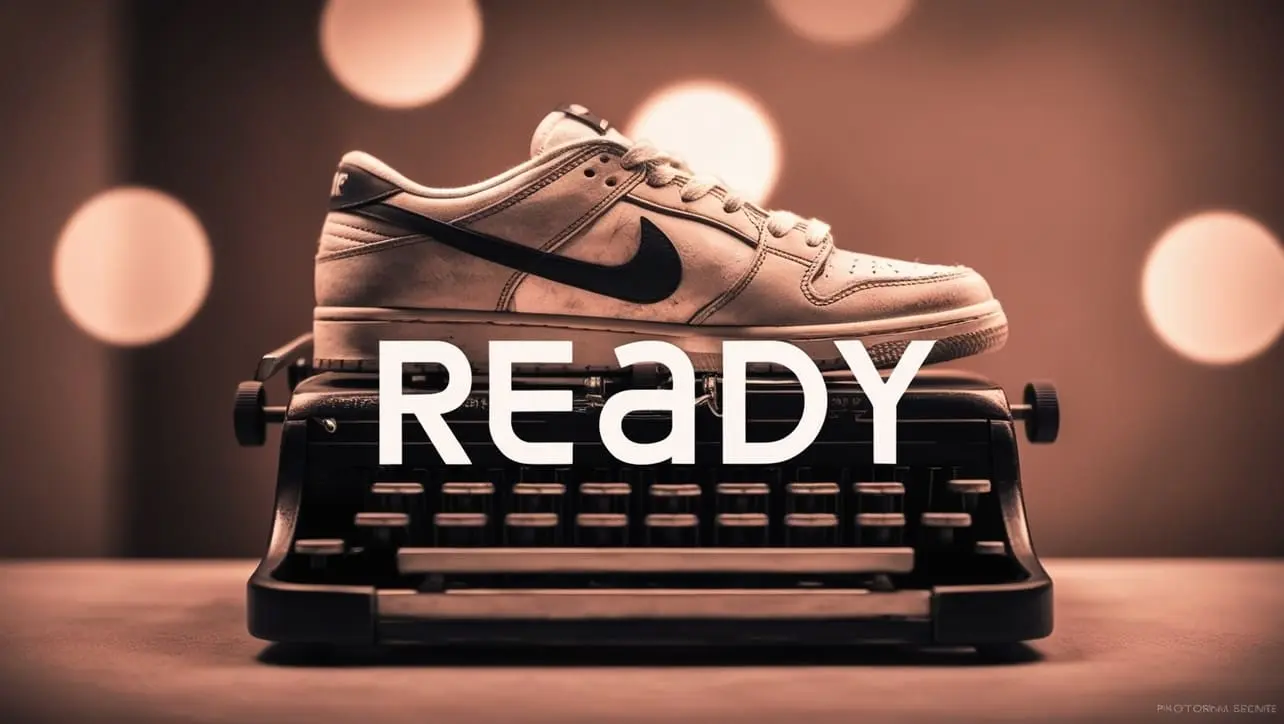
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery jQuery.ajaxSetup() Method
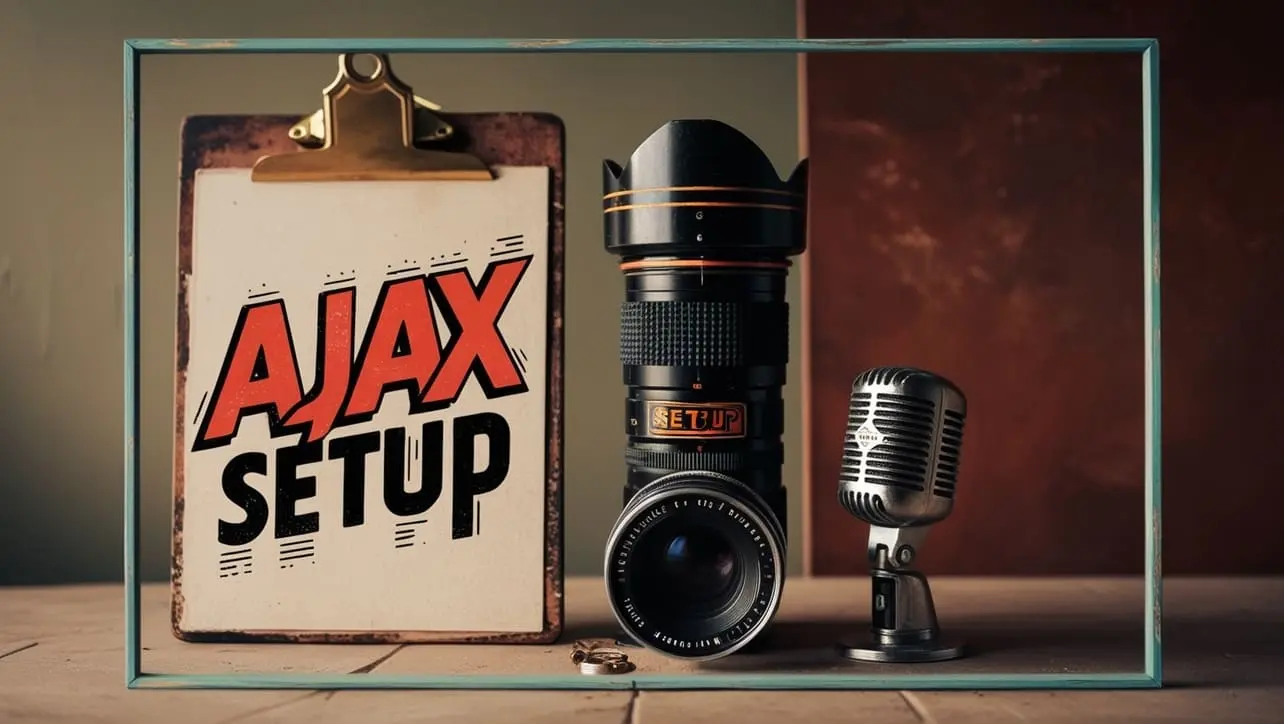
Photo Credit to CodeToFun
🙋 Introduction
jQuery provides a variety of methods to facilitate AJAX (Asynchronous JavaScript and XML) interactions, enabling web applications to retrieve data from a server asynchronously without interfering with the display and behavior of the existing page. One powerful tool in jQuery's arsenal is the jQuery.ajaxSetup()
method. This method allows you to configure global AJAX settings, simplifying your code and ensuring consistency across multiple AJAX requests.
In this guide, we'll explore how to use jQuery.ajaxSetup()
effectively with clear examples to enhance your web development skills.
🧠 Understanding jQuery.ajaxSetup() Method
The jQuery.ajaxSetup()
method is used to set default values for future AJAX requests. By configuring global settings, you can avoid repetition and ensure that all AJAX requests adhere to a consistent configuration, making your code cleaner and more maintainable.
💡 Syntax
The syntax for the jQuery.ajaxSetup()
method is straightforward:
jQuery.ajaxSetup(options)
Parameters:
- options: An object containing key-value pairs to configure the default settings for AJAX requests.
📝 Example
Setting Default AJAX Options:
Suppose you frequently make AJAX requests to the same server and need to include certain headers or settings for each request. You can use
jQuery.ajaxSetup()
to set these defaults once:example.jsCopied$.ajaxSetup({ url: 'https://api.example.com/data', method: 'GET', dataType: 'json', headers: { 'Authorization': 'Bearer YOUR_API_KEY' } });
This configuration ensures that every AJAX request you make will use the specified URL, method, data type, and headers unless overridden.
Making an AJAX Request with Default Settings:
With the default settings configured, making an AJAX request becomes simpler:
example.jsCopied$.ajax({ success: function(response) { console.log(response); } });
This request will automatically use the defaults set by $.ajaxSetup().
Overriding Default Settings:
If you need to override the default settings for a specific request, you can do so by passing the new settings directly into the $.ajax() method:
example.jsCopied$.ajax({ url: 'https://api.example.com/other-data', method: 'POST', data: { key: 'value' }, success: function(response) { console.log(response); } });
This request will use the new URL and method specified, while other settings will still fall back to the defaults.
Handling Global AJAX Events:
Using $.ajaxSetup(), you can also set global event handlers for AJAX requests, such as beforeSend, complete, and error. This is useful for adding uniform behavior across all AJAX requests:
example.jsCopied$.ajaxSetup({ beforeSend: function() { console.log('AJAX request is about to be sent'); }, complete: function() { console.log('AJAX request completed'); }, error: function(jqXHR, textStatus, errorThrown) { console.error('AJAX request failed:', textStatus, errorThrown); } });
Resetting AJAX Settings:
If you need to reset the AJAX settings to their defaults at any point, you can call $.ajaxSetup({}) with an empty object:
example.jsCopied$.ajaxSetup({});
This will clear any settings previously defined.
🎉 Conclusion
The jQuery jQuery.ajaxSetup()
method is an invaluable tool for setting default configurations for AJAX requests. By using this method, you can ensure consistency across your AJAX interactions, reduce repetitive code, and handle global events efficiently.
Mastering jQuery.ajaxSetup()
will streamline your AJAX implementation, making your web applications more robust and easier to maintain.
👨💻 Join our Community:
Author
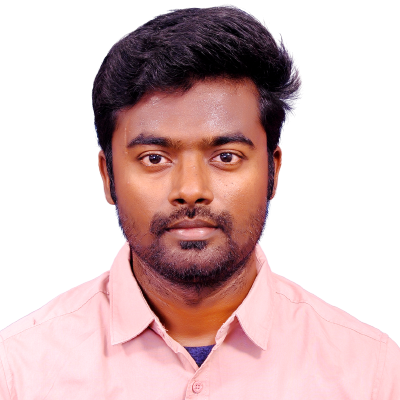
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.ajaxSetup() Method), please comment here. I will help you immediately.