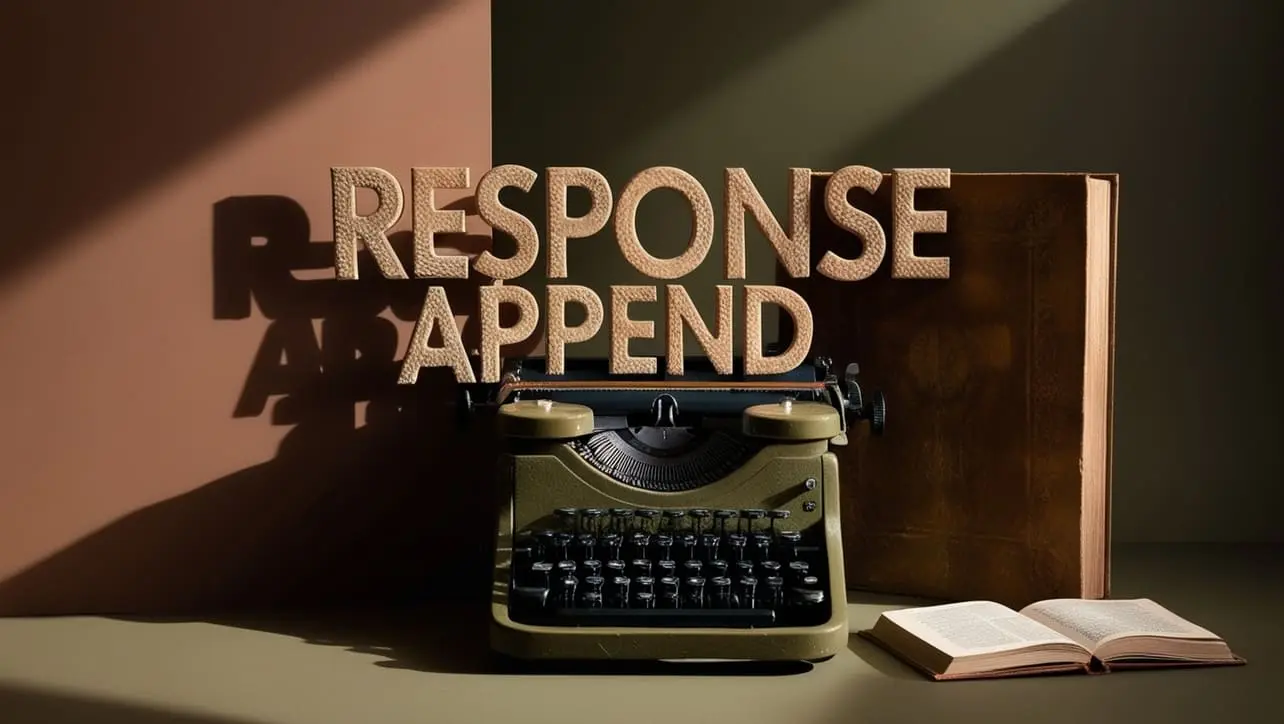
Express res.sendFile() Method
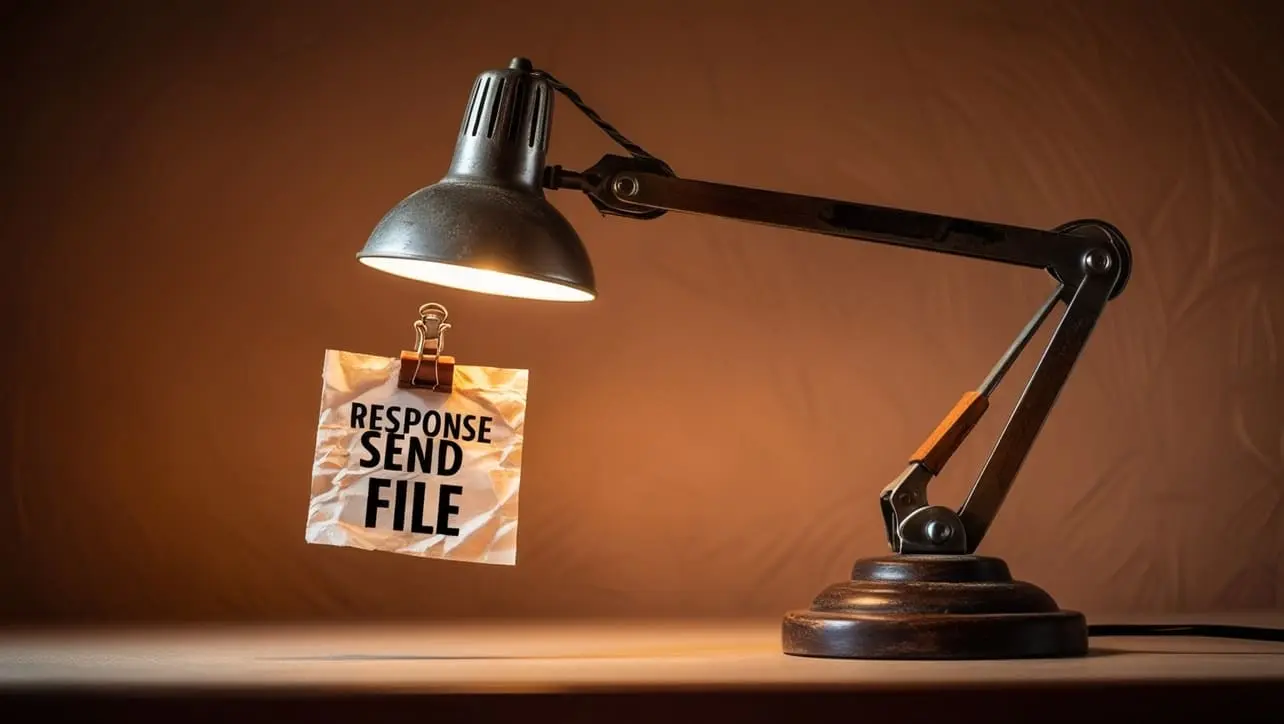
Photo Credit to CodeToFun
🙋 Introduction
In web development, serving files is a common task, whether it's static assets, images, or entire HTML documents.
Express.js simplifies this process with the res.sendFile()
method, designed to effortlessly send files as responses.
In this guide, we'll explore the syntax, use cases, and best practices of the res.sendFile()
method in Express.js.
💡 Syntax
The syntax for the res.sendFile()
method is straightforward:
res.sendFile(path [, options] [, callback])
- path: A string representing the file path or a complete file path.
- options (optional): An object with additional options for sending the file.
- callback (optional): A callback function that will be executed once the file is sent.
❓ How res.sendFile() Works
The res.sendFile()
method is used to send a file as an HTTP response to a client. It automatically sets the appropriate Content-Type header based on the file extension and provides options for additional configurations.
const express = require('express');
const app = express();
app.get('/download/:file', (req, res) => {
const fileName = req.params.file;
const filePath = `./downloads/${fileName}`;
res.sendFile(filePath, { root: __dirname });
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
In this example, a route is created to handle requests for downloading files. The res.sendFile()
method is used to send the requested file from the 'downloads' directory.
📚 Use Cases
Serving Static Files:
Use
res.sendFile()
to serve static files like HTML, CSS, and JavaScript from a specified directory.example.jsCopiedapp.use(express.static('public')); app.get('/home', (req, res) => { res.sendFile('index.html', { root: __dirname + '/public' }); });
File Download Routes:
Create routes dedicated to file downloads, using
res.sendFile()
to send files in response to client requests.example.jsCopiedapp.get('/download/:file', (req, res) => { const fileName = req.params.file; const filePath = `./downloads/${fileName}`; res.sendFile(filePath, { root: __dirname }); });
🏆 Best Practices
Security Considerations:
Ensure that file paths are properly sanitized and validated to prevent directory traversal attacks.
example.jsCopiedapp.get('/download/:file', (req, res) => { const fileName = req.params.file; // Validate file name to prevent directory traversal if (!isValidFileName(fileName)) { res.status(400).send('Invalid file name'); return; } const filePath = `./downloads/${fileName}`; res.sendFile(filePath, { root: __dirname }); });
Setting Custom Headers:
Use the options parameter to set custom headers if needed, such as Content-Disposition for controlling file download behavior.
example.jsCopiedapp.get('/download/:file', (req, res) => { const fileName = req.params.file; const filePath = `./downloads/${fileName}`; const options = { headers: { 'Content-Disposition': `attachment; filename=${fileName}` } }; res.sendFile(filePath, options); });
🎉 Conclusion
The res.sendFile()
method in Express.js simplifies the process of serving files, making it an essential tool for handling various file-related scenarios. Whether you're serving static assets or providing file downloads, understanding how to use res.sendFile()
will enhance your Express.js applications.
Now, equipped with knowledge about the res.sendFile()
method, go ahead and streamline your file-serving tasks in Express.js!
👨💻 Join our Community:
Author
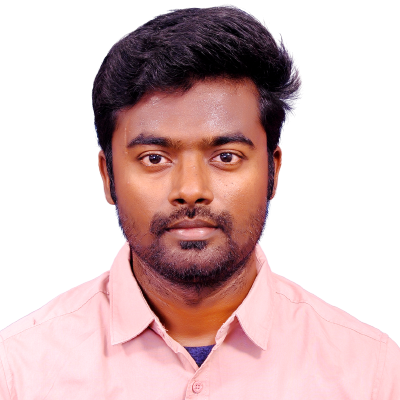
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.sendFile() Method), please comment here. I will help you immediately.