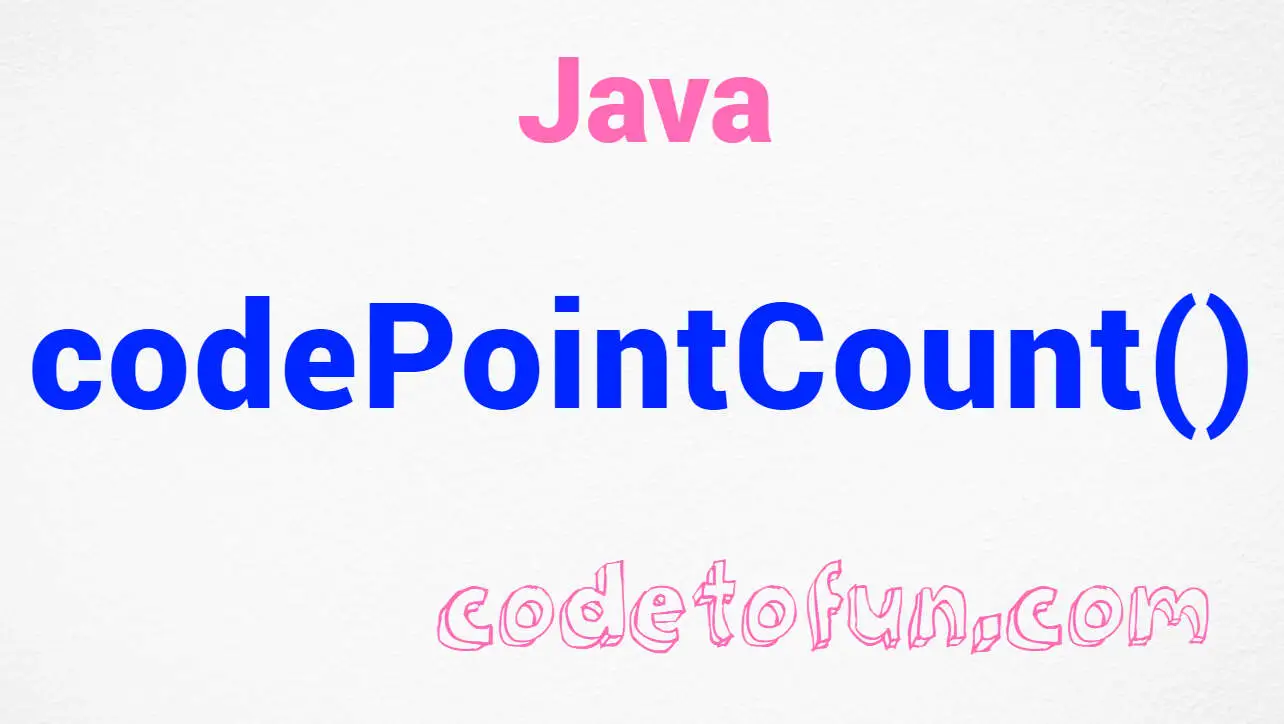
Java Basic
Java Interview Programs
- Java Interview Programs
- Java Abundant Number
- Java Amicable Number
- Java Armstrong Number
- Java Average of N Numbers
- Java Automorphic Number
- Java Biggest of three numbers
- Java Binary to Decimal
- Java Common Divisors
- Java Composite Number
- Java Condense a Number
- Java Cube Number
- Java Decimal to Binary
- Java Decimal to Octal
- Java Disarium Number
- Java Even Number
- Java Evil Number
- Java Factorial of a Number
- Java Fibonacci Series
- Java GCD
- Java Happy Number
- Java Harshad Number
- Java LCM
- Java Leap Year
- Java Magic Number
- Java Matrix Addition
- Java Matrix Division
- Java Matrix Multiplication
- Java Matrix Subtraction
- JS Matrix Transpose
- Java Maximum Value of an Array
- Java Minimum Value of an Array
- Java Multiplication Table
- Java Natural Number
- Java Number Combination
- Java Odd Number
- Java Palindrome Number
- Java Pascalβs Triangle
- Java Perfect Number
- Java Perfect Square
- Java Power of 2
- Java Power of 3
- Java Pronic Number
- Java Prime Factor
- Java Prime Number
- Java Smith Number
- Java Strong Number
- Java Sum of Array
- Java Sum of Digits
- Java Swap Two Numbers
- Java Triangular Number
Java Program to Check Triangular Number
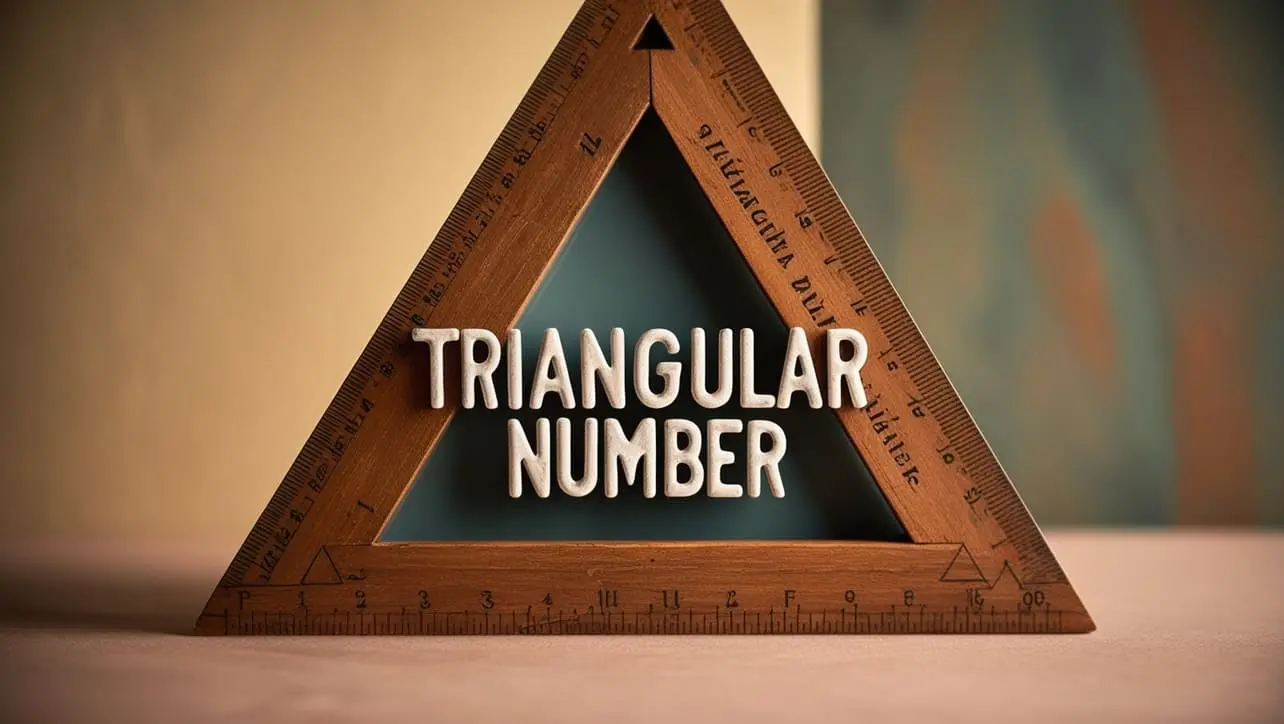
Photo Credit to CodeToFun
π Introduction
In the realm of programming, various mathematical properties of numbers often pique our interest. One such property is whether a number is a triangular number.
A triangular number or triangle number is the sum of the natural numbers up to a certain value.
In this tutorial, we'll delve into a Java program to check if a given number is a triangular number.
π Example
Let's explore the Java code that checks whether a given number is a triangular number.
public class TriangularNumberChecker {
// Function to check if a number is a triangular number
static boolean isTriangularNumber(int num) {
// Formula to check triangular number
int n = (int) Math.sqrt(2 * num);
return (n * (n + 1)) / 2 == num;
}
// Driver program
public static void main(String[] args) {
// Replace this value with your desired number
int number = 10;
// Check if the number is a triangular number
if (isTriangularNumber(number)) {
System.out.println(number + " is a triangular number.");
} else {
System.out.println(number + " is not a triangular number.");
}
}
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main method.
10 is a triangular number.
Compile and run the program to check if the given number is a triangular number.
π§ How the Program Works
- The program defines a class TriangularNumberChecker containing a static method isTriangularNumber that checks if a given number is a triangular number using the formula.
- Inside the main method, replace the value of number with the desired number to check.
- The program then calls the isTriangularNumber method and prints whether the number is a triangular number or not.
π Between the Given Range
Let's take a look at the java code that checks and displays triangular numbers in the range of 1 to 50.
public class TriangularNumbers {
// Function to check if a number is triangular
static boolean isTriangular(int num) {
int sum = 0;
int n = 1;
while (sum < num) {
sum += n;
++n;
}
return sum == num;
}
// Function to display triangular numbers in the range 1 to 50
static void displayTriangularNumbers() {
System.out.println("Triangular Numbers in the Range 1 to 50:");
for (int i = 1; i <= 50; ++i) {
if (isTriangular(i)) {
System.out.print(i + " ");
}
}
System.out.println();
}
// Driver program
public static void main(String[] args) {
// Call the function to display triangular numbers
displayTriangularNumbers();
}
}
π» Testing the Program
Triangular Numbers in the Range 1 to 50: 1 3 6 10 15 21 28 36 45
Compile and run the program to see the triangular numbers in the range of 1 to 50.
π§ How the Program Works
- The program defines a class TriangularNumbers containing two static functions: isTriangular and displayTriangularNumbers.
- The isTriangular function checks if a given number is triangular using the formula for triangular numbers.
- The displayTriangularNumbers function iterates through the range of 1 to 50 and prints triangular numbers.
- The main function calls the displayTriangularNumbers function to output the triangular numbers in the specified range.
π§ Understanding the Concept of Triangular Numbers
Before delving into the code, let's take a moment to understand the concept of triangular numbers.
A triangular number is the sum of the natural numbers up to a certain value, and it can be represented by the formula Tn = n * (n + 1) / 2, where n is a non-negative integer.
For example, T3 = 1 + 2 + 3 = 6 is a triangular number.
π’ Optimizing the Program
While the provided program is effective, you can explore and implement optimizations based on the properties of triangular numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
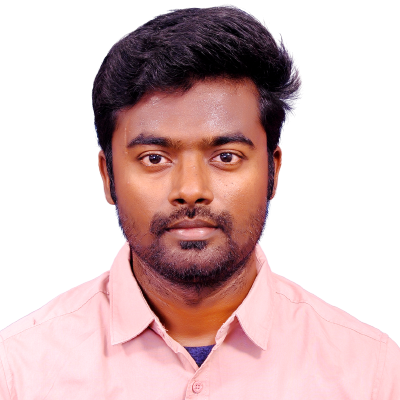
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Program to Check Triangular Number), please comment here. I will help you immediately.