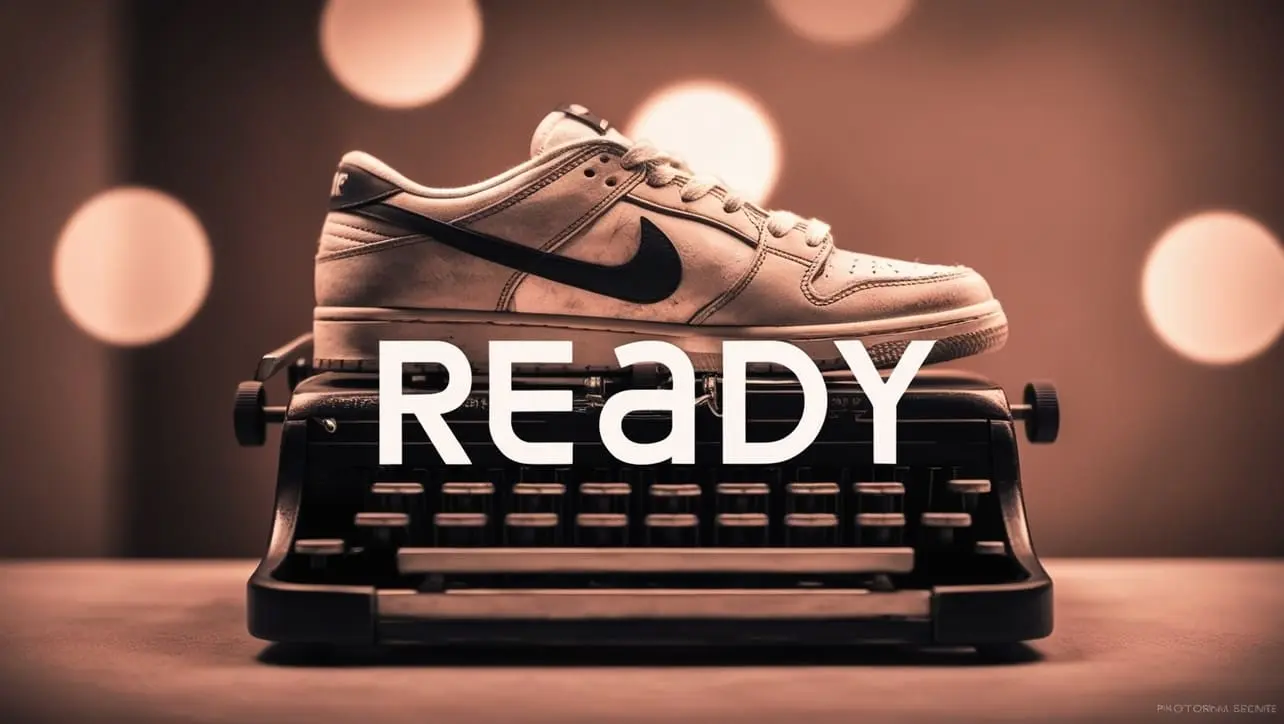
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .dblclick() Method
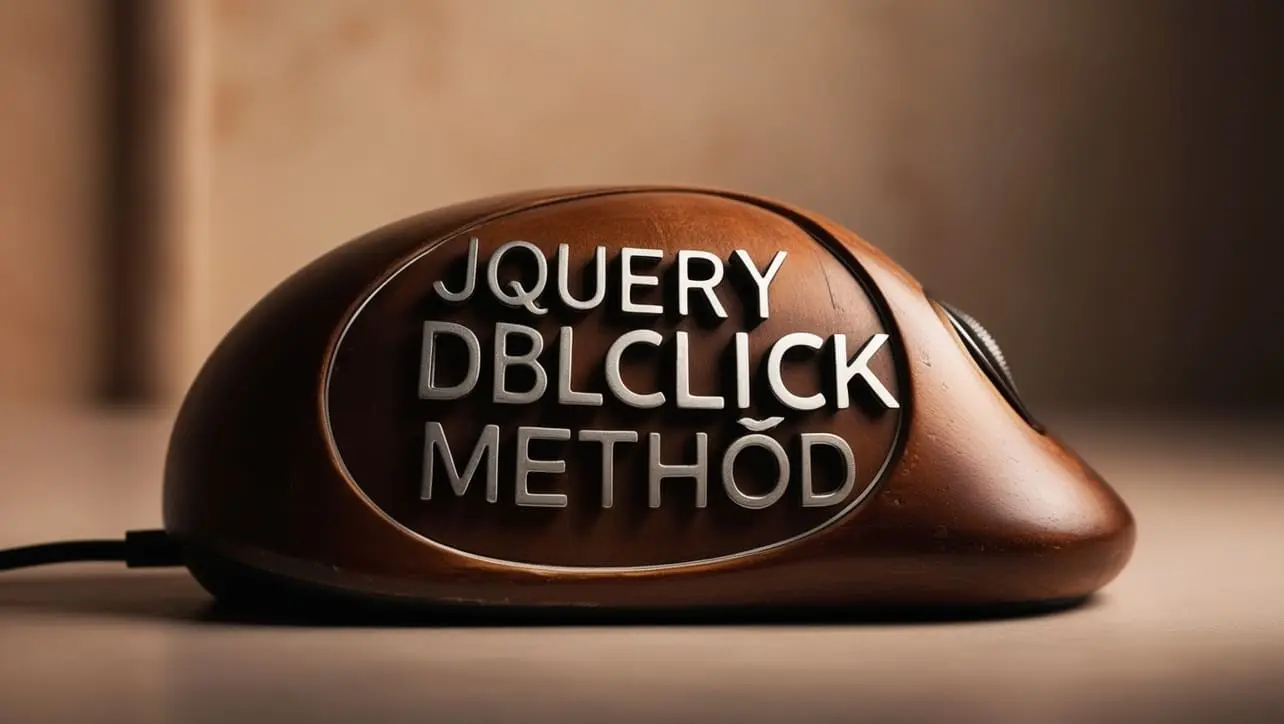
Photo Credit to CodeToFun
🙋 Introduction
jQuery empowers developers with a plethora of methods to enhance user interactions on web pages. One such method is .dblclick()
, which allows you to detect double-click events on elements. Leveraging this method enables you to create more responsive and interactive web applications.
In this comprehensive guide, we'll delve into the usage of the jQuery .dblclick()
method with clear examples to illustrate its versatility.
🧠 Understanding .dblclick() Method
The .dblclick()
method is specifically designed to trigger a function when a double-click event occurs on the selected element(s). This method simplifies the process of handling double-click actions, such as opening pop-up windows, toggling visibility, or executing custom behaviors.
💡 Syntax
The syntax for the .dblclick()
method is straightforward:
$(selector).dblclick(function);
📝 Example
Double-Clicking to Toggle Visibility:
Let's say you have an element that you want to hide or show when it's double-clicked. You can achieve this using the
.dblclick()
method as follows:index.htmlCopied<div id="toggleDiv">Double-click me!</div>
example.jsCopied$("#toggleDiv").dblclick(function() { $(this).toggle(); });
This code will toggle the visibility of the toggleDiv element whenever it's double-clicked.
Opening a Modal on Double-Click:
You can utilize the
.dblclick()
method to open a modal window when a certain element is double-clicked. Here's an example using Bootstrap modal:index.htmlCopied<button id="openModalBtn">Open Modal</button> <div class="modal" id="myModal"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title">Double-clicked Modal</h5> </div> <div class="modal-body"> This modal was opened by double-clicking a button. </div> <div class="modal-footer"> <button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button> </div> </div> </div> </div>
example.jsCopied$("#openModalBtn").dblclick(function() { $("#myModal").modal("show"); });
This code will open the modal when the button with the ID openModalBtn is double-clicked.
Custom Action on Double-Click:
You can define custom actions to be executed when an element is double-clicked. For instance, let's change the background color of a paragraph:
index.htmlCopied<p id="changeColorPara">Double-click to change color!</p>
example.jsCopied$("#changeColorPara").dblclick(function() { $(this).css("background-color", "lightgreen"); });
Double-clicking the paragraph will change its background color to light green.
🎉 Conclusion
The jQuery .dblclick()
method provides a straightforward way to handle double-click events on elements, enabling you to create more interactive and responsive web experiences. Whether you need to toggle visibility, open modal windows, or execute custom actions, this method offers flexibility and ease of implementation.
By incorporating .dblclick()
into your web development arsenal, you can enhance user interactions and elevate the overall user experience.
👨💻 Join our Community:
Author
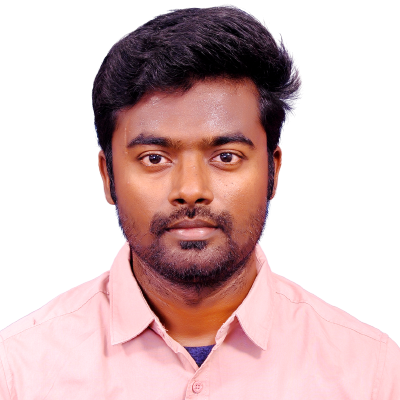
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .dblclick() Method), please comment here. I will help you immediately.