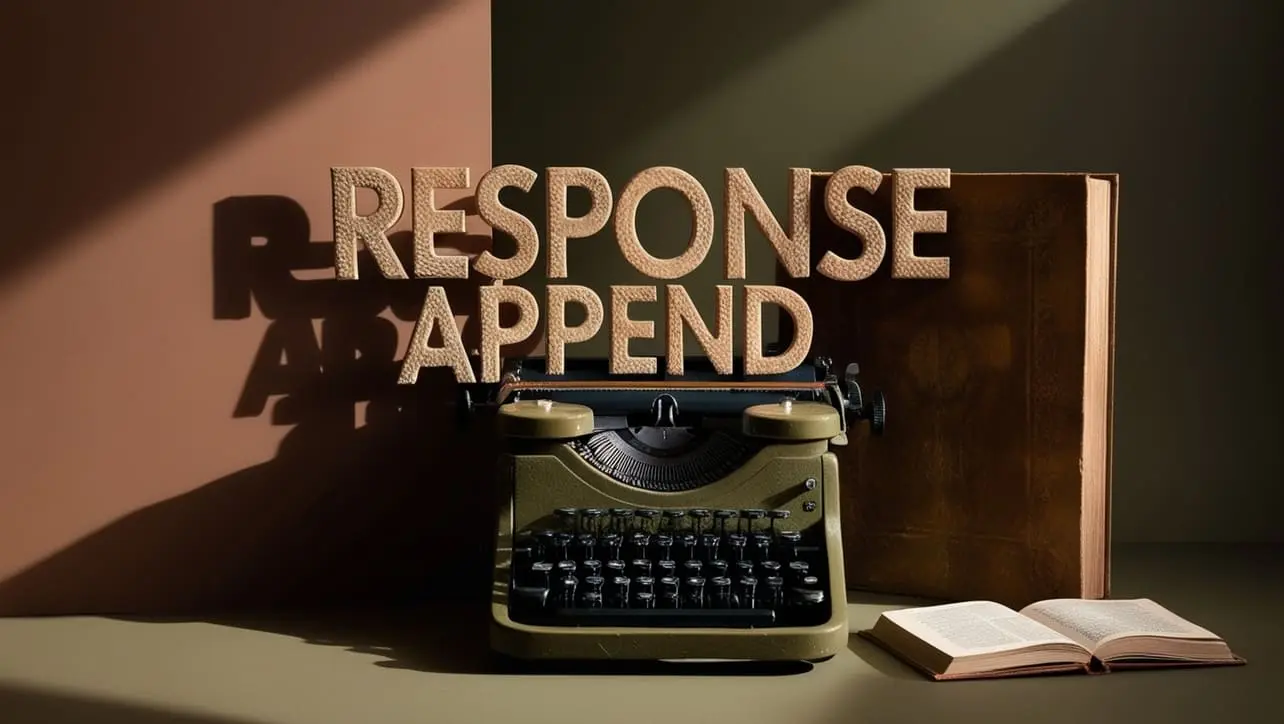
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.location() Method
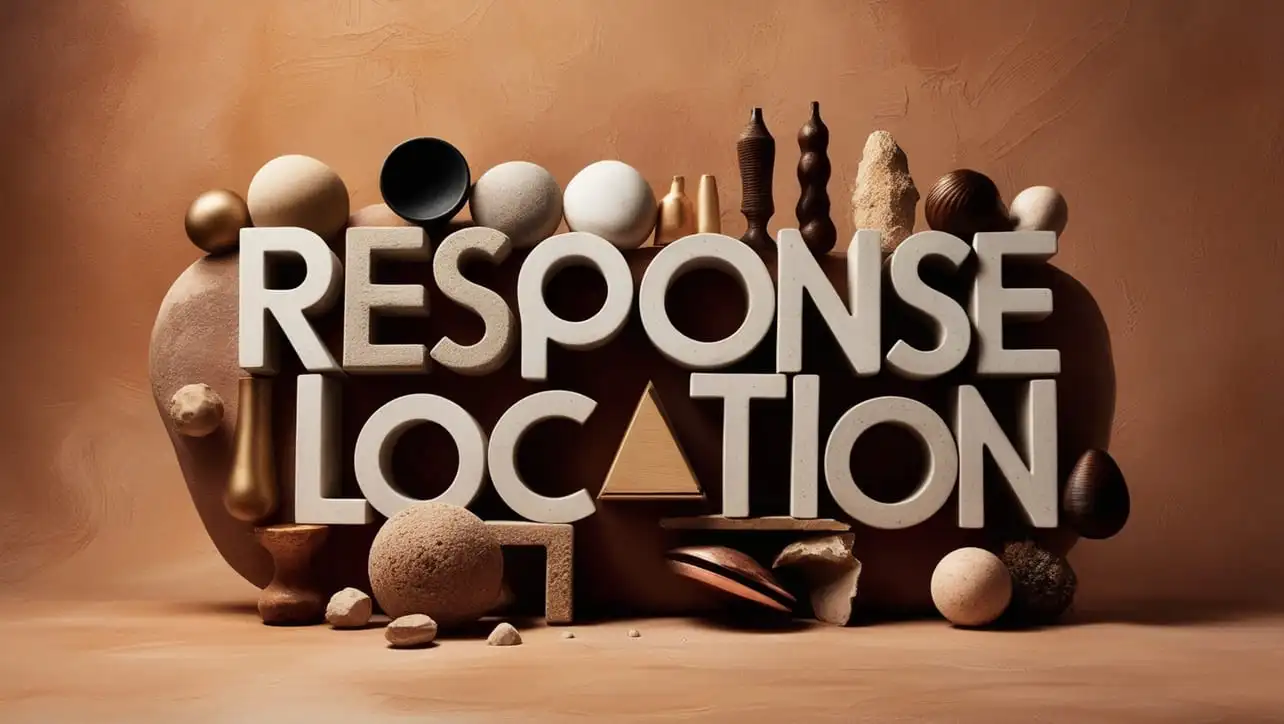
Photo Credit to CodeToFun
🙋 Introduction
In the world of web development, managing redirects is a common task. Express.js, a robust web application framework for Node.js, provides various methods to handle HTTP responses effectively.
This guide explores the res.location()
method, which is specifically designed for setting the Location header in HTTP responses. Join us as we delve into the syntax, use cases, and best practices of this powerful Express.js feature.
💡 Syntax
The syntax for the res.location()
method is straightforward:
res.location(path)
- path: A string representing the URL or path to set as the value of the Location header.
🏷️ Setting the Location Header
The res.location()
method in Express.js is primarily used for indicating the URL to which a client should be redirected.
app.get('/redirect', (req, res) => {
// Set the Location header for redirect
res.location('https://example.com/new-location');
// Send a 302 Found status code and redirect to the specified location
res.status(302).send('Redirecting to the new location');
});
In this example, the res.location()
method is used to set the Location header, and then a 302 status code is sent to initiate the redirection.
📚 Use Cases
Redirecting After Form Submission:
Use
res.location()
after processing a form submission to redirect users to a success page.example.jsCopiedapp.post('/submit-form', (req, res) => { // Process form submission // Set the location header to redirect to a success page res.location('/success'); res.status(302).send('Form submitted successfully'); });
Handling Resource Moves:
When a resource is permanently moved, use
res.location()
with a 301 status code to inform clients of the new location.example.jsCopiedapp.get('/old-resource', (req, res) => { // Set the location header to indicate the new location of the resource res.location('/new-resource'); res.status(301).send('Resource has moved permanently'); });
🏆 Best Practices
Combine with Status Codes:
Combine
res.location()
with appropriate HTTP status codes to convey the nature of the redirect, whether it's temporary (302) or permanent (301).example.jsCopiedapp.get('/temp-redirect', (req, res) => { // Set the Location header for temporary redirect res.location('https://example.com/new-location'); // Send a 302 Found status code and redirect to the specified location res.status(302).send('Temporary redirect to the new location'); });
Include Informative Messages:
Include informative messages in your response to give users context about the redirection.
example.jsCopiedapp.get('/moved-permanently', (req, res) => { // Set the Location header for permanent redirect res.location('https://example.com/new-location'); // Send a 301 Moved Permanently status code and provide an informative message res.status(301).send('This resource has moved permanently. Please update your bookmarks.'); });
🎉 Conclusion
The res.location()
method in Express.js simplifies the process of setting the Location header for redirects. Whether you're redirecting users after form submissions or handling resource moves, understanding how to leverage res.location()
enhances your ability to manage HTTP responses effectively.
Now equipped with knowledge about the res.location()
method, go ahead and streamline your redirection logic in your Express.js applications!
👨💻 Join our Community:
Author
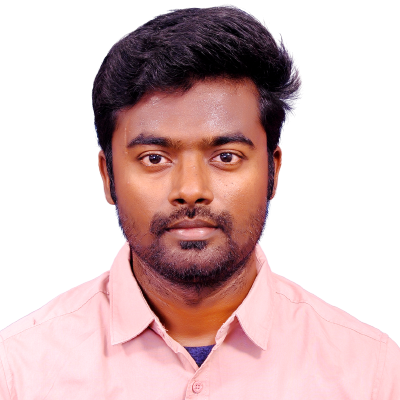
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.location() Method), please comment here. I will help you immediately.