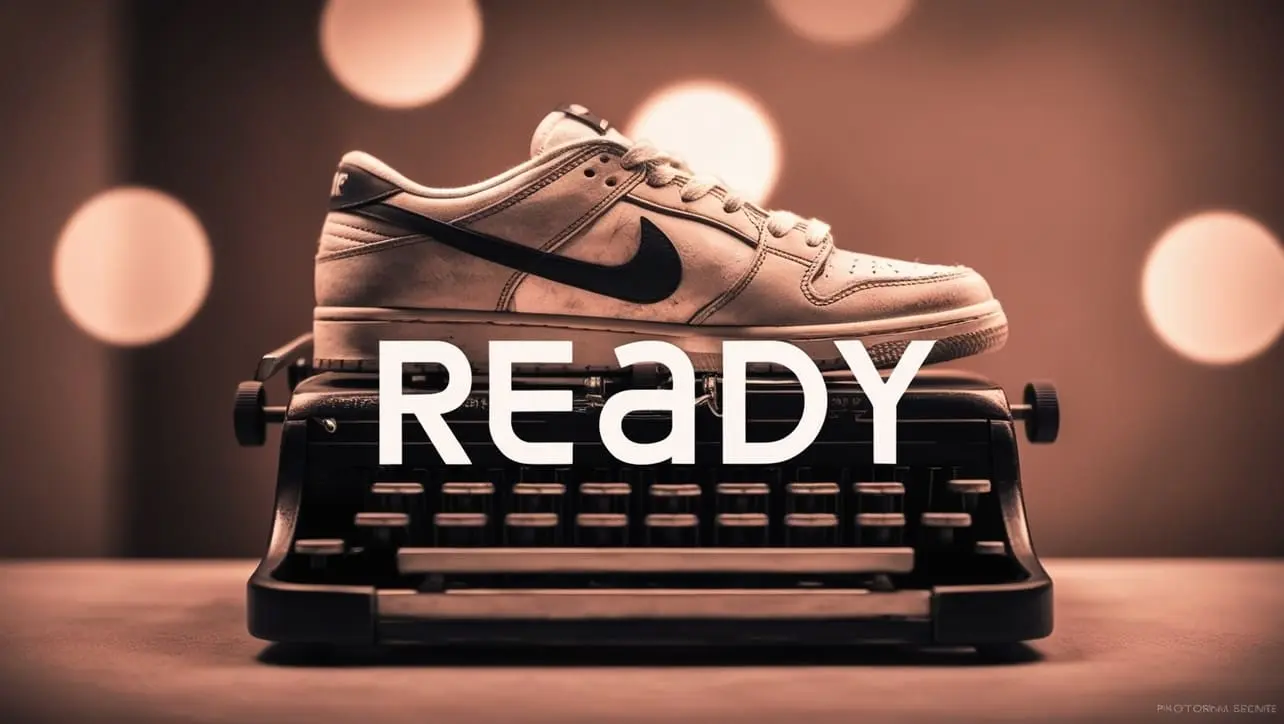
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery deferred.isRejected() Method
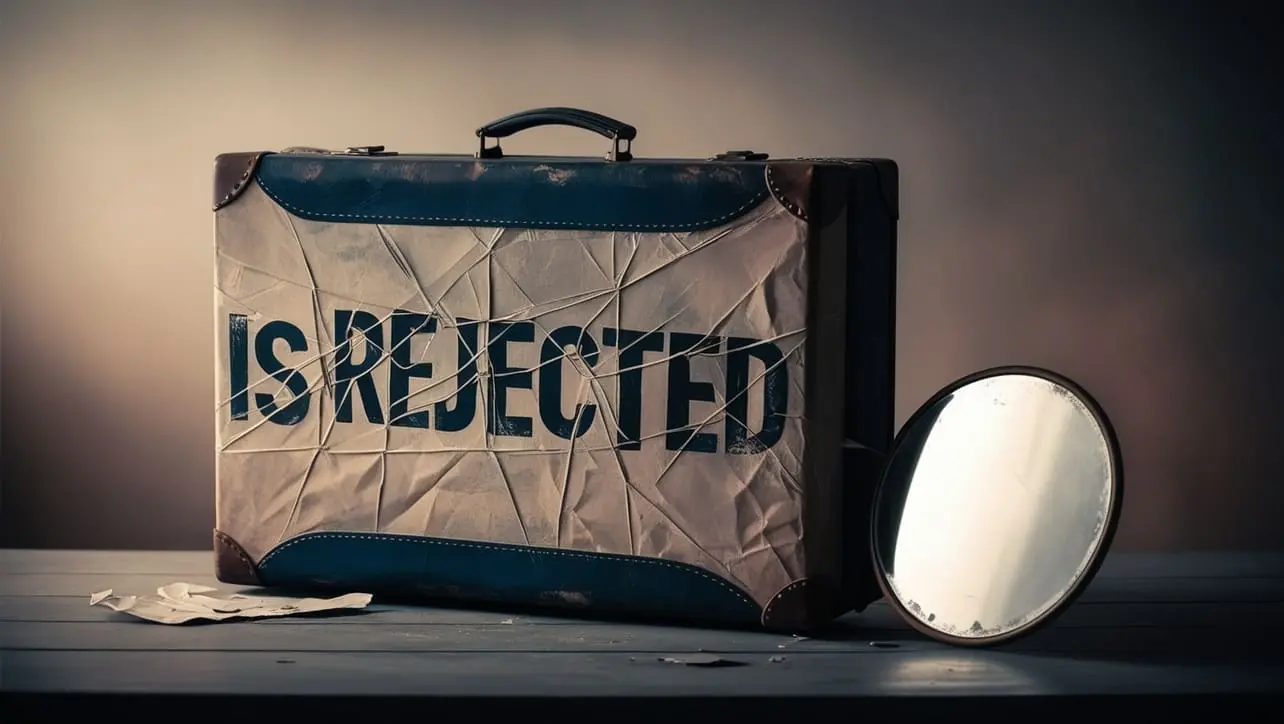
Photo Credit to CodeToFun
Introduction
Error handling is a critical aspect of building resilient and dependable applications, particularly in JavaScript development. jQuery offers a suite of tools to manage asynchronous operations effectively, including the deferred.isRejected()
method.
This method allows developers to detect whether a deferred object has been rejected, providing valuable insights into the status of asynchronous tasks.
In this guide, we'll delve into the functionality of deferred.isRejected()
, examining its syntax, applications, and best practices for error state detection.
Understanding deferred.isRejected() Method
The deferred.isRejected()
method is part of jQuery's Deferred Object API, designed to work with promises and other asynchronous operations. It enables developers to determine if a deferred object has been rejected, indicating that an error has occurred during its execution.
Syntax
The syntax for the deferred.isRejected()
method is straightforward:
deferred.isRejected()
Return Value
- true: Indicates that the deferred object has been rejected.
- false: Indicates that the deferred object has not been rejected.
Example
Let's dive into a simple example to illustrate the usage of the deferred.isRejected()
method:
var deferred = $.Deferred();
// Simulate an asynchronous operation
setTimeout(function() {
deferred.reject("Error: Operation timed out");
}, 5000);
// Check if the deferred object is rejected
if(deferred.isRejected()) {
console.log("The operation has been rejected");
} else {
console.log("The operation is still pending or resolved");
}
Best Practices
When working with the deferred.isRejected()
method, consider the following best practices:
Contextual Error Handling:
Combine
deferred.isRejected()
with deferred.fail() to implement comprehensive error handling strategies tailored to specific asynchronous tasks.Error Recovery:
Design robust error recovery mechanisms that leverage the information provided by
deferred.isRejected()
to restore application functionality gracefully.Asynchronous Control Flow:
Use
deferred.isRejected()
judiciously within asynchronous control flow constructs such as Promise.all() or $.when() to coordinate error handling across multiple asynchronous tasks.Testing and Validation:
Thoroughly test error detection logic using simulated error scenarios to ensure the reliability and accuracy of
deferred.isRejected()
in detecting error states.Documentation and Communication:
Document the usage and behavior of
deferred.isRejected()
within your codebase to facilitate collaboration and understanding among team members.
Use Cases
Error State Detection:
Determine whether an asynchronous operation has encountered an error condition, allowing for appropriate error handling and recovery mechanisms.
Conditional Logic:
Incorporate
deferred.isRejected()
within conditional statements to execute specific code paths based on the error state of asynchronous tasks.Dynamic UI Updates:
Update the user interface dynamically based on the error status of asynchronous operations, providing feedback or alternative options as needed.
Error Logging:
Log error states detected by
deferred.isRejected()
for debugging purposes, enabling developers to diagnose and address issues efficiently.
Conclusion
The deferred.isRejected()
method in jQuery serves as a valuable tool for detecting error states in asynchronous operations, enabling developers to build robust and resilient applications.
By incorporating deferred.isRejected()
into your error handling strategies and adhering to best practices, you can effectively monitor the status of asynchronous tasks and respond appropriately to error conditions. Harness the power of deferred.isRejected()
to enhance the reliability and stability of your JavaScript applications, delivering exceptional user experiences even in the face of unexpected errors.
Join our Community:
Author
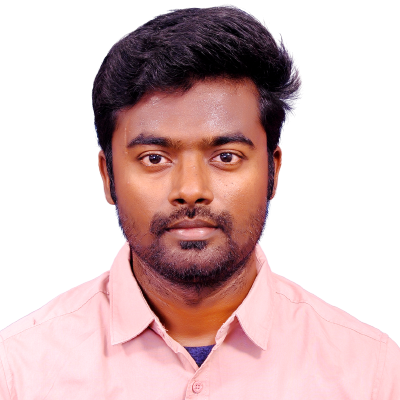
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.isRejected() Method), please comment here. I will help you immediately.