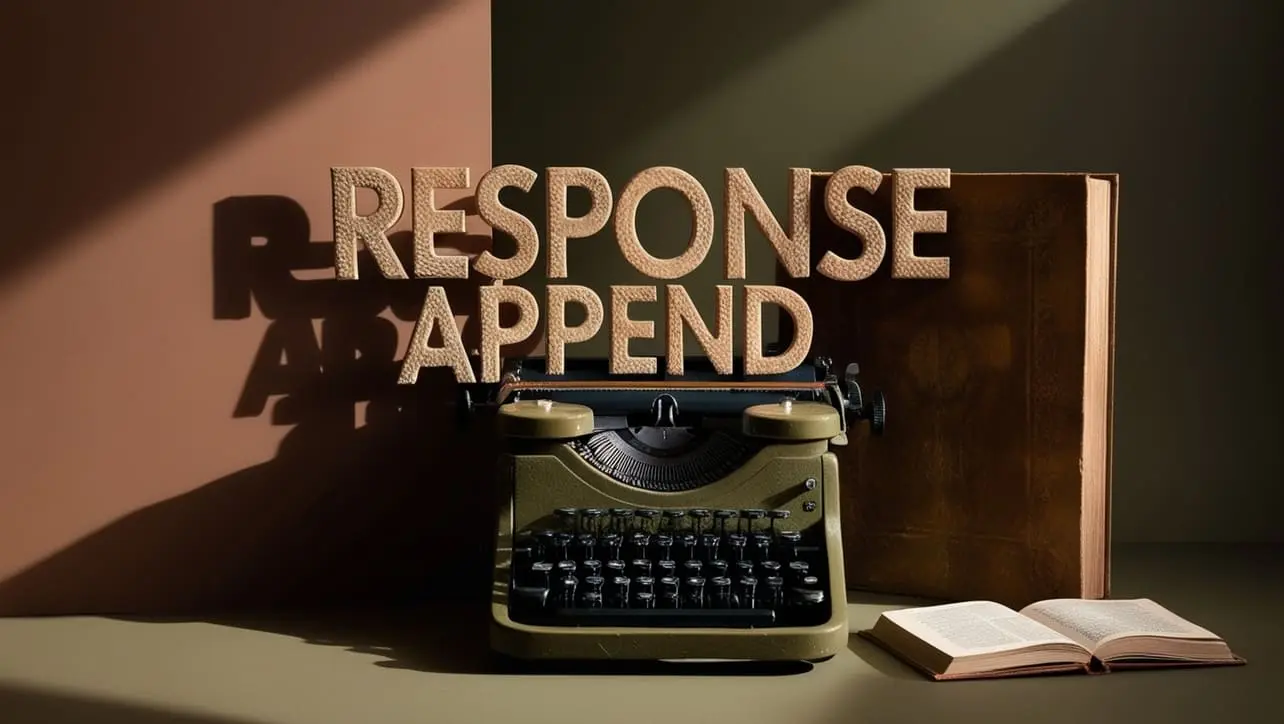
Express router.route() Method
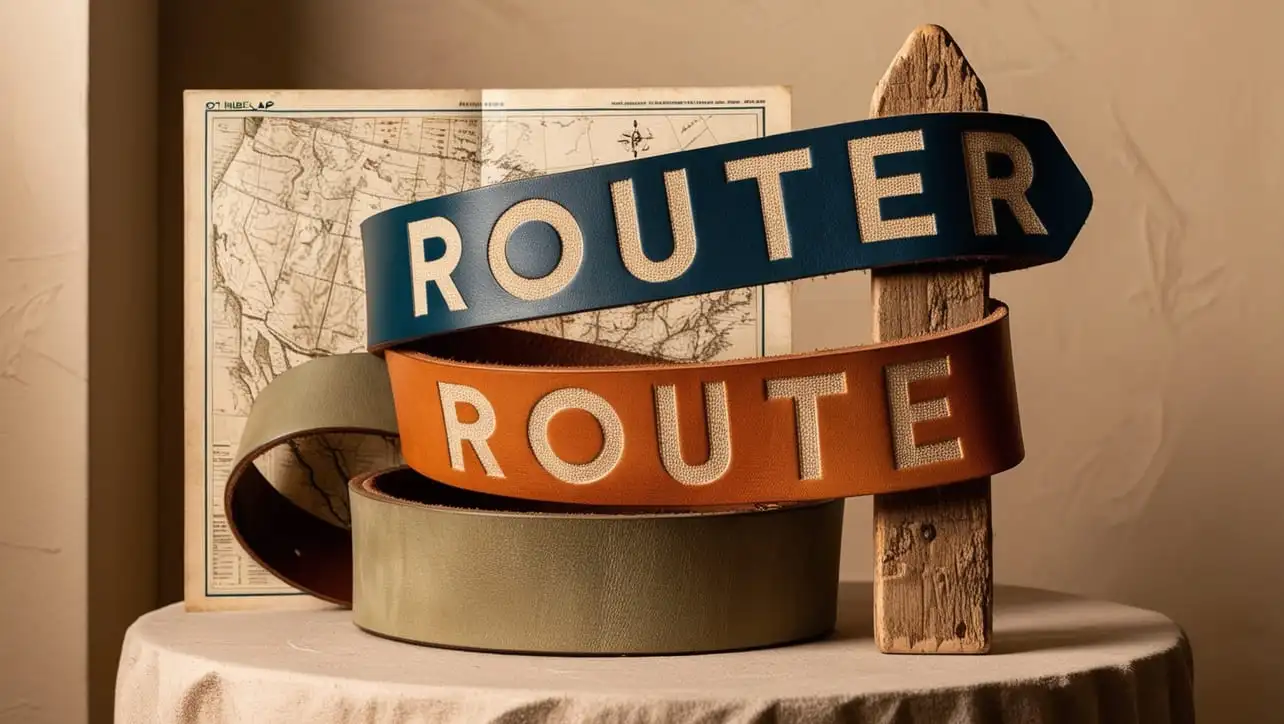
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a popular web application framework for Node.js, offers various features to simplify route handling. One such feature is the router.route()
method, which allows you to define multiple route handlers for a specific route path.
In this guide, we'll explore the syntax, use cases, and best practices of the router.route()
method to help you streamline and organize your Express.js routes.
💡 Syntax
The syntax for the router.route()
method is concise and facilitates the chaining of HTTP method handlers:
const express = require('express');
const router = express.Router();
router.route('/path')
.get(callback)
.post(callback)
.put(callback)
.delete(callback);
Here, you can chain multiple HTTP method handlers for the specified route path.
❓ How router.route() Works
The router.route()
method allows you to group route handlers for a specific path, making your code more modular and easier to maintain. By chaining different HTTP method handlers, you can handle various types of requests for the same route path.
const express = require('express');
const router = express.Router();
router.route('/users')
.get((req, res) => {
// Logic to handle GET request for /users
res.send('Get all users');
})
.post((req, res) => {
// Logic to handle POST request for /users
res.send('Create a new user');
})
.put((req, res) => {
// Logic to handle PUT request for /users
res.send('Update all users');
})
.delete((req, res) => {
// Logic to handle DELETE request for /users
res.send('Delete all users');
});
In this example, the router.route('/users') groups together handlers for GET, POST, PUT, and DELETE requests on the '/users' route path.
📚 Use Cases
Organizing CRUD Operations:
Group CRUD (Create, Read, Update, Delete) operations for a resource under a single route using
router.route()
.example.jsCopiedconst express = require('express'); const router = express.Router(); router.route('/products') .get((req, res) => { // Logic to retrieve all products res.send('Get all products'); }) .post((req, res) => { // Logic to create a new product res.send('Create a new product'); }) .put((req, res) => { // Logic to update all products res.send('Update all products'); }) .delete((req, res) => { // Logic to delete all products res.send('Delete all products'); });
Middleware Integration:
Integrate middleware functions for authentication or authorization within the
router.route()
structure.example.jsCopiedconst express = require('express'); const router = express.Router(); const authenticateUser = (req, res, next) => { // Implement your authentication logic here if (req.isAuthenticated()) { return next(); } else { res.status(401).send('Unauthorized'); } }; router.route('/dashboard') .get(authenticateUser, (req, res) => { // Logic to render the dashboard for authenticated users res.send('Dashboard for authenticated user'); }) .post(authenticateUser, (req, res) => { // Logic to update the dashboard for authenticated users res.send('Update dashboard for authenticated user'); });
🏆 Best Practices
Maintainability through Modularity:
Organize your routes in a modular fashion, grouping related handlers together using
router.route()
. This enhances code readability and maintainability.example.jsCopiedconst express = require('express'); const router = express.Router(); const userRoute = router.route('/users'); const productRoute = router.route('/products'); userRoute .get(getAllUsers) .post(createUser) .put(updateAllUsers) .delete(deleteAllUsers); productRoute .get(getAllProducts) .post(createProduct) .put(updateAllProducts) .delete(deleteAllProducts);
Middleware Reusability:
Leverage middleware functions across multiple HTTP methods within the same route path, reducing code duplication.
example.jsCopiedconst express = require('express'); const router = express.Router(); const authenticateUser = (req, res, next) => { // Implement your authentication logic here if (req.isAuthenticated()) { return next(); } else { res.status(401).send('Unauthorized'); } }; router.route('/dashboard') .get(authenticateUser, (req, res) => { // Logic to render the dashboard for authenticated users res.send('Dashboard for authenticated user'); }) .post(authenticateUser, (req, res) => { // Logic to update the dashboard for authenticated users res.send('Update dashboard for authenticated user'); });
🎉 Conclusion
The router.route()
method in Express.js is a powerful tool for organizing and streamlining route handling. Whether you're managing CRUD operations or integrating middleware, understanding how to use router.route()
will contribute to the clarity and maintainability of your Express.js applications.
Now equipped with knowledge about the router.route()
method, go ahead and optimize your route-handling code in Express.js!
👨💻 Join our Community:
Author
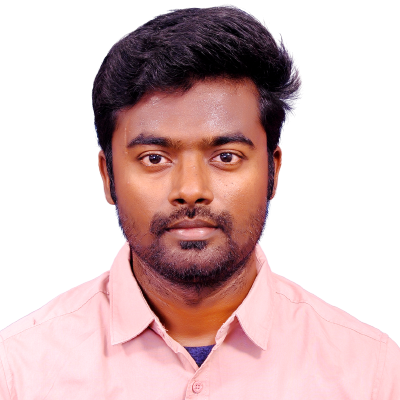
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express router.route() Method), please comment here. I will help you immediately.