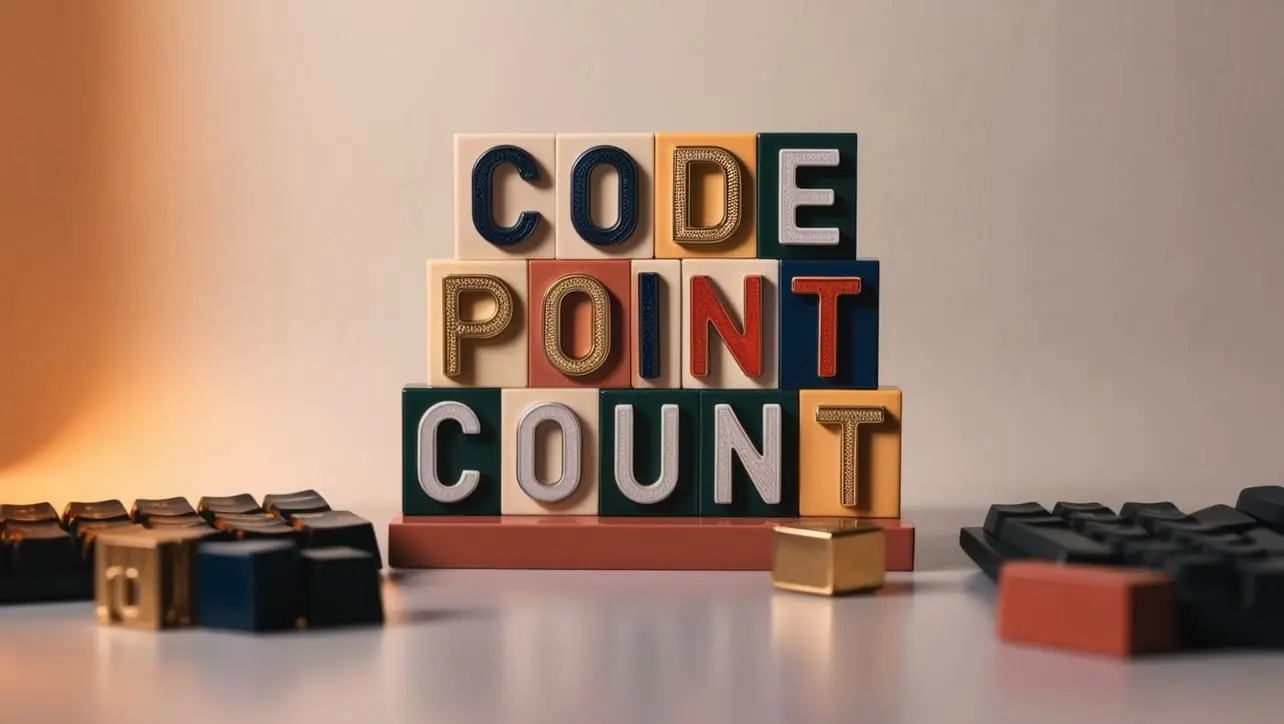
Java string copyValueOf() Method
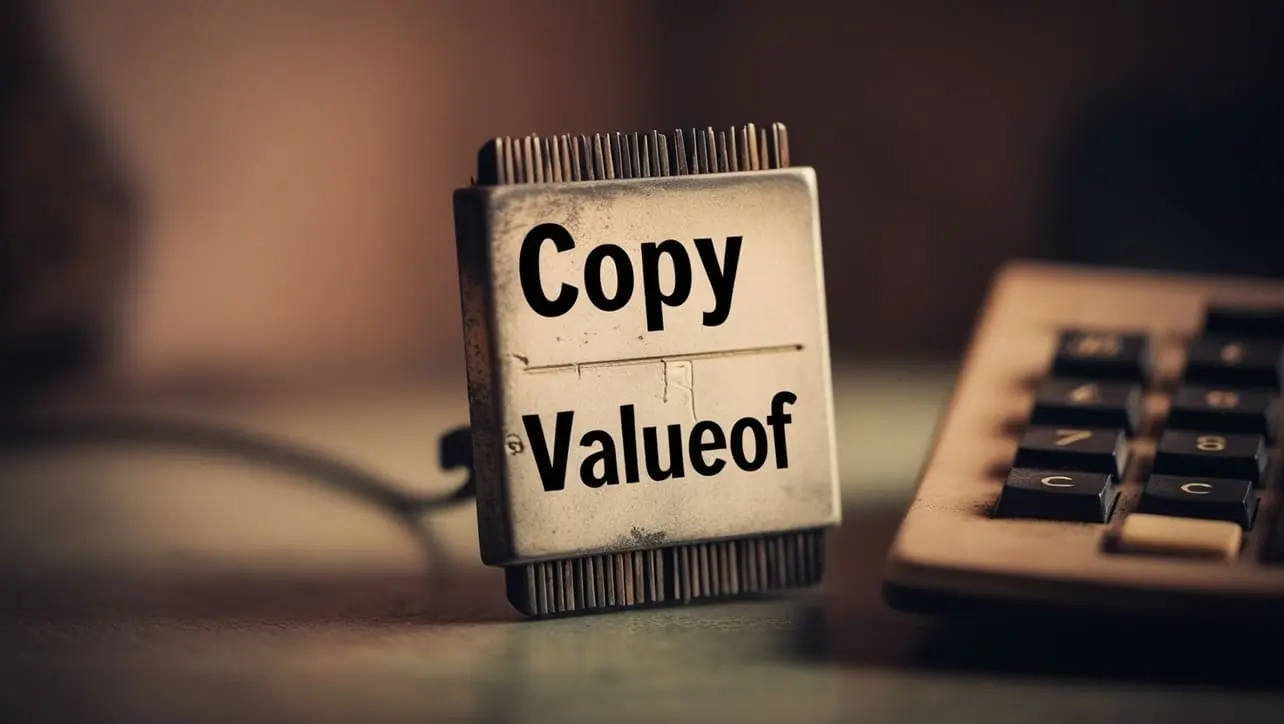
Photo Credit to CodeToFun
đ Introduction
In Java programming, the String class provides various methods for manipulating and extracting information from strings.
The copyValueOf()
method is one such method that allows you to create a new string by copying the characters of an array or a portion of an array.
In this tutorial, we'll explore the usage and functionality of the copyValueOf()
method in Java.
đĄ Syntax
The signature of the copyValueOf()
method is as follows:
public static String copyValueOf(char[] data)
public static String copyValueOf(char[] data, int offset, int count)
- The first variant takes an array of characters data and creates a new string using all the characters in the array.
- The second variant takes three parameters: data, offset, and count. It creates a string using count characters from the data array, starting at the specified offset.
đ Example
Let's delve into examples to illustrate how the copyValueOf()
method works.
public class CopyValueOfExample {
public static void main(String[] args) {
char[] charArray = {'H','e','l','l','o'};
// Using the first variant
String result1 = String.copyValueOf(charArray);
System.out.println("Example 1: " + result1); // Output: Hello
// Using the second variant
String result2 = String.copyValueOf(charArray, 1, 3);
System.out.println("Example 2: " + result2); // Output: ell
}
}
đģ Output
Example 1: Hello Example 2: ell
đ§ How the Program Works
In Example 1, the copyValueOf()
method is used to create a new string from the entire charArray.
In Example 2, it creates a string using a subset of the array starting from index 1 and taking 3 characters.
âŠī¸ Return Value
The copyValueOf()
method returns a new String object that represents the characters copied from the specified array.
đ Common Use Cases
The copyValueOf()
method is useful when you have a character array, and you want to create a string from its contents. This is particularly handy when dealing with character data obtained from sources like files or network streams.
đ Notes
- Keep in mind that the
copyValueOf()
method is static and is called on the String class itself. - If you have a char[] array and you want to create a string using the entire array, you can also use the String(char[] value) constructor.
đĸ Optimization
The copyValueOf()
method is optimized for creating strings from character arrays. There's typically no need for additional optimization.
đ Conclusion
The copyValueOf()
method in Java provides a convenient way to create strings from character arrays. Whether you need to construct a string from the entire array or a subset, this method offers flexibility and simplicity.
Feel free to experiment with different character arrays and explore the behavior of the copyValueOf()
method in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
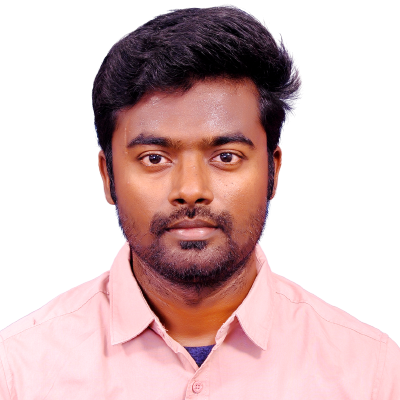
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Java
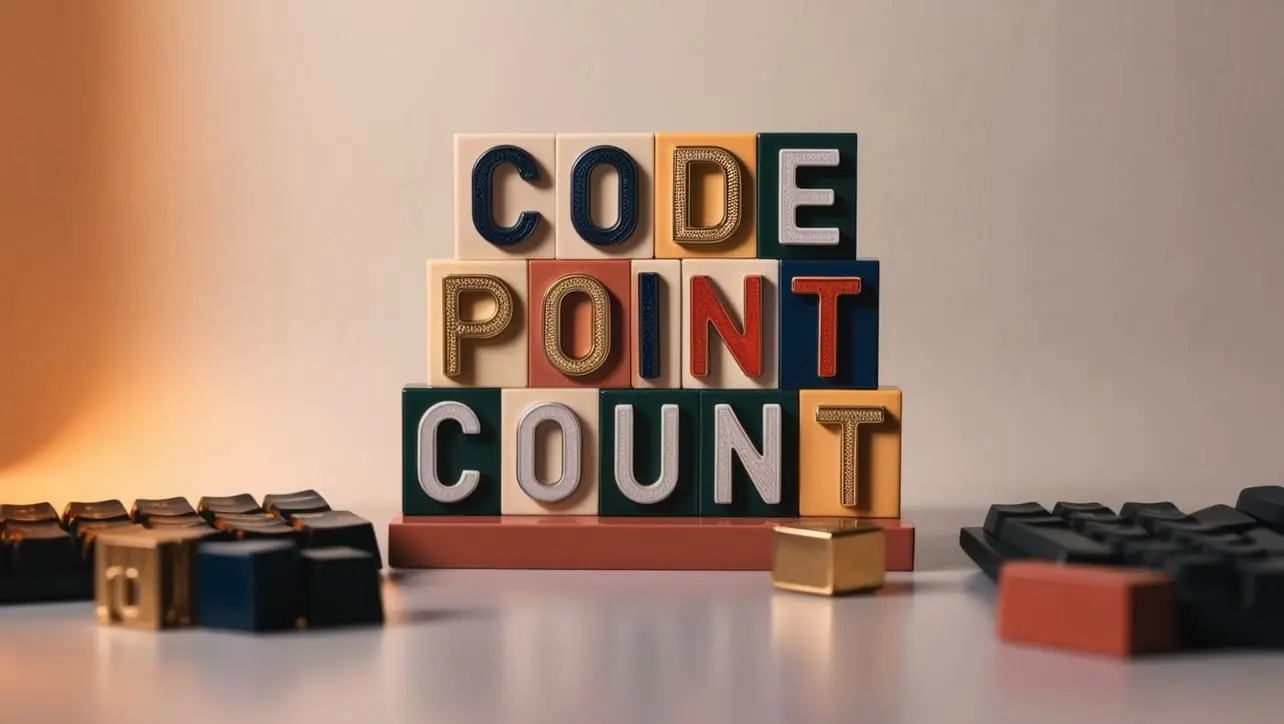
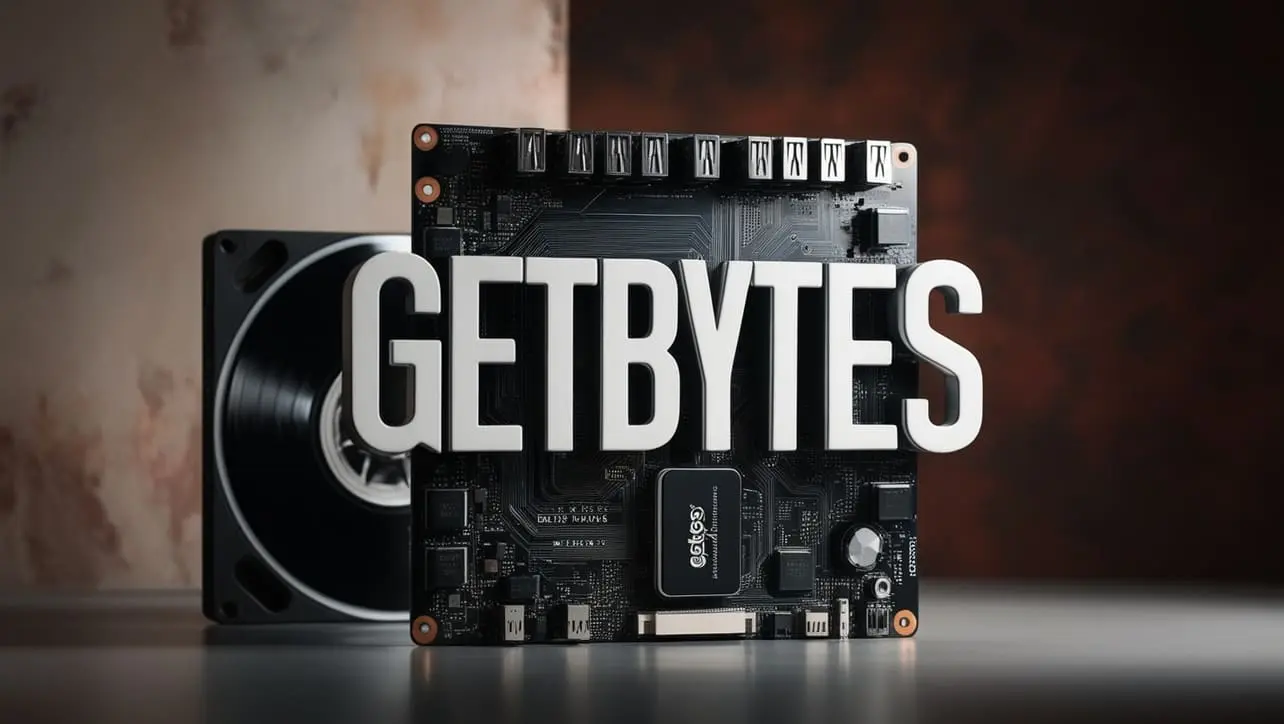
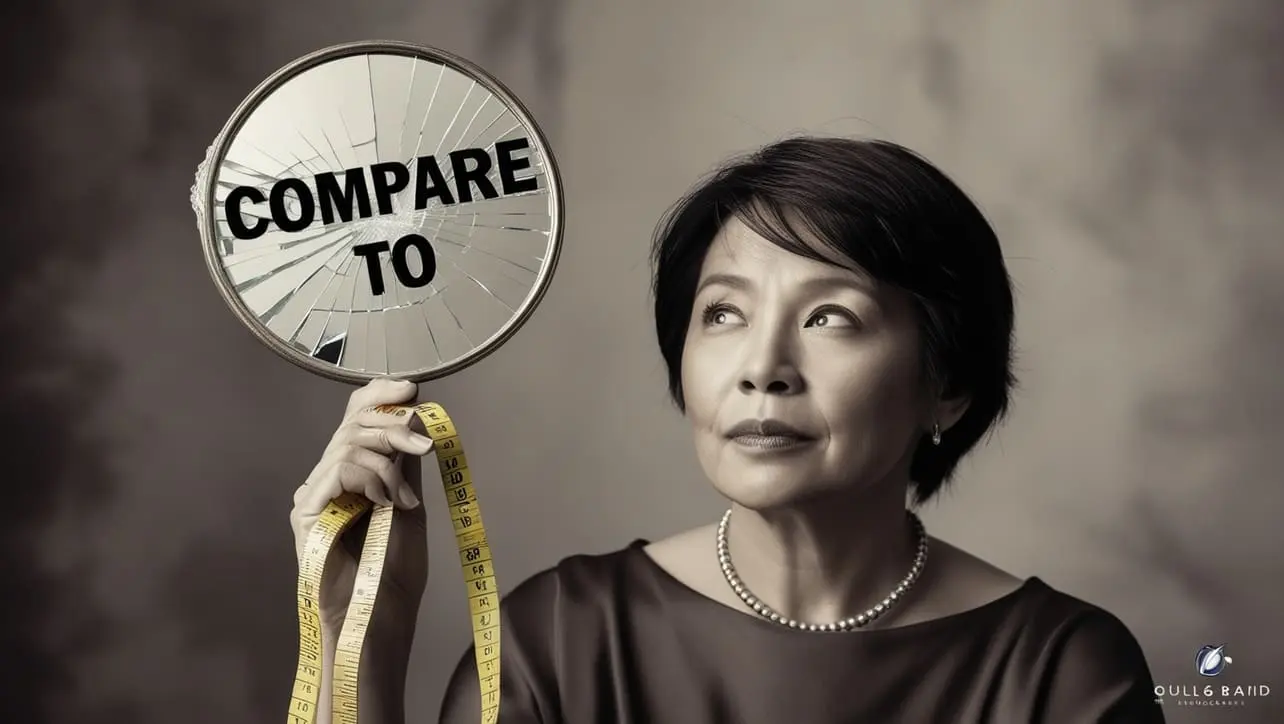
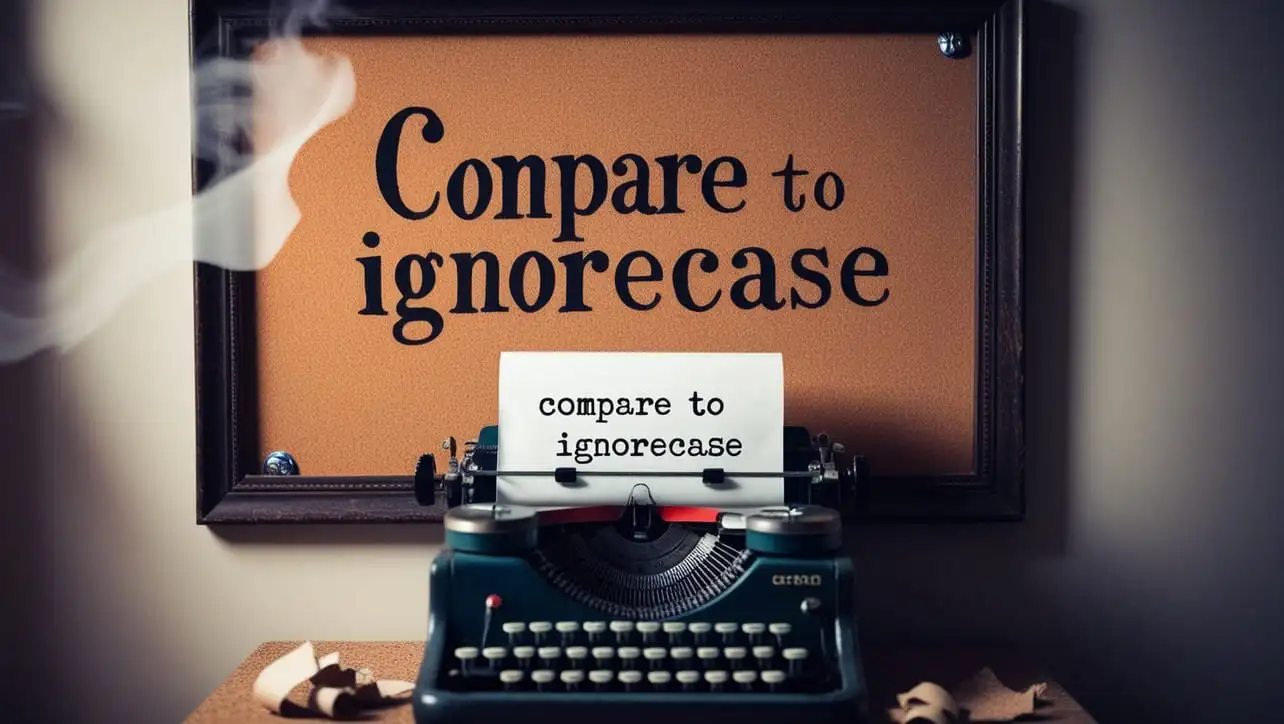
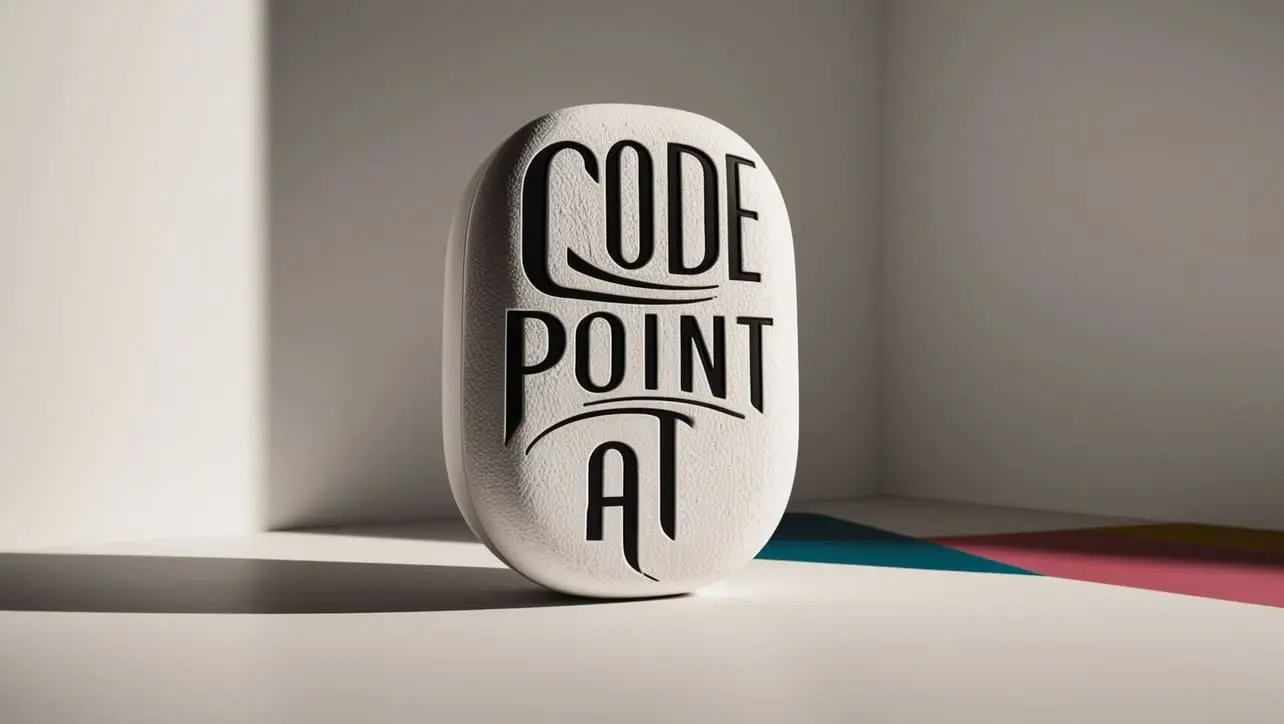
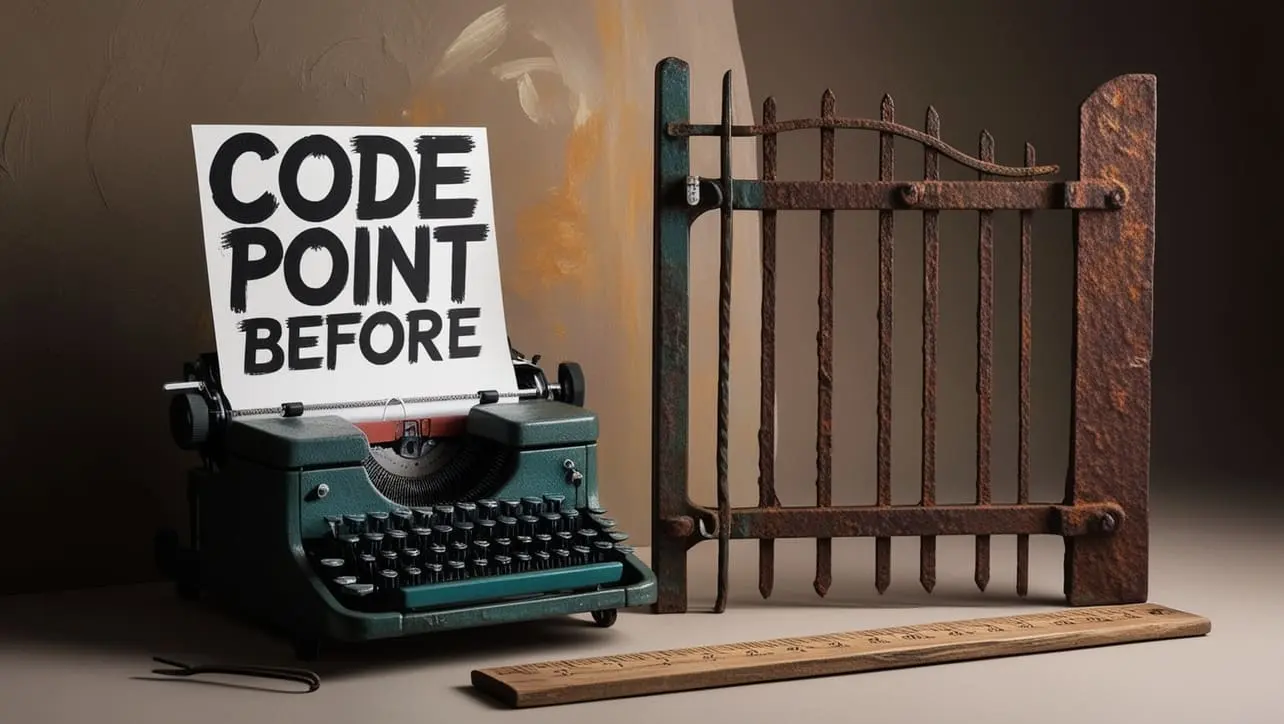
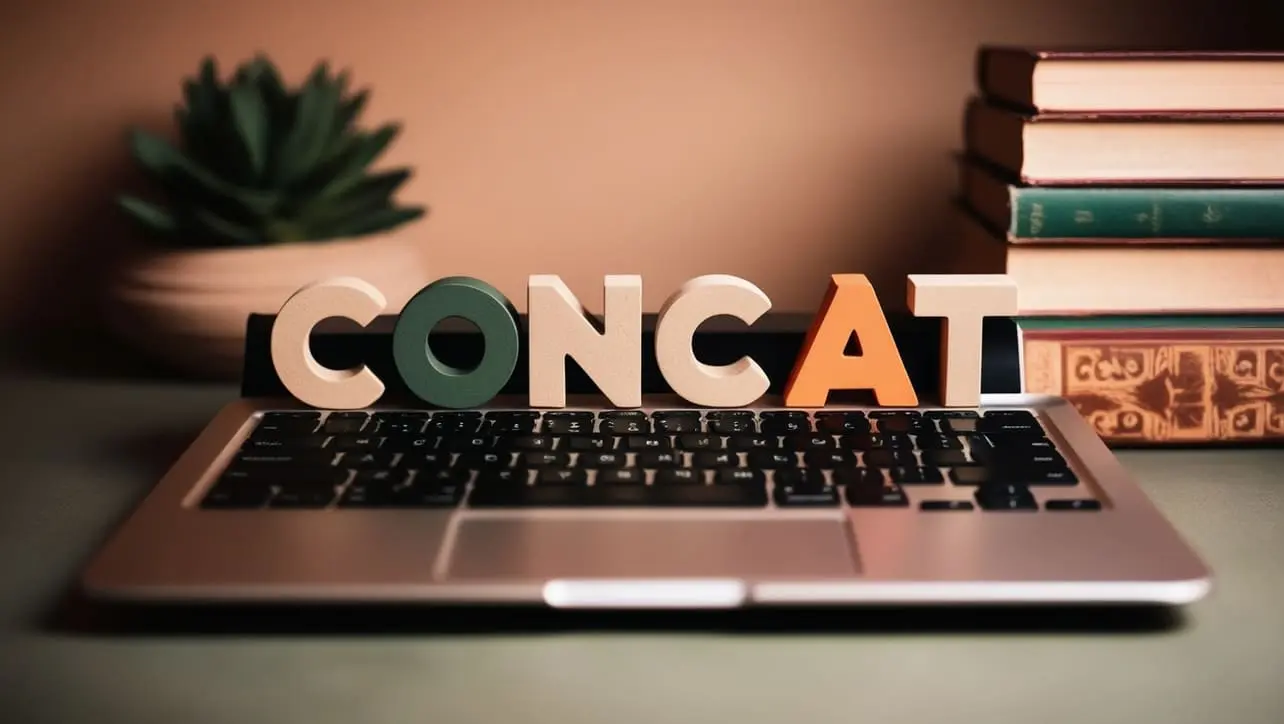
If you have any doubts regarding this article (Java string copyValueOf() Method), please comment here. I will help you immediately.