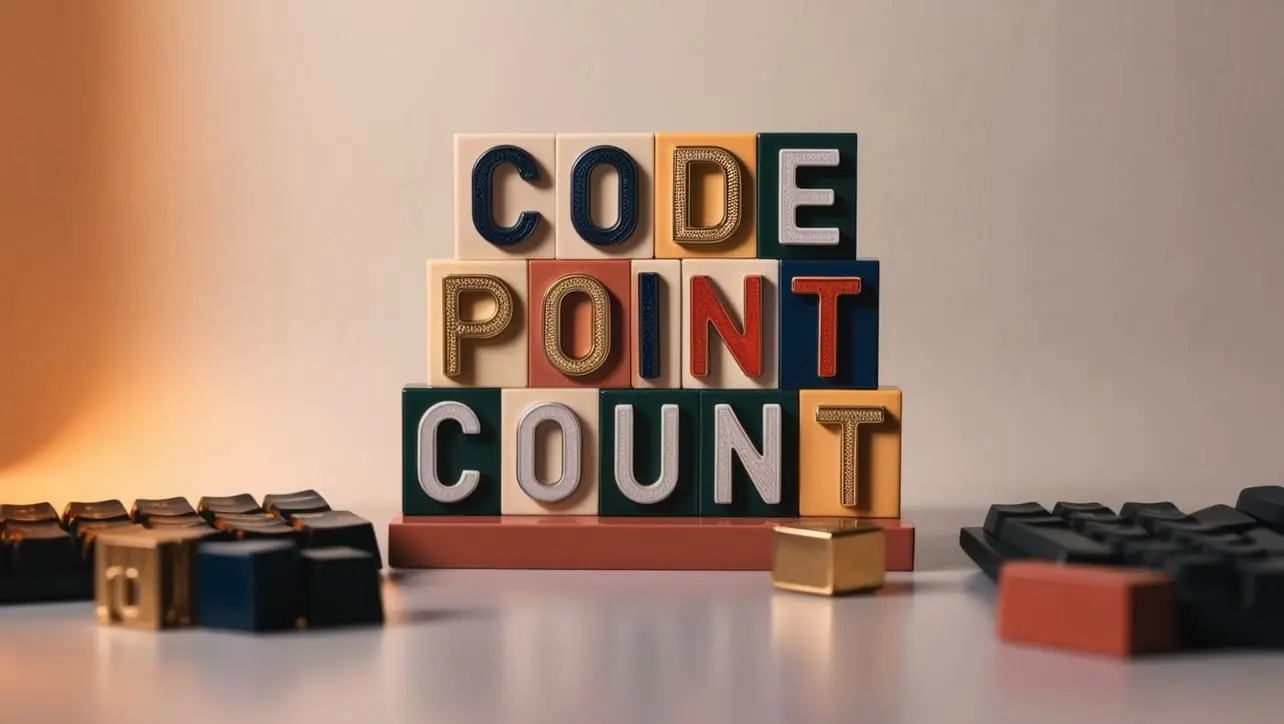
Java Topics
- Java Intro
- Java String Methods
- Java Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Java Star Pattern
- Java Number Pattern
- Java Alphabet Pattern
Java Program to Check Power of 3
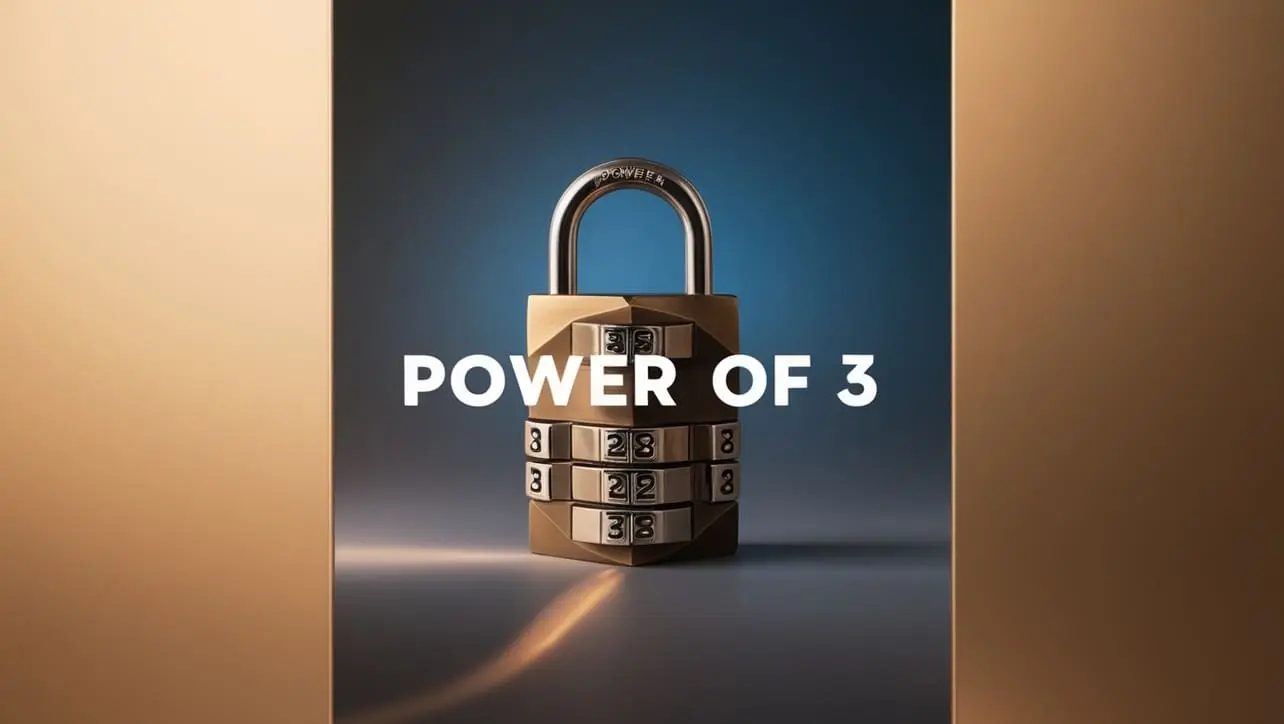
Photo Credit to CodeToFun
Introduction
In the realm of programming, one often encounters the need to check if a given number is a power of another.
In this context, we will explore a Java program designed specifically to check if a number is a power of 3.
The logic behind this program involves repeatedly dividing the number by 3 until it becomes 1, indicating that it is a power of 3.
Example
Let's delve into the Java code that achieves this functionality.
public class CheckPowerOf3 {
// Function to check if a number is a power of 3
static boolean isPowerOf3(int n) {
// Keep dividing the number by 3 until it is greater than 1
while (n % 3 == 0 && n > 1) {
n /= 3;
}
// If the final value is 1, the original number is a power of 3
return n == 1;
}
// Driver program
public static void main(String[] args) {
// Replace this value with your desired number
int number = 27;
// Check if the number is a power of 3
if (isPowerOf3(number)) {
System.out.println(number + " is a power of 3");
} else {
System.out.println(number + " is not a power of 3");
}
}
}
Testing the Program
To test the program with different numbers, simply replace the value of number in the main method.
27 is a power of 3
Compile and run the program to see if the given number is a power of 3.
How the Program Works
- The program defines a class CheckPowerOf3 containing a static method isPowerOf3 that takes an integer as input and checks if it is a power of 3.
- Inside the method, it repeatedly divides the number by 3 until it becomes greater than 1.
- If the final value is 1, the original number is a power of 3, and the method returns true; otherwise, it returns false.
- The driver program in the main method tests a specific number and prints whether it is a power of 3 or not.
Between the Given Range
Let's take a look at the java code that checks for powers of 3 in the given range.
public class PowerOfThreeChecker {
// Function to check if a number is a power of 3
static boolean isPowerOfThree(int num) {
// If num is less than or equal to 0, it can't be a power of 3
if (num <= 0) {
return false;
}
// Keep dividing num by 3 until it becomes 1
while (num % 3 == 0) {
num /= 3;
}
// If num becomes 1, it is a power of 3; otherwise, it's not
return num == 1;
}
// Driver program
public static void main(String[] args) {
System.out.println("Power of 3 in the range 1 to 20:");
// Check and print powers of 3 in the specified range
for (int i = 1; i <= 20; i++) {
if (isPowerOfThree(i)) {
System.out.print(i + " ");
}
}
}
}
Testing the Program
Power of 3 in the range 1 to 20: 1 3 9
To test the program, compile and run it. The output should display the powers of 3 in the specified range.
How the Program Works
- The program defines a class PowerOfThreeChecker containing a static method isPowerOfThree that checks if a given number is a power of 3.
- Inside the method, it ensures that the number is greater than 0 and then repeatedly divides it by 3 until it becomes 1.
- The main method prints the powers of 3 in the range from 1 to 20.
Understanding the Concept of Power of 3
Before diving into the code, let's understand the concept of a power of 3.
A number is considered a power of 3 if it can be expressed as 3^n, where n is an integer.
For example, 1, 3, 9, 27, and so on are powers of 3.
Optimizing the Program
While the provided program is straightforward, there are alternative approaches to check if a number is a power of 3. Consider exploring other mathematical techniques for optimization.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
Join our Community:
Author
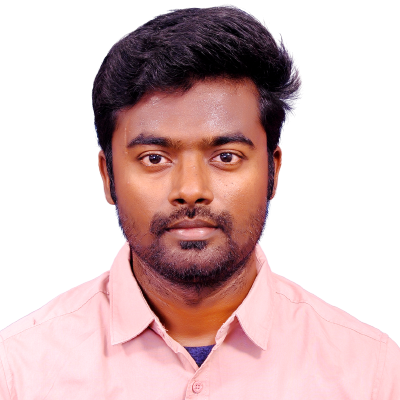
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Java
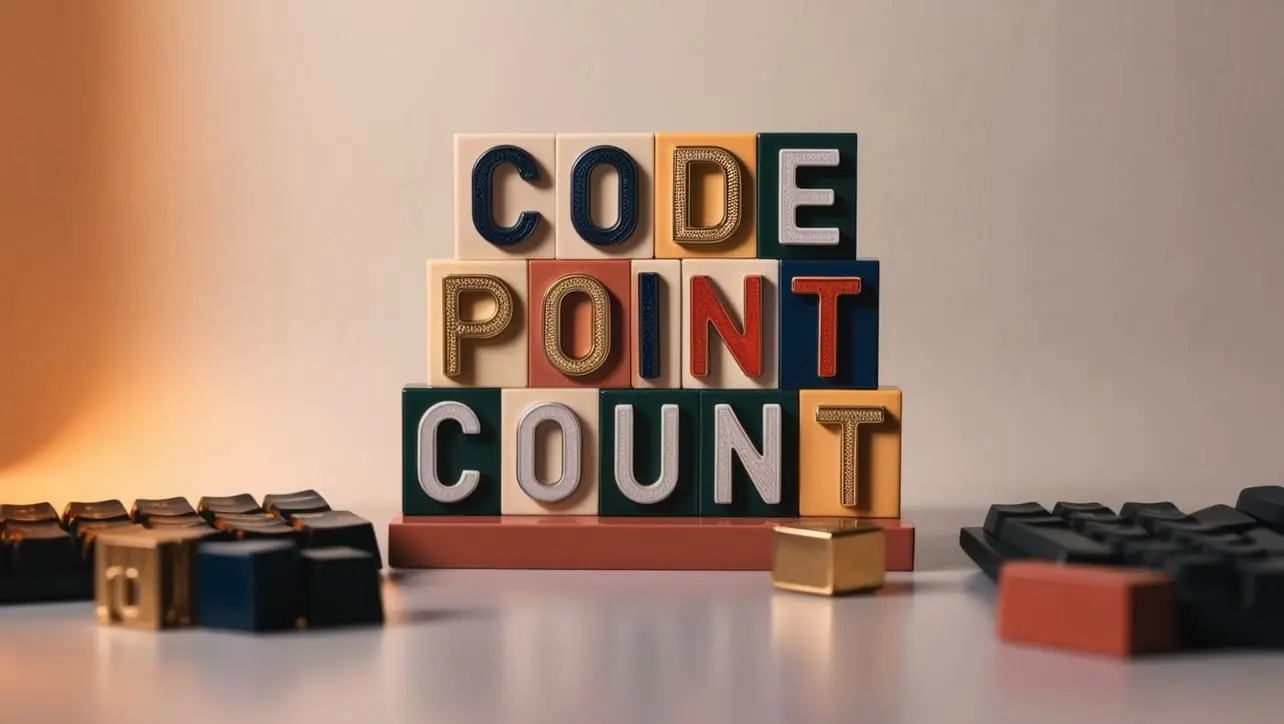
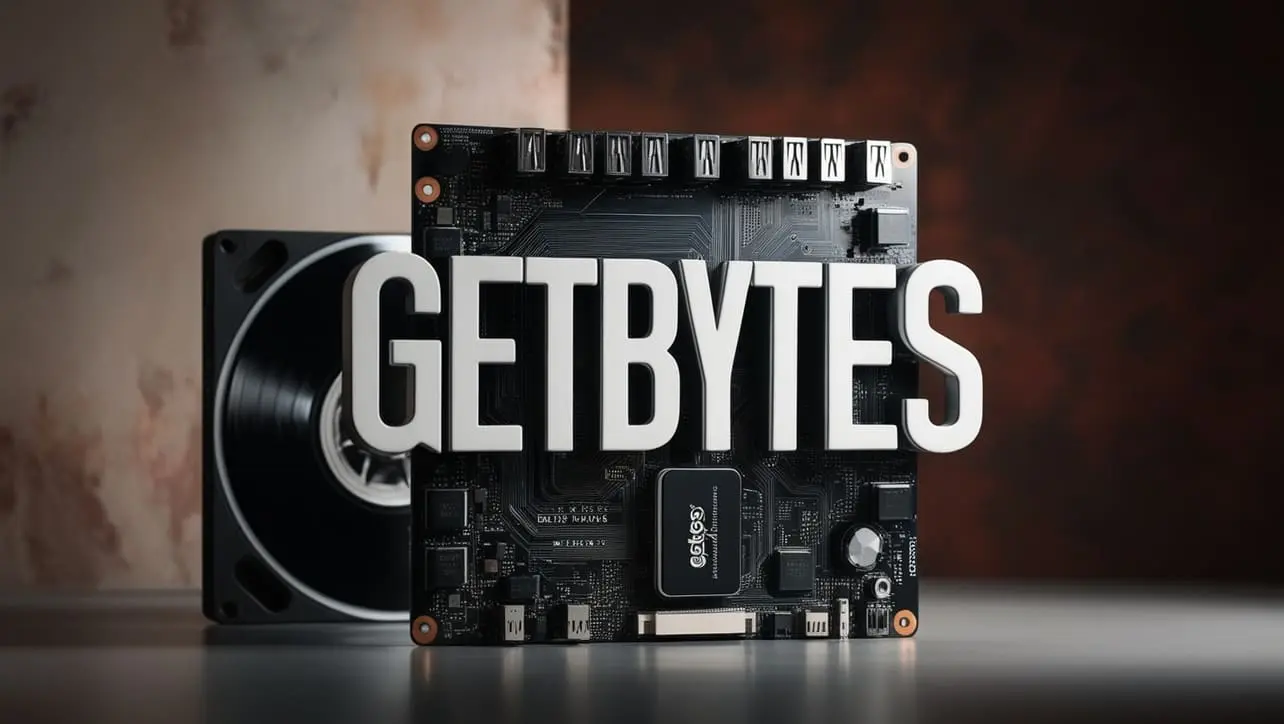
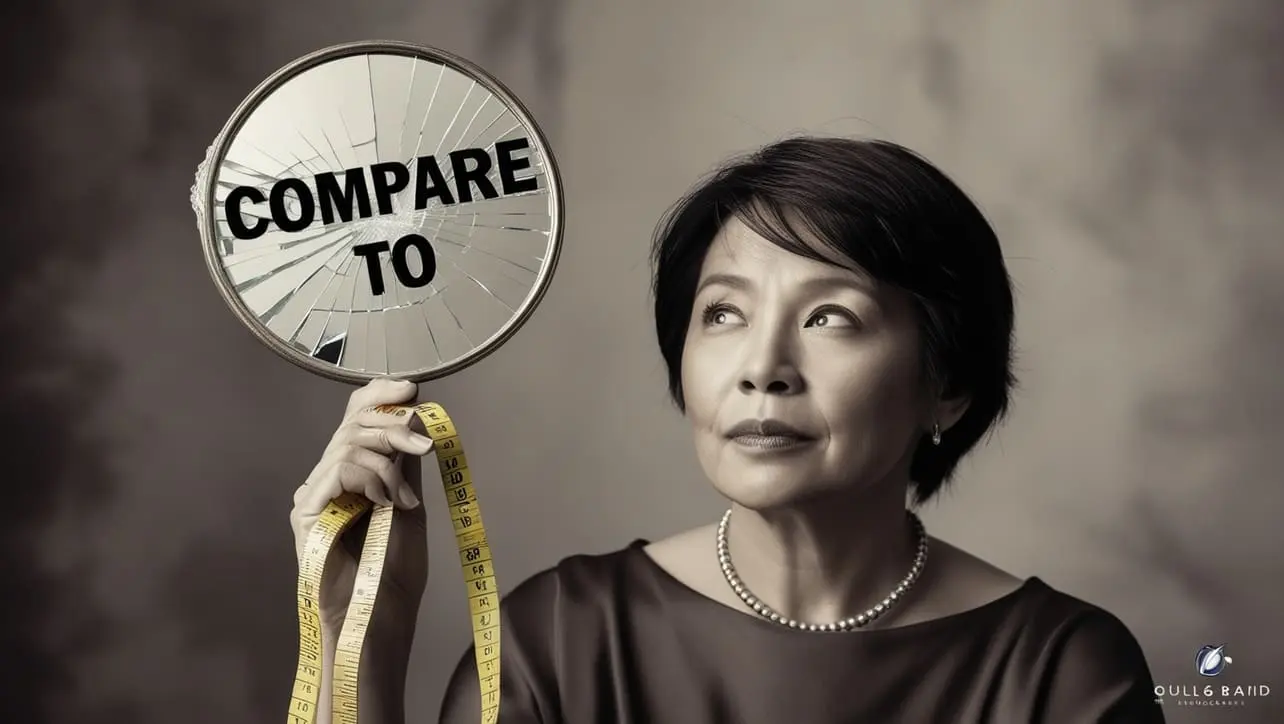
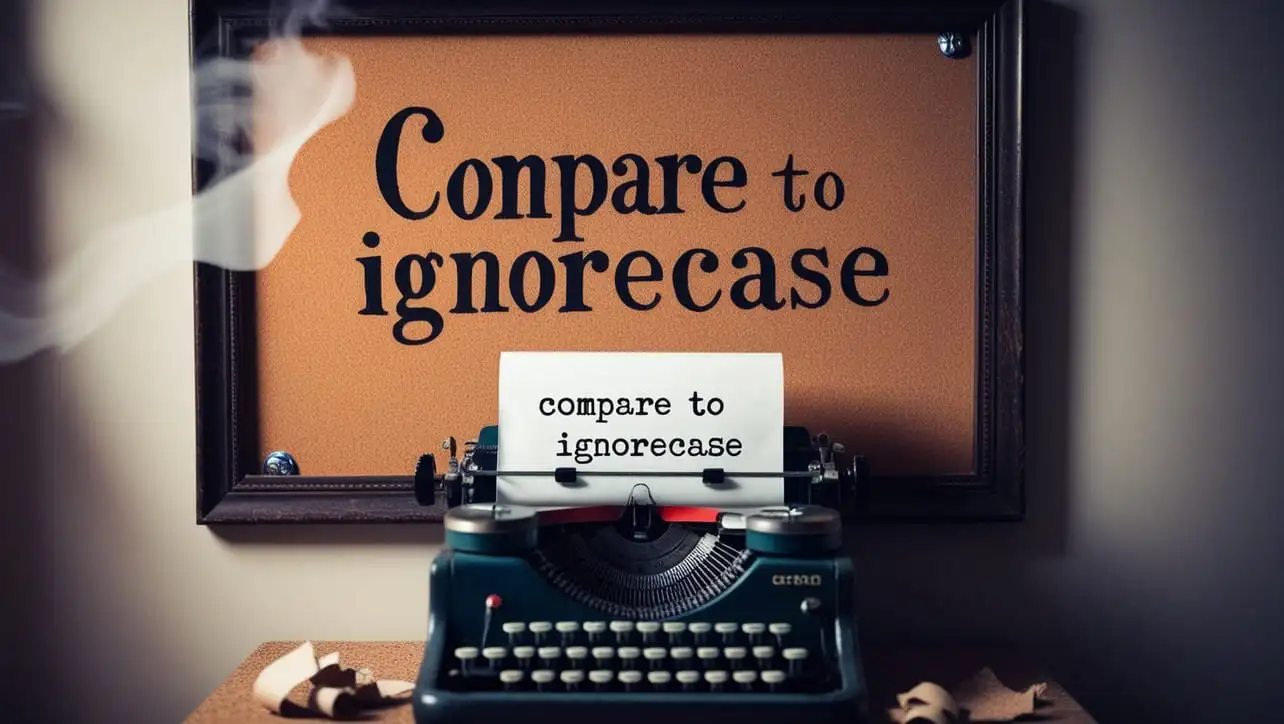
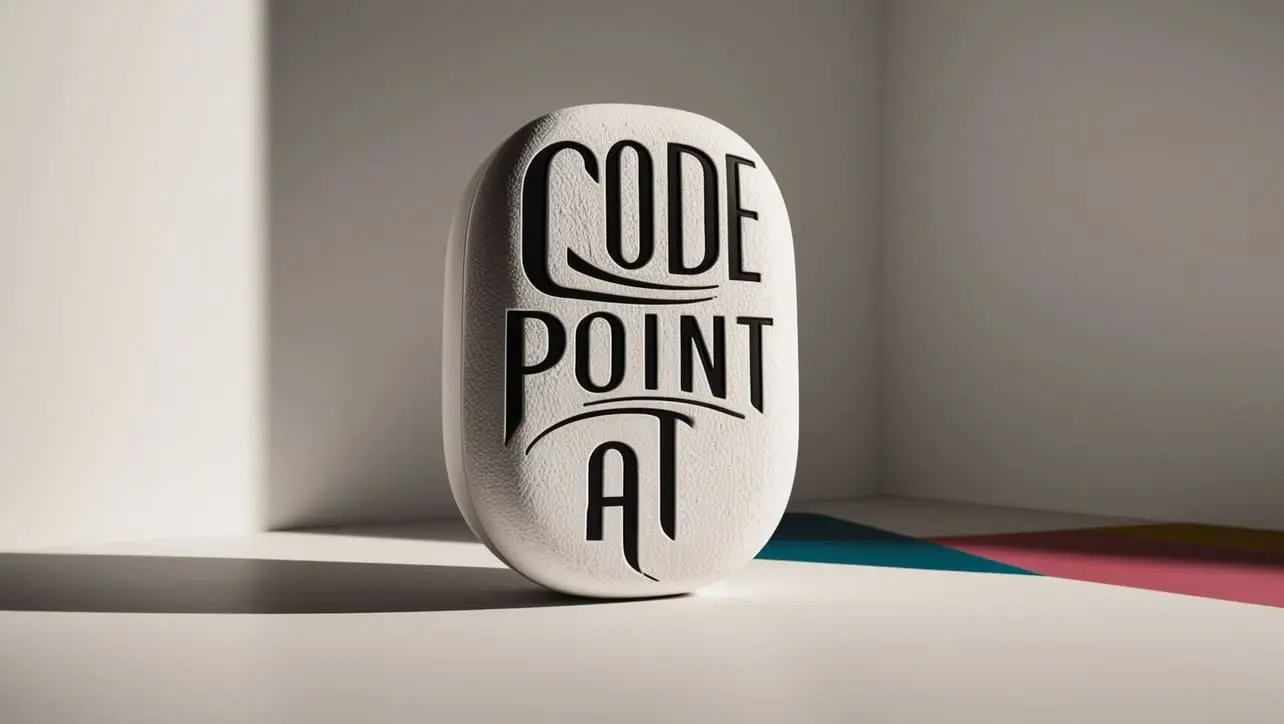
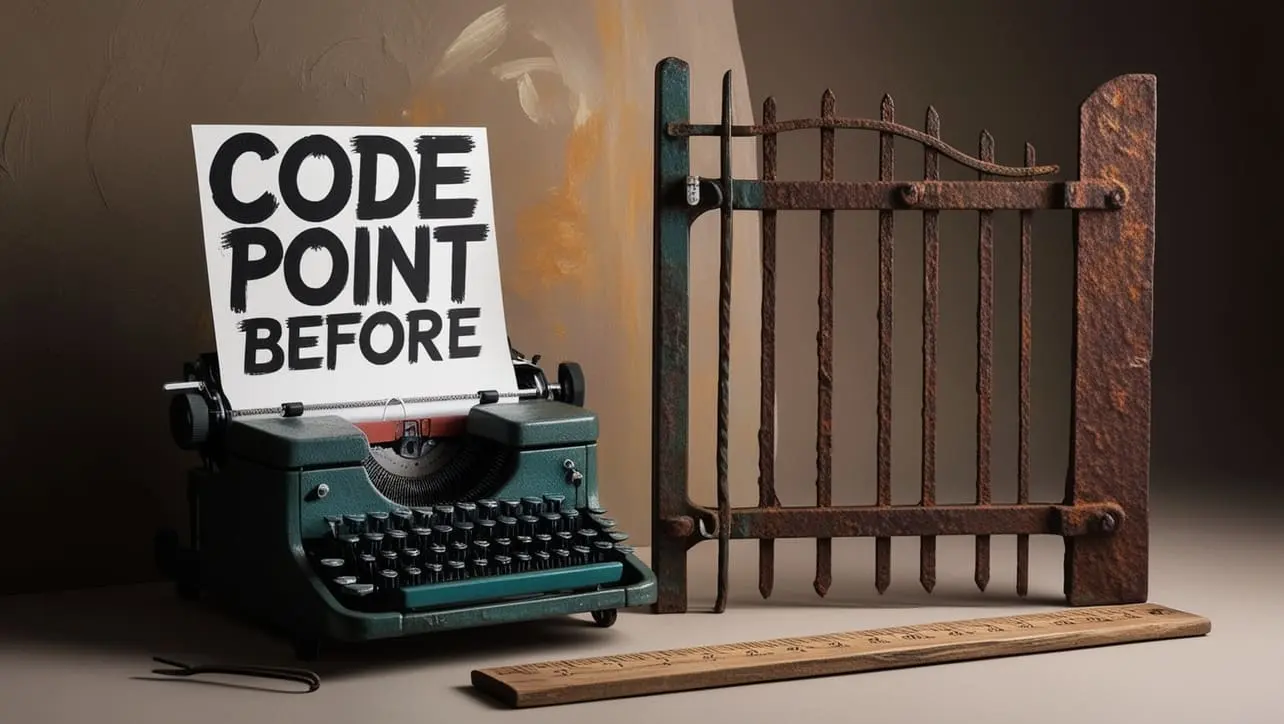
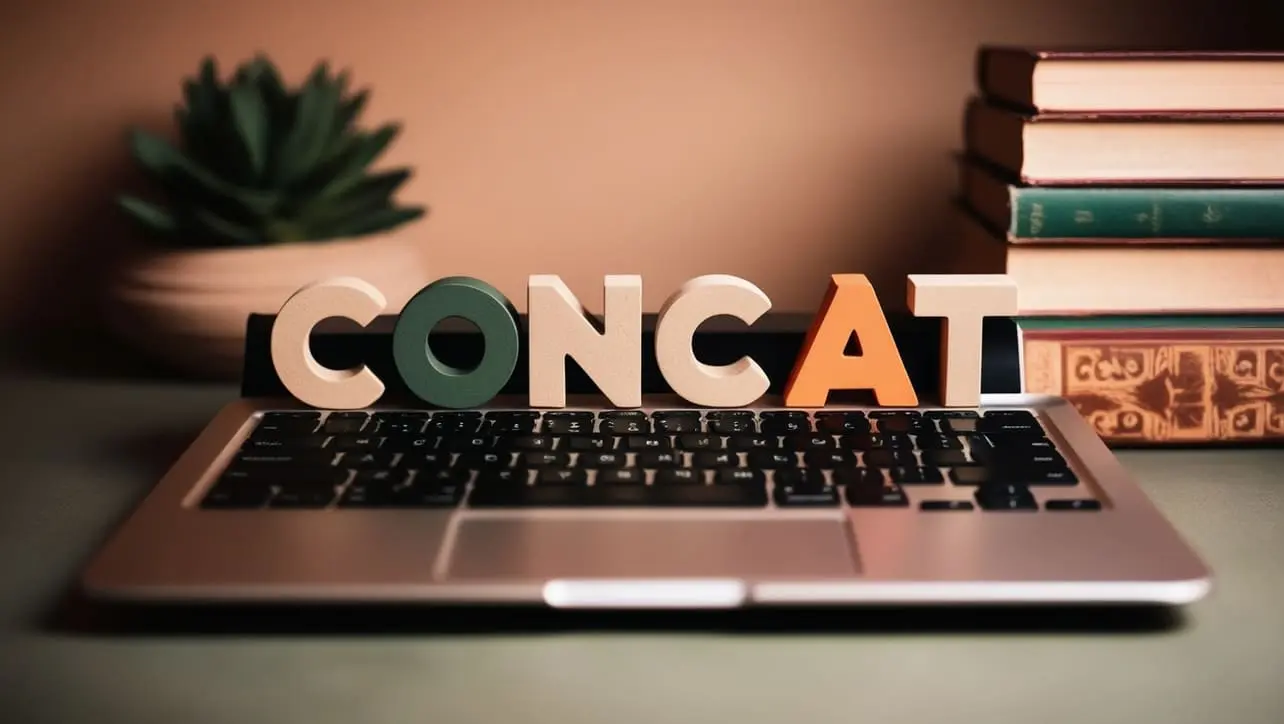
If you have any doubts regarding this article (Java Program to Check Power of 3), please comment here. I will help you immediately.