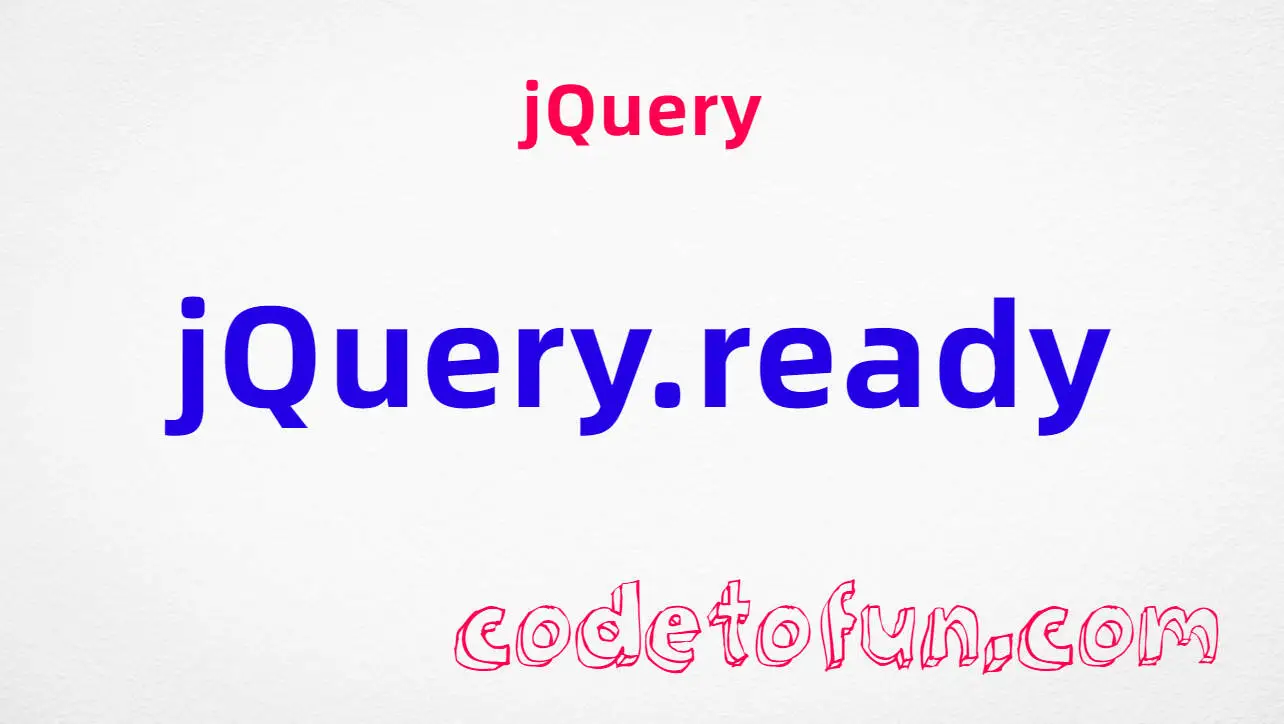
jQuery Basic
jQuery Deferred
- jQuery Deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
jQuery deferred.done() Method
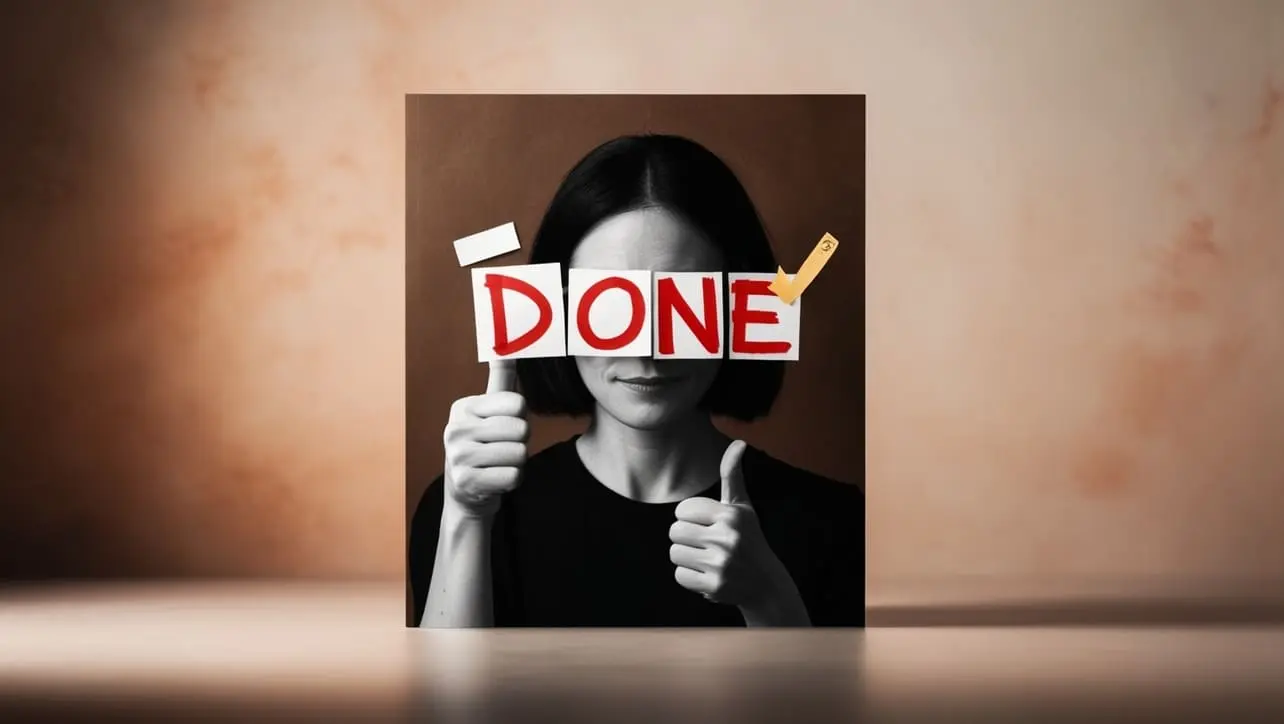
Photo Credit to CodeToFun
🙋 Introduction
In the realm of asynchronous programming, managing successful outcomes is just as crucial as handling errors. jQuery's deferred.done()
method is a powerful tool that enables developers to handle successful completion of asynchronous operations gracefully.
In this guide, we'll delve into the nuances of the deferred.done()
method, exploring its syntax, typical use cases, and best practices.
🧠 Understanding deferred.done() Method
The deferred.done()
method is part of jQuery's Deferred Object API, which facilitates the handling of asynchronous operations like AJAX requests, animations, and timeouts. This method allows developers to specify actions to be taken when the associated operation is successfully completed.
💡 Syntax
The syntax for the deferred.done()
method is straightforward:
deferred.done( doneCallback )
- doneCallback: A function to be executed when the deferred object is resolved successfully. It may accept arguments containing data from the successful operation.
📝 Example
Let's dive into a simple example to illustrate the usage of the deferred.done()
method:
$.ajax({
url: "example.com/api",
method: "GET"
}).done(function(response) {
// Process the successful response
console.log("Request successful:", response);
});
🏆 Best Practices
When working with the deferred.done()
method, consider the following best practices:
Modularization:
Encapsulate logic within doneCallback functions to keep code organized and maintainable.
Error Handling:
While
deferred.done()
focuses on successful outcomes, ensure that error handling logic is also in place to address potential issues.Data Processing:
Utilize data returned from successful operations efficiently, validating and transforming it as needed before further processing.
Event Triggering:
Trigger custom events or callbacks within doneCallback functions to notify other parts of the application about successful completion of operations.
Testing:
Test scenarios involving successful outcomes to validate the behavior of your code under various conditions.
📚 Use Cases
AJAX Requests:
Perform actions such as updating the UI or processing data retrieved from the server upon successful completion of AJAX requests.
Animation Effects:
Execute code to handle successful completion of animation effects, such as displaying a success message or triggering additional animations.
Promises:
Integrate
deferred.done()
with promises to execute code when asynchronous JavaScript operations resolve successfully.Timeouts:
Implement actions to be taken when operations complete within a specified time frame, ensuring timely execution of code blocks.
🎉 Conclusion
The deferred.done()
method in jQuery empowers developers to handle successful completion of asynchronous operations with finesse, enabling the creation of robust and responsive web applications.
By understanding its syntax, exploring common use cases, and adhering to best practices, you can leverage the deferred.done()
method to orchestrate seamless user experiences and streamline asynchronous workflows. Incorporate this method into your toolkit to harness the full potential of asynchronous programming in JavaScript with confidence.
👨💻 Join our Community:
Author
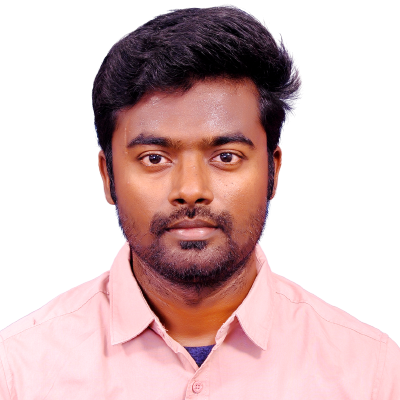
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.done() Method), please comment here. I will help you immediately.