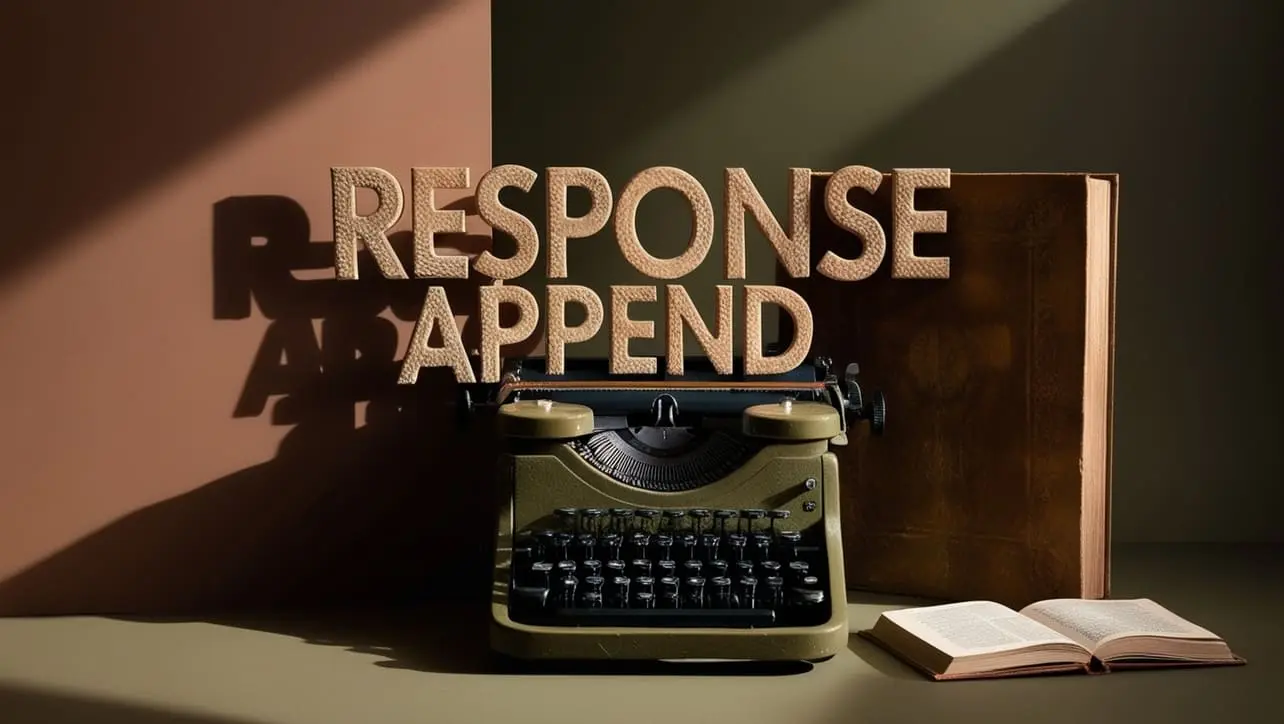
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
- express Response
Properties
Methods
- express Router
Express res.app Property
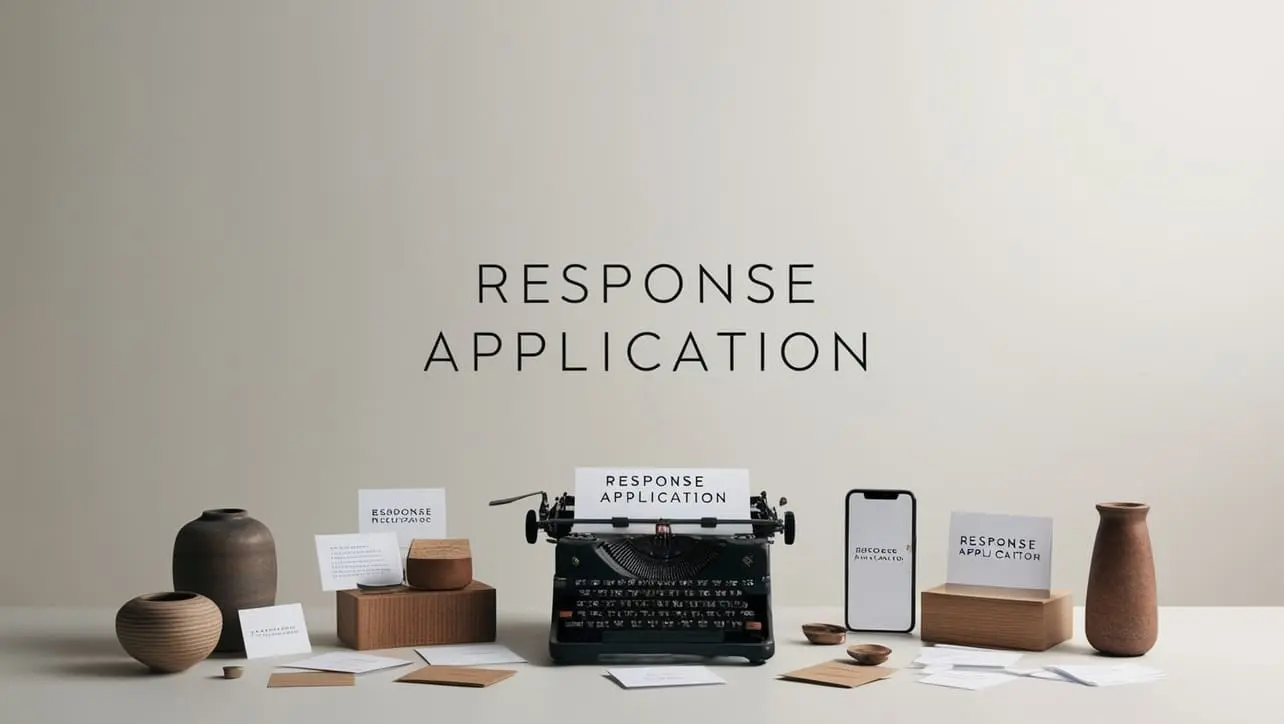
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a widely-used Node.js web application framework, equips developers with powerful tools to handle HTTP requests and responses effectively. One interesting property of the response object (res) is res.app
, providing access to properties and methods related to the Express application.
In this guide, we'll explore the syntax, use cases, and best practices associated with the res.app
properties.
💡 Syntax
Accessing res.app
is simple and can be done within a route handler or middleware:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
// Accessing res.app properties
const appName = res.app.get('appName');
res.send(`Welcome to ${appName}`);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
❓ How res.app Works
The res.app
property provides a reference to the Express application instance. It allows you to access various properties and methods associated with the application, enhancing flexibility and control within route handlers and middleware.
// Setting a custom property on the app instance
const express = require('express');
const app = express();
app.set('appName', 'My Express App');
app.get('/', (req, res) => {
// Accessing the app name using res.app
const appName = res.app.get('appName');
res.send(`Welcome to ${appName}`);
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, the application instance sets a custom property (appName), and res.app
is used to retrieve and utilize this property within a route handler.
📚 Use Cases
Application Configuration:
Store and retrieve global configuration settings using
res.app
properties.application-configuration.jsCopied// Setting and accessing global configuration settings const express = require('express'); const app = express(); app.set('appName', 'My Express App'); app.set('version', '1.0.0'); app.get('/', (req, res) => { // Accessing application configuration using res.app const appName = res.app.get('appName'); const appVersion = res.app.get('version'); res.send(`Welcome to ${appName} (v${appVersion})`); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
Centralized Data Storage:
Utilize
res.app
for centralized data storage, sharing information across route handlers.centralized-data-storage.jsCopied// Centralized data storage using res.app const express = require('express'); const app = express(); // Store shared data in the app instance app.locals.sharedData = { key: 'value' }; app.get('/', (req, res) => { // Accessing shared data using res.app const data = res.app.locals.sharedData; res.json(data); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
🏆 Best Practices
Limit Property Usage:
While
res.app
provides powerful capabilities, limit its usage to scenarios where accessing application-level properties is necessary.limit-property-usage.jsCopied// Good practice: Use res.app judiciously const appName = res.app.get('appName');
Leverage Application-Wide Settings:
Take advantage of
res.app
for accessing and modifying application-wide settings.application-wide-settings.jsCopied// Setting and accessing application-wide settings app.set('appName', 'My Express App'); const appName = res.app.get('appName');
🎉 Conclusion
The res.app
properties in Express.js provide a versatile means of accessing the underlying application instance. By understanding its syntax, use cases, and best practices, you can leverage res.app
to enhance the flexibility and functionality of your Express.js applications.
Now, equipped with knowledge about res.app
, explore its capabilities and integrate it judiciously into your Express.js projects!
👨💻 Join our Community:
Author
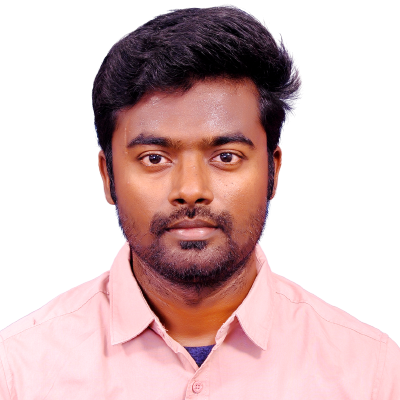
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express res.app Property), please comment here. I will help you immediately.