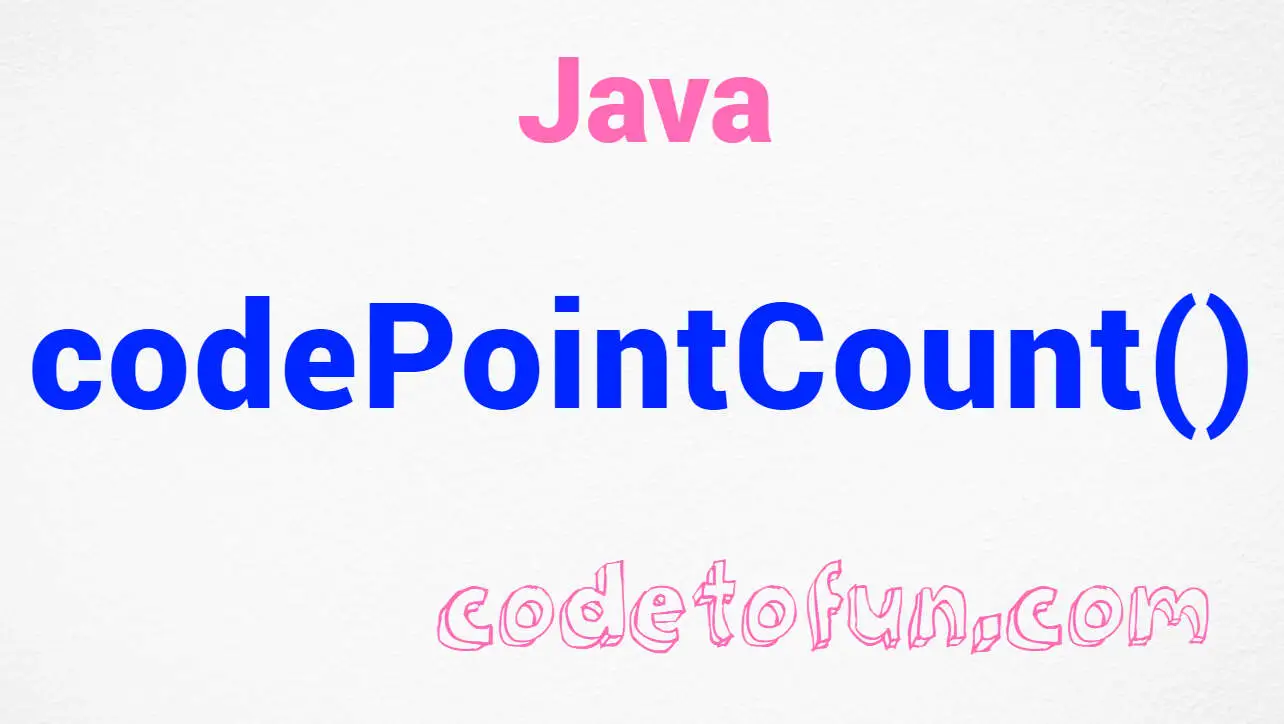
Java Basic
Java Interview Programs
- Java Interview Programs
- Java Abundant Number
- Java Amicable Number
- Java Armstrong Number
- Java Average of N Numbers
- Java Automorphic Number
- Java Biggest of three numbers
- Java Binary to Decimal
- Java Common Divisors
- Java Composite Number
- Java Condense a Number
- Java Cube Number
- Java Decimal to Binary
- Java Decimal to Octal
- Java Disarium Number
- Java Even Number
- Java Evil Number
- Java Factorial of a Number
- Java Fibonacci Series
- Java GCD
- Java Happy Number
- Java Harshad Number
- Java LCM
- Java Leap Year
- Java Magic Number
- Java Matrix Addition
- Java Matrix Division
- Java Matrix Multiplication
- Java Matrix Subtraction
- JS Matrix Transpose
- Java Maximum Value of an Array
- Java Minimum Value of an Array
- Java Multiplication Table
- Java Natural Number
- Java Number Combination
- Java Odd Number
- Java Palindrome Number
- Java Pascalβs Triangle
- Java Perfect Number
- Java Perfect Square
- Java Power of 2
- Java Power of 3
- Java Pronic Number
- Java Prime Factor
- Java Prime Number
- Java Smith Number
- Java Strong Number
- Java Sum of Array
- Java Sum of Digits
- Java Swap Two Numbers
- Java Triangular Number
Java Program to Check Power of 2
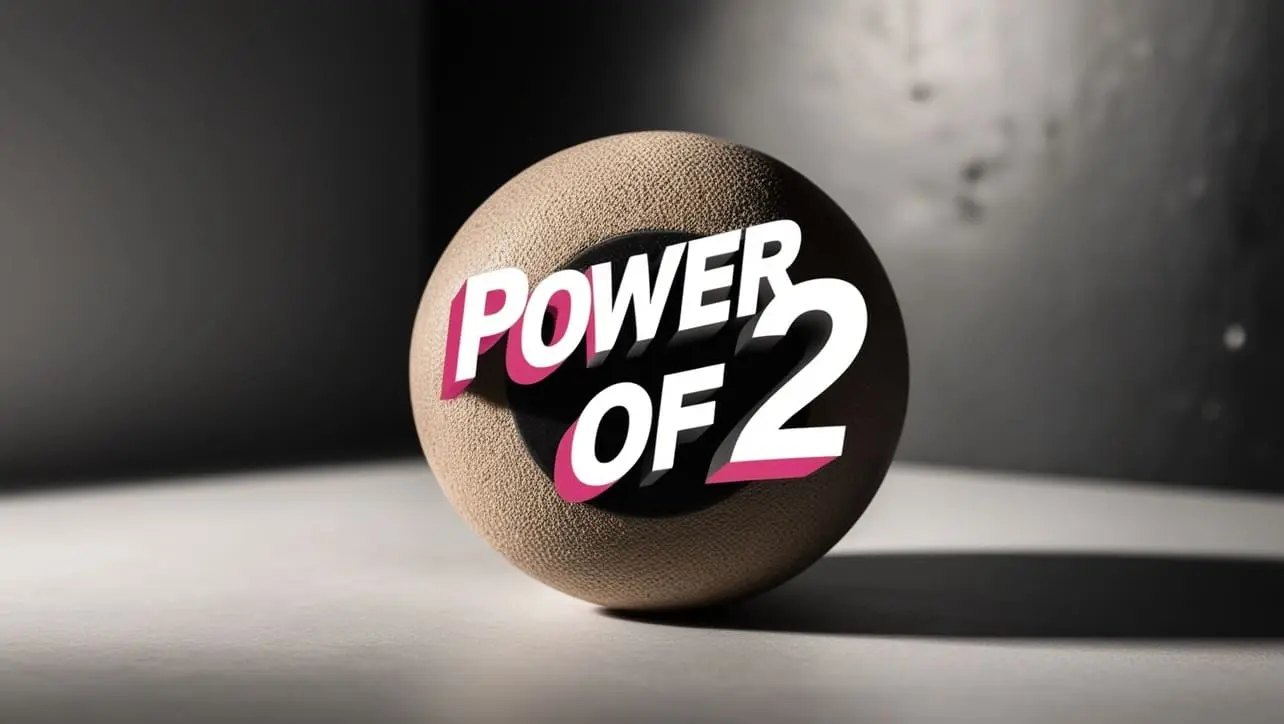
Photo Credit to CodeToFun
π Introduction
In the realm of programming, efficiency and optimization are key considerations. One common optimization involves determining whether a given number is a power of 2.
Checking if a number is a power of 2 is a fundamental operation with applications in various domains.
In this tutorial, we'll explore a Java program that efficiently checks whether a given number is a power of 2.
π Example
Let's take a look at the Java code that accomplishes this functionality.
public class PowerOfTwoChecker {
// Function to check if a number is a power of 2
static boolean isPowerOfTwo(int num) {
// A number is a power of 2 if and only if it has a single set bit.
// Using bitwise AND operation to check this condition.
return (num > 0) && ((num & (num - 1)) == 0);
}
// Driver program
public static void main(String[] args) {
// Replace this value with your desired number
int number = 16;
// Check if the number is a power of 2
if (isPowerOfTwo(number)) {
System.out.println(number + " is a power of 2.");
} else {
System.out.println(number + " is not a power of 2.");
}
}
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main method.
16 is a power of 2.
Compile and run the program to see whether the given number is a power of 2.
π§ How the Program Works
- The program defines a class PowerOfTwoChecker containing a static method isPowerOfTwo that takes an integer as input and returns true if the number is a power of 2 and false otherwise.
- Inside the method, it uses a bitwise AND operation to check if the number has a single set bit, which is a characteristic of power-of-2 numbers.
- The main method demonstrates the usage of the isPowerOfTwo method by checking if a given number is a power of 2.
π Between the Given Range
Let's dive into the java code that performs the check for powers of 2 in the range 1 to 20.
public class PowerOfTwoChecker {
// Function to check if a number is a power of 2
static boolean isPowerOfTwo(int num) {
return (num > 0) && ((num & (num - 1)) == 0);
}
// Driver program to check powers of 2 in the range 1 to 20
public static void main(String[] args) {
System.out.println("Power of 2 in the range 1 to 20:");
for (int i = 1; i <= 20; i++) {
if (isPowerOfTwo(i)) {
System.out.print(i + " ");
}
}
}
}
π» Testing the Program
Power of 2 in the range 1 to 20: 1 2 4 8 16
Compile and run the program to see the powers of 2 in the range from 1 to 20.
π§ How the Program Works
- The program defines a class PowerOfTwoChecker containing a static method isPowerOfTwo to check if a number is a power of 2.
- Inside the main method, it iterates through numbers from 1 to 20.
- For each number, it calls the isPowerOfTwo method to check if it's a power of 2.
- If the number is a power of 2, it is printed.
π§ Understanding the Concept of Power of 2
A number is considered a power of 2 if and only if it has a single set bit.
For example, 2, 4, 8, and 16 are powers of 2 because their binary representations have only one bit set.
Understanding this concept is crucial for efficient programming and algorithm design.
π’ Optimizing the Program
The provided program is a straightforward implementation. However, there are more advanced techniques for checking if a number is a power of 2, such as using logarithmic operations or counting the number of set bits. Depending on your specific use case, you might explore these optimizations.
Feel free to incorporate and modify this code as needed for your specific requirements. Happy coding!
π¨βπ» Join our Community:
Author
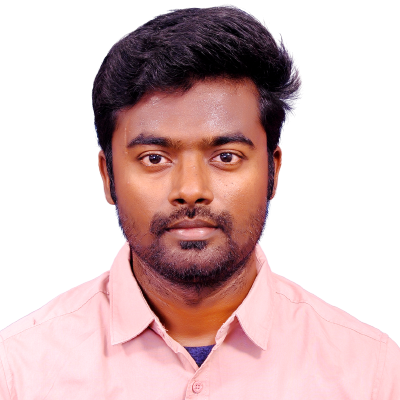
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Program to Check Power of 2), please comment here. I will help you immediately.