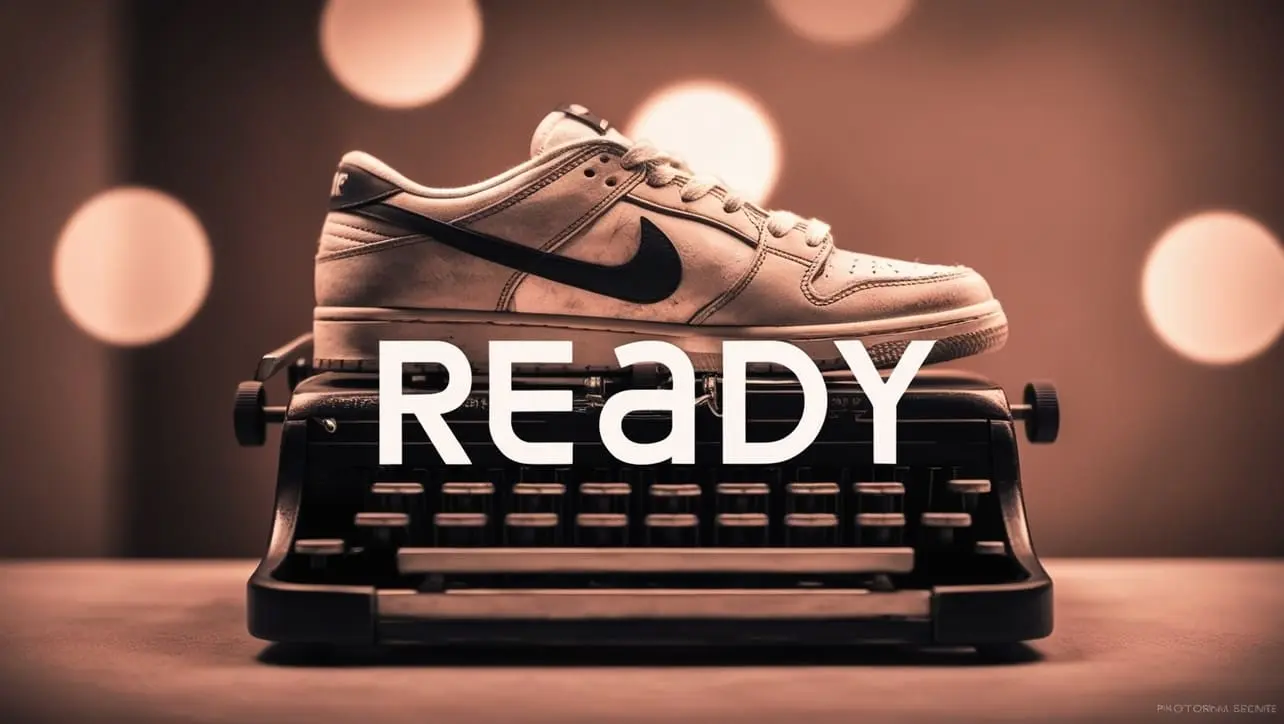
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .context Property
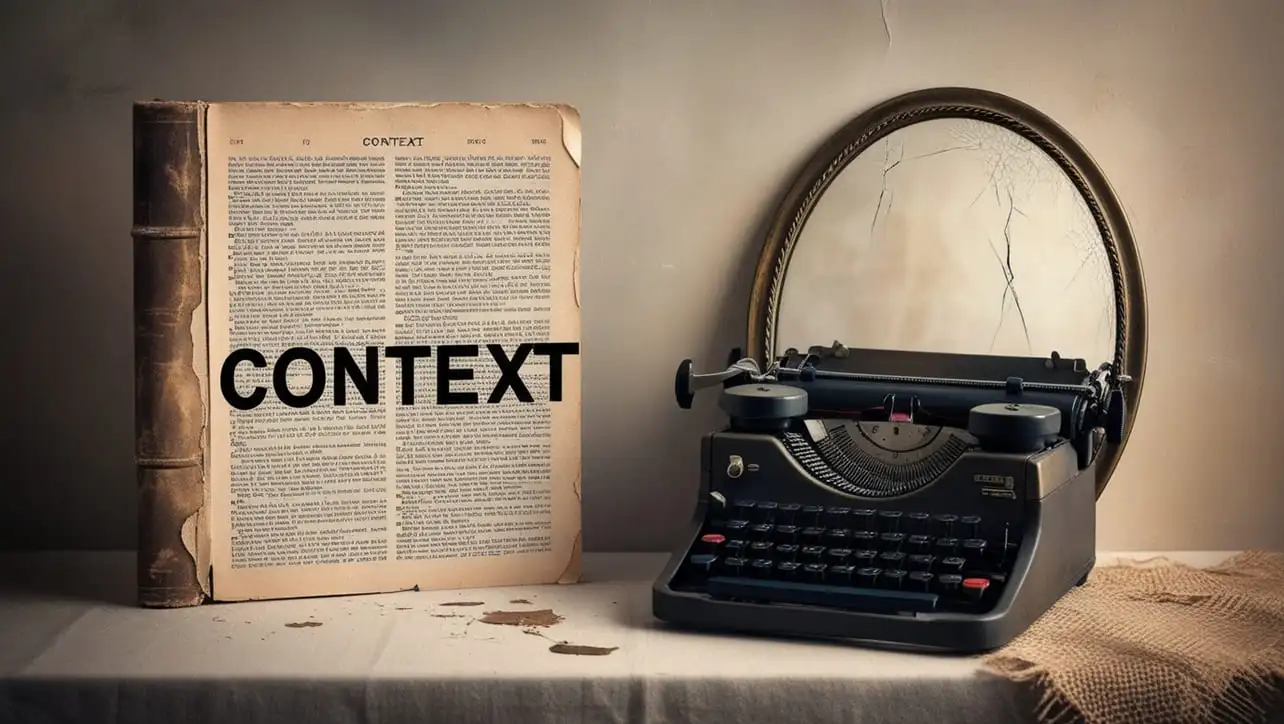
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a wide range of tools and properties to simplify web development. One of these useful properties is the .context
property, which provides context about the selector used in a jQuery object. Understanding the .context
property can help you better comprehend the scope and origin of your jQuery selectors, aiding in debugging and enhancing your code's clarity.
In this guide, we'll explore the .context
property with detailed explanations and practical examples.
🧠 Understanding .context Property
The .context
property in jQuery refers to the DOM node that served as the context for the jQuery object when it was created. It essentially indicates the starting point from which the jQuery selector was run. This property is particularly useful when you need to understand or manipulate the scope of your jQuery operations.
💡 Syntax
The syntax for the .context
property is straightforward:
$(selector, context).context
📝 Example
Basic Usage of .context:
Let's start with a simple example to understand the basic usage of the
.context
property.index.htmlCopied<div id="container"> <p>Paragraph 1</p> <p>Paragraph 2</p> </div>
example.jsCopiedvar contextExample = $("#container p").context; console.log(contextExample);
In this example, the
.context
property will refer to the document because no specific context was provided.Using a Specific Context:
You can specify a particular context for your jQuery selector to limit the scope of the selection.
index.htmlCopied<div id="wrapper"> <div id="container"> <p>Paragraph 1</p> <p>Paragraph 2</p> </div> <div id="anotherContainer"> <p>Paragraph 3</p> <p>Paragraph 4</p> </div> </div>
example.jsCopiedvar specificContextExample = $("p", "#container").context; console.log(specificContextExample);
In this case, the
.context
property will refer to the #container div, as it was specified as the context for the selector.Comparing Contexts:
Understanding how different contexts affect your jQuery selectors can be very useful.
example.jsCopiedvar defaultContext = $("p").context; var specifiedContext = $("p", "#container").context; console.log(defaultContext); // Logs the document console.log(specifiedContext); // Logs the #container element
This comparison shows how specifying a context changes the origin from which the selector operates.
Practical Example - Manipulating Elements within a Specific Context:
Using the
.context
property, you can perform actions within a specific part of the DOM more efficiently.index.htmlCopied<div id="main"> <div id="section1"> <button>Click Me</button> </div> <div id="section2"> <button>Click Me</button> </div> </div>
example.jsCopied$("#section1 button").click(function() { var contextElement = $(this).context; $(contextElement).css("background-color", "yellow"); });
Here, clicking the button in #section1 will change the background color of #section1 to yellow, demonstrating the use of
.context
to affect a specific part of the DOM.
🎉 Conclusion
The jQuery .context
property is a powerful feature that provides insight into the scope and origin of your jQuery selectors. By understanding and utilizing the .context
property, you can write more precise and efficient jQuery code.
Whether you're debugging complex selectors or working within specific parts of your DOM, the .context
property is an invaluable tool for web developers.
👨💻 Join our Community:
Author
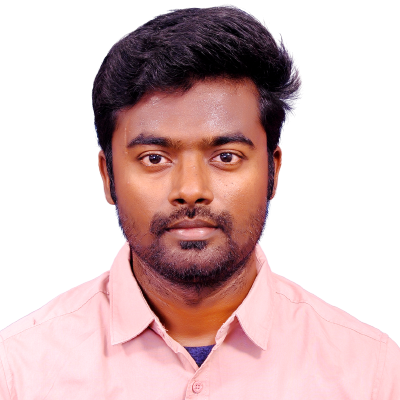
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .context Property), please comment here. I will help you immediately.