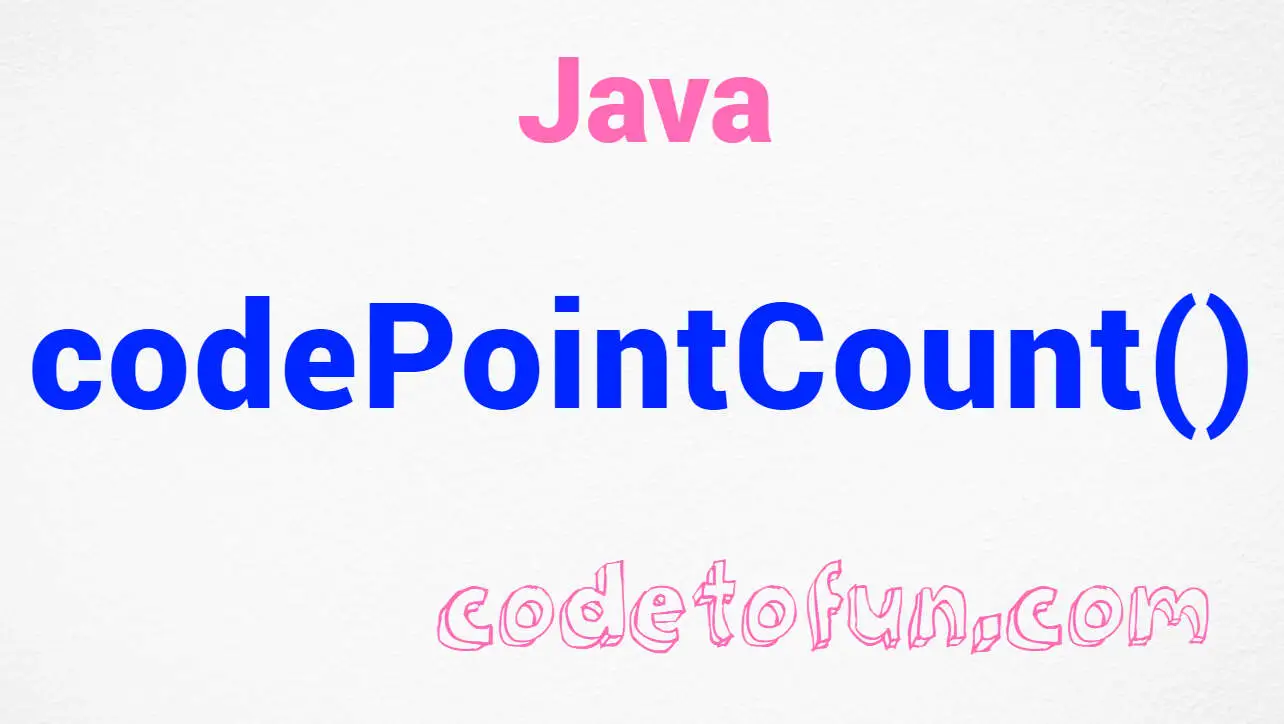
Java String Methods
Java string codePointBefore() Method
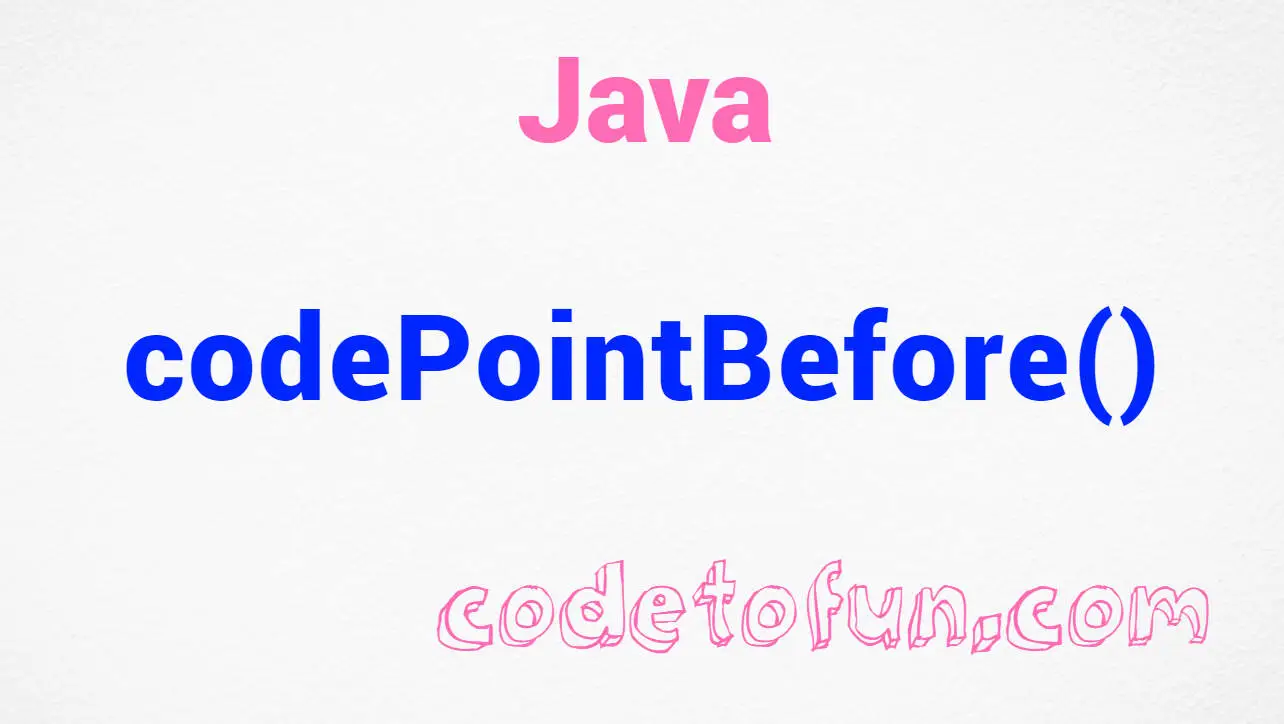
Photo Credit to CodeToFun
đ Introduction
In Java programming, strings play a crucial role, and various methods are available for string manipulation.
The codePointBefore()
method is one such method provided by the java.lang.String class.
It returns the Unicode code point before the specified index in a string.
In this tutorial, we'll explore the usage and functionality of the codePointBefore()
method in Java.
đĄ Syntax
The syntax for the codePointBefore()
method is as follows:
int codePointBefore(int index)
- index: The index to get the code point before.
đ Example
Let's delve into an example to illustrate how the codePointBefore()
method works.
public class CodePointBeforeExample {
public static void main(String[] args) {
String text = "Java\uD83D\uDE00"; // "Java" followed by a smiling face emoji
// Get the code point before index 4
int codePoint = text.codePointBefore(4);
// Output the result
System.out.println("Code point before index 4: " + codePoint); // Output: 86 (Unicode for 'J')
}
}
đģ Output
Code point before index 4: 97
đ§ How the Program Works
In this example, the codePointBefore()
method is used to obtain the Unicode code point before index 4 in the string "Java\uD83D\uDE00", which represents "Java" followed by a smiling face emoji.
âŠī¸ Return Value
The codePointBefore()
method returns the Unicode code point (as an integer) before the specified index in the string.
đ Common Use Cases
The codePointBefore()
method is useful when you need to work with Unicode characters and manipulate strings at the code point level. It allows you to access and analyze characters in a more granular way, especially in the presence of supplementary characters.
đ Notes
- The index parameter should be greater than 0 and less than the length of the string to avoid IndexOutOfBoundsException.
- The method considers the supplementary characters in the Unicode standard, which means it correctly handles characters outside the Basic Multilingual Plane (BMP).
đĸ Optimization
The codePointBefore()
method is optimized for efficiently retrieving the code point before a specified index. No specific optimizations are typically required.
đ Conclusion
The codePointBefore()
method in Java is a valuable tool for working with Unicode characters in strings. It provides a way to navigate and manipulate strings at the code point level, enabling more sophisticated string handling in internationalization and multilingual applications.
Feel free to experiment with different strings and explore the behavior of the codePointBefore()
method in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
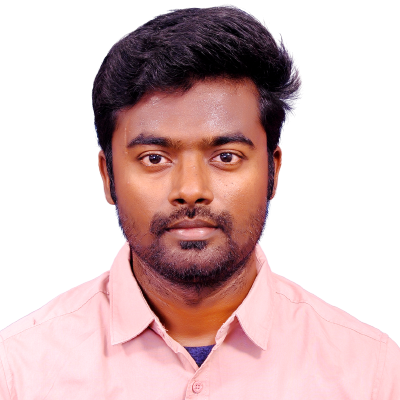
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java string codePointBefore() Method), please comment here. I will help you immediately.