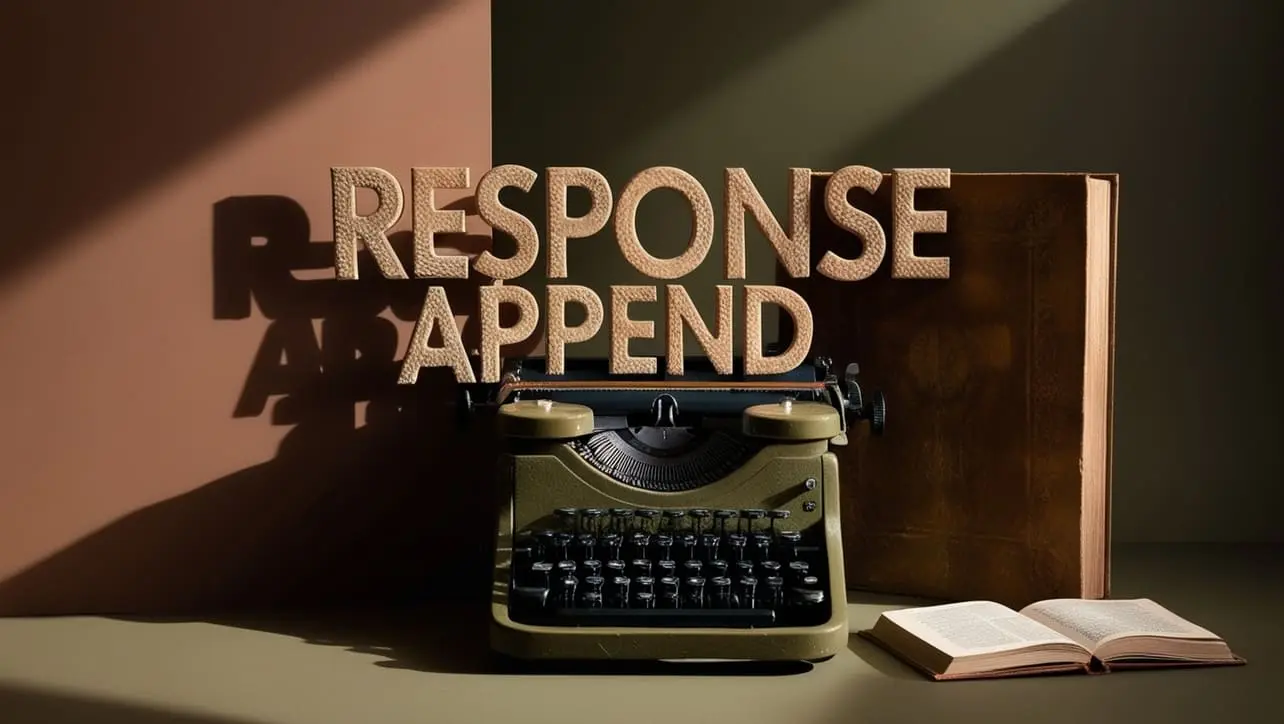
Express req.route Property
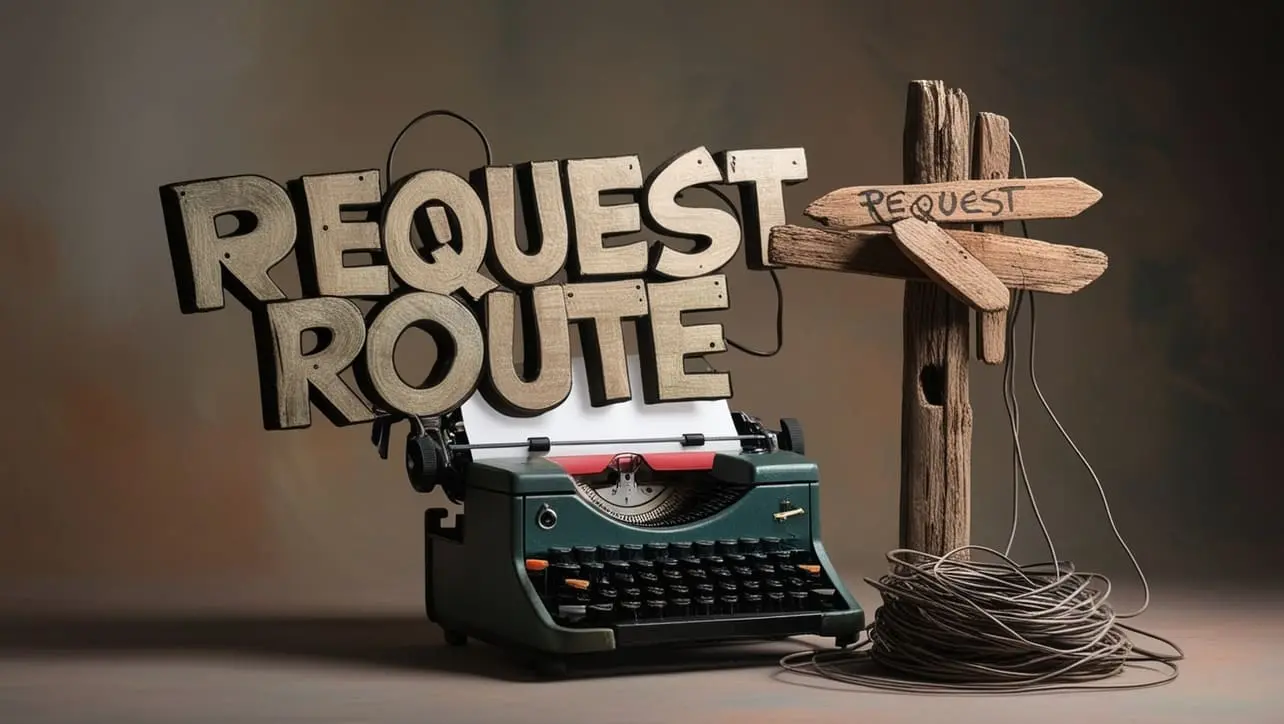
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a powerful web application framework for Node.js, provides developers with various features to handle HTTP requests. One interesting property available within route handler functions is req.route
.
In this guide, we'll explore the req.route
properties, which provide information about the currently matched route during request processing.
💡 Syntax
The syntax for accessing req.route
properties within route handler functions is as follows:
console.log(req.route.path); // Accessing the path property
console.log(req.route.methods); // Accessing the methods property
❓ How req.route Works
The req.route
object in Express.js contains information about the route that matched the current request. It provides details such as the route's path, HTTP methods, and other relevant information. This can be particularly useful when you want to perform different actions based on the characteristics of the matched route.
// Define a route with multiple HTTP methods
app.route('/books')
.get((req, res) => {
console.log(req.route.path); // Output: /books
console.log(req.route.methods); // Output: { get: true }
res.send('Get books');
})
.post((req, res) => {
console.log(req.route.path); // Output: /books
console.log(req.route.methods); // Output: { post: true }
res.send('Add a new book');
});
In this example, the req.route
object is used within route handler functions to log information about the matched route, including the path and HTTP methods.
🛠️ req.route Properties
req.route.path:
The path property of
req.route
returns the path pattern of the currently matched route.example.jsCopiedapp.get('/books/:id', (req, res) => { console.log(req.route.path); // Output: /books/:id res.send('Get book by ID'); });
req.route.methods:
The methods property of
req.route
returns an object indicating which HTTP methods are supported by the currently matched route.example.jsCopiedapp.route('/books') .get((req, res) => { console.log(req.route.methods); // Output: { get: true } res.send('Get books'); }) .post((req, res) => { console.log(req.route.methods); // Output: { post: true } res.send('Add a new book'); });
📚 Use Cases
Logging and Analytics:
Leverage
req.route
for logging route information, aiding in analytics or debugging.example.jsCopiedapp.use((req, res, next) => { console.log(`Route matched: ${req.route.path}, Method: ${Object.keys(req.route.methods)[0]}`); next(); });
Dynamic Route Handling:
Use
req.route
to dynamically handle different routes based on the matched path.example.jsCopiedapp.get('/:category/:id', (req, res) => { const category = req.params.category; const id = req.params.id; if (category === 'books') { // Logic for handling book routes } else if (category === 'movies') { // Logic for handling movie routes } res.send(`Handling dynamic route for ${category} with ID ${id}`); });
🏆 Best Practices
Check for req.route Existence:
Before accessing properties of
req.route
, ensure that a route has been matched. It may be undefined for middleware functions that are not associated with a specific route.example.jsCopiedapp.use((req, res, next) => { if (req.route) { console.log(`Route matched: ${req.route.path}, Method: ${Object.keys(req.route.methods)[0]}`); } next(); });
🎉 Conclusion
Understanding the req.route
properties in Express.js provides valuable insights into the currently matched route during request processing. By leveraging req.route
, you can enhance your route handling logic, perform dynamic actions, and gain valuable information about your application's routing behavior.
Now, with a better understanding of req.route
, go ahead and explore how it can enhance your Express.js projects!
👨💻 Join our Community:
Author
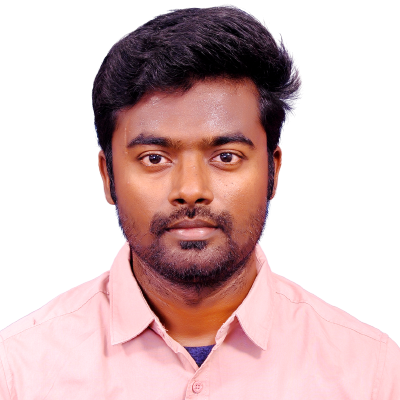
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.route Property), please comment here. I will help you immediately.