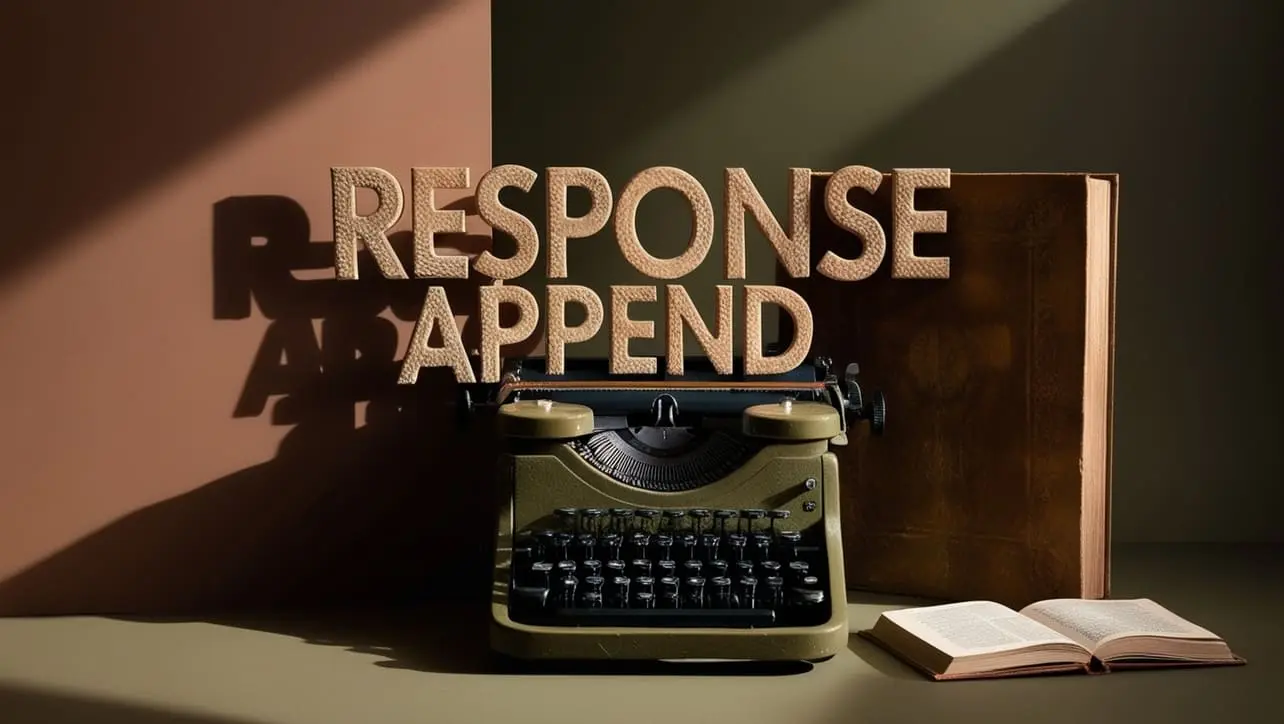
Express.js Response
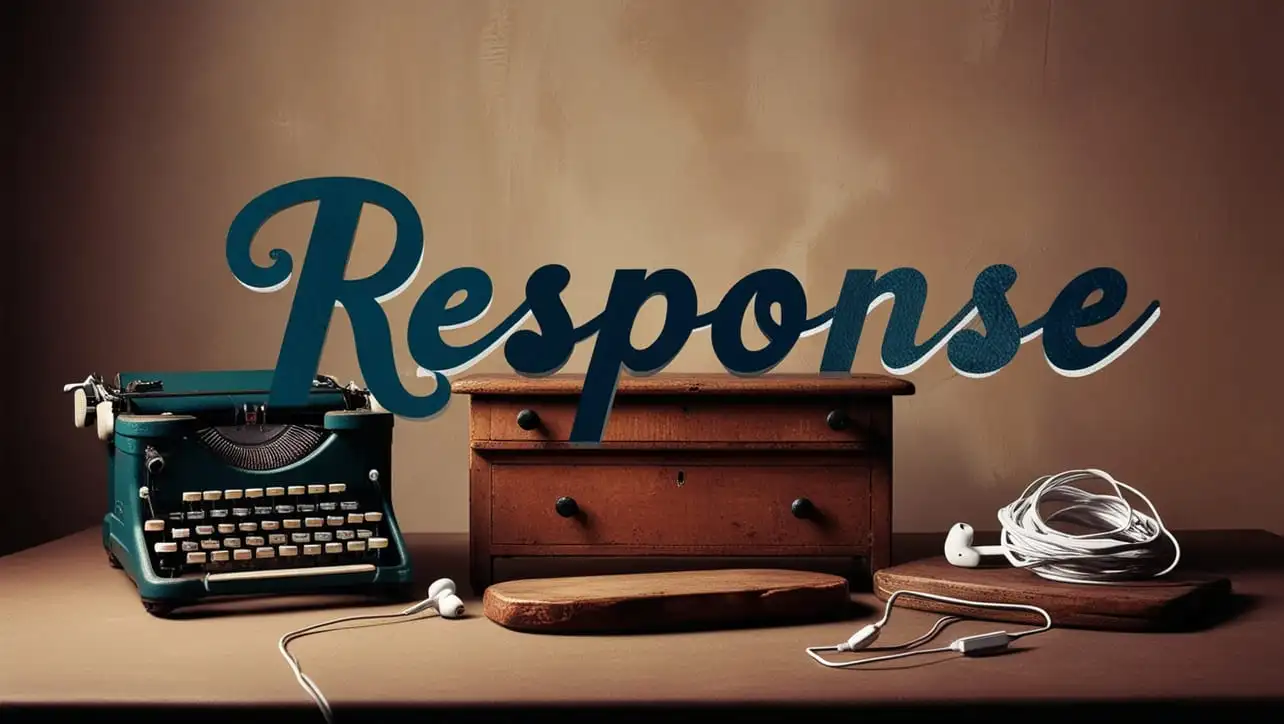
Photo Credit to CodeToFun
Introduction
In the realm of web development with Express.js, understanding how to craft and manage responses is essential.
The res
object, short for "response," is at the core of shaping what your server sends back to clients.
This page serves as a comprehensive guide to the res
object, exploring its methods, capabilities, and best practices.
What is the Response Object?
In Express.js, the res
object represents the HTTP response that an Express app sends when it receives an HTTP request. It provides a set of methods and properties to manipulate and control the content, headers, and status codes of the response.
Initializing the res Object
Unlike the app object, the res
object is not explicitly created; it is automatically available in the callback functions handling routes or middleware. As a developer, you interact with it to construct and send the desired response.
Anatomy of the res Object
Sending Basic Responses:
The res.send() method is a versatile tool for sending responses in various formats, such as HTML, plain text, JSON, or even status codes.
sending-basic-responses.jsCopiedapp.get('/hello', (req, res) => { res.send('Hello, Express!'); }); app.get('/json', (req, res) => { res.send({ message: 'Express is awesome!' }); });
Sending JSON Responses:
The res.json() method specifically formats and sends JSON responses, setting the appropriate headers automatically.
sending-json-responses.jsCopiedapp.get('/api/data', (req, res) => { const data = { name: 'John Doe', age: 25, occupation: 'Developer' }; res.json(data); });
Setting Status Codes:
The res.status() method allows you to set the HTTP status code for the response, indicating success, failure, or redirection.
setting-status-codes.jsCopiedapp.get('/not-found', (req, res) => { res.status(404).send('Not Found'); });
Key Methods and Functions
Manipulating Response Headers:
Customizing response headers is a common requirement. The res.setHeader() method allows you to set custom headers for the response.
manipulating-response-headers.jsCopiedapp.get('/custom-headers', (req, res) => { res.setHeader('X-Custom-Header', 'Hello, Custom Header!'); res.send('Check the headers of this response.'); });
Error Handling in Responses:
Effectively handling errors is crucial for a seamless user experience. Utilize the res.status() method in conjunction with appropriate HTTP status codes to communicate errors gracefully.
error-handling-in-responses.jsCopiedapp.get('/error', (req, res) => { // Simulate an error const error = new Error('Something went wrong!'); res.status(500).send(error.message); });
Advanced Features
Streaming Responses:
The res.write() method allows you to stream responses, making it efficient to handle large datasets and deliver content progressively to clients.
streaming-responses.htmlCopiedapp.get('/stream-data', (req, res) => { res.write('This '); setTimeout(() => res.write('is '), 1000); setTimeout(() => res.write('streamed '), 2000); setTimeout(() => res.end('data.'), 3000); });
Redirecting Requests:
The res.redirect() method simplifies the process of redirecting clients to a different URL.
redirecting-requests.htmlCopiedapp.get('/old-url', (req, res) => { res.redirect('/new-url'); });
Example
Let's combine some of the discussed concepts in a simple example:
app.get('/example', (req, res) => {
// Sending a JSON response with a custom status code and header
res.status(200).setHeader('X-Custom-Header', 'Example Header').json({ message: 'Express.js example' });
});
This example showcases setting the status code, custom header, and sending a JSON response.
Conclusion
Mastering the res
object is fundamental to controlling server responses in Express.js. This overview has explored its various methods and functions, empowering you to shape and deliver responses efficiently.
Join our Community:
Author
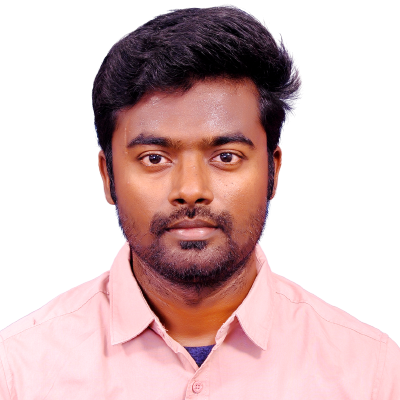
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express.js Response), please comment here. I will help you immediately.