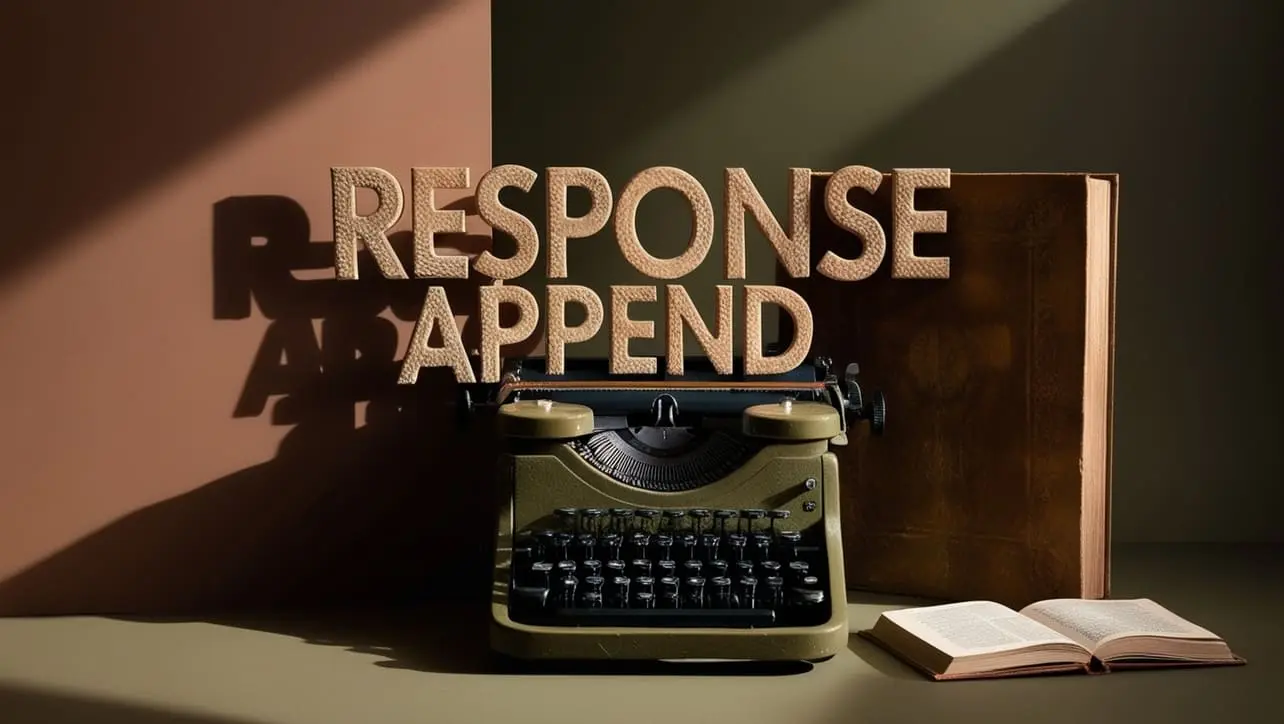
Express router.METHOD() Method
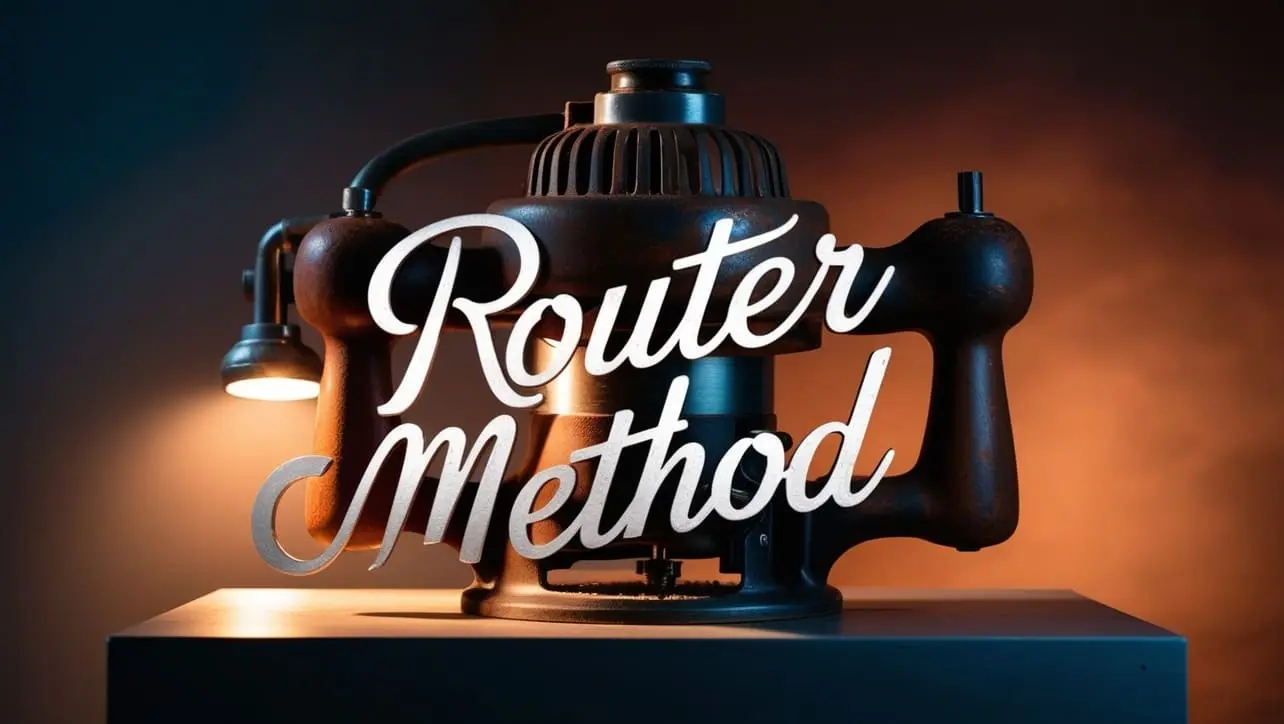
Photo Credit to CodeToFun
🙋 Introduction
In Express.js, the router.METHOD()
method is a fundamental feature that allows you to handle different HTTP methods on specific routes using the Express Router.
This guide explores the syntax, usage, and practical examples of router.METHOD()
, offering insights into how it can be leveraged to create modular and organized route handling in your Express applications.
💡 Syntax
The syntax for the router.METHOD()
method is as follows:
router.METHOD(path, callback)
- METHOD: Represents an HTTP method such as GET, POST, PUT, DELETE, etc.
- path: A string representing the route or path for which the specified HTTP method is being handled.
- callback: The route handler function that processes requests for the specified method and route.
❓ How router.METHOD() Works
Express Router's router.METHOD()
allows you to define route handlers for specific HTTP methods on a given path. This facilitates a cleaner and more organized approach to handling different types of requests.
const express = require('express');
const router = express.Router();
router.get('/example', (req, res) => {
res.send('Handled a GET request at /example');
});
router.post('/example', (req, res) => {
res.send('Handled a POST request at /example');
});
module.exports = router;
In this example, the router handles both GET and POST requests for the '/example' route.
📚 Use Cases
Separation of Concerns:
Organize your route handling by separating different HTTP methods into distinct sections of your application, improving code readability and maintainability.
example.jsCopied// routes/users.js const express = require('express'); const router = express.Router(); router.get('/', (req, res) => { // Logic for retrieving user data res.send('List of users'); }); router.post('/', (req, res) => { // Logic for creating a new user res.send('User created successfully'); }); module.exports = router;
Method-Specific Middleware:
Apply middleware functions specific to certain HTTP methods to enhance route handling flexibility.
example.jsCopiedrouter.post('/login', validateLoginInput, (req, res) => { // Logic for handling login res.send('Login successful'); });
In this example, validateLoginInput is a middleware function applied only to POST requests to /login.
🏆 Best Practices
Code Organization:
Organize your routes by method and path, keeping related functionality together for better maintainability.
example.jsCopied// routes/users.js const express = require('express'); const router = express.Router(); router.get('/', (req, res) => { // Logic for retrieving user data res.send('List of users'); }); router.post('/', (req, res) => { // Logic for creating a new user res.send('User created successfully'); }); module.exports = router;
Method-Specific Middleware:
Leverage method-specific middleware to handle tasks like validation or authentication tailored to the specific HTTP method.
example.jsCopiedrouter.post('/create', validateInput, authenticateUser, (req, res) => { // Logic for creating a resource res.send('Resource created successfully'); });
🎉 Conclusion
The router.METHOD()
method in Express.js provides a powerful mechanism for handling different HTTP methods within your application's routes. By adopting this approach, you can achieve a modular and organized code structure, making your Express applications more scalable and maintainable.
Now, armed with knowledge about router.METHOD()
, take advantage of Express Router to streamline your route handling and build robust web applications!
👨💻 Join our Community:
Author
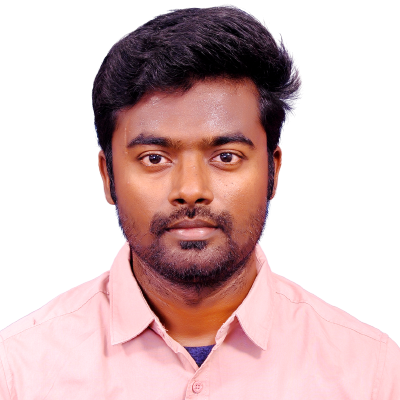
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express router.METHOD() Method), please comment here. I will help you immediately.