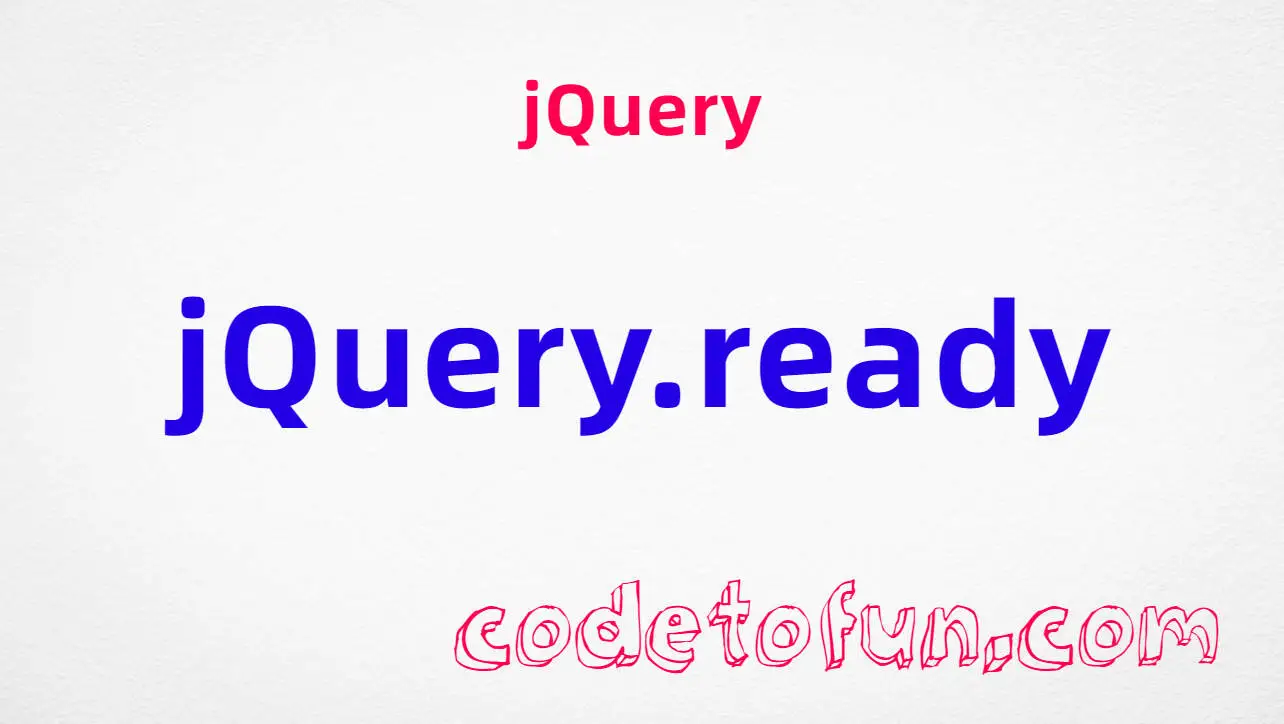
jQuery Basic
jQuery Callbacks
jQuery callbacks.fireWith() Method
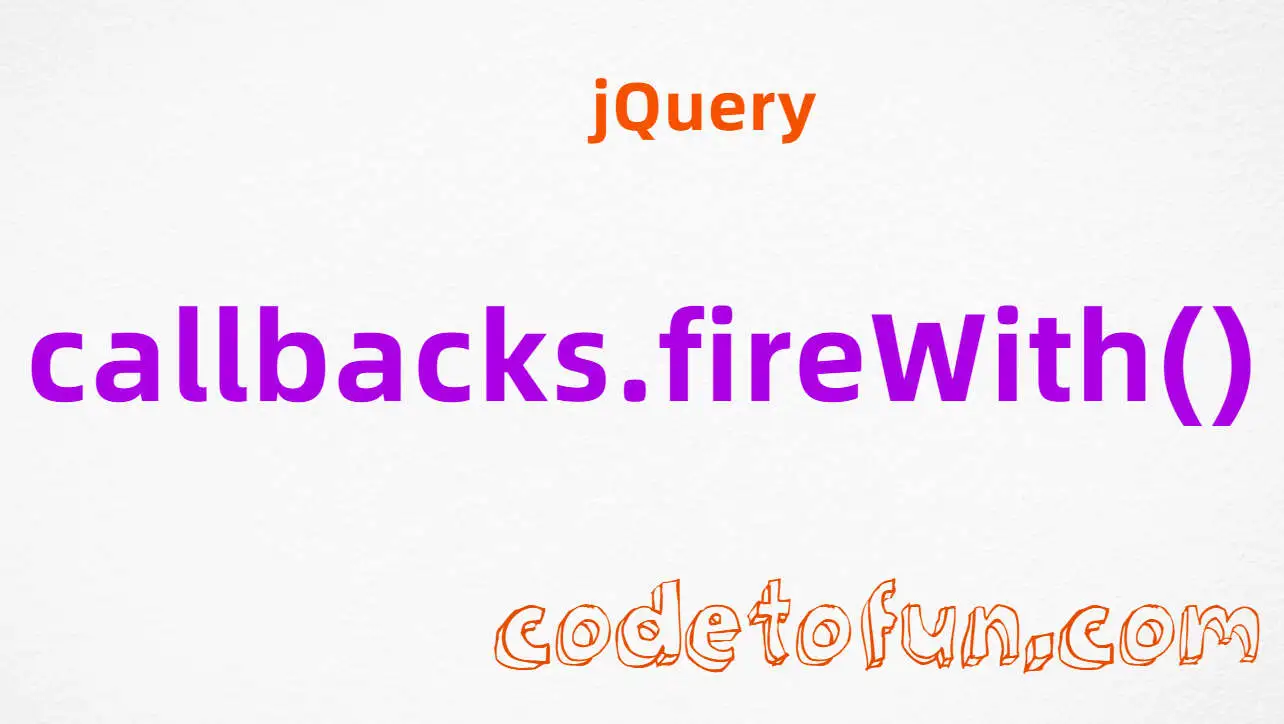
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a myriad of tools to manage the flow of asynchronous code, enabling developers to create dynamic and interactive web applications. Among these tools is the callbacks.fireWith()
method, a versatile function that facilitates the execution of callback functions with a specific context and arguments.
In this comprehensive guide, we'll explore the intricacies of callbacks.fireWith()
, including its syntax, practical applications, and best practices for implementation.
🧠 Understanding callbacks.fireWith() Method
The callbacks.fireWith()
method is an essential component of jQuery's Callbacks Object API, designed to execute a set of callback functions in a specified context, with custom arguments if needed. It is particularly useful in scenarios where you need to trigger a series of functions asynchronously, passing along relevant data.
💡 Syntax
The syntax for the callbacks.fireWith()
method is straightforward:
callbacks.fireWith( context [, args ] )
- context: The context in which the callback functions should be executed. This can be any JavaScript object.
- args (optional): An array or array-like object containing arguments to be passed to the callback functions.
📝 Example
Let's dive into a simple example to illustrate the usage of the callbacks.fireWith()
method:
var callbacks = $.Callbacks();
callbacks.add(function(message) {
console.log("First callback:", message);
});
callbacks.add(function(message) {
console.log("Second callback:", message.toUpperCase());
});
callbacks.fireWith(window, ["Hello, world!"]);
🏆 Best Practices
When working with the callbacks.fireWith()
method, consider the following best practices:
Context Sensitivity:
Choose an appropriate context for executing callback functions, ensuring access to necessary variables, functions, or properties.
Argument Consistency:
Provide consistent and meaningful arguments when firing callbacks, promoting clarity and predictability in your codebase.
Error Handling:
Implement error handling mechanisms within callback functions to gracefully manage unexpected exceptions or failures.
Documentation:
Document the purpose and usage of callback functions within your codebase to facilitate collaboration and maintainability.
Testing:
Thoroughly test callback functions and their associated behaviors to validate functionality and identify potential edge cases.
📚 Use Cases
Custom Event Handling:
Execute callback functions in response to custom events triggered within your application, allowing for modular and extensible code architecture.
Plugin Development:
Utilize
callbacks.fireWith()
within jQuery plugins to notify subscribers of specific events or state changes, enhancing the plugin's flexibility and usability.Asynchronous Operations:
Coordinate the execution of callback functions in asynchronous operations such as AJAX requests, animations, or timeouts, ensuring proper sequencing and synchronization.
Dynamic Content Updates:
Trigger callback functions to update dynamic content on webpages based on user interactions, server responses, or other external events.
🎉 Conclusion
The callbacks.fireWith()
method in jQuery empowers developers to orchestrate the execution of callback functions with precision and flexibility, enabling the creation of dynamic and responsive web applications.
By mastering its syntax, exploring practical applications, and adhering to best practices, you can leverage callbacks.fireWith()
to streamline your code and enhance the user experience. Incorporate this powerful tool into your toolkit to unlock the full potential of asynchronous JavaScript programming with jQuery.
👨💻 Join our Community:
Author
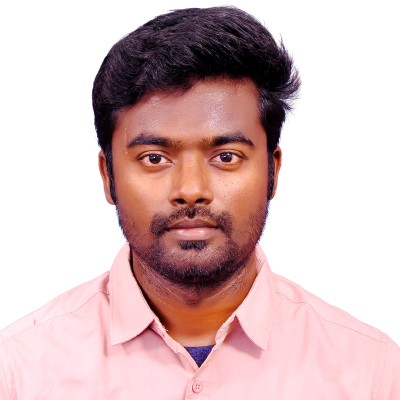
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee