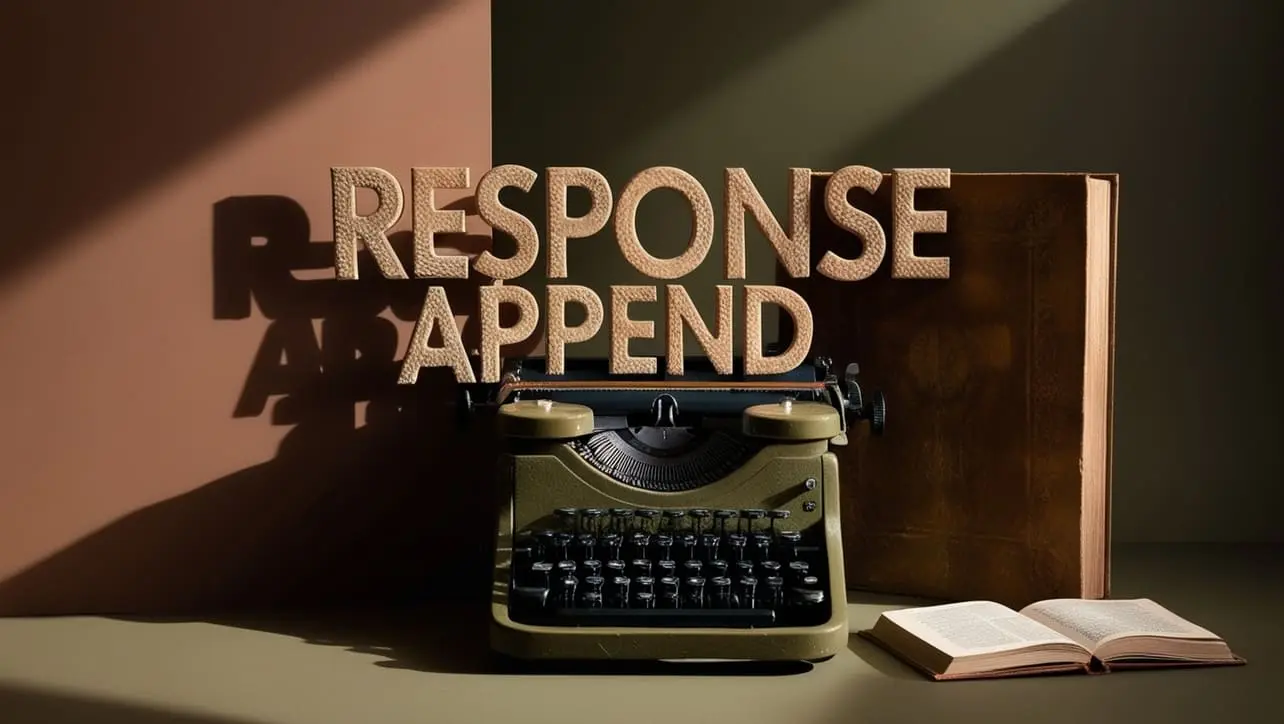
Express router.param() Method
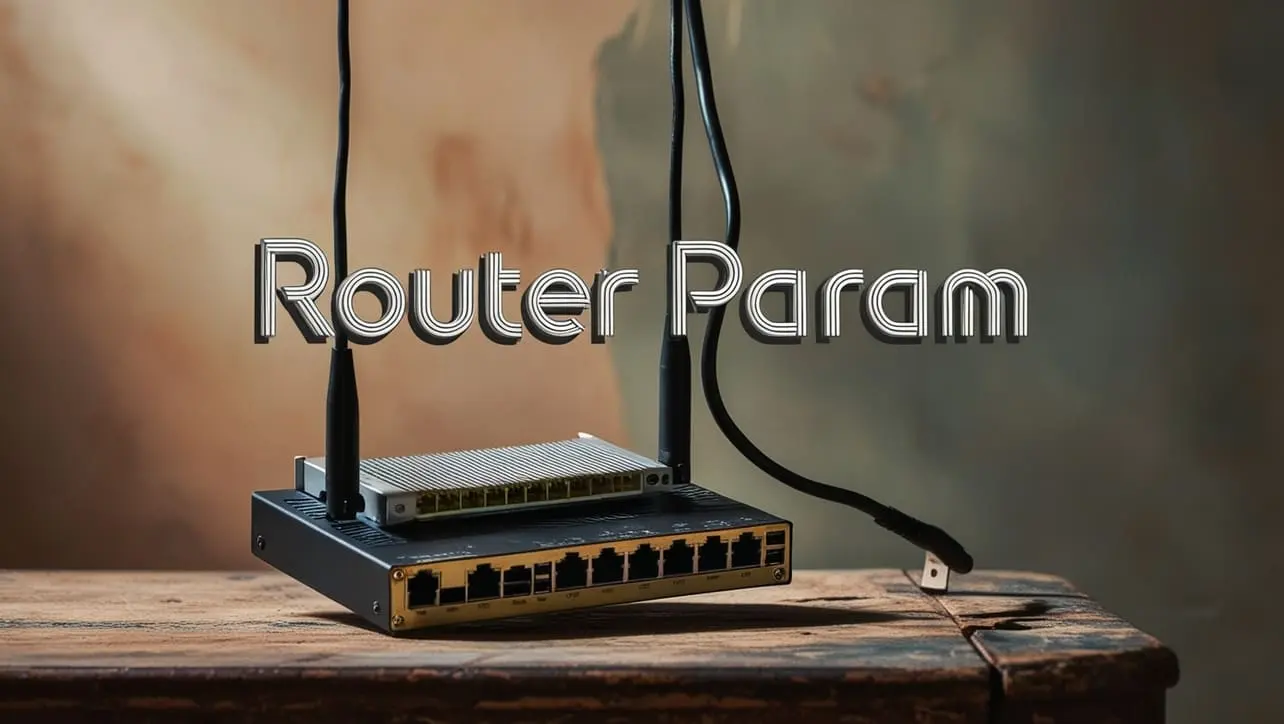
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a powerful Node.js web application framework, provides developers with tools to create dynamic and flexible routes. The router.param()
method is a key feature that allows you to handle dynamic route parameters efficiently.
In this guide, we'll dive into the syntax, usage, and examples of the router.param()
method, demonstrating how it facilitates handling dynamic parameters in Express.js.
💡 Syntax
The syntax for the router.param()
method is straightforward:
router.param(paramName, callback)
- paramName: A string representing the name of the route parameter.
- callback: The callback function that is invoked when the specified parameter is present in a route.
❓ How router.param() Works
The router.param()
method is used to define middleware that is executed when a specific parameter is present in a route. This is particularly useful for validating, modifying, or pre-processing parameters before reaching the route handler.
const express = require('express');
const router = express.Router();
// Define a middleware for handling the 'userId' parameter
router.param('userId', (req, res, next, userId) => {
// Perform validation or modification on 'userId'
// For simplicity, let's assume validation passes
req.userId = userId;
next();
});
// Define a route using the 'userId' parameter
router.get('/users/:userId', (req, res) => {
res.send(`User ID: ${req.userId}`);
});
module.exports = router;
In this example, the router.param()
middleware is used to validate and set the userId parameter before it reaches the route handler.
📚 Use Cases
Parameter Validation:
Use
router.param()
to validate dynamic parameters, ensuring they meet specific criteria before reaching the route handler.example.jsCopiedrouter.param('postId', (req, res, next, postId) => { // Validate the 'postId' parameter if (isValidPostId(postId)) { req.postId = postId; next(); } else { res.status(404).send('Invalid Post ID'); } });
Pre-processing Parameters:
Leverage
router.param()
for pre-processing parameters, transforming them before they are used in route handlers.example.jsCopiedrouter.param('category', (req, res, next, category) => { // Convert the 'category' parameter to lowercase req.category = category.toLowerCase(); next(); });
🏆 Best Practices
Order Matters:
When using multiple
router.param()
calls, the order in which they are defined matters. Express executes them in the order they are declared, so place more specific parameter handlers before generic ones.example.jsCopiedrouter.param('userId', (req, res, next, userId) => { // Handle 'userId' parameter // ... next(); }); router.param('postId', (req, res, next, postId) => { // Handle 'postId' parameter // ... next(); });
Reusability:
Encapsulate common parameter-handling logic in separate functions and reuse them across different routes.
example.jsCopiedconst handleUserIdParam = (req, res, next, userId) => { // Common logic for handling 'userId' // ... next(); }; router.param('userId', handleUserIdParam); router.get('/users/:userId', handleUserIdParam, (req, res) => { // Route handler // ... });
🎉 Conclusion
The router.param()
method in Express.js provides a powerful mechanism for handling dynamic route parameters. Whether you need to validate, preprocess, or modify parameters, understanding how to leverage router.param()
will enhance your ability to create dynamic and efficient routes in Express.js.
Now equipped with the knowledge of router.param()
, go ahead and make your Express.js applications more dynamic and robust!
👨💻 Join our Community:
Author
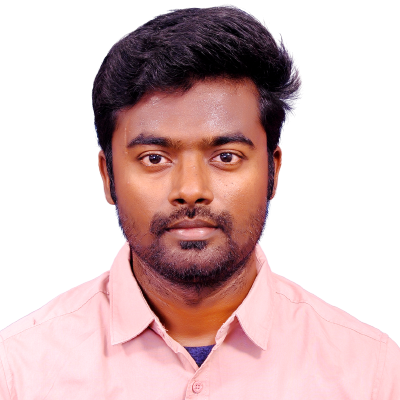
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express router.param() Method), please comment here. I will help you immediately.