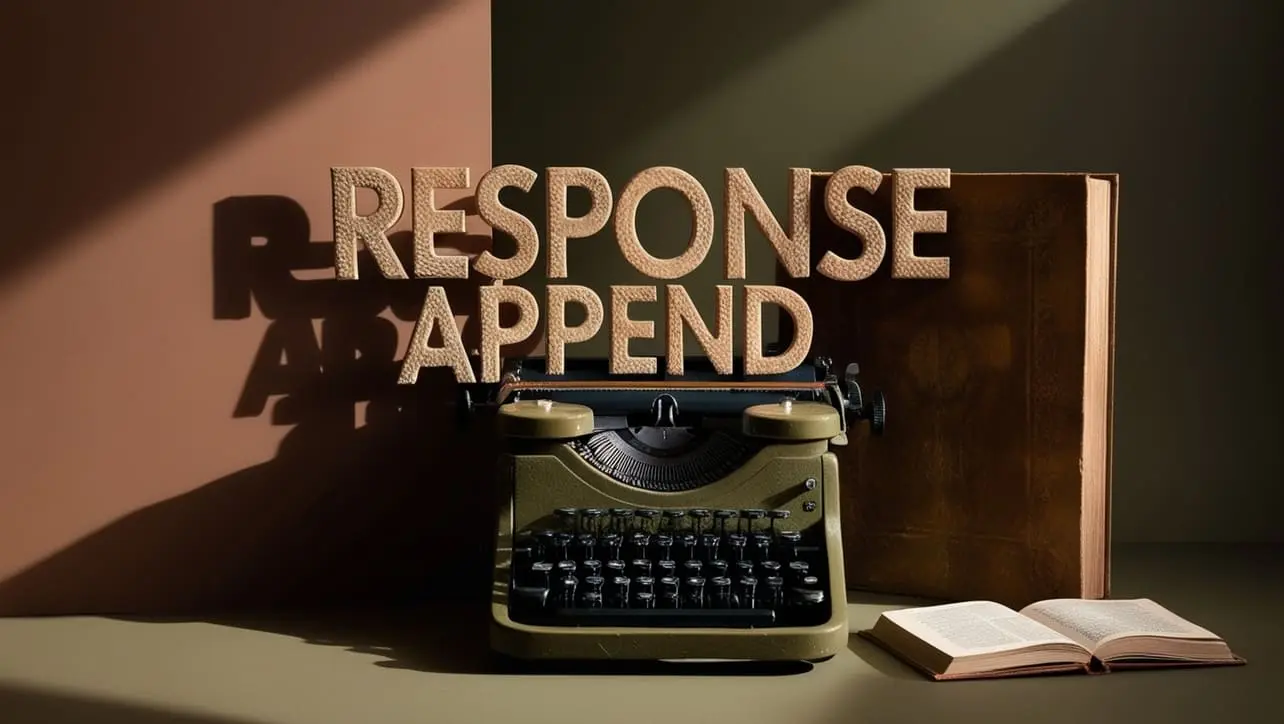
Express res.type() Method
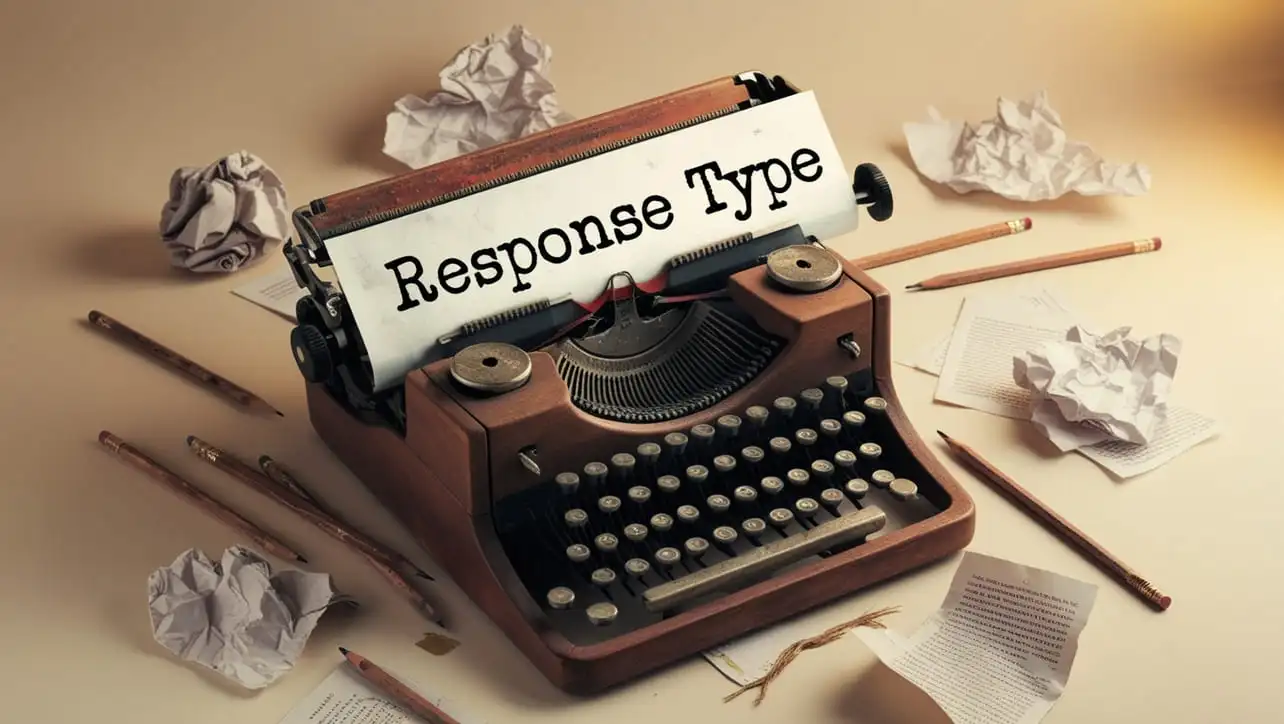
Photo Credit to CodeToFun
🙋 Introduction
In the world of web development, setting the proper Content-Type header is crucial for serving the correct content to clients.
Express.js provides the res.type()
method, a powerful tool for specifying the Content-Type of the response.
Join us as we explore the syntax, use cases, and best practices of the res.type()
method in Express.js.
💡 Syntax
The syntax for the res.type()
method is straightforward:
res.type(type)
- type: A string representing the Content-Type to set for the response.
⚙️ Setting Content-Type
With res.type()
, you can explicitly set the Content-Type for the response, ensuring that clients interpret the content correctly.
app.get('/json', (req, res) => {
res.type('application/json');
res.json({ message: 'Hello, JSON!' });
});
In this example, the res.type('application/json') sets the Content-Type to JSON before sending a JSON response.
📚 Use Cases
Serving Different Content Types:
Use
res.type()
to dynamically set the Content-Type based on the type of content you are serving, such as images, documents, or other file types.example.jsCopiedapp.get('/download/:filename', (req, res) => { const filename = req.params.filename; // Logic to determine the file type const fileType = determineFileType(filename); // Set Content-Type based on the file type res.type(fileType); // Stream the file to the client // (Implementation of file streaming is not shown in this example) });
Handling Multiple Formats in a Single Route:
Leverage
res.type()
to handle multiple response formats in a single route, providing flexibility to clients.example.jsCopiedapp.get('/data', (req, res) => { const format = req.query.format || 'json'; // Set Content-Type based on the requested format if (format === 'json') { res.type('application/json'); res.json({ message: 'Hello, JSON!' }); } else if (format === 'xml') { res.type('application/xml'); res.send('<message>Hello, XML!</message>'); } else { res.status(400).send('Invalid format requested'); } });
🏆 Best Practices
Set Content-Type Early in the Route Handler:
Set the Content-Type as early as possible in your route handler to avoid potential conflicts and ensure the correct header is sent.
example.jsCopiedapp.get('/html', (req, res) => { // Set Content-Type before rendering HTML res.type('text/html'); // Render HTML content res.render('index', { title: 'Express App' }); });
Use Standard MIME Types:
Ensure you use standard MIME types when setting Content-Type to improve compatibility across different clients.
example.jsCopiedapp.get('/image', (req, res) => { // Set Content-Type for serving an image res.type('image/png'); // Stream the image to the client // (Implementation of image streaming is not shown in this example) });
🎉 Conclusion
The res.type()
method in Express.js is a valuable tool for explicitly setting the Content-Type of the response. Whether you are serving different content types, handling multiple formats, or ensuring compatibility, understanding how to use res.type()
is essential for effective web development with Express.js.
Now, armed with knowledge about the res.type()
method, go ahead and enhance your Express.js projects with precise control over Content-Type headers!
👨💻 Join our Community:
Author
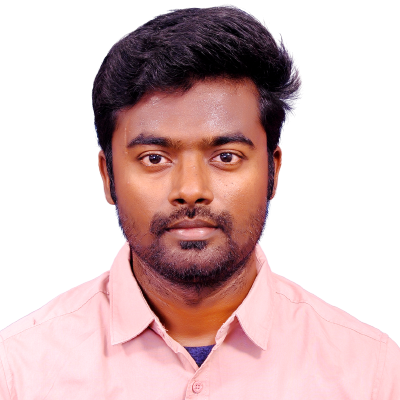
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.type() Method), please comment here. I will help you immediately.