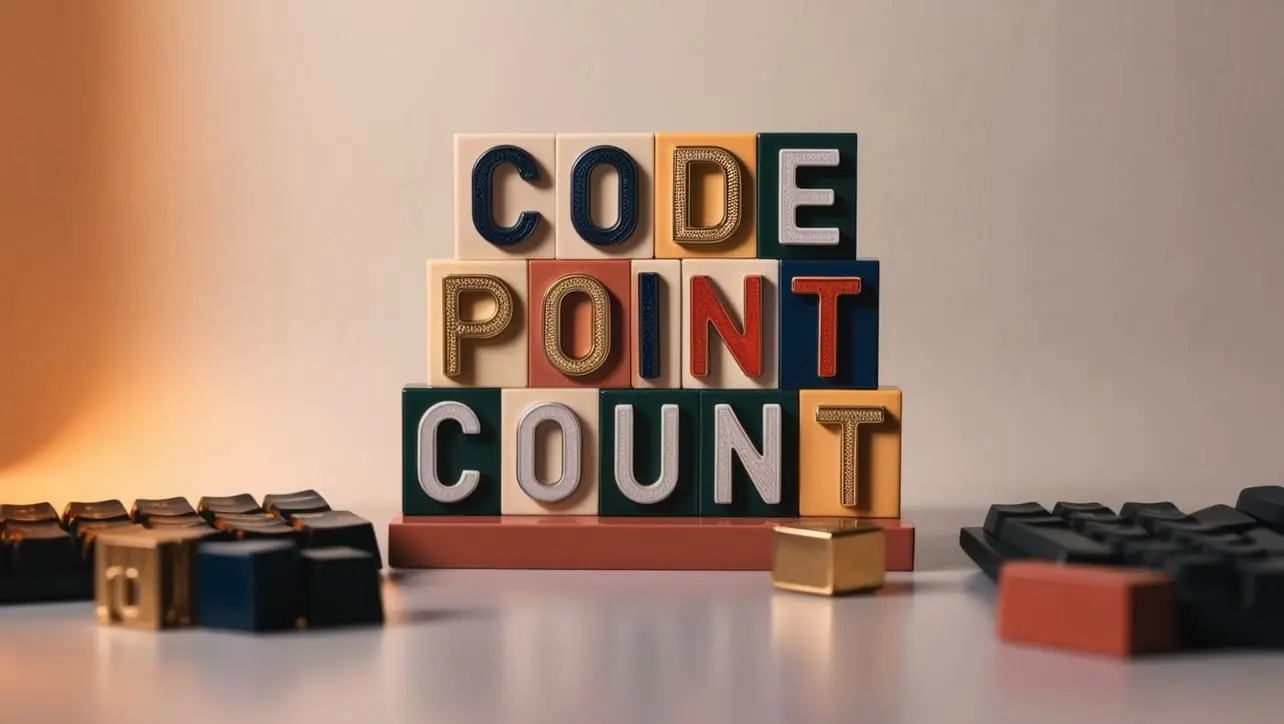
Java Topics
- Java Intro
- Java String Methods
- Java Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Java Star Pattern
- Java Number Pattern
- Java Alphabet Pattern
Java Program to check Perfect Square
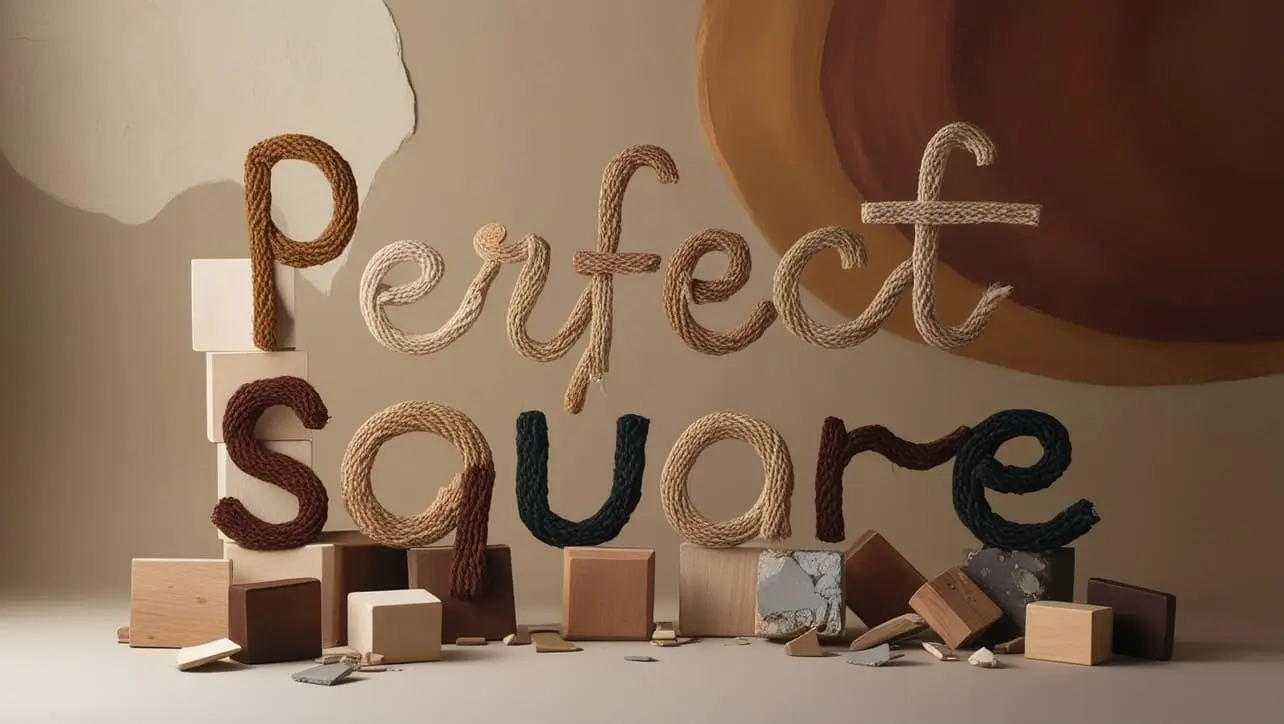
Photo Credit to CodeToFun
π Introduction
In the realm of programming, various mathematical problems challenge programmers to devise efficient solutions. One such problem is determining whether a given number is a perfect square.
A perfect square is a number that is the square of an integer, meaning it can be expressed as the product of an integer multiplied by itself.
In this tutorial, we'll explore a Java program that efficiently checks whether a given number is a perfect square or not.
π Example
Let's take a look at the Java code that accomplishes this task.
public class PerfectSquareChecker {
// Function to check if a number is a perfect square
static boolean isPerfectSquare(int number) {
for (int i = 1; i * i <= number; i++) {
// If i * i is equal to the number, it's a perfect square
if (i * i == number) {
return true;
}
}
return false;
}
// Driver program
public static void main(String[] args) {
// Replace this value with your desired number
int testNumber = 16;
// Check if the number is a perfect square
if (isPerfectSquare(testNumber)) {
System.out.println(testNumber + " is a perfect square.");
} else {
System.out.println(testNumber + " is not a perfect square.");
}
}
}
π» Testing the Program
To test the program with different numbers, simply replace the value of testNumber in the main method.
16 is a perfect square.
Compile and run the program to see if the number is a perfect square.
π§ How the Program Works
- The program defines a class PerfectSquareChecker containing a static method isPerfectSquare that takes an integer as input and returns true if the number is a perfect square, and false otherwise.
- Inside the method, it iterates through integers starting from 1 until the square of the current integer is greater than the given number.
- If the square of the current integer is equal to the given number, the method returns true indicating a perfect square.
- The main program then checks if a specific number (in this case, 16) is a perfect square and prints the result.
π Between the Given Range
Let's take a look at the java code that checks for perfect squares in the specified range.
public class PerfectSquareChecker {
// Function to check if a number is a perfect square
static boolean isPerfectSquare(int num) {
int sqrt = (int) Math.sqrt(num);
return sqrt * sqrt == num;
}
// Driver program
public static void main(String[] args) {
System.out.println("Perfect Squares in the range 1 to 50:");
// Check for perfect squares in the range
for (int i = 1; i <= 50; i++) {
if (isPerfectSquare(i)) {
System.out.print(i + " ");
}
}
}
}
π» Testing the Program
Perfect Squares in the Range 1 to 50: 1 4 9 16 25 36 49
Compile and run the program to see the perfect squares in the range from 1 to 50.
π§ How the Program Works
- The program defines a class PerfectSquareChecker containing a static method isPerfectSquare that checks if a number is a perfect square.
- Inside the method, it calculates the square root of the number and checks if the square of the square root equals the original number.
- The main method iterates through numbers from 2 to 50 and prints those that are perfect squares.
π§ Understanding the Concept of Perfect Square
Before diving into the code, let's take a moment to understand the concept of perfect squares.
A perfect square is a number that can be expressed as the product of an integer multiplied by itself.
For example, 4, 9, and 16 are perfect squares because they are the squares of 2, 3, and 4, respectively.
π’ Optimizing the Program
While the provided program is effective, there are alternative methods for checking perfect squares that involve mathematical properties. Consider exploring and implementing such optimizations to enhance the efficiency of your program.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
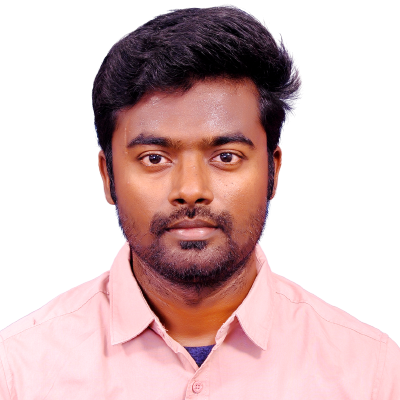
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Java
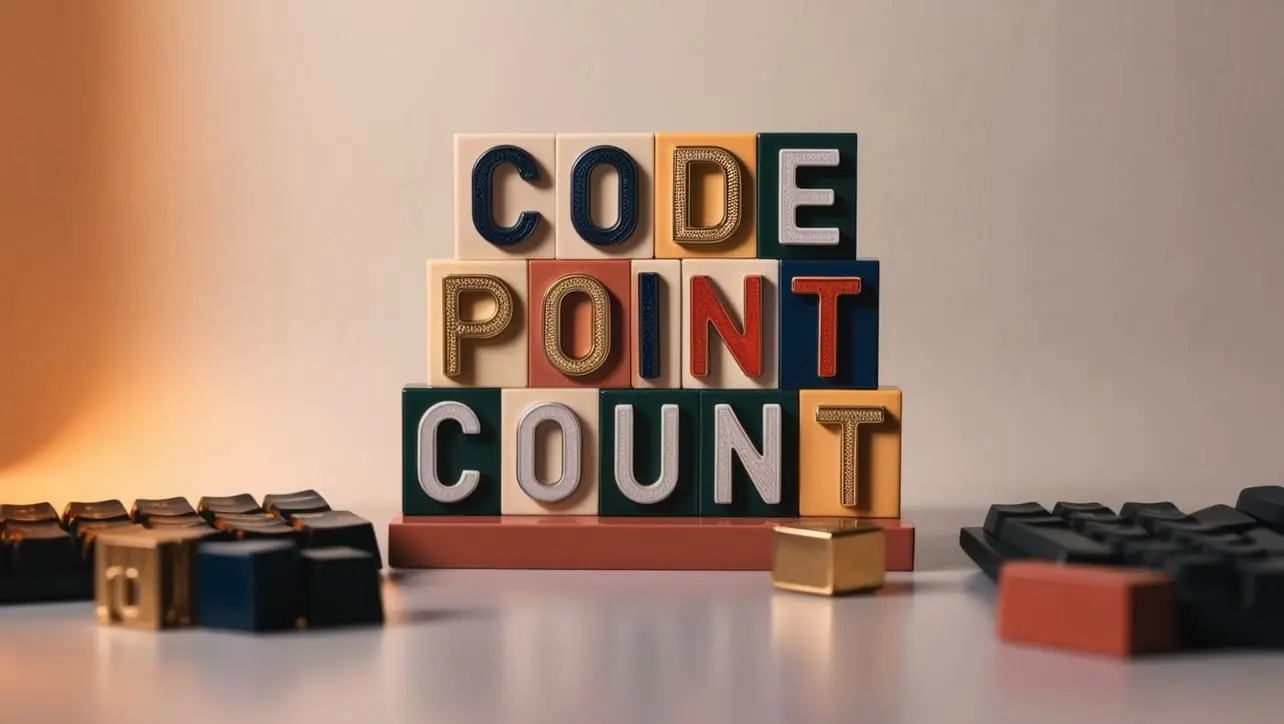
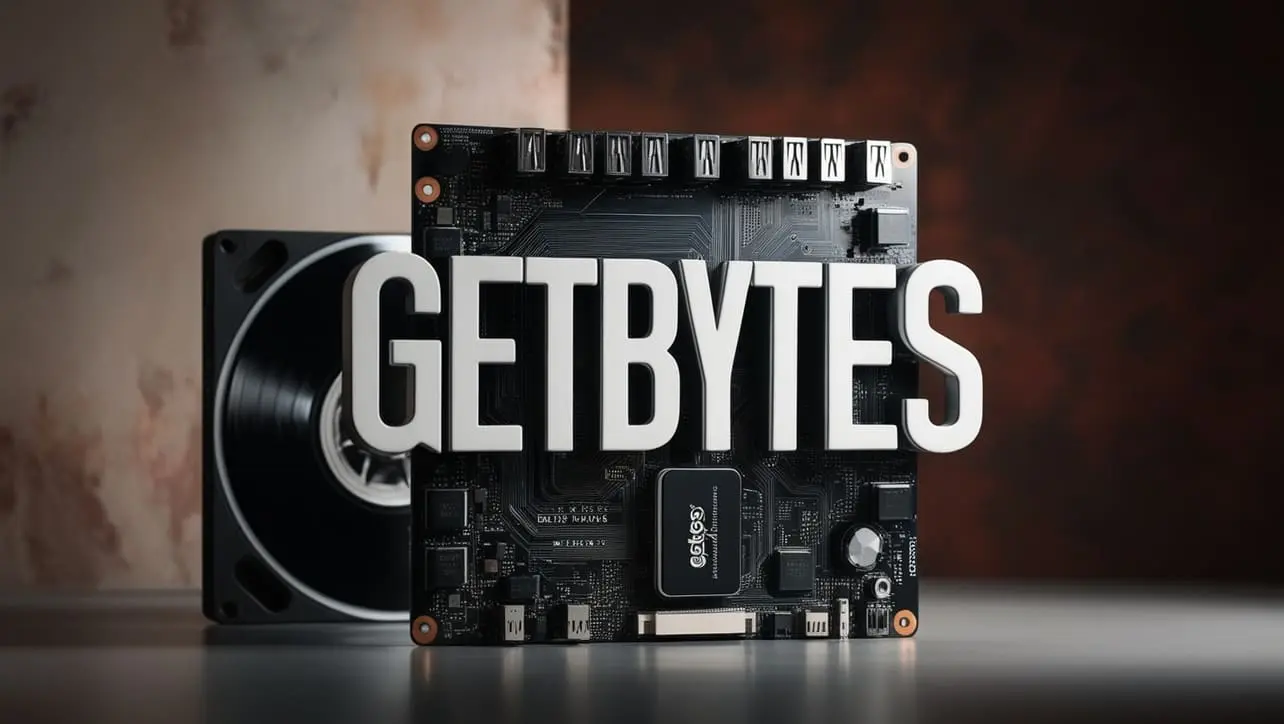
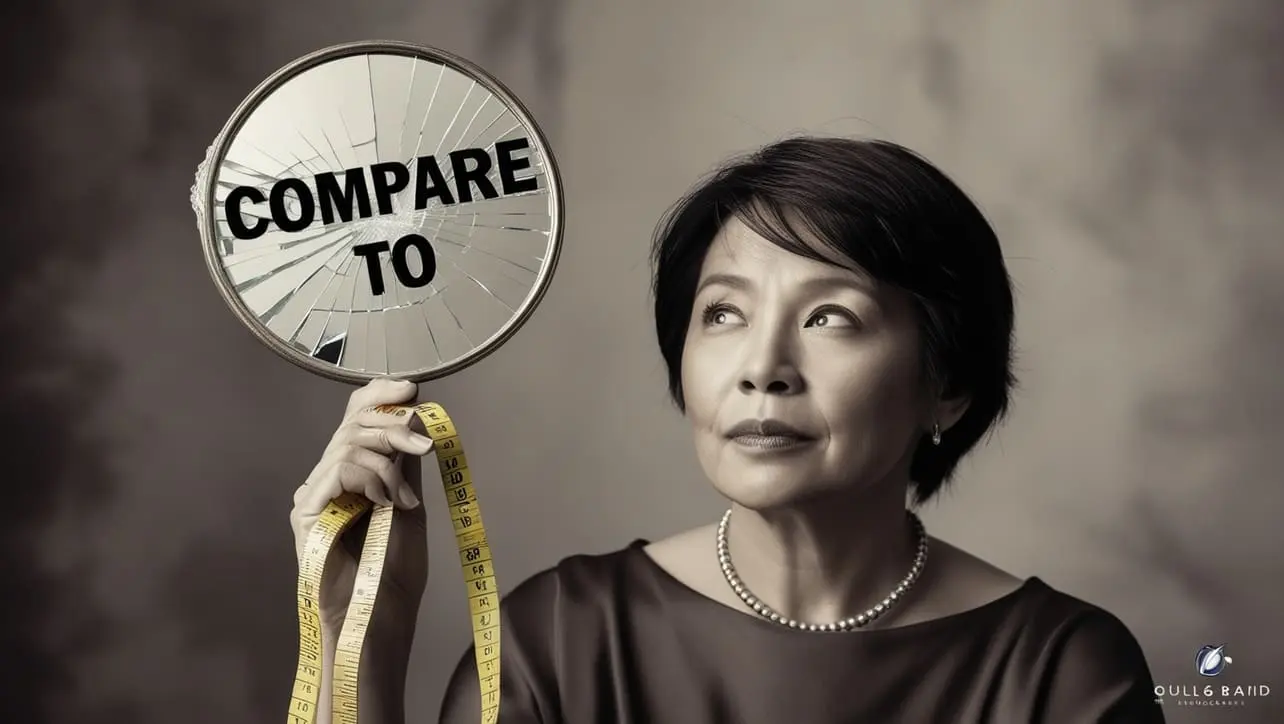
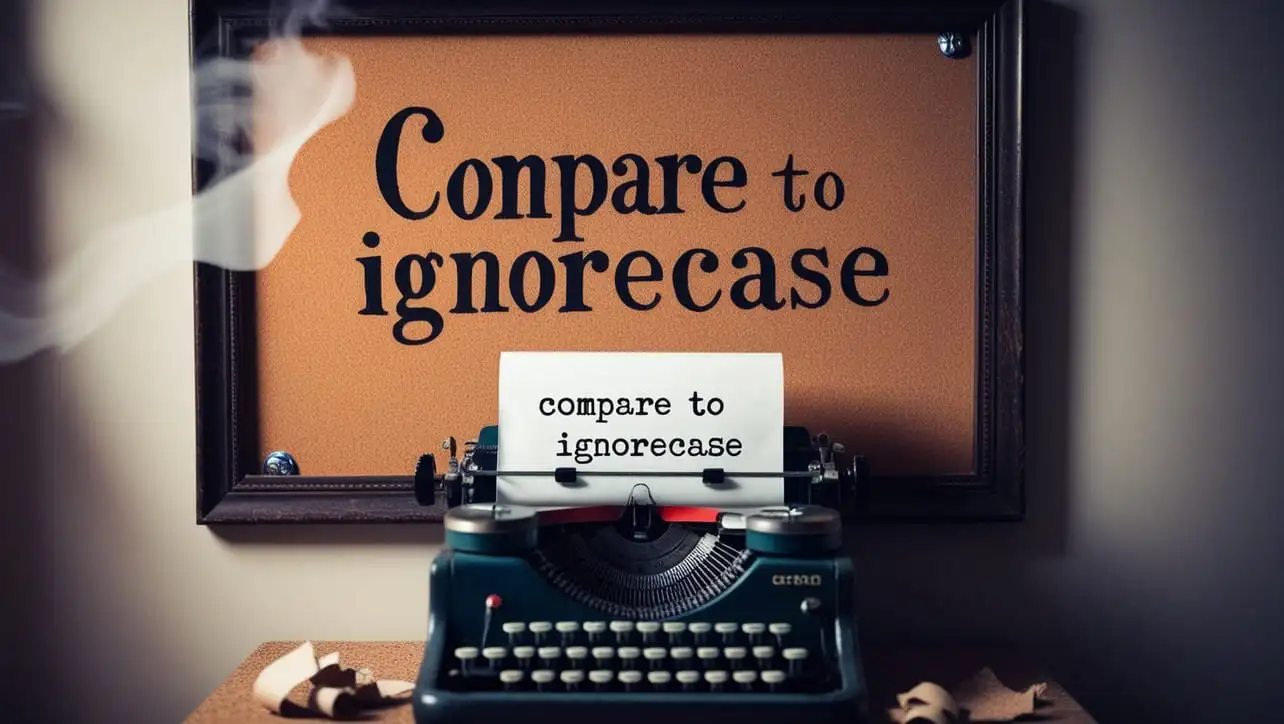
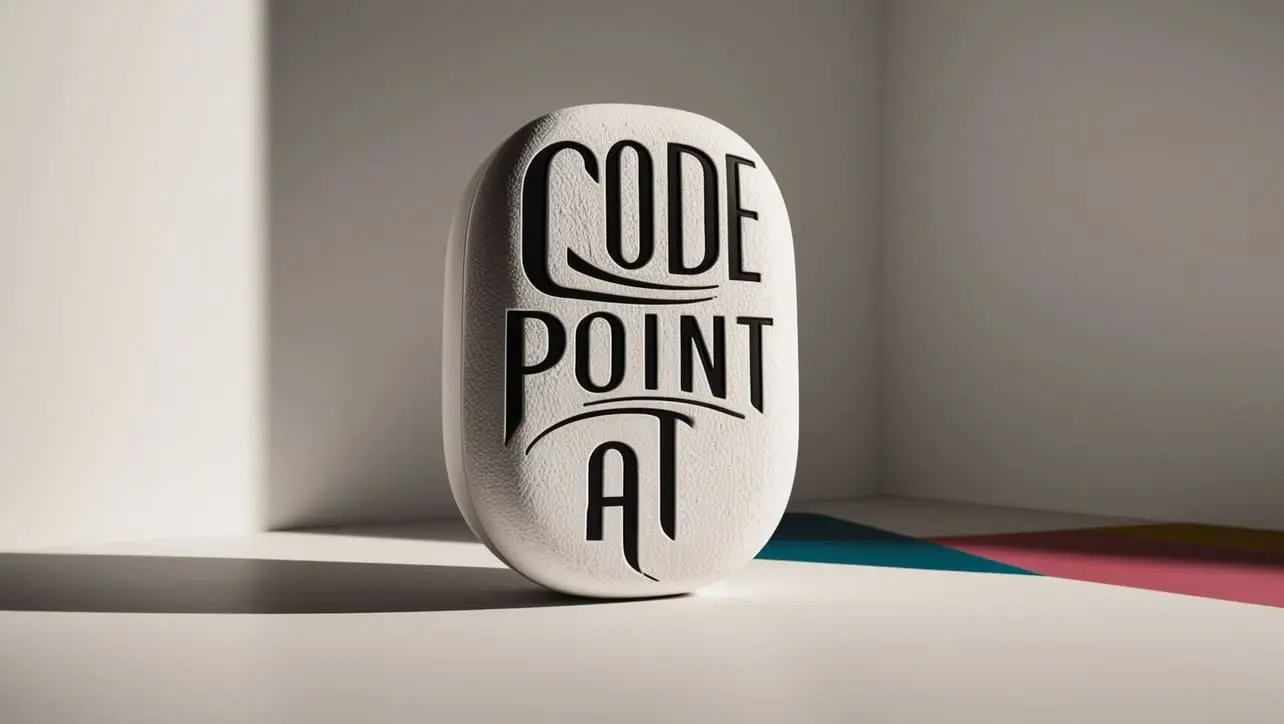
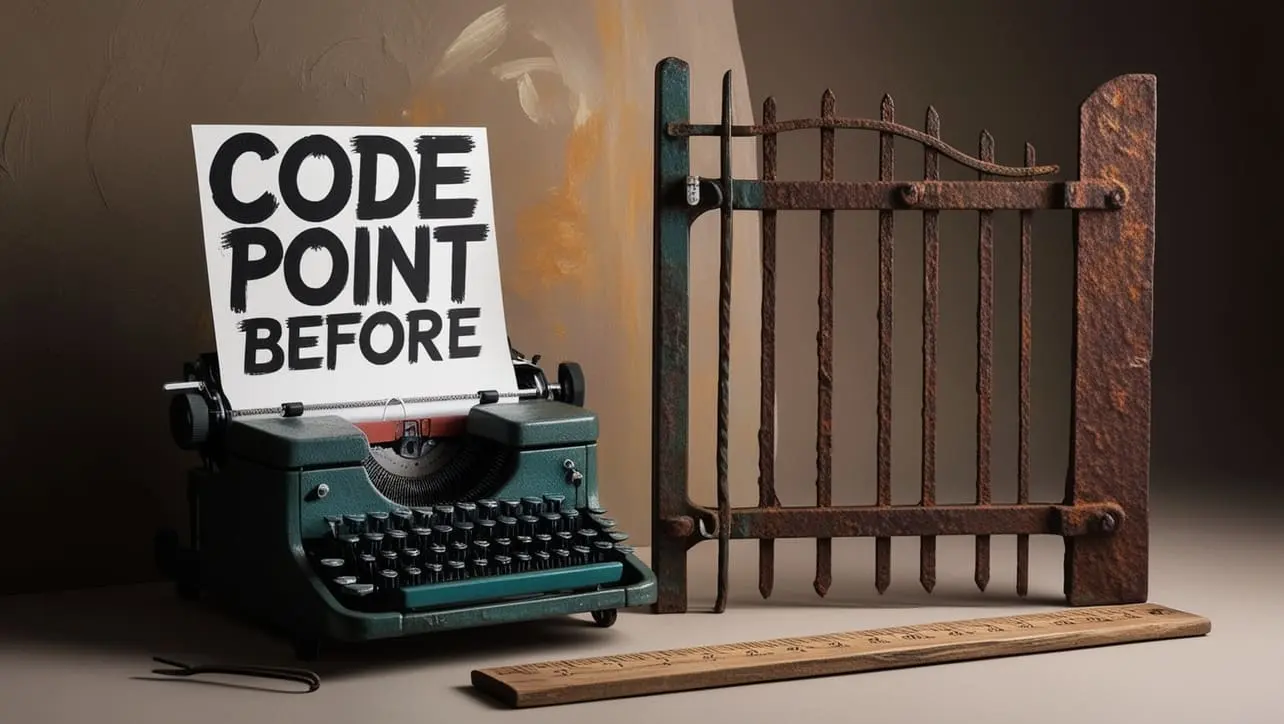
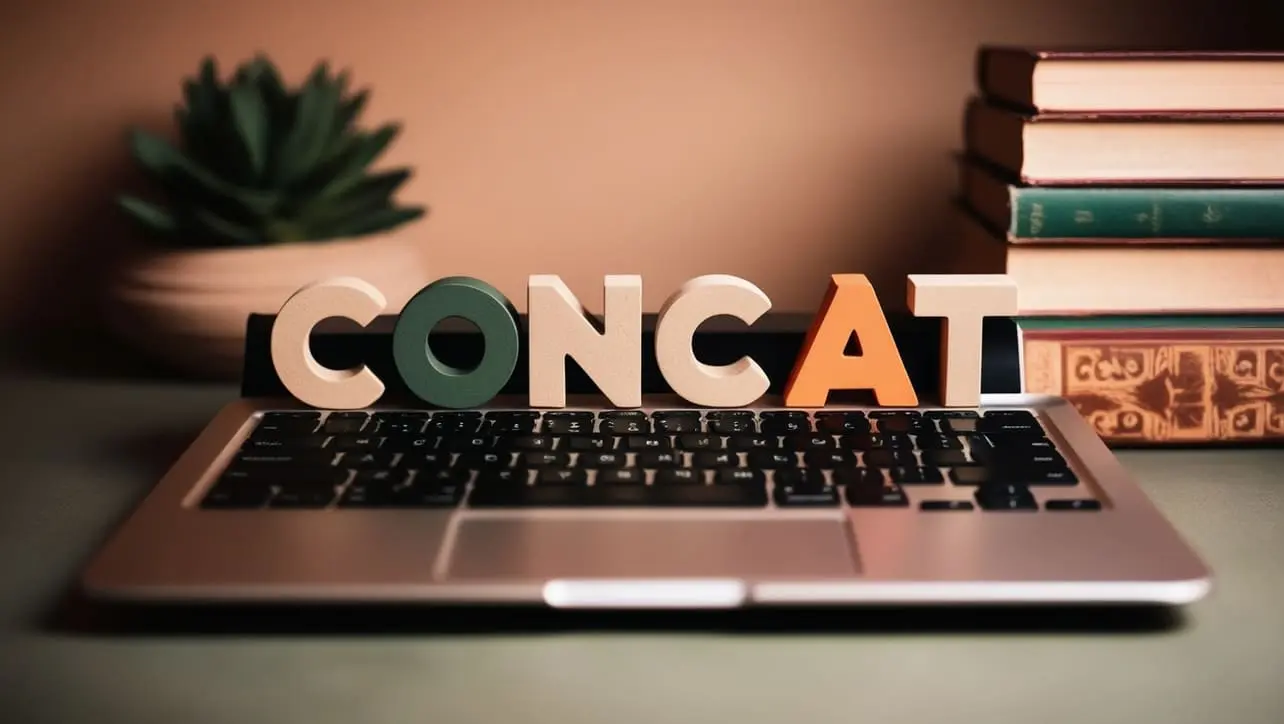
If you have any doubts regarding this article (Java Program to check Perfect Square), please comment here. I will help you immediately.