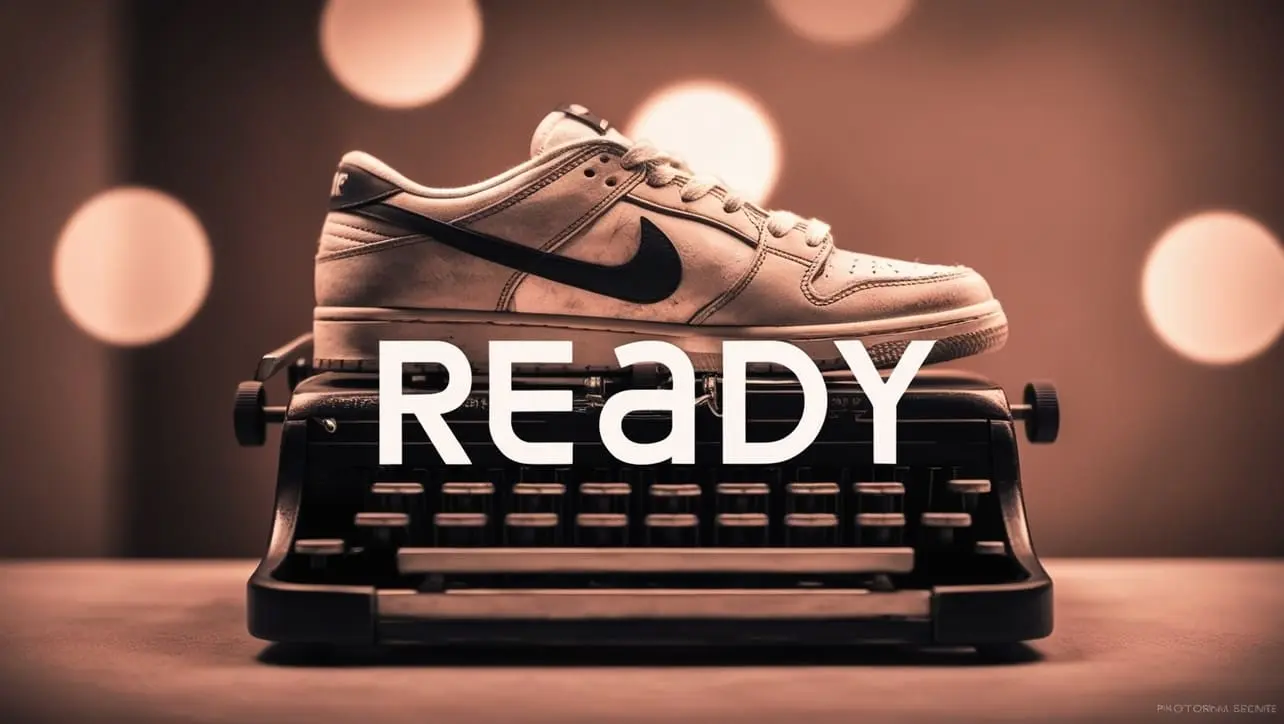
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .ajaxSend() Method
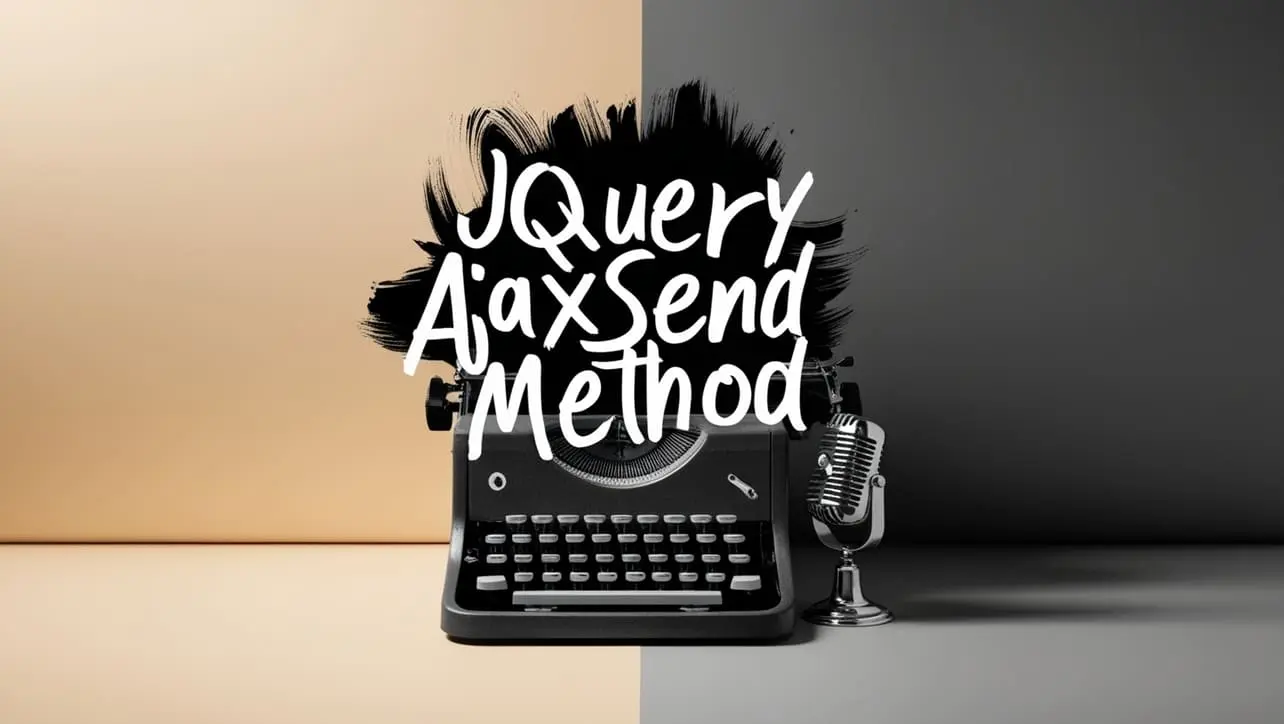
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a robust set of tools for handling AJAX requests, making asynchronous data retrieval and manipulation seamless. One such tool is the ajaxSend()
method, an event handler that executes before an AJAX request is sent. This method allows developers to perform actions or modify the settings of the request dynamically.
In this guide, we'll explore the usage of the ajaxSend()
method with clear examples to help you understand its potential.
🧠 Understanding .ajaxSend() Method
The ajaxSend()
method in jQuery is triggered just before an AJAX request is sent. It provides an opportunity to run custom code, modify request parameters, or perform any pre-request setup. This method is particularly useful for adding custom headers, displaying loading indicators, or logging request details.
💡 Syntax
The syntax for the .ajaxSend()
method is straightforward:
$(document).ajaxSend(function(event, jqXHR, ajaxOptions) {
// Your code here
});
Parameters:
- event: The jQuery event object.
- jqXHR: The jQuery-wrapped XMLHttpRequest object.
- ajaxOptions: The options used in the AJAX request.
📝 Example
Adding Custom Headers:
Suppose you need to add a custom header to all AJAX requests. You can use the
ajaxSend()
method to achieve this:example.jsCopied$(document).ajaxSend(function(event, jqXHR, ajaxOptions) { jqXHR.setRequestHeader("Authorization", "Bearer your-auth-token"); });
This will add an Authorization header with the specified token to every AJAX request.
Displaying a Loading Indicator:
You can show a loading indicator to improve user experience while an AJAX request is in progress:
index.htmlCopied<div id="loadingIndicator" style="display:none;">Loading...</div>
example.jsCopied$(document).ajaxSend(function(event, jqXHR, ajaxOptions) { $("#loadingIndicator").show(); });
This will display the loading indicator whenever an AJAX request is sent.
Logging Request Details:
For debugging purposes, you might want to log the details of each AJAX request:
example.jsCopied$(document).ajaxSend(function(event, jqXHR, ajaxOptions) { console.log("Request URL: " + ajaxOptions.url); console.log("Request Type: " + ajaxOptions.type); });
This will log the URL and request type of each AJAX request to the console.
Hiding the Loading Indicator on Completion:
To hide the loading indicator once the AJAX request is complete, use the ajaxComplete() method:
example.jsCopied$(document).ajaxComplete(function(event, jqXHR, ajaxOptions) { $("#loadingIndicator").hide(); });
Combining
ajaxSend()
and ajaxComplete() ensures the loading indicator is displayed during the request and hidden afterward.Conditionally Modifying Requests:
You can conditionally modify AJAX requests based on specific criteria:
example.jsCopied$(document).ajaxSend(function(event, jqXHR, ajaxOptions) { if (ajaxOptions.url.includes("secure")) { jqXHR.setRequestHeader("Authorization", "Bearer secure-token"); } });
This adds an Authorization header only to requests with URLs containing secure.
🎉 Conclusion
The jQuery ajaxSend()
method is a versatile tool for executing code before an AJAX request is sent. Whether you need to add custom headers, display loading indicators, log request details, or modify requests conditionally, ajaxSend()
provides a convenient and efficient solution.
By mastering this method, you can enhance the functionality and user experience of your web applications.
👨💻 Join our Community:
Author
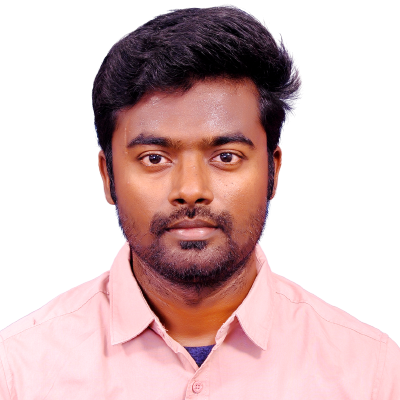
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .ajaxSend() Method), please comment here. I will help you immediately.