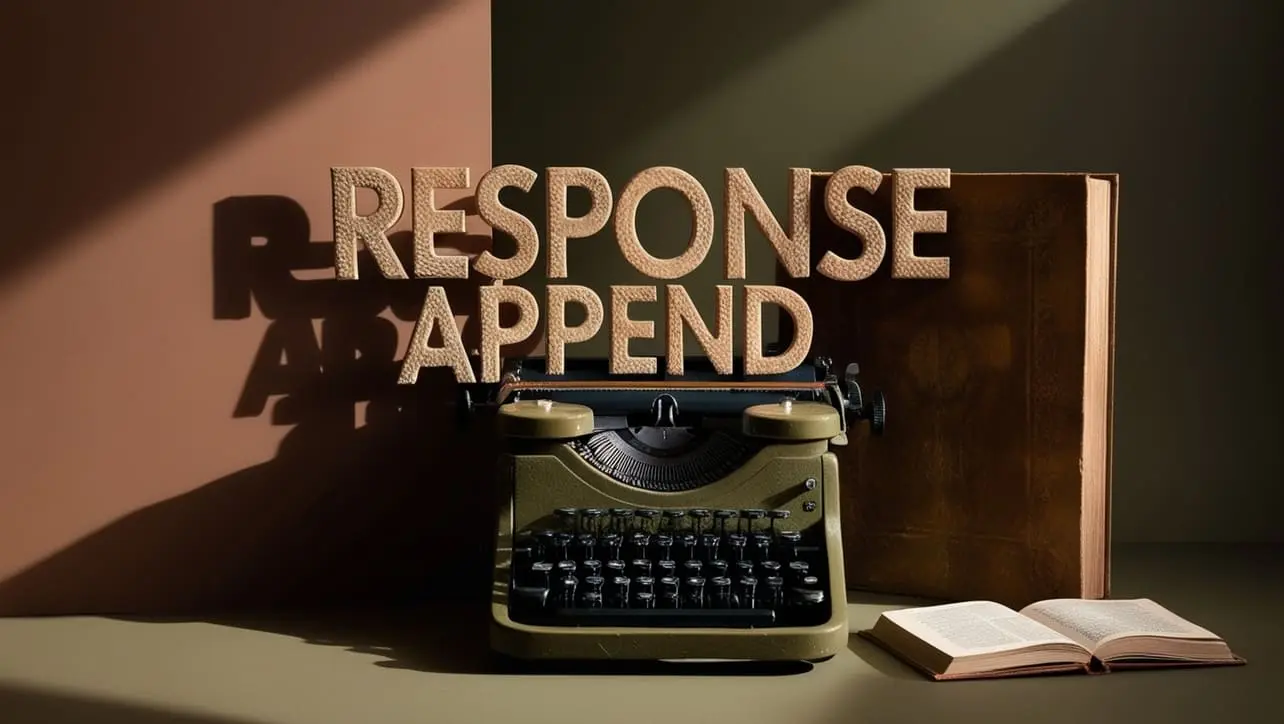
Express res.render() Method
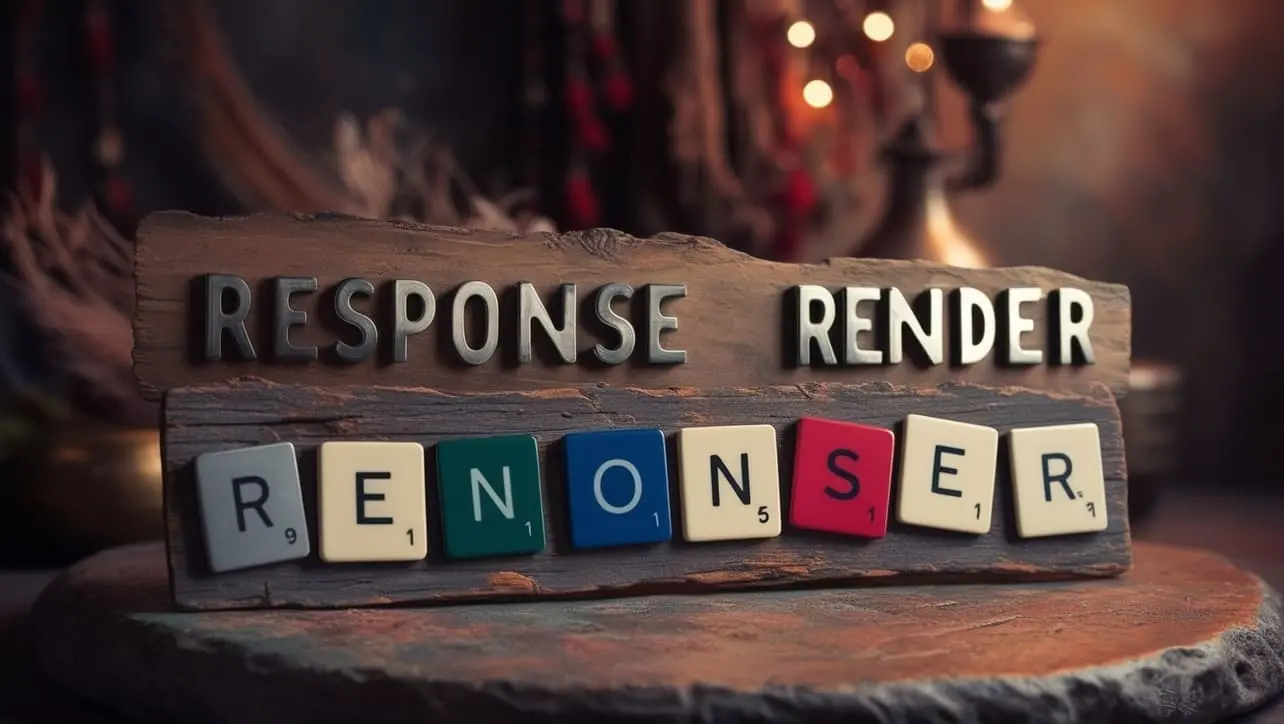
Photo Credit to CodeToFun
Introduction
Express.js, a powerful Node.js web application framework, provides various methods for rendering dynamic content. One such method is res.render()
, which allows you to render views and templates.
In this guide, we'll explore the syntax, use cases, and best practices of the res.render()
method to enhance your Express.js application's rendering capabilities.
Syntax
The syntax for the res.render()
method is straightforward:
res.render(view, [locals], callback)
- view: The name of the view or template to render.
- locals: An optional object containing local variables that will be passed to the view.
- callback: An optional callback function to handle the rendering result.
How res.render() Works
The res.render()
method is used to render HTML or other types of views using a template engine. Express looks for the view in the views directory by default, but you can configure the views directory and template engine as needed.
// Set the view engine to use EJS
app.set('view engine', 'ejs');
app.get('/home', (req, res) => {
// Render the 'home' view with the provided locals
res.render('home', { title: 'Express App', user: req.user });
});
In this example, the res.render()
method renders the 'home' view using the EJS template engine and passes local variables like the title and user to the view.
Use Cases
Dynamic Page Rendering:
Use
res.render()
to dynamically render user profiles based on the provided username.example.jsCopiedapp.get('/profile/:username', (req, res) => { const username = req.params.username; // Fetch user data from the database const userData = getUserData(username); // Render the 'profile' view with user data res.render('profile', { username, userData }); });
Error Pages:
Utilize
res.render()
to display custom error pages when a 404 or 500 error occurs.example.jsCopied// Middleware for handling 404 errors app.use((req, res) => { res.status(404).render('404', { url: req.originalUrl }); }); // Middleware for handling 500 errors app.use((err, req, res, next) => { console.error(err.stack); res.status(500).render('500'); });
Best Practices
Organize Views in a Directory:
Organize your views in a separate directory to keep your project structure clean and maintainable.
example.jsCopiedproject |-- views | |-- home.ejs | |-- profile.ejs | |-- 404.ejs | |-- 500.ejs |-- app.js
Use Template Engines:
Choose a template engine (like EJS, Handlebars, or Pug) based on your preferences and project requirements.
plaintextCopied// Set the view engine to use Handlebars app.set('view engine', 'hbs');
Leverage Layouts and Partials:
Enhance code reusability by using layouts and partials provided by your chosen template engine.
handlebarsCopied<!-- views/layout.hbs --> <html> <head> <title>{{ title }}</title> </head> <body> {{{ body }}} </body> </html> <!-- views/home.hbs --> {{!< layout}} <h1>{{ title }}</h1>
Conclusion
The res.render()
method in Express.js is a powerful tool for rendering dynamic content and views in your web applications. Whether you're rendering user profiles, error pages, or other dynamic content, understanding how to leverage res.render()
will enhance your Express.js development experience.
Now, armed with knowledge about the res.render()
method, go ahead and elevate the rendering capabilities of your Express.js projects!
Join our Community:
Author
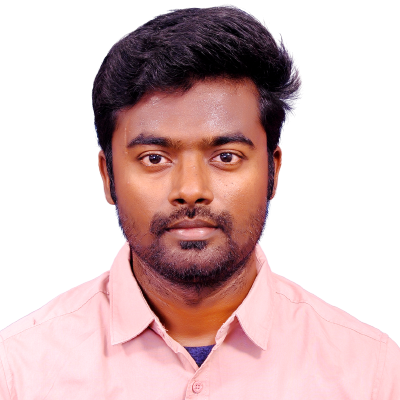
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.render() Method), please comment here. I will help you immediately.