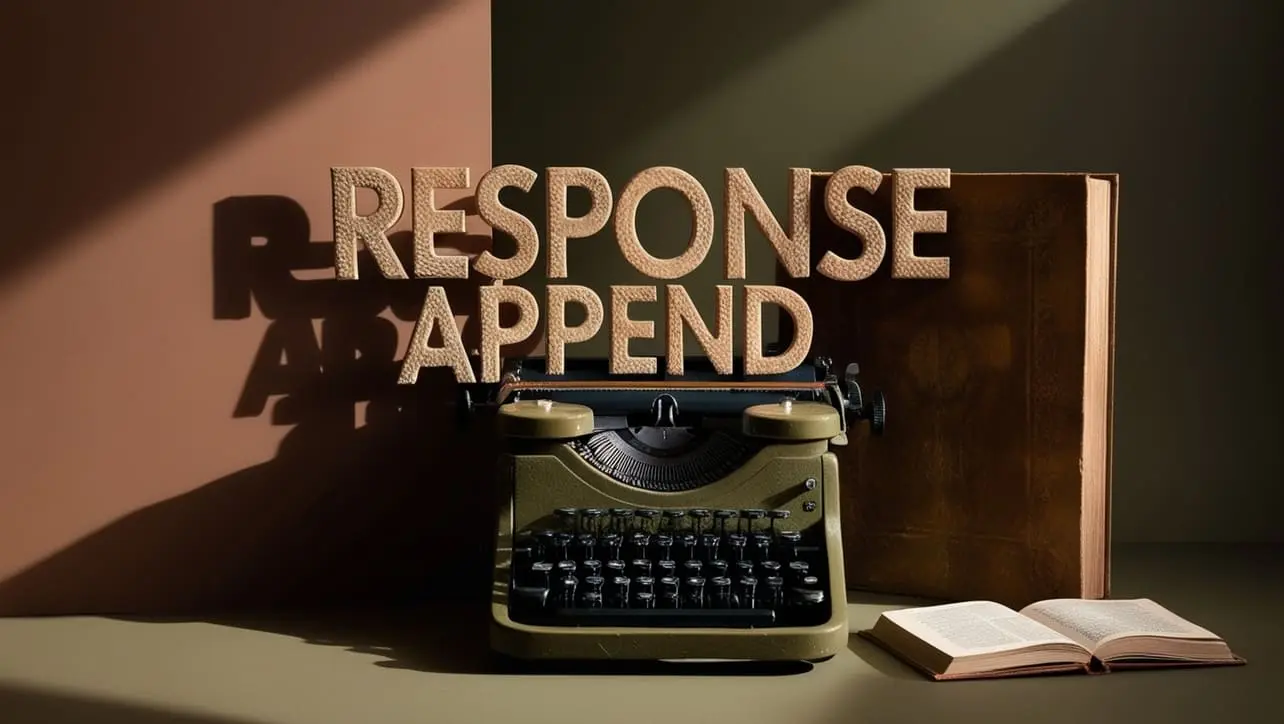
Express res.status() Method
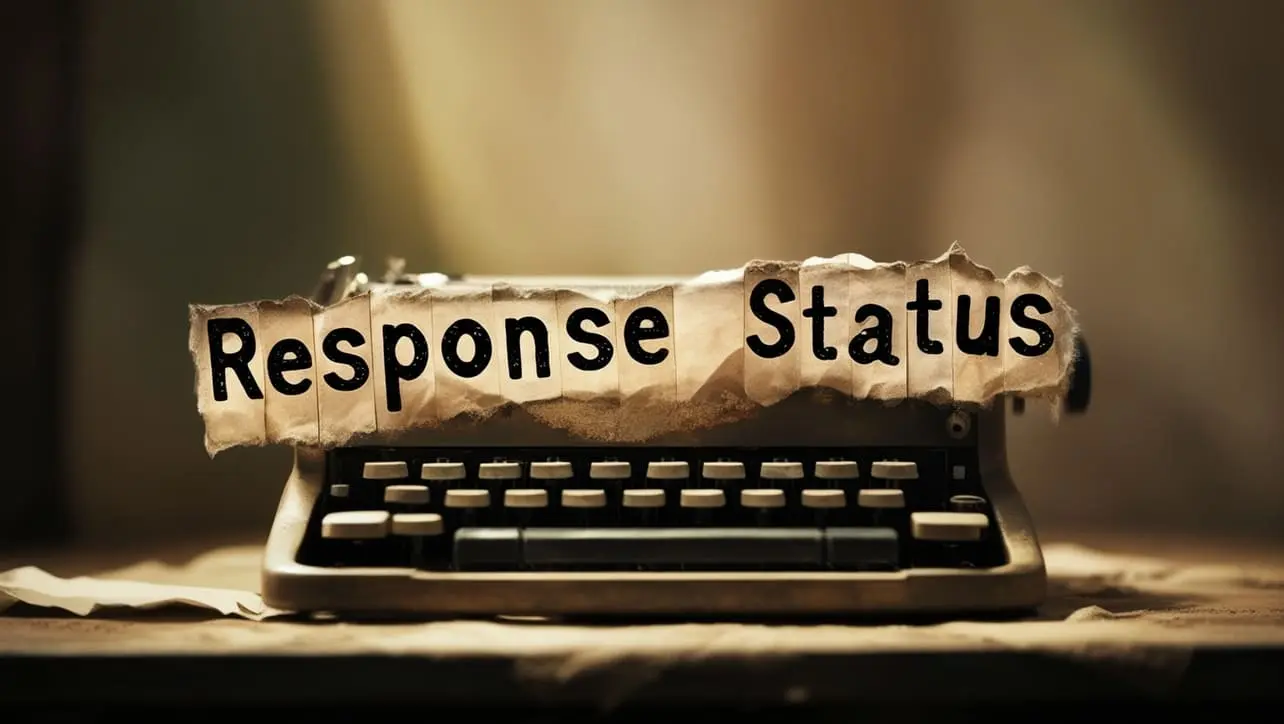
Photo Credit to CodeToFun
🙋 Introduction
In Express.js, controlling the HTTP response status code is crucial for conveying the outcome of a request to the client. The res.status()
method is a powerful tool that allows developers to explicitly set the HTTP status code for a response.
This guide will walk you through the syntax, use cases, and best practices of the res.status()
method in Express.js.
💡 Syntax
The syntax for the res.status()
method is straightforward:
res.status(statusCode)
- statusCode: The HTTP status code to be set for the response.
🚧 Setting Custom Status Codes
With res.status()
, you can easily customize the HTTP status code returned to the client. This is particularly useful when you want to provide more context about the outcome of a request.
app.get('/error', (req, res) => {
// Simulate an error scenario
const error = new Error('Internal Server Error');
res.status(500).send({ error: error.message });
});
In this example, the route /error sets a custom status code of 500 (Internal Server Error) to indicate an error condition.
📚 Use Cases
Handling Errors:
Use
res.status()
to dynamically set the appropriate status code based on the outcome of the request, such as data availability or errors.example.jsCopiedapp.get('/api/data', (req, res) => { // Check if the required data is available if (dataIsNotAvailable()) { res.status(404).send('Data Not Found'); } else { res.status(200).send('Data Found'); } });
Redirecting Users:
Set a 301 status code (Moved Permanently) to inform clients that a resource has permanently moved to a new location.
example.jsCopiedapp.get('/old-route', (req, res) => { // Redirect users to a new route res.status(301).redirect('/new-route'); });
🏆 Best Practices
Use Descriptive Status Codes:
Choose status codes that accurately represent the nature of the response. This helps clients understand the outcome without relying solely on the response body.
example.jsCopiedapp.post('/create', (req, res) => { if (creationSuccessful()) { res.status(201).send('Resource Created Successfully'); } else { res.status(400).send('Bad Request - Unable to Create Resource'); } });
Centralize Status Code Logic:
Avoid duplicating status code logic across multiple routes. Centralize the logic in utility functions or middleware to maintain consistency.
example.jsCopied// Centralized status code utility function const sendResponseWithCode = (res, statusCode, message) => { res.status(statusCode).send(message); }; // Using the utility function in a route app.get('/status', (req, res) => { sendResponseWithCode(res, 418, 'I am a teapot'); });
Utilize Redirects Appropriately:
When redirecting, use status codes like 301 (Moved Permanently) for permanent moves and 302 (Found) or 307 (Temporary Redirect) for temporary redirects.
example.jsCopiedapp.get('/old-url', (req, res) => { // Redirect users to a new URL temporarily res.status(307).redirect('/temporary-url'); });
🎉 Conclusion
The res.status()
method in Express.js provides developers with fine-grained control over the HTTP status codes returned to clients. By leveraging this method, you can enhance the clarity and context of your responses, leading to a more effective and understandable communication between your server and clients.
Now, armed with knowledge about the res.status()
method, go ahead and tailor your Express.js applications to deliver precise and informative HTTP status codes!
👨💻 Join our Community:
Author
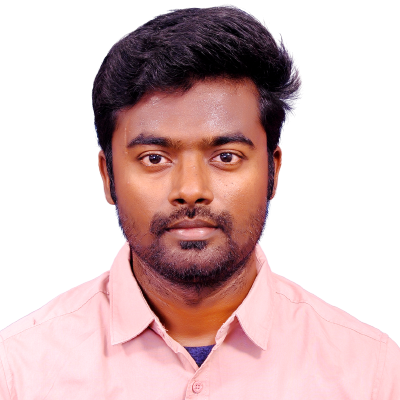
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.status() Method), please comment here. I will help you immediately.