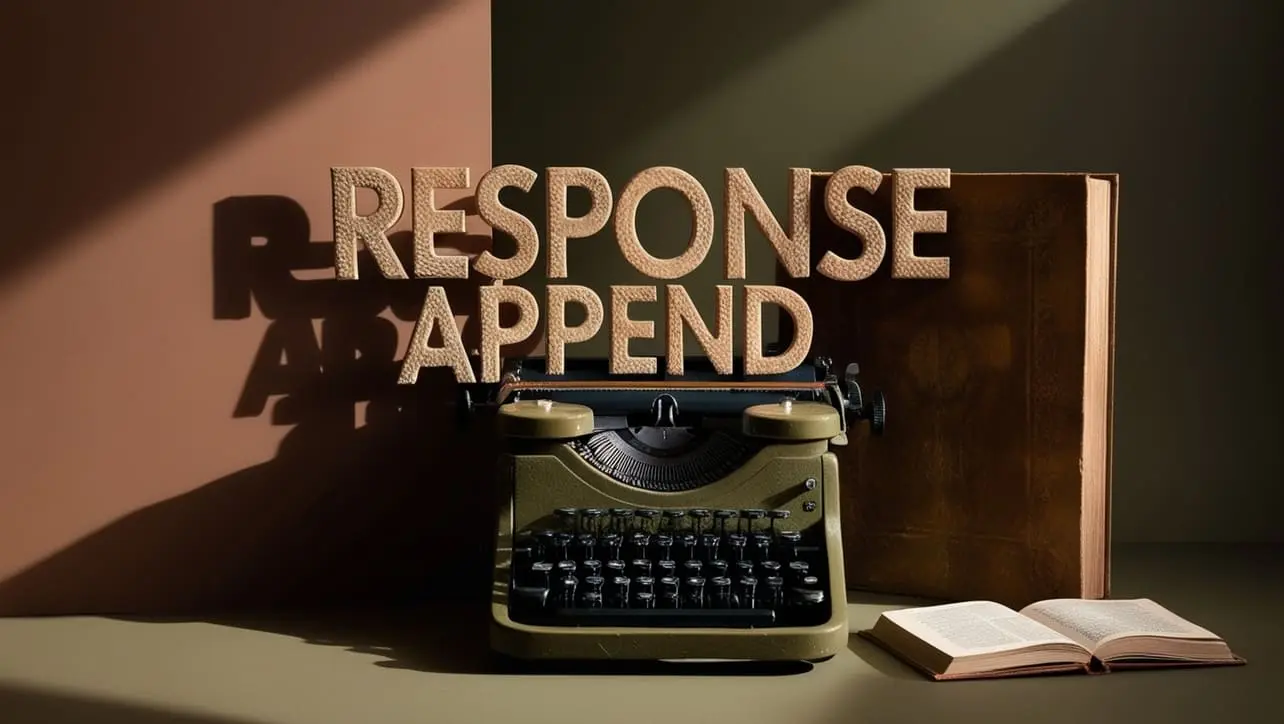
Express res.json() Method
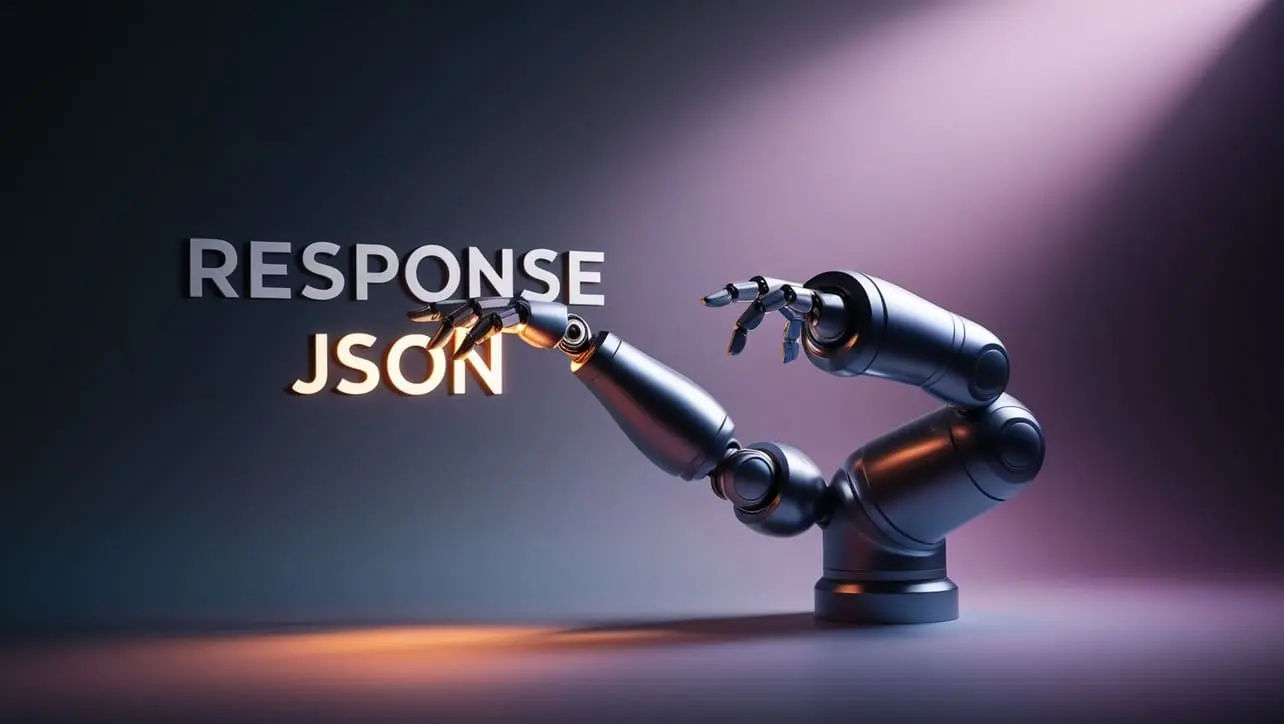
Photo Credit to CodeToFun
🙋 Introduction
In the world of web development, efficient handling and formatting of responses are crucial.
Express.js, a powerful web application framework for Node.js, provides the res.json()
method for sending JSON responses to clients.
Join us as we explore the syntax, usage, and practical examples of this handy Express.js feature.
💡 Syntax
The syntax for the res.json()
method is straightforward:
res.json([status] [, body])
- status (optional): The HTTP response status code. If not specified, it defaults to 200.
- body (optional): The JSON data to be sent in the response.
❓ How res.json() Works
The res.json()
method simplifies the process of sending JSON responses to clients. It automatically sets the Content-Type header to application/json and converts the provided JSON object into a string for transmission.
app.get('/api/user', (req, res) => {
const user = {
id: 1,
username: 'john_doe',
email: 'john@example.com'
};
// Sending a JSON response with status 200
res.json(user);
});
In this example, the res.json()
method is used to send a JSON response containing user information.
📚 Use Cases
API Responses:
Use
res.json()
to send JSON responses when building APIs, providing structured data to clients.example.jsCopiedapp.get('/api/posts', (req, res) => { const posts = [ { id: 1, title: 'Introduction to Express.js' }, { id: 2, title: 'Handling JSON in Node.js' }, // ... more posts ]; // Sending a JSON response with status 200 res.json(posts); });
Error Responses:
Leverage
res.json()
for sending error responses in a consistent JSON format.example.jsCopiedapp.get('/api/error', (req, res) => { // Simulating an error response const error = { status: 404, message: 'Resource not found' }; // Sending a JSON error response with status 404 res.status(404).json(error); });
🏆 Best Practices
Set Appropriate Status Codes:
Specify the appropriate HTTP status code based on the nature of the response. For success, use 200, and for errors, choose relevant codes like 404 or 500.
example.jsCopied// Sending a JSON success response with status 201 (Created) res.status(201).json({ message: 'Resource created successfully' });
Handle Asynchronous Operations:
Ensure that the
res.json()
method is called only once in asynchronous operations to prevent headers from being sent multiple times.example.jsCopiedapp.get('/api/async', async (req, res) => { // Simulating an asynchronous operation const data = await fetchData(); // Sending a JSON response with the fetched data res.json(data); });
🎉 Conclusion
The res.json()
method in Express.js simplifies the process of sending JSON responses, making it an essential tool for building modern web applications. Whether you're creating APIs or handling error responses, understanding how to use res.json()
effectively enhances the communication between your server and clients.
Now, equipped with knowledge about the res.json()
method, go ahead and streamline your JSON responses in your Express.js projects!
👨💻 Join our Community:
Author
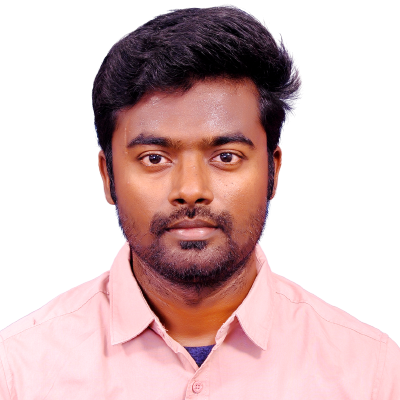
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.json() Method), please comment here. I will help you immediately.