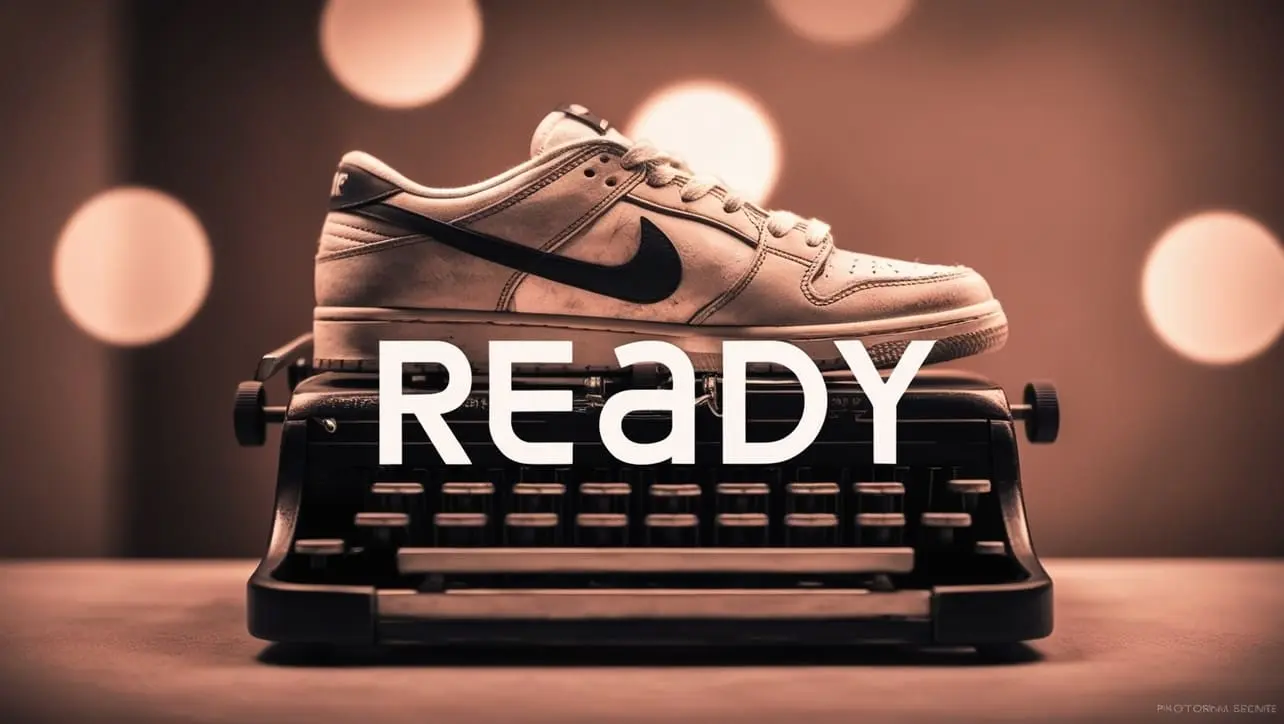
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .closest() Method
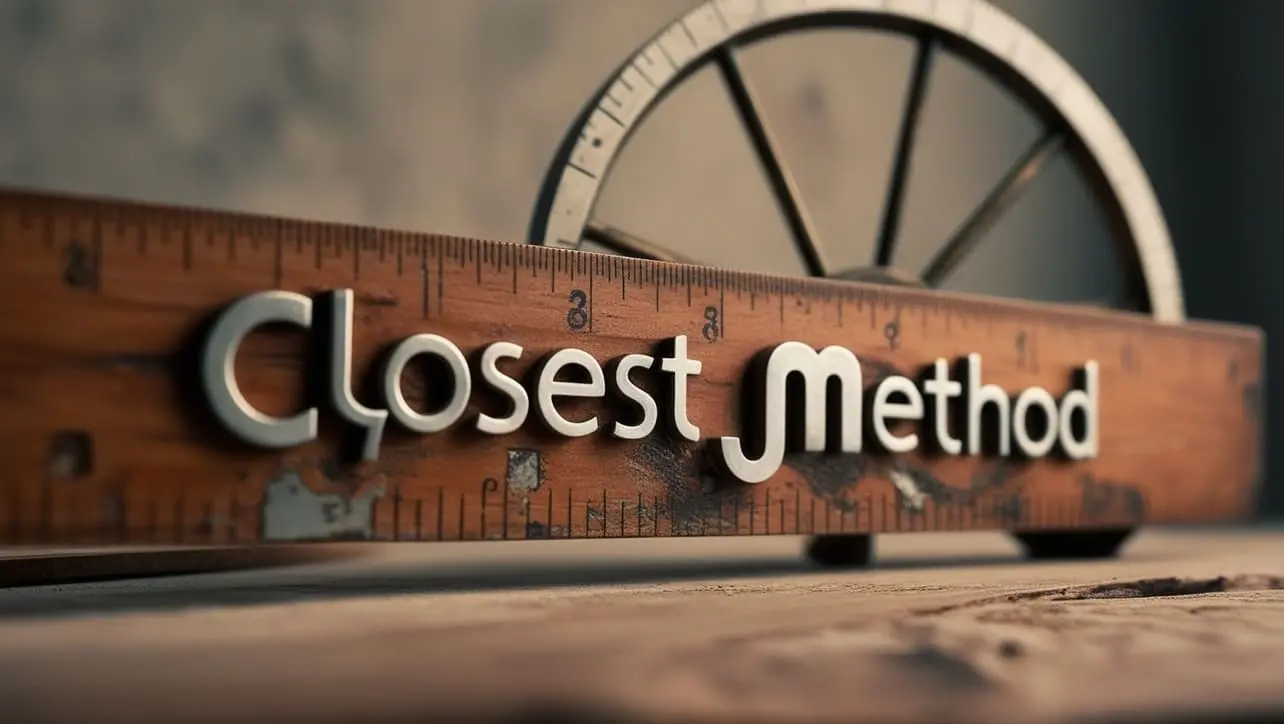
Photo Credit to CodeToFun
🙋 Introduction
In jQuery, traversing the DOM (Document Object Model) is a common task when manipulating elements. The .closest()
method is a valuable tool for navigating up through the DOM tree to find the closest ancestor that matches a selector. This method simplifies DOM traversal, making it easier to locate specific elements relative to the current context.
In this guide, we'll explore the usage of the jQuery .closest()
method with clear examples to illustrate its functionality and versatility.
🧠 Understanding .closest() Method
The .closest()
method in jQuery is used to search through the ancestors of the selected elements in the DOM tree and retrieve the first element that matches the specified selector. It traverses upwards from the current element until it finds a match or reaches the root of the DOM tree.
💡 Syntax
The syntax for the .closest()
method is straightforward:
.closest(selector)
📝 Example
Finding the Closest Parent Element:
Suppose you have a nested structure of HTML elements and you want to find the closest parent <div> element of a specific <span> element with a class of .target. You can achieve this using the
.closest()
method as follows:index.htmlCopied<div> <p> <span class="target">Target Element</span> </p> </div>
example.jsCopied$(".target").closest("div").css("border", "2px solid red");
This will add a red border to the closest parent <div> element of the .target span.
Traversing Multiple Levels Up the DOM Tree:
You can traverse multiple levels up the DOM tree by chaining the
.closest()
method. For example, let's find the closest ancestor <div> element with a class of .container starting from an <a> element:index.htmlCopied<div class="container"> <div> <a href="#">Link</a> </div> </div>
example.jsCopied$("a").closest("div").closest(".container").css("background-color", "lightblue");
This will set the background color of the closest ancestor <div> with the class .container to light blue.
Using .closest() with Event Handling:
The
.closest()
method is often used in event handling to target specific elements relative to the event trigger. For instance, let's attach a click event to all buttons and change the text color of their closest parent <div>:index.htmlCopied<div> <button>Button 1</button> </div> <div> <button>Button 2</button> </div>
example.jsCopied$("button").click(function() { $(this).closest("div").css("color", "green"); });
This will change the text color of the closest parent <div> of the clicked button to green.
Efficiently Targeting Elements:
When using
.closest()
, ensure that the selector passed as an argument accurately reflects the element you want to target. This helps in efficiently locating the desired ancestor element without unnecessary traversal.
🎉 Conclusion
The jQuery .closest()
method provides a convenient way to navigate up through the DOM tree and locate ancestor elements based on a specified selector. Whether you need to find the closest parent element, traverse multiple levels up the DOM tree, or handle events relative to specific elements, this method offers a versatile solution.
By mastering its usage, you can streamline DOM traversal and enhance the interactivity and functionality of your web applications.
👨💻 Join our Community:
Author
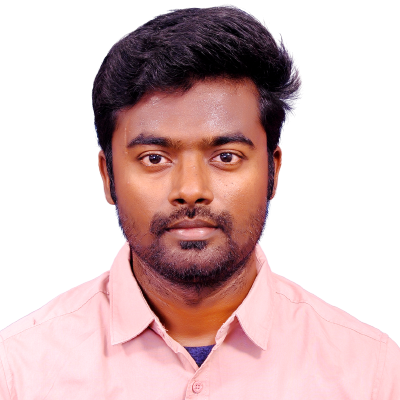
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .closest() Method), please comment here. I will help you immediately.