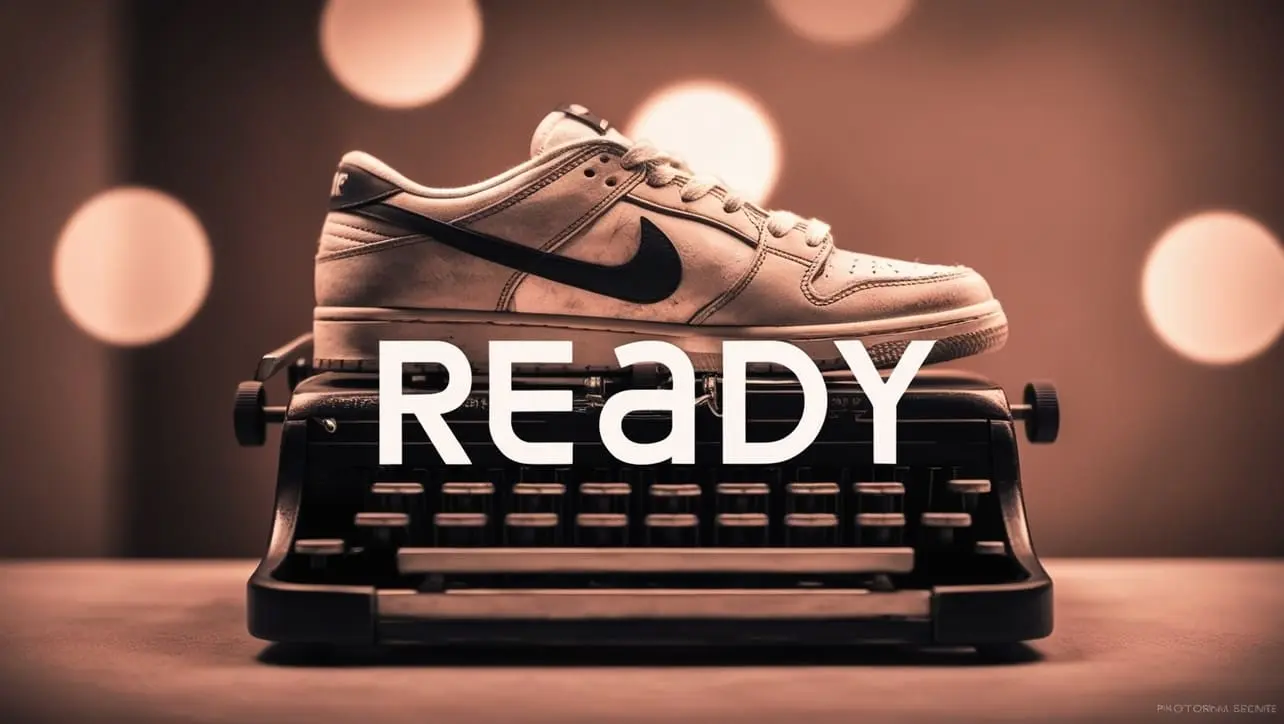
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery deferred.promise() Method
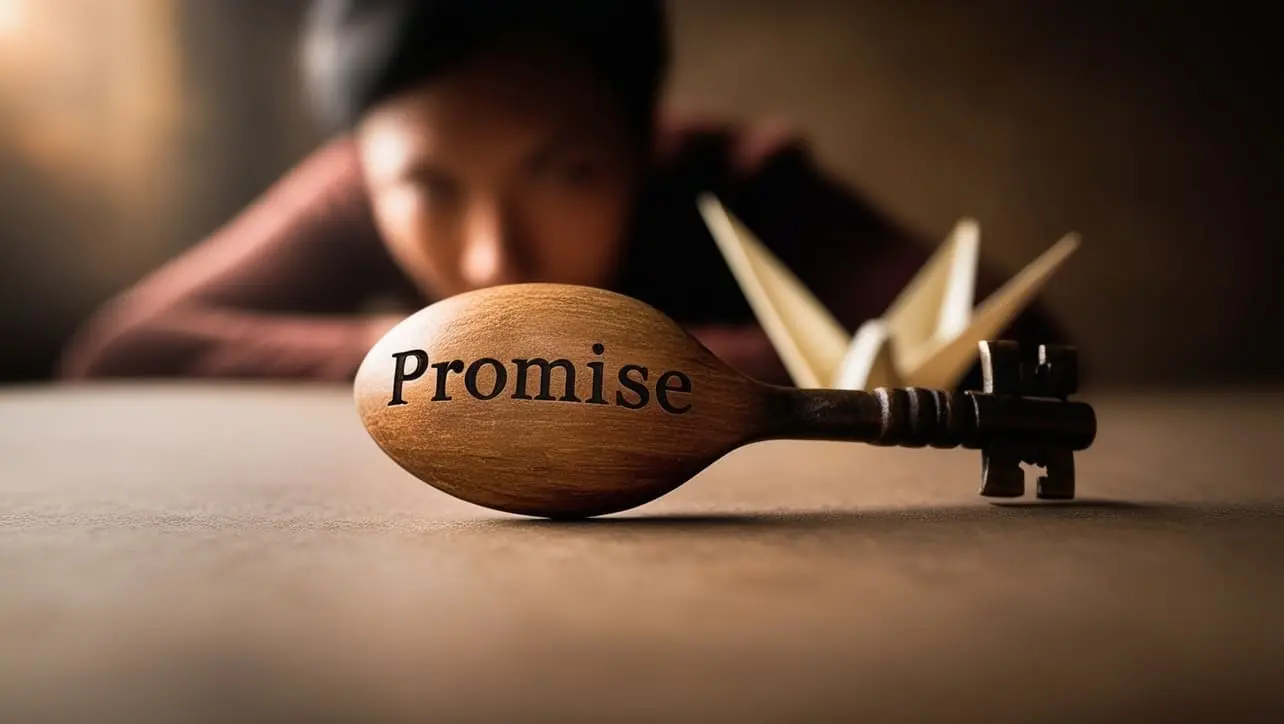
Photo Credit to CodeToFun
🙋 Introduction
Asynchronous programming is fundamental in modern web development, allowing applications to perform tasks concurrently without blocking the main execution thread. jQuery simplifies asynchronous operations with its Deferred Object API, offering powerful tools like the deferred.promise()
method.
In this comprehensive guide, we'll explore the intricacies of deferred.promise()
, examining its syntax, practical applications, and best practices for leveraging its capabilities.
🧠 Understanding deferred.promise() Method
The deferred.promise()
method is a cornerstone of jQuery's Deferred Object API, enabling developers to create and manage promises for asynchronous operations. Promises represent the eventual completion or failure of an asynchronous task, providing a standardized interface for handling its outcome.
💡 Syntax
The syntax for the deferred.promise()
method is straightforward:
deferred.promise( target )
- target (optional): An object onto which the promise methods will be added. If omitted, a new plain JavaScript object will be created.
📝 Example
Let's dive into a simple example to illustrate the usage of the deferred.promise()
method:
function fetchData() {
var deferred = $.Deferred();
// Simulate asynchronous data fetching
setTimeout(function() {
deferred.resolve({
data: "Sample data"
});
}, 2000);
return deferred.promise();
}
var promise = fetchData();
promise.then(function(response) {
console.log("Data fetched:", response.data);
}).catch(function(error) {
console.error("Error fetching data:", error);
});
🏆 Best Practices
When working with the deferred.promise()
method, consider the following best practices:
Return Promises:
Ensure that functions returning promises follow a consistent pattern to facilitate interoperability and composability with other asynchronous operations.
Error Handling:
Handle errors gracefully by chaining .catch() methods to promise chains, providing robust error recovery mechanisms and enhancing application resilience.
Composition:
Leverage promise composition techniques such as Promise.all() or Promise.race() to orchestrate multiple asynchronous tasks efficiently, optimizing performance and resource utilization.
Documentation:
Document promise-based APIs thoroughly, including method signatures, expected inputs, and possible outcomes, to aid developers in understanding and using them effectively.
Testing:
Conduct rigorous testing of promise-based code paths, including edge cases and error scenarios, to validate correctness and reliability under various conditions.
📚 Use Cases
AJAX Requests:
Use
deferred.promise()
to encapsulate AJAX requests, allowing for cleaner and more organized code when handling asynchronous data fetching from servers.Chaining Operations:
Chain promise methods such as .then() and .catch() to perform sequential asynchronous operations, enhancing code readability and maintainability.
Timeouts:
Implement timeouts on promises to handle scenarios where an asynchronous operation takes longer than expected, preventing potential performance issues.
Event Handling:
Utilize promises to manage asynchronous events in web applications, such as user interactions or external triggers.
Animation Sequences:
Coordinate complex animation sequences by wrapping them in promises, ensuring synchronized execution and seamless transitions.
🎉 Conclusion
The deferred.promise()
method in jQuery empowers developers to harness the power of promises, enabling elegant and efficient management of asynchronous operations in web applications.
By mastering its syntax, exploring diverse use cases, and adhering to best practices, you can leverage deferred.promise()
to build robust, responsive, and scalable applications that deliver exceptional user experiences. Incorporate promises into your development workflow to unlock new possibilities and elevate your JavaScript programming prowess.
👨💻 Join our Community:
Author
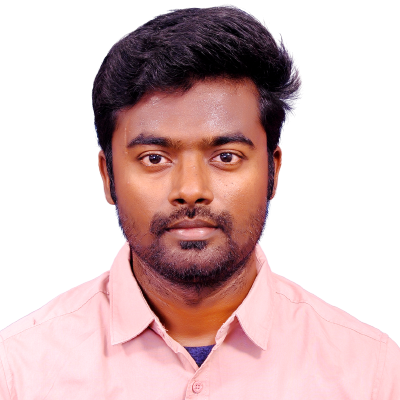
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.promise() Method), please comment here. I will help you immediately.