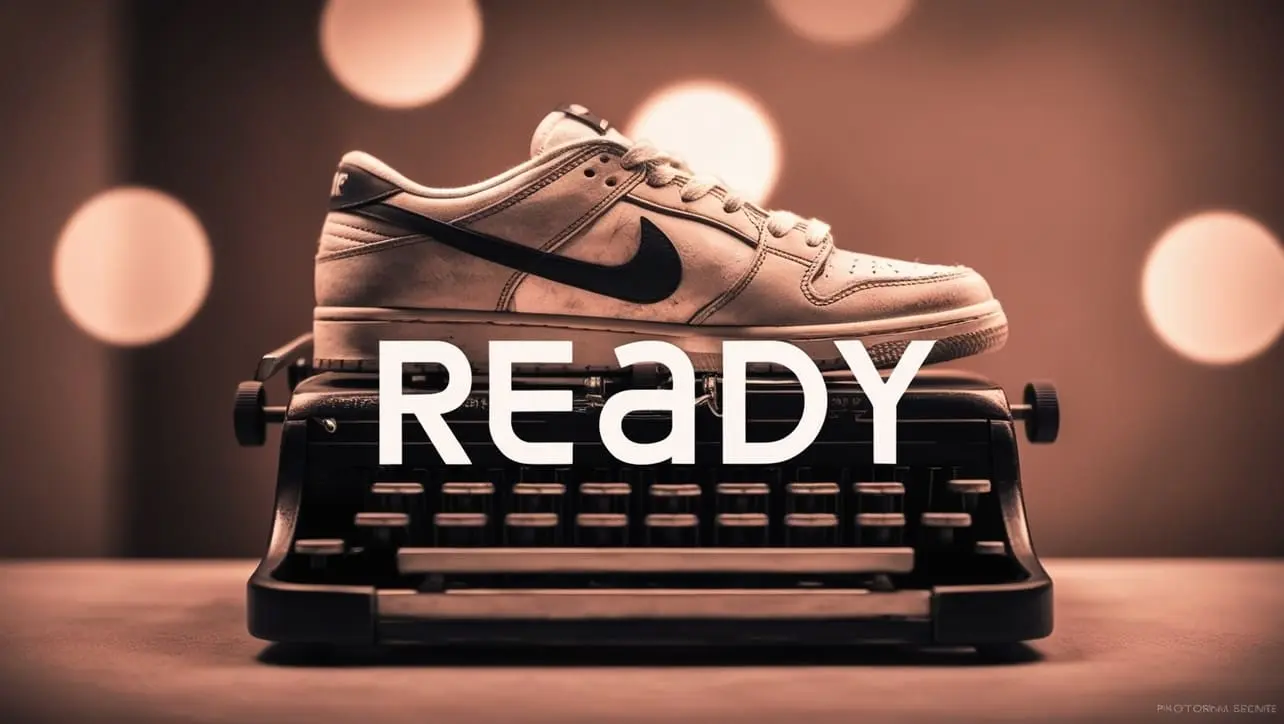
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery jQuery.cssHooks Property
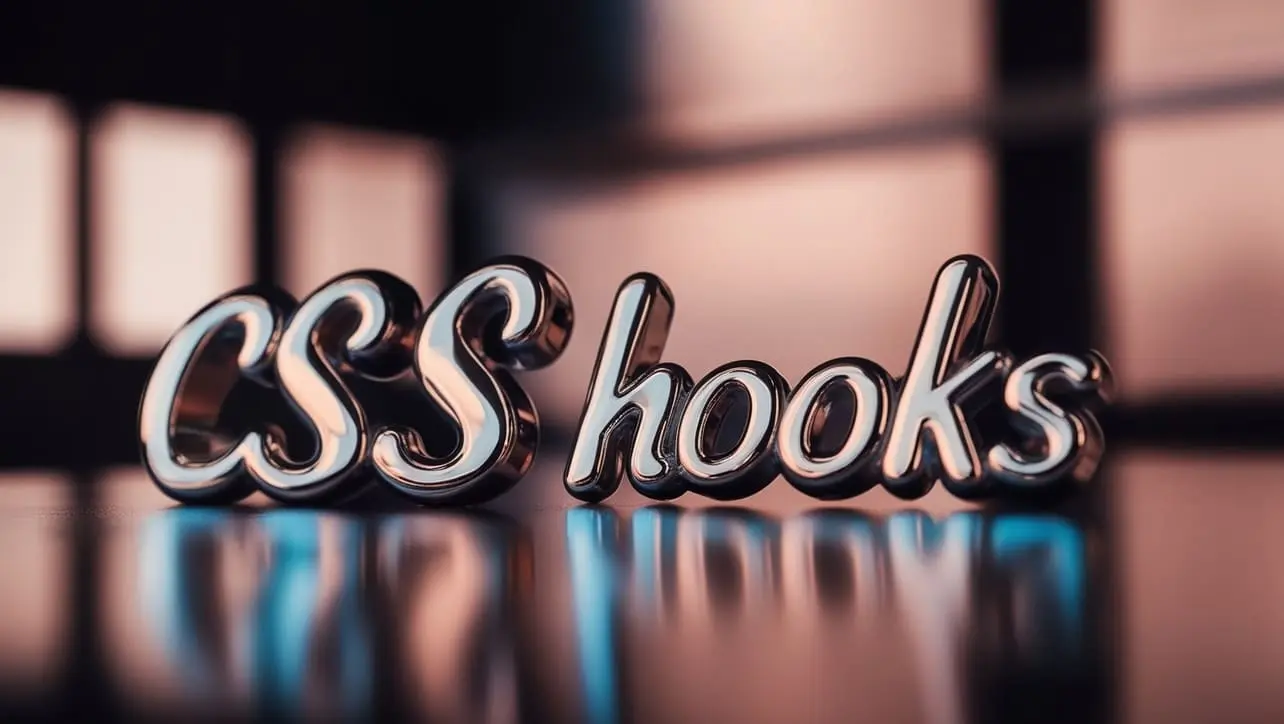
Photo Credit to CodeToFun
🙋 Introduction
jQuery is a versatile library that enhances web development by providing numerous methods for manipulating HTML elements, handling events, and interacting with CSS. One advanced feature that jQuery offers is the jQuery.cssHooks
property. This property allows you to extend jQuery's capabilities to support custom CSS properties and provides a way to define getter and setter functions for CSS properties.
In this guide, we will explore the jQuery.cssHooks
property in detail and provide examples to demonstrate its usage.
🧠 Understanding jQuery.cssHooks Property
The jQuery.cssHooks
property is an object that allows you to define custom getter and setter functions for CSS properties. This can be particularly useful for handling browser inconsistencies, adding support for new CSS properties, or manipulating properties that are not directly accessible through standard jQuery methods.
💡 Syntax
The syntax for the jQuery.cssHooks
property is straightforward:
jQuery.cssHooks[property] = {
get: function(element, computed, extra) {
// Return the value of the custom property
},
set: function(element, value) {
// Set the value of the custom property
}
};
📝 Example
Creating a Custom CSS Hook:
Let's create a custom CSS hook for the backgroundColor property. This example demonstrates how to get and set the background color of an element using a custom hook.
example.jsCopiedjQuery.cssHooks.backgroundColor = { get: function(element, computed, extra) { return $(element).css('background-color'); }, set: function(element, value) { element.style.backgroundColor = value; } };
Now you can use this hook to get and set the background color of an element:
index.htmlCopied<div id="myDiv">Hello World!</div>
example.jsCopied$("#myDiv").css("backgroundColor", "lightgreen"); console.log($("#myDiv").css("backgroundColor"));
Handling Browser Inconsistencies:
Some CSS properties are not consistent across different browsers. Using
jQuery.cssHooks
, you can create hooks to handle these inconsistencies. For example, let's create a hook for the opacity property that ensures consistent behavior across all browsers:example.jsCopiedjQuery.cssHooks.opacity = { get: function(element, computed) { if (computed) { // Use jQuery's built-in method for cross-browser compatibility var opacity = jQuery.style(element, "opacity"); return opacity === "" ? "1" : opacity; } }, set: function(element, value) { element.style.opacity = value; } };
Adding Support for New CSS Properties:
As web standards evolve, new CSS properties are introduced. With
jQuery.cssHooks
, you can add support for these new properties even if they are not yet supported by jQuery. For example, let's add support for the hypothetical customProperty:example.jsCopiedjQuery.cssHooks.customProperty = { get: function(element, computed) { return element.style.getPropertyValue('--custom-property') || 'default'; }, set: function(element, value) { element.style.setProperty('--custom-property', value); } };
Now you can use the customProperty just like any other CSS property in jQuery:
example.jsCopied$("#myDiv").css("customProperty", "customValue"); console.log($("#myDiv").css("customProperty"));
🎉 Conclusion
The jQuery.cssHooks
property is a powerful feature that extends the flexibility of jQuery in handling CSS properties. By defining custom getter and setter functions, you can manage browser inconsistencies, add support for new CSS properties, and manipulate properties that are not directly accessible.
Mastering jQuery.cssHooks
can greatly enhance your ability to create dynamic and cross-browser compatible web pages.
👨💻 Join our Community:
Author
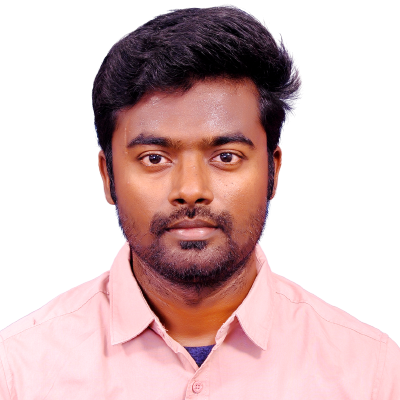
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.cssHooks Property), please comment here. I will help you immediately.