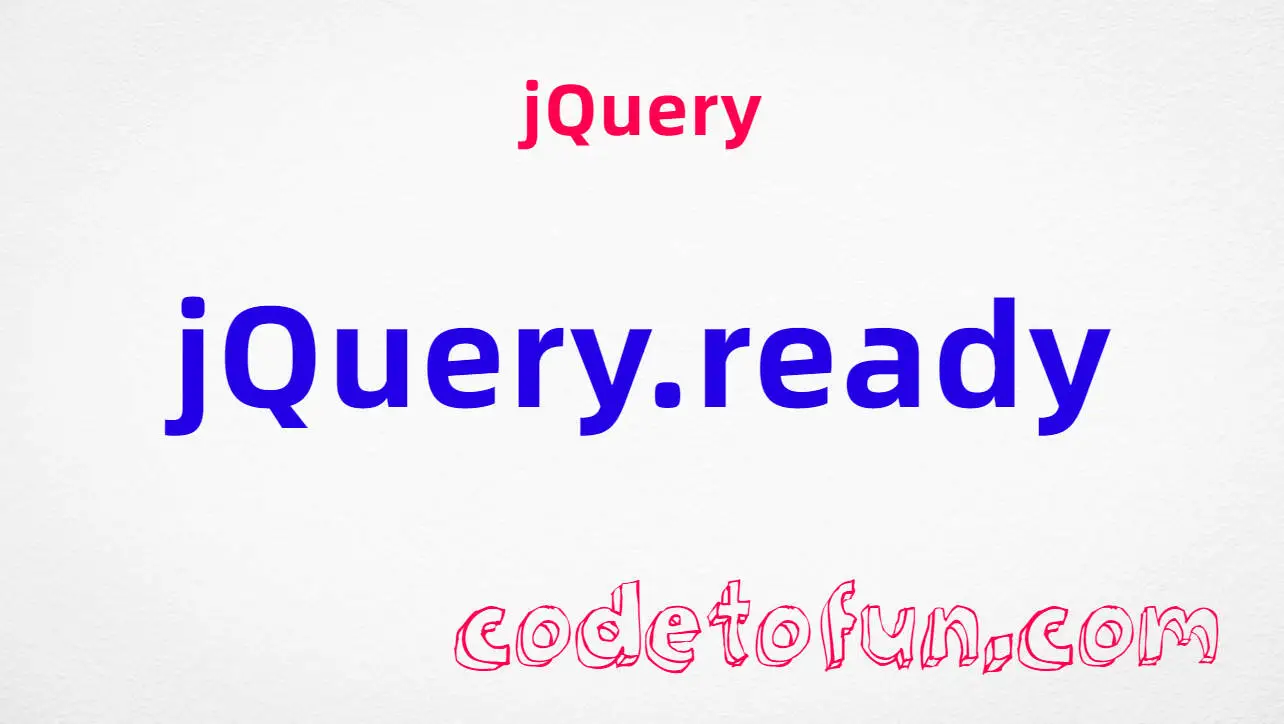
jQuery Basic
jQuery Deferred
- jQuery Deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
jQuery deferred.fail() Method
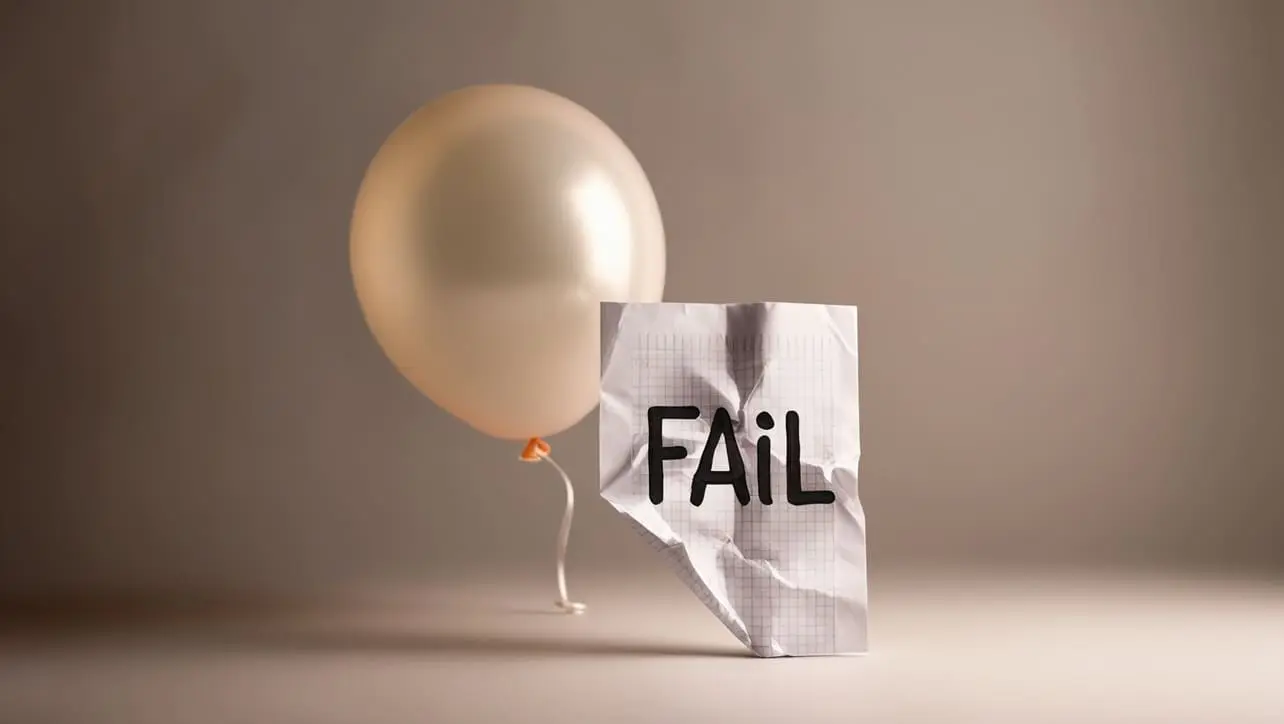
Photo Credit to CodeToFun
🙋 Introduction
Error handling is a crucial aspect of writing robust and reliable code, especially in JavaScript programming. Fortunately, jQuery provides powerful tools to manage errors gracefully. One such tool is the deferred.fail()
method, which allows developers to handle errors in asynchronous operations effectively.
In this guide, we'll delve into the intricacies of deferred.fail()
method, exploring its syntax, common use cases, and best practices.
🧠 Understanding deferred.fail() Method
The deferred.fail()
method is a part of jQuery's Deferred Object API, which facilitates the handling of asynchronous operations such as AJAX requests, animations, and timeouts. It is designed to be chained onto a deferred object, allowing you to specify what should happen if the associated operation encounters an error.
💡 Syntax
The syntax for the deferred.fail()
method is straightforward:
deferred.fail( errorHandler )
- errorHandler: A function that is executed when the deferred object is rejected or encounters an error. It accepts arguments that provide information about the error.
📝 Example
Let's dive into a simple example to illustrate the usage of the deferred.fail()
method:
$.ajax({
url: "example.com/api",
method: "GET"
}).done(function(response) {
// Process the successful response
}).fail(function(xhr, status, error) {
// Handle the error
console.error("Request failed:", status, error);
});
🏆 Best Practices
When working with the deferred.fail()
method, consider the following best practices:
Be Specific:
Provide meaningful error messages or log detailed information about errors to facilitate debugging.
Chain Methods:
Leverage method chaining to organize error handling logic alongside other asynchronous operations, improving code readability and maintainability.
Fallback Mechanisms:
Implement fallback mechanisms or alternative strategies in case of errors, ensuring graceful degradation of functionality.
Testing:
Thoroughly test error handling scenarios to identify and address potential vulnerabilities or edge cases.
Documentation:
Document error handling procedures and conventions within your codebase to aid collaboration and future maintenance efforts.
📚 Use Cases
AJAX Requests:
Handle errors gracefully when making AJAX requests to a server. This includes scenarios such as network errors, server errors, or invalid responses.
Animation Effects:
When animating elements on a webpage, use
deferred.fail()
to handle errors that may occur during the animation process, ensuring a smooth user experience.Promises:
Integrate
deferred.fail()
with promises to manage errors in asynchronous JavaScript operations comprehensively.Timeouts:
Implement error handling logic for operations that may exceed a specified time limit, preventing issues like unresponsive UIs.
🎉 Conclusion
The deferred.fail()
method in jQuery empowers developers to tackle errors in asynchronous operations effectively, enhancing the reliability and robustness of their code.
By understanding its syntax, exploring common use cases, and adhering to best practices, you can master error handling in JavaScript with confidence. Incorporate deferred.fail()
into your toolkit to build resilient web applications that gracefully handle unexpected circumstances.
👨💻 Join our Community:
Author
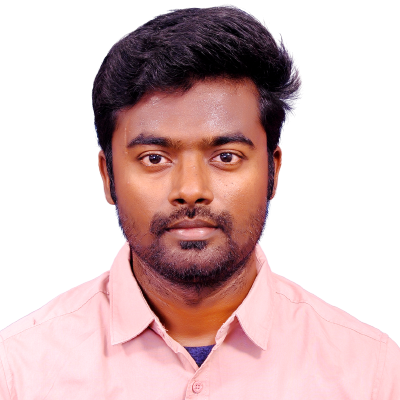
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.fail() Method), please comment here. I will help you immediately.