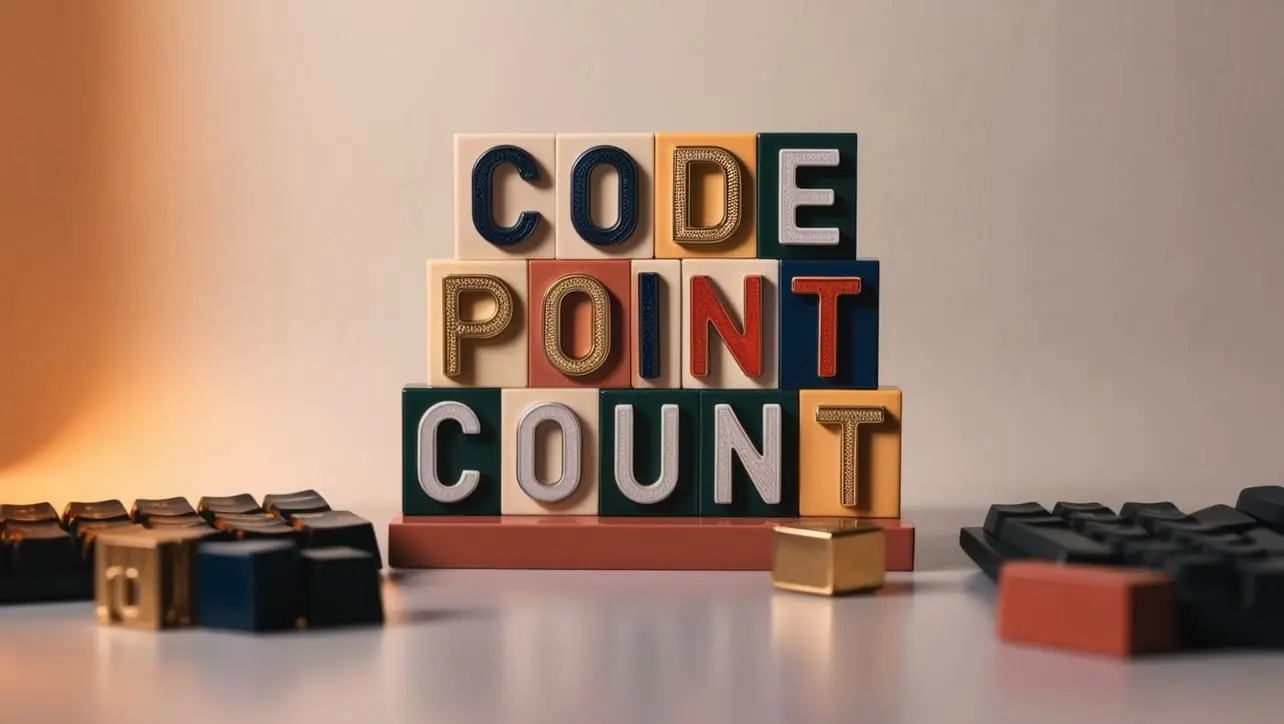
Java string equalsIgnoreCase() Method
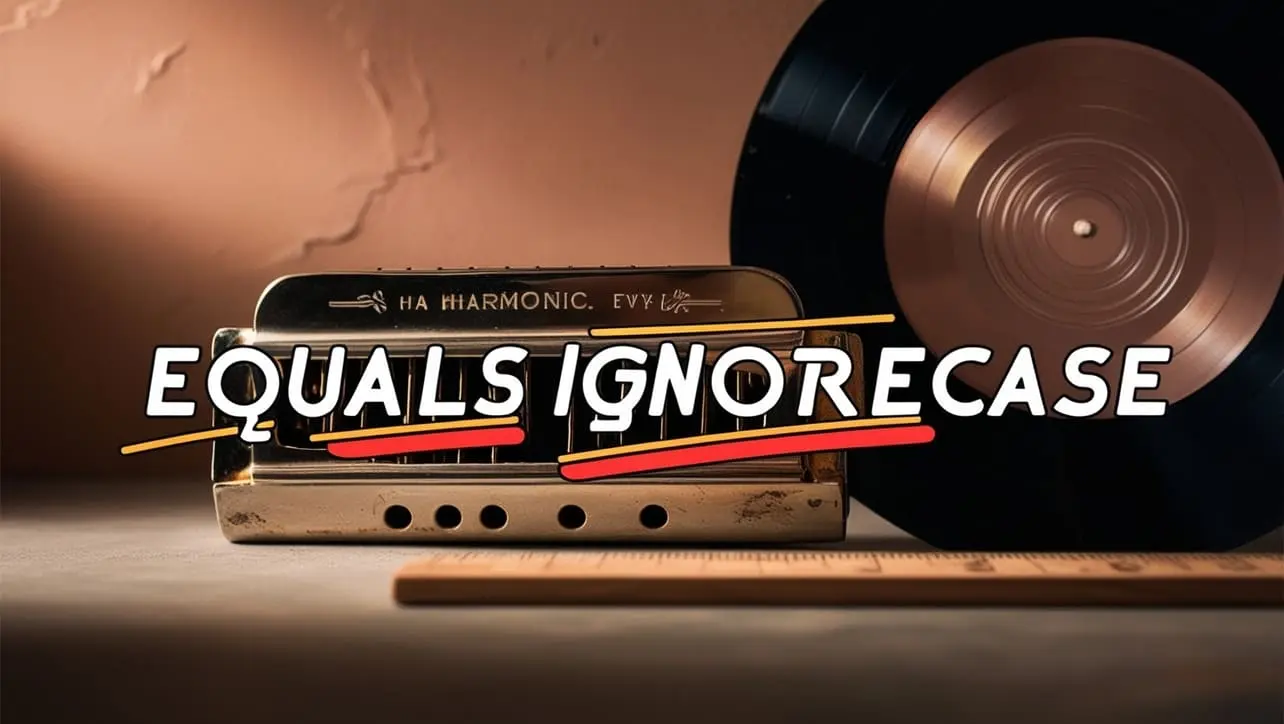
Photo Credit to CodeToFun
đ Introduction
In Java programming, working with strings is a common and fundamental task.
The equalsIgnoreCase()
method is a part of the java.lang.String class and is used to compare two strings for equality, ignoring their case.
In this tutorial, we'll explore the usage and functionality of the equalsIgnoreCase()
method in Java.
đĄ Syntax
The method signature for equalsIgnoreCase()
is as follows:
public boolean equalsIgnoreCase
(String anotherString);
This method takes a single parameter, anotherString, and returns a boolean value indicating whether the strings are equal, disregarding case.
đ Example
Let's dive into an example to illustrate how the equalsIgnoreCase()
method works.
public class StringComparison {
public static void main(String[] args) {
String str1 = "Hello, World!";
String str2 = "HELLO, world!";
// Compare strings ignoring case
boolean result = str1.equalsIgnoreCase(str2);
// Output the result
if (result) {
System.out.println("The strings are equal (ignoring case).");
} else {
System.out.println("The strings are not equal.");
}
}
}
đģ Output
The strings are equal (ignoring case).
đ§ How the Program Works
In this example, the equalsIgnoreCase()
method compares the strings "Hello, World!" and "HELLO, world!" and prints the result, ignoring case.
âŠī¸ Return Value
The equalsIgnoreCase()
method returns true if the two strings are equal, ignoring case. Otherwise, it returns false.
đ Common Use Cases
The equalsIgnoreCase()
method is particularly useful when you need to compare strings in a case-insensitive manner. This is beneficial in scenarios where case differences should not affect the comparison result.
đ Notes
- The
equalsIgnoreCase()
method is more forgiving than the standard equals() method when it comes to case differences.
đĸ Optimization
The equalsIgnoreCase()
method is already optimized for efficient case-insensitive string comparison. No additional optimization is typically needed.
đ Conclusion
The equalsIgnoreCase()
method in Java is a convenient tool for comparing strings without considering case differences. It simplifies tasks such as checking user input, handling configuration values, and other scenarios where case sensitivity is not crucial.
Feel free to experiment with different strings and explore the behavior of the equalsIgnoreCase()
method in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
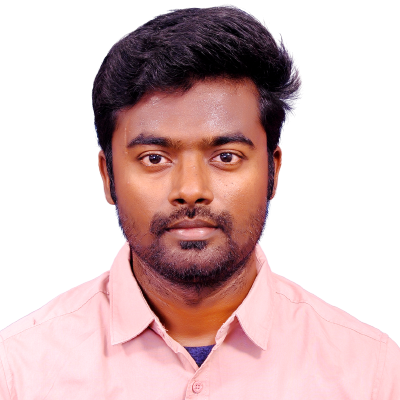
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Java
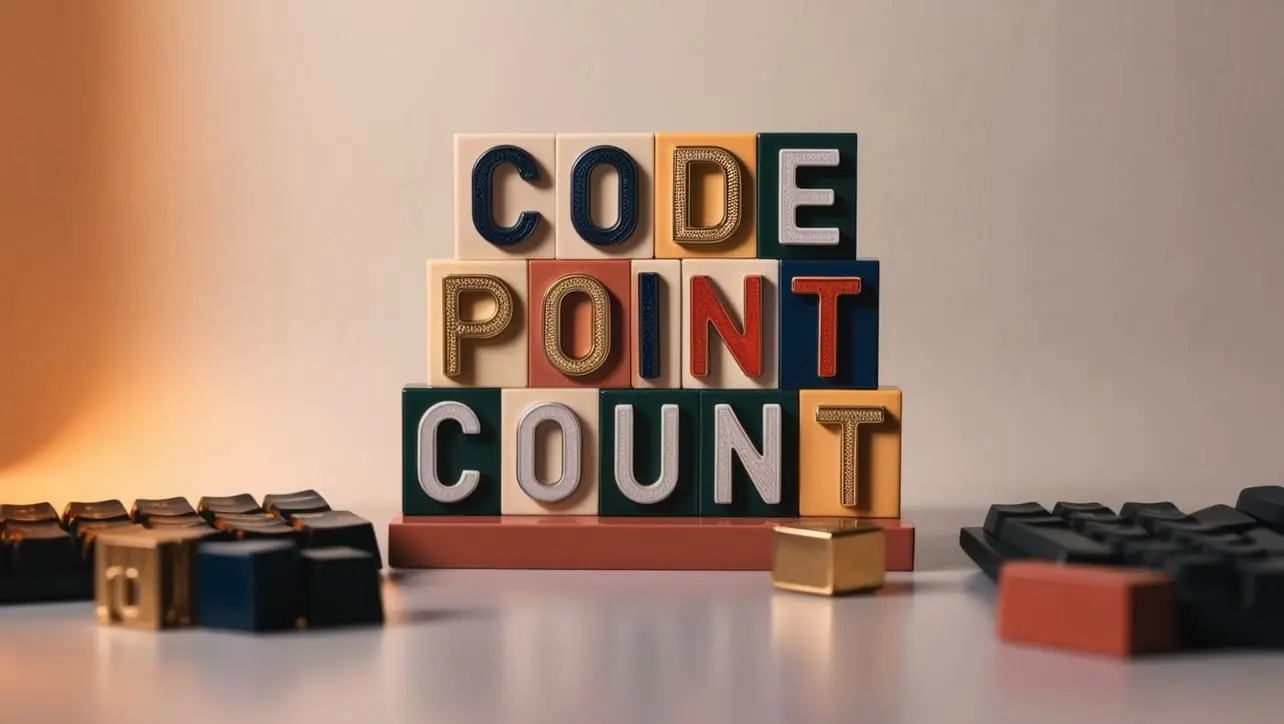
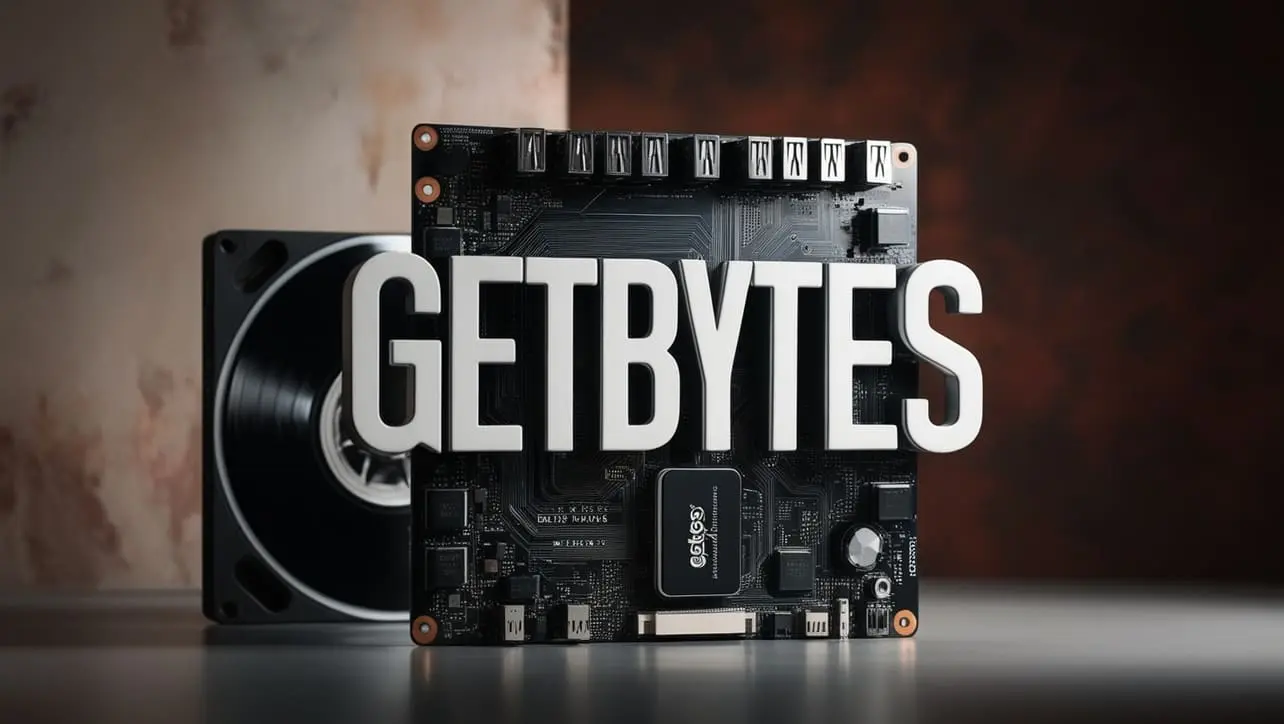
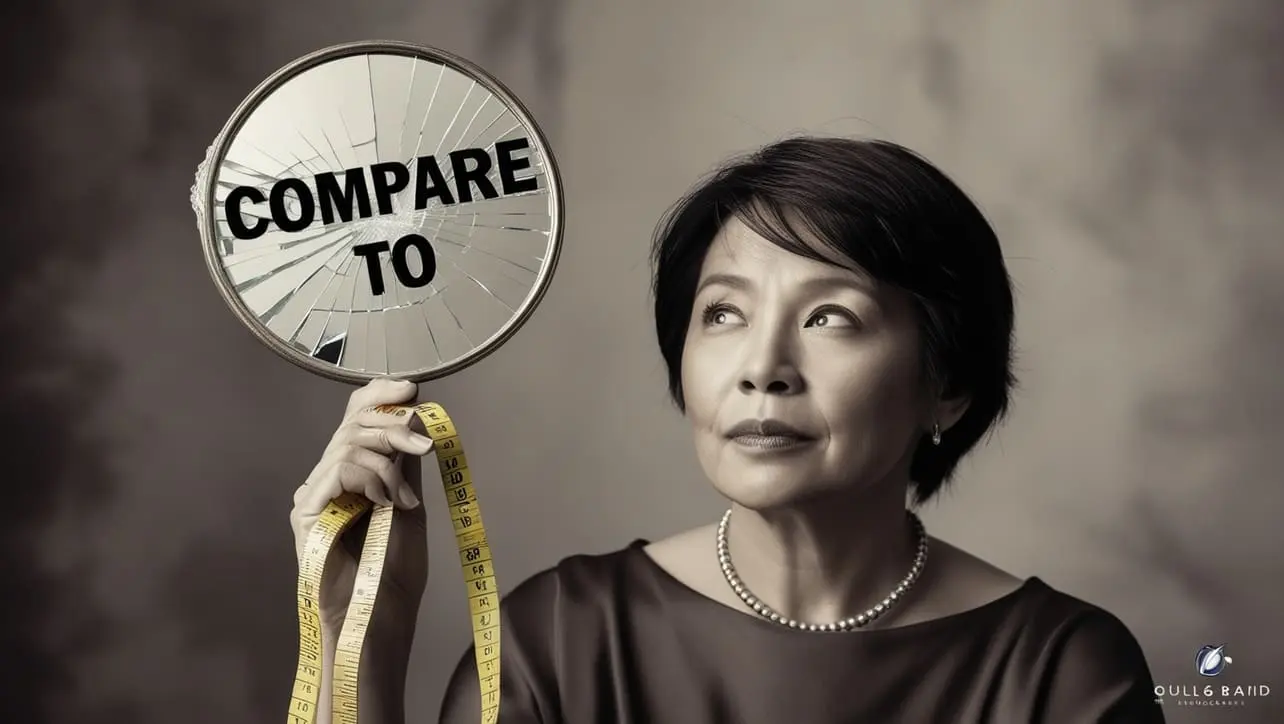
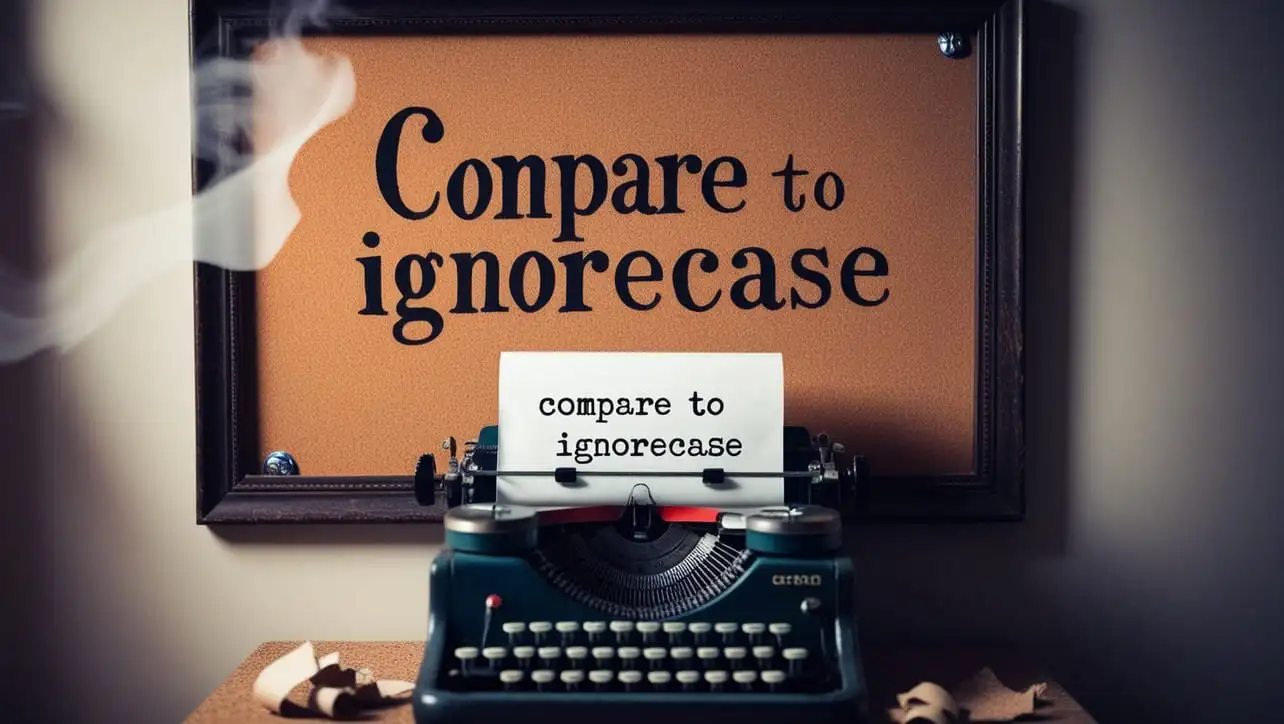
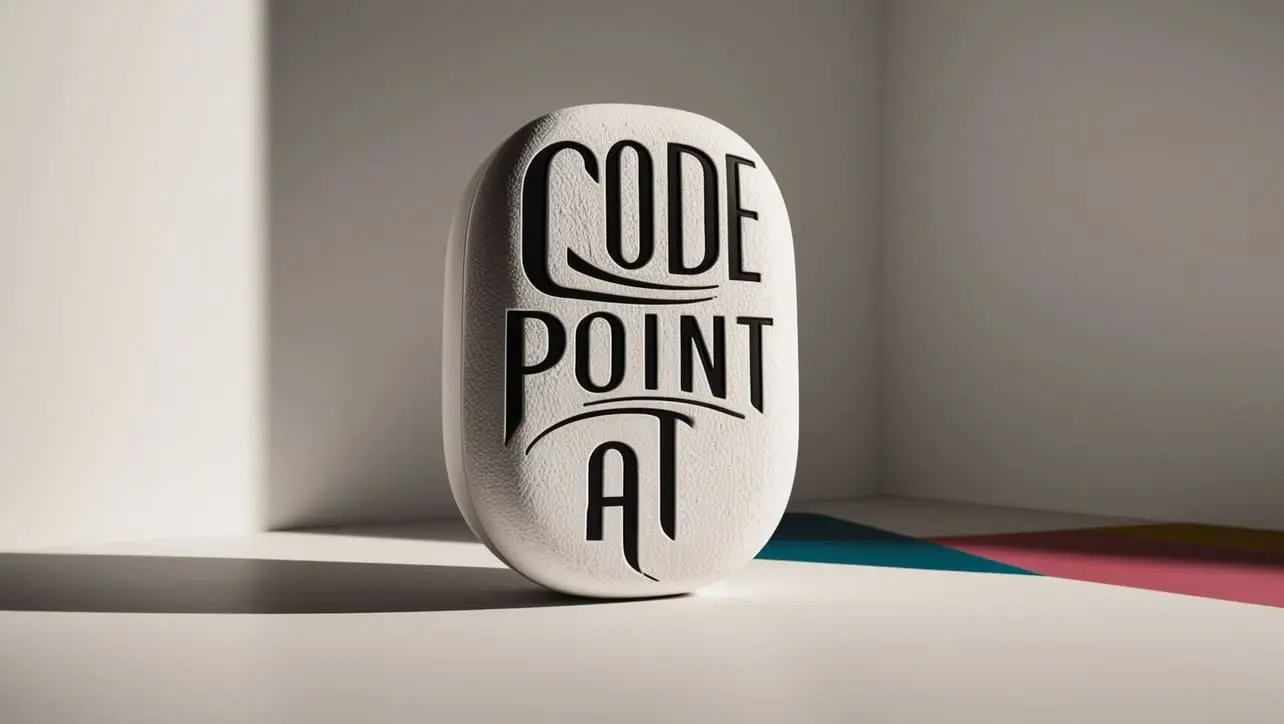
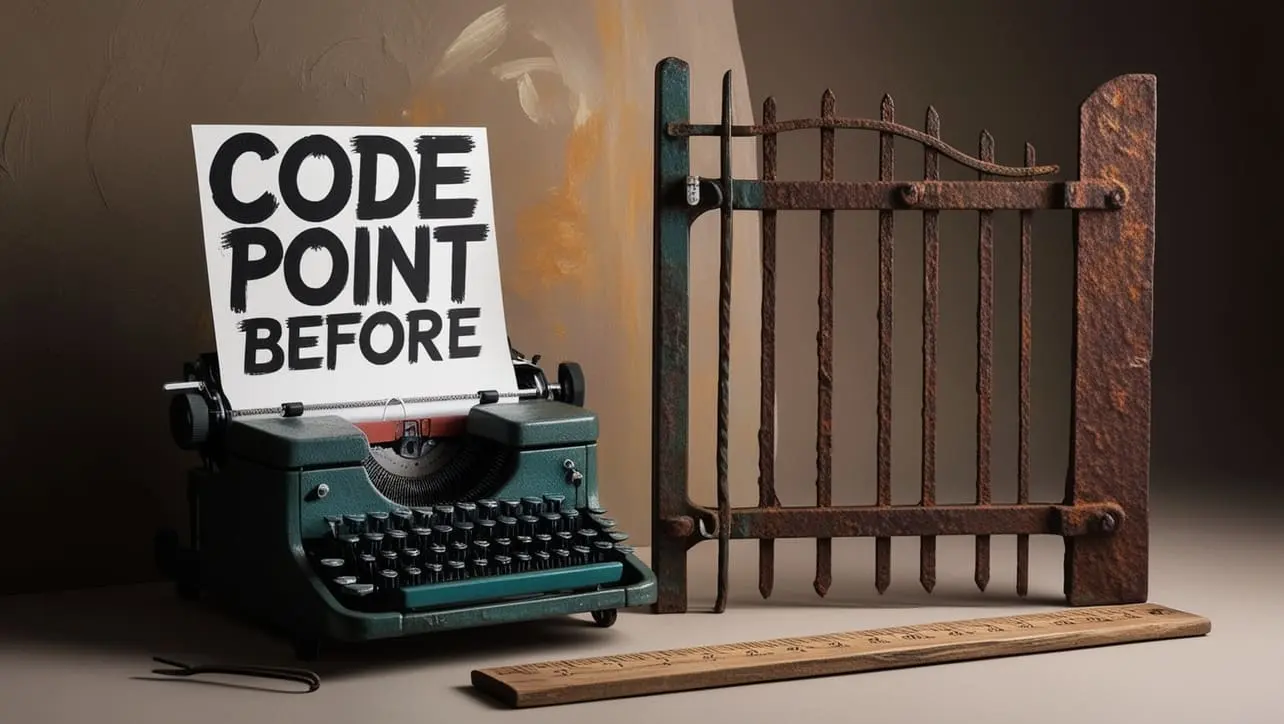
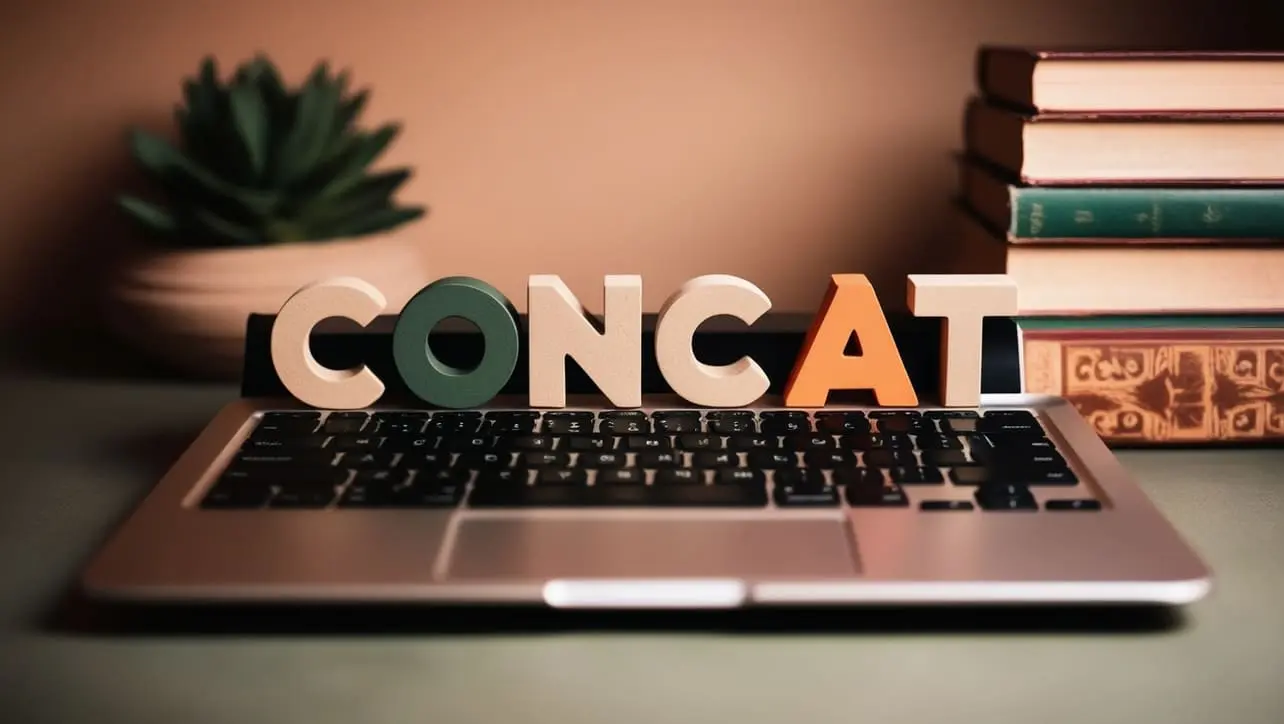
If you have any doubts regarding this article (Java string equalsIgnoreCase() Method), please comment here. I will help you immediately.