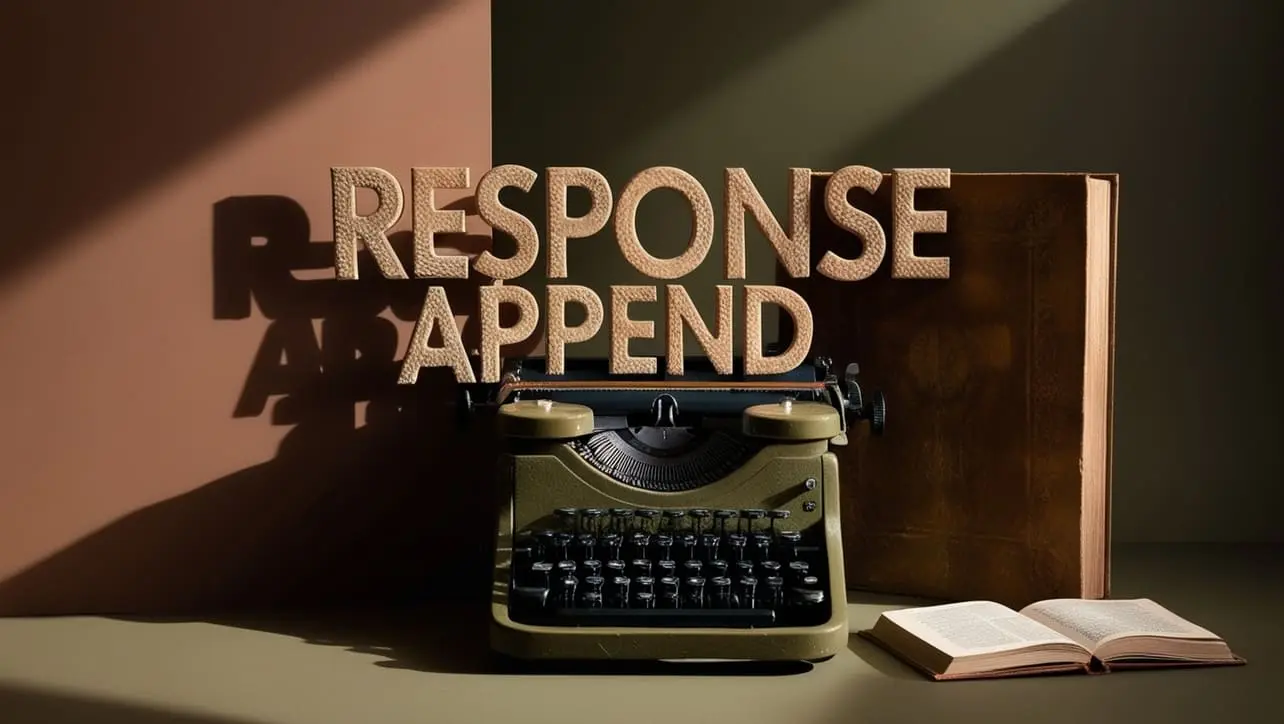
Express res.sendStatus() Method
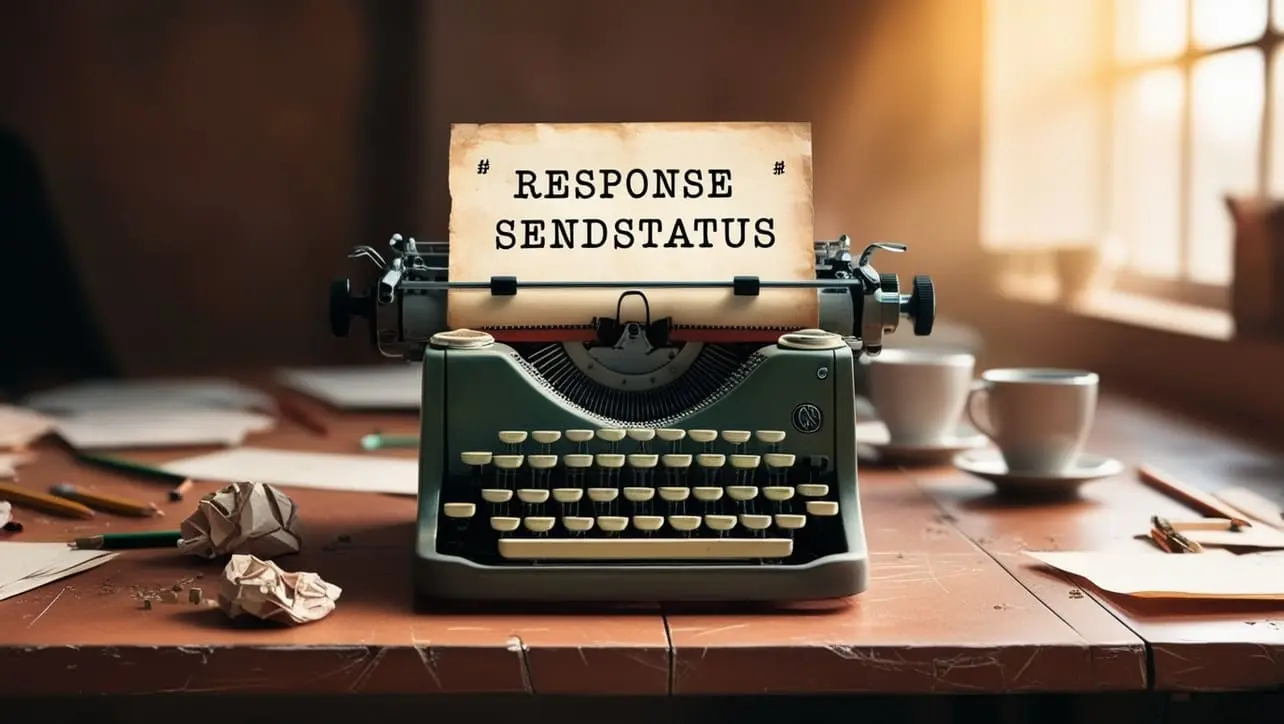
Photo Credit to CodeToFun
Introduction
When building web applications with Express.js, sending appropriate HTTP status codes is crucial for conveying the outcome of a request.
The res.sendStatus()
method simplifies this process by allowing you to send a specific status code along with an optional status message.
In this guide, we'll explore the syntax, use cases, and best practices of the res.sendStatus()
method in Express.js.
Syntax
The syntax for the res.sendStatus()
method is straightforward:
res.sendStatus(statusCode)
- statusCode: A numeric HTTP status code to be sent in the response.
How res.sendStatus() Works
res.sendStatus()
sets the response status code and sends its string representation as the response body. It's a convenient method when you want to send a minimal response with just the status code and a default status message.
app.get('/success', (req, res) => {
// Send a 200 OK status with default message "OK"
res.sendStatus(200);
});
app.get('/notfound', (req, res) => {
// Send a 404 Not Found status with default message "Not Found"
res.sendStatus(404);
});
In this example, different routes demonstrate how to use res.sendStatus()
with specific status codes.
Use Cases
Simplifying Success Responses:
Use
res.sendStatus()
to quickly send success responses without specifying a custom message.example.jsCopiedapp.post('/submit', (req, res) => { // Process form submission // If successful, send a 200 OK status res.sendStatus(200); });
Handling Errors:
Easily handle error scenarios by sending appropriate status codes with
res.sendStatus()
.example.jsCopiedapp.get('/resource/:id', (req, res) => { const resourceId = req.params.id; // Query the database for the resource // If not found, send a 404 Not Found status if (!resourceExists(resourceId)) { res.sendStatus(404); } else { // Send the resource data res.json(getResourceData(resourceId)); } });
Best Practices
Be Explicit in Code:
When using
res.sendStatus()
, be explicit about the status codes you're sending to ensure clarity in your code.example.jsCopied// Prefer this res.sendStatus(404); // Over this res.status(404).send('Not Found');
Leverage Default Messages:
Take advantage of the default status messages provided by
res.sendStatus()
to keep your responses concise.example.jsCopiedapp.post('/submit', (req, res) => { // Process form submission // If successful, send a 200 OK status with default message "OK" res.sendStatus(200); });
Conclusion
The res.sendStatus()
method in Express.js streamlines the process of sending HTTP status codes and default messages. Whether you're handling success responses or managing errors, incorporating res.sendStatus()
can lead to cleaner and more maintainable code.
Now, armed with knowledge about the res.sendStatus()
method, simplify your response handling in Express.js projects and ensure effective communication with clients.
Join our Community:
Author
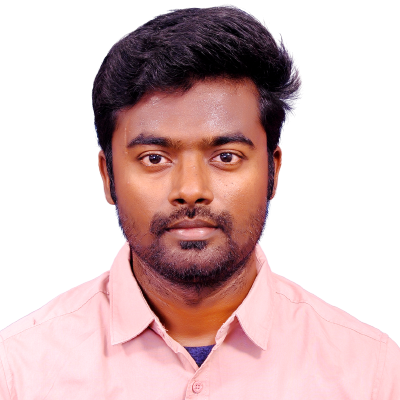
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.sendStatus() Method), please comment here. I will help you immediately.