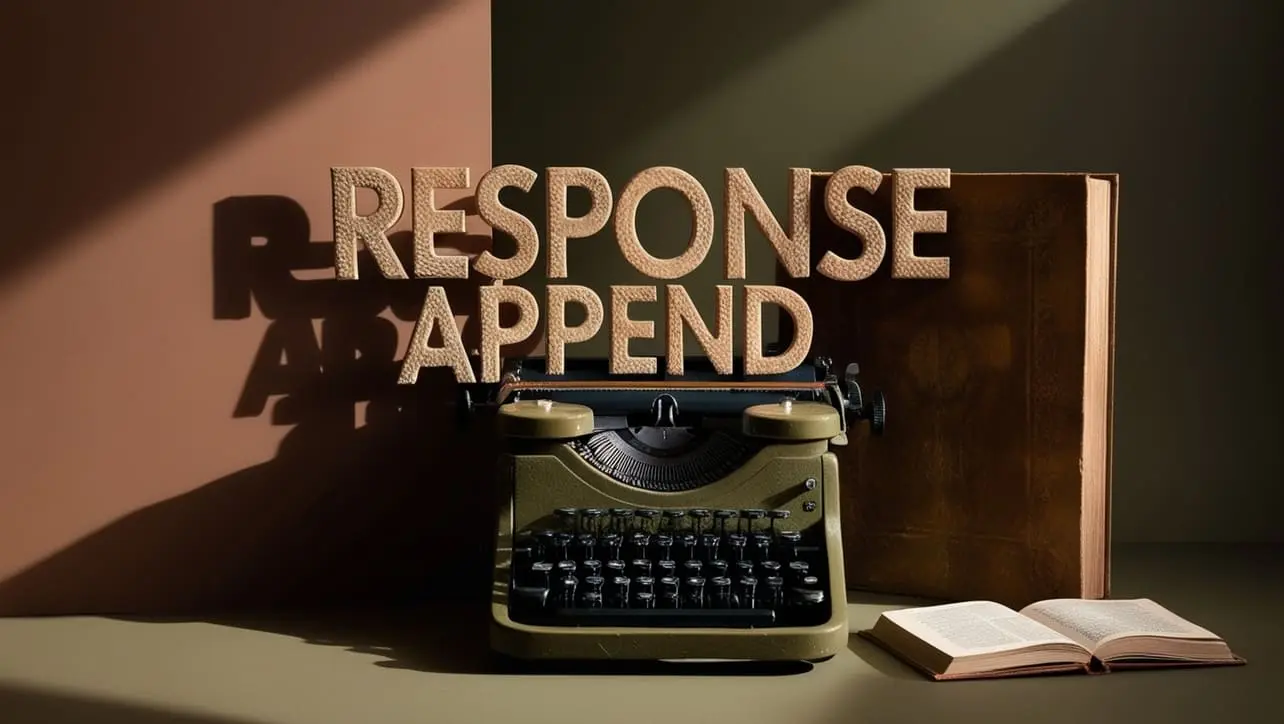
Express res.clearCookie() Method
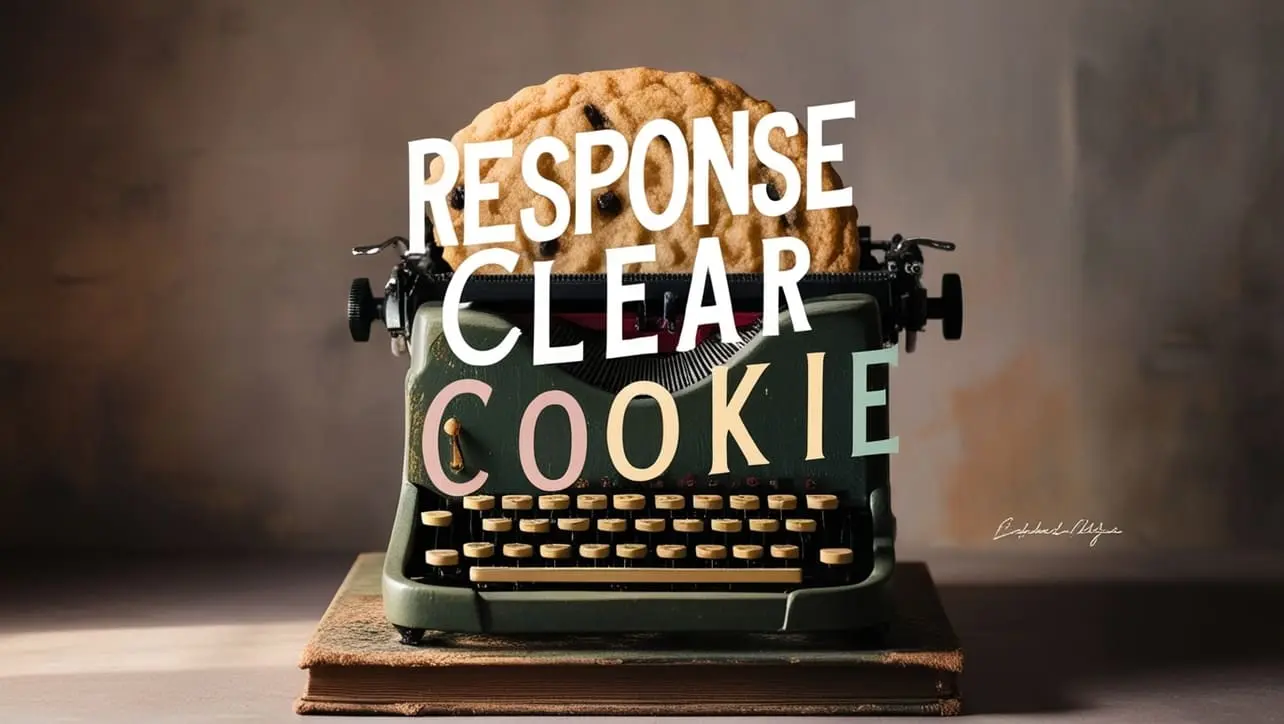
Photo Credit to CodeToFun
🙋 Introduction
Cookies play a crucial role in web development, enabling the storage of user-specific information on the client side. In Express.js, the res.clearCookie()
method provides a way to efficiently manage cookies.
This guide explores the syntax, use cases, and best practices for utilizing res.clearCookie()
in your Express.js applications.
💡 Syntax
The syntax for the res.clearCookie()
method is straightforward:
res.clearCookie(name, [options])
- name: The name of the cookie to be cleared.
- options: An optional object containing additional parameters like the cookie's domain, path, etc.
❓ How res.clearCookie() Works
The res.clearCookie()
method is particularly useful when you need to remove a cookie previously set in the client's browser.
app.get('/logout', (req, res) => {
// Clear the 'userToken' cookie upon logout
res.clearCookie('userToken');
res.send('Logged out successfully');
});
In this example, the /logout route uses res.clearCookie()
to delete the 'userToken' cookie when a user logs out.
📚 Use Cases
User Logout:
Use
res.clearCookie()
in scenarios where you want to clear multiple cookies, providing a clean logout experience for users.example.jsCopiedapp.get('/logout', (req, res) => { // Clear multiple cookies upon logout res.clearCookie('userToken'); res.clearCookie('userData'); res.send('Logged out successfully'); });
Session Expiry:
Leverage
res.clearCookie()
to handle session expirations by removing the session cookie.example.jsCopiedapp.get('/expire-session', (req, res) => { // Clear the session cookie upon session expiration res.clearCookie('sessionID'); res.send('Session expired'); });
🏆 Best Practices
Specify Cookie Options:
When using
res.clearCookie()
, consider specifying the same options used when setting the cookie to ensure proper removal.example.jsCopiedapp.get('/logout', (req, res) => { // Clear the 'userToken' cookie with specified options res.clearCookie('userToken', { path: '/', domain: '.example.com' }); res.send('Logged out successfully'); });
Use Secure and HttpOnly Flags:
For sensitive cookies, set the secure and httpOnly flags when clearing cookies to enhance security.
example.jsCopiedapp.get('/logout', (req, res) => { // Clear the 'userToken' cookie with secure and httpOnly flags res.clearCookie('userToken', { secure: true, httpOnly: true }); res.send('Logged out successfully'); });
🎉 Conclusion
The res.clearCookie()
method in Express.js is a powerful tool for managing cookies. Whether you're handling user logouts or expiring sessions, understanding how to use res.clearCookie()
ensures a seamless and secure cookie management experience.
Now equipped with knowledge about the res.clearCookie()
method, you can enhance your Express.js applications by efficiently managing cookies.
👨💻 Join our Community:
Author
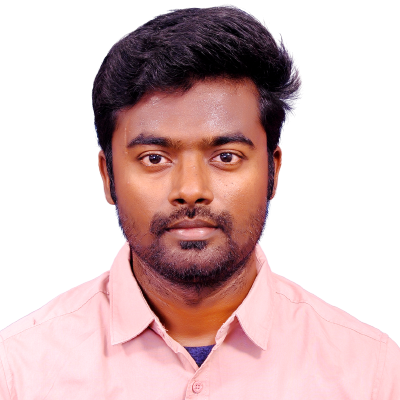
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.clearCookie() Method), please comment here. I will help you immediately.