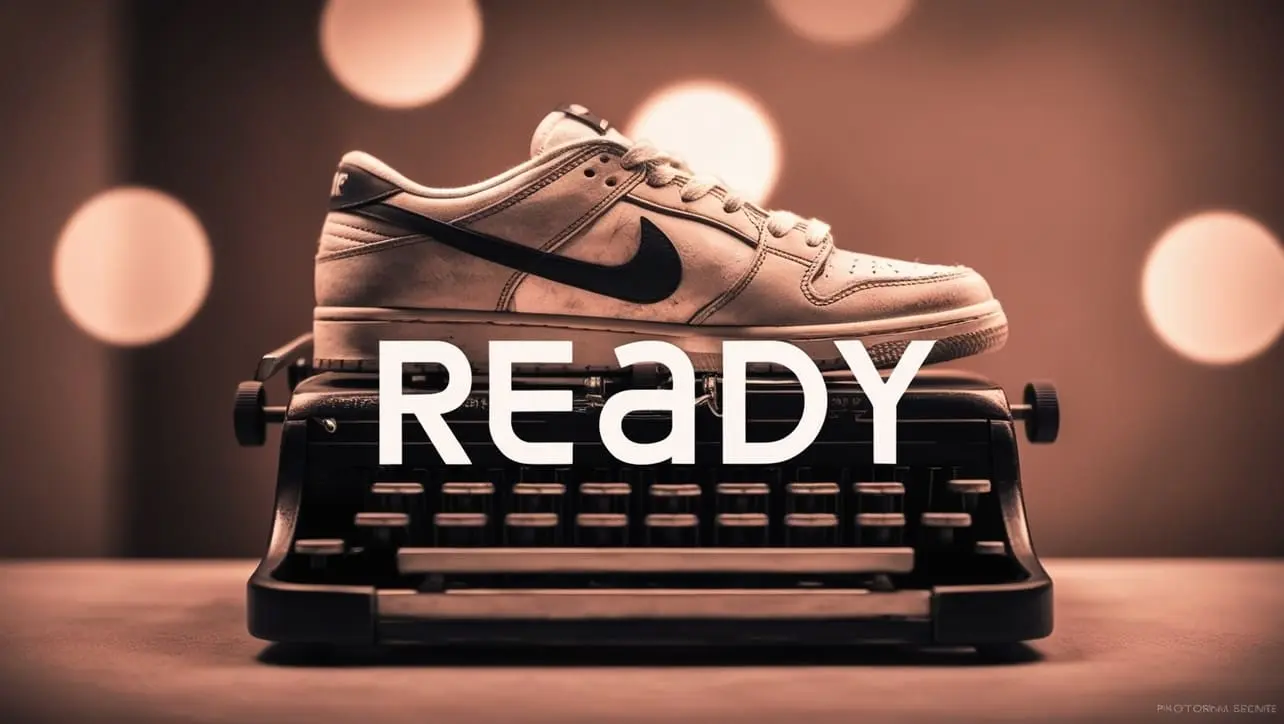
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery deferred.catch() Method
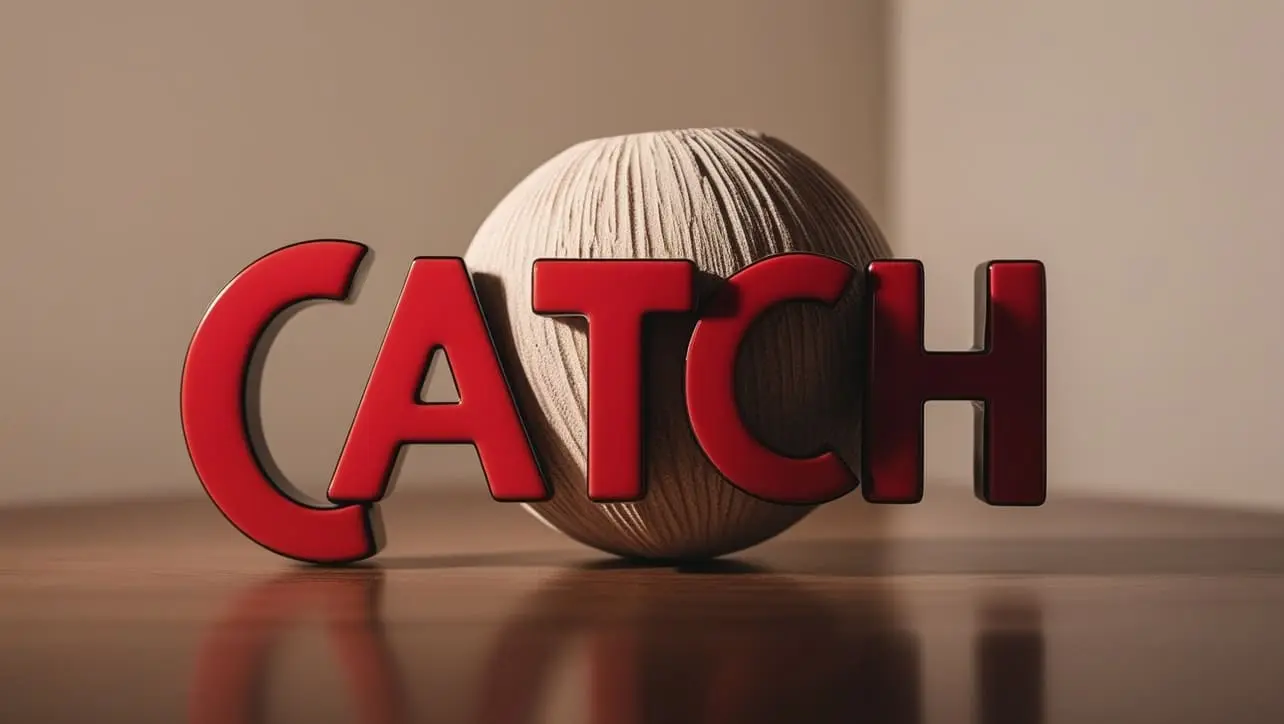
Photo Credit to CodeToFun
Introduction
In JavaScript programming, error handling is paramount for building robust and reliable applications, especially when dealing with asynchronous operations. jQuery offers a suite of tools to facilitate error management, including the deferred.catch()
method.
This guide explores the intricacies of deferred.catch()
, providing insights into its syntax, practical applications, and best practices.
Understanding deferred.catch() Method
The deferred.catch()
method is an essential part of jQuery's Deferred Object API, tailored for error handling in asynchronous operations. It allows developers to define a centralized error handler for a sequence of asynchronous tasks, simplifying the code structure and improving maintainability.
Syntax
The syntax for the deferred.catch()
method is straightforward:
deferred.catch( errorHandler )
- errorHandler: A function that is executed when the associated deferred object or promise is rejected or encounters an error. It accepts arguments providing information about the error.
Example
Let's dive into a simple example to illustrate the usage of the deferred.catch()
method:
myDeferredFunction()
.then(function(result) {
// Process the result
})
.catch(function(error) {
// Handle the error
console.error("An error occurred:", error);
});
Best Practices
When working with the deferred.catch()
method, consider the following best practices:
Error Propagation:
Propagate errors appropriately through the promise chain to ensure consistent and predictable error handling behavior.
Contextual Logging:
Provide context-specific error messages or log detailed information about errors to aid debugging and troubleshooting efforts.
Graceful Degradation:
Implement fallback mechanisms or alternative strategies to gracefully degrade functionality in the event of errors, preserving the user experience.
Unit Testing:
Thoroughly test error handling scenarios to verify the correctness and robustness of your error handling logic, addressing potential edge cases and vulnerabilities.
Documentation:
Document error handling conventions and practices within your codebase to facilitate collaboration and knowledge sharing among team members.
Use Cases
Promises:
Use
deferred.catch()
in conjunction with promises to centralize error handling logic across multiple asynchronous tasks, enhancing code readability and maintainability.AJAX Requests:
Streamline error handling for AJAX requests by attaching a single .catch() method to handle any network errors, server failures, or invalid responses.
Chained Deferreds:
Simplify error management in chained deferred operations, ensuring consistent handling of errors throughout the execution flow.
Async/Await:
Integrate
deferred.catch()
with the async/await syntax to gracefully handle errors in modern JavaScript codebases, promoting cleaner and more concise error handling.
Conclusion
The deferred.catch()
method in jQuery empowers developers to streamline error handling in asynchronous operations, promoting cleaner and more maintainable code.
By mastering its syntax, exploring common use cases, and adhering to best practices, you can build resilient web applications that gracefully handle errors and deliver a seamless user experience. Incorporate deferred.catch()
into your toolkit to elevate the reliability and robustness of your JavaScript applications.
Join our Community:
Author
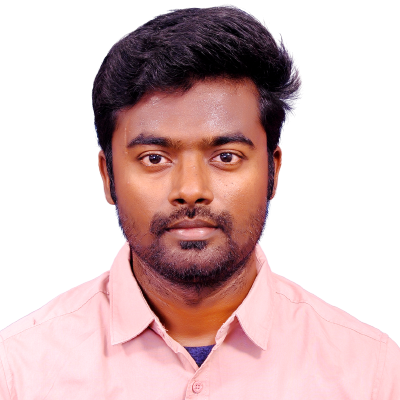
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.catch() Method), please comment here. I will help you immediately.