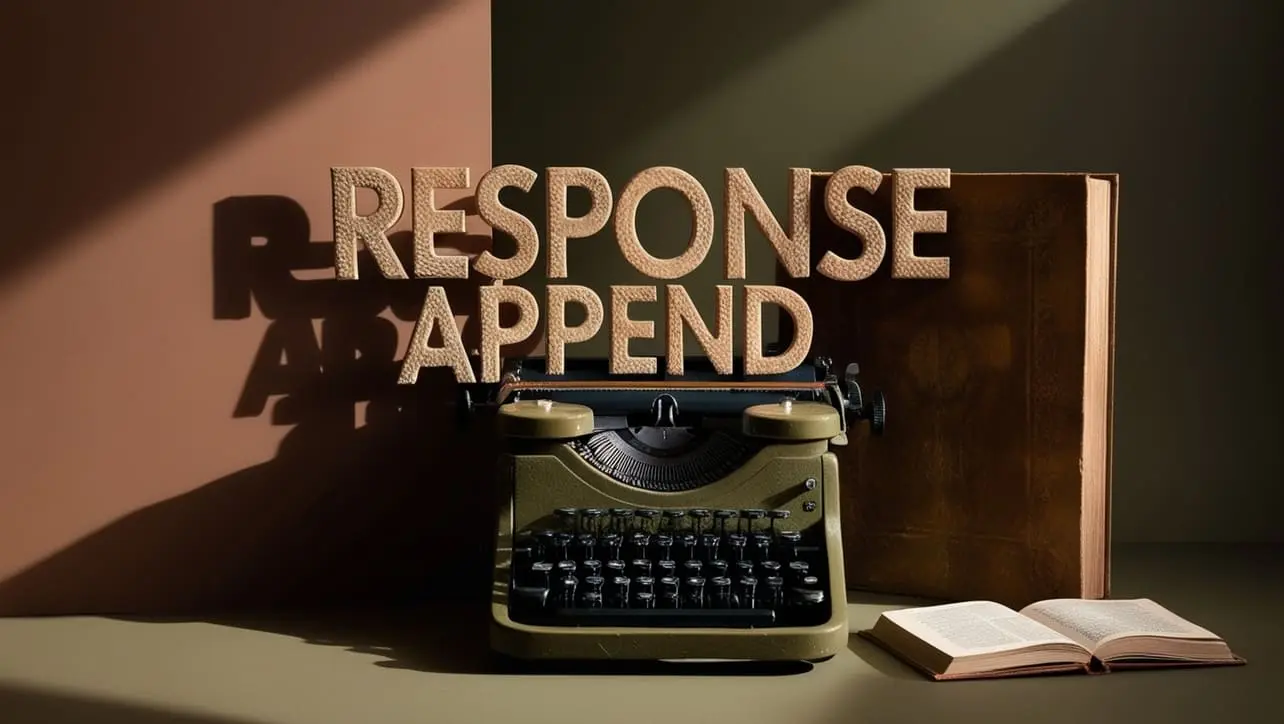
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.path Property
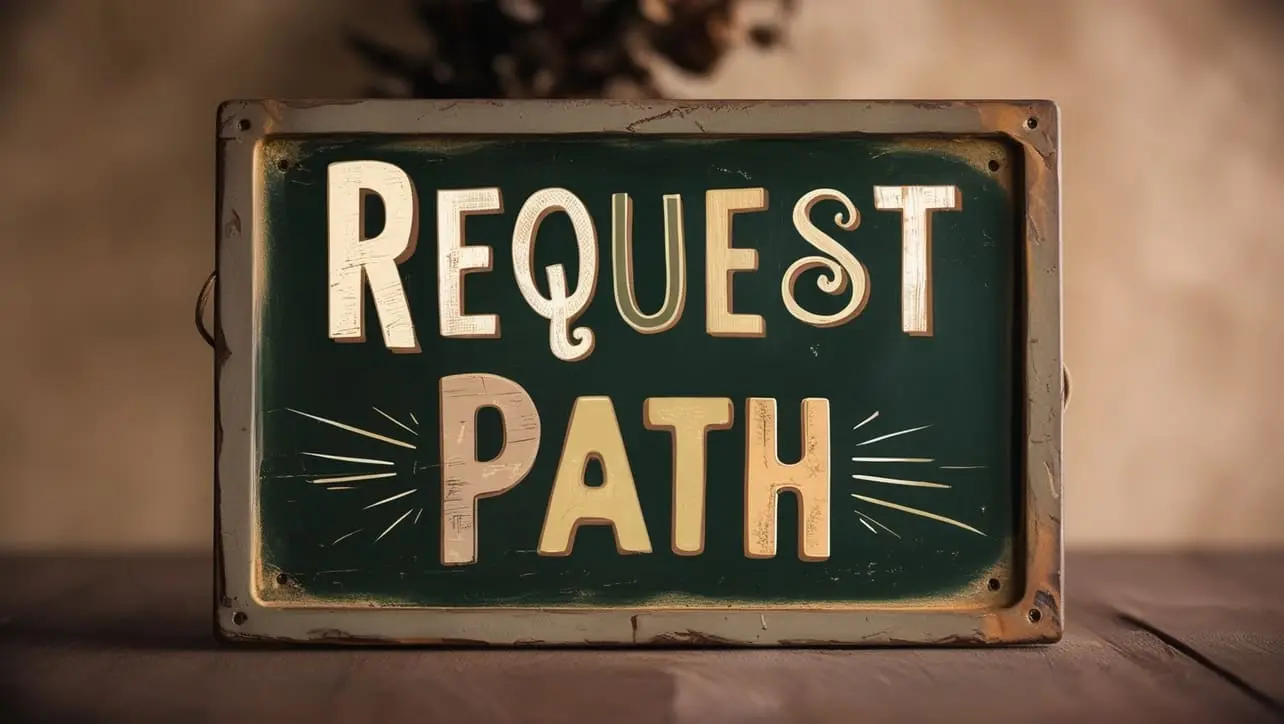
Photo Credit to CodeToFun
🙋 Introduction
In Express.js, handling routes is fundamental to building web applications. The req.path
property provides a convenient way to access the current path requested by the client.
This guide explores the syntax, usage, and practical examples of req.path
in Express.js.
💡 Syntax
The syntax for using the req.path
property is straightforward:
const currentPath = req.path;
- req: The request object provided by Express.
- path: The property that holds the current path of the request.
❓ How req.path Works
The req.path
property in Express.js returns the path portion of the requested URL. This is particularly useful when you need to conditionally handle requests based on the route or perform actions specific to certain paths within your application.
app.get('/about', (req, res) => {
const currentPath = req.path;
res.send(`You are on the ${currentPath} page.`);
});
In this example, the req.path
property is used to dynamically include the current path in the response.
📚 Use Cases
Dynamic Routing:
Leverage
req.path
to dynamically handle routes and customize responses based on the current path.example.jsCopiedapp.get('/products/:productId', (req, res) => { const productId = req.params.productId; const currentPath = req.path; // Perform actions based on the current path or product ID // ... res.send(`Product ${productId} details at ${currentPath}`); });
Navigation Highlighting:
Use
req.path
to determine the active link in your navigation, allowing you to highlight the current page dynamically.example.jsCopiedconst navLinks = [ { path: '/', label: 'Home' }, { path: '/about', label: 'About' }, { path: '/contact', label: 'Contact' }, // ... ]; app.use((req, res, next) => { // Find the active link based on the current path const activeLink = navLinks.find(link => link.path === req.path); // Set the active status for rendering navigation res.locals.activeLink = activeLink; next(); });
🏆 Best Practices
Use with Dynamic Routes:
Pair
req.path
with dynamic routes to create flexible and dynamic route handling within your Express.js application.example.jsCopiedapp.get('/blog/:postId', (req, res) => { const postId = req.params.postId; const currentPath = req.path; // Perform actions based on the current path or post ID // ... res.send(`Blog post ${postId} at ${currentPath}`); });
Avoid Hardcoding Paths:
Avoid hardcoding paths in your application logic. Instead, use
req.path
dynamically to adapt to changes in your route structure.example.jsCopied// Bad practice: Hardcoding paths app.get('/static-page', (req, res) => { const currentPath = req.path; res.send(`You are on the ${currentPath} page.`); });
🎉 Conclusion
The req.path
property in Express.js is a valuable tool for navigating routes and dynamically handling requests. By understanding its usage and best practices, you can enhance your Express.js applications with more flexible and responsive route handling.
Now, with a grasp of req.path
, navigate your routes efficiently in your Express.js projects!
👨💻 Join our Community:
Author
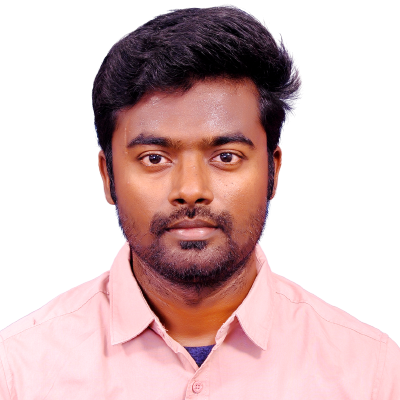
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.path Property), please comment here. I will help you immediately.