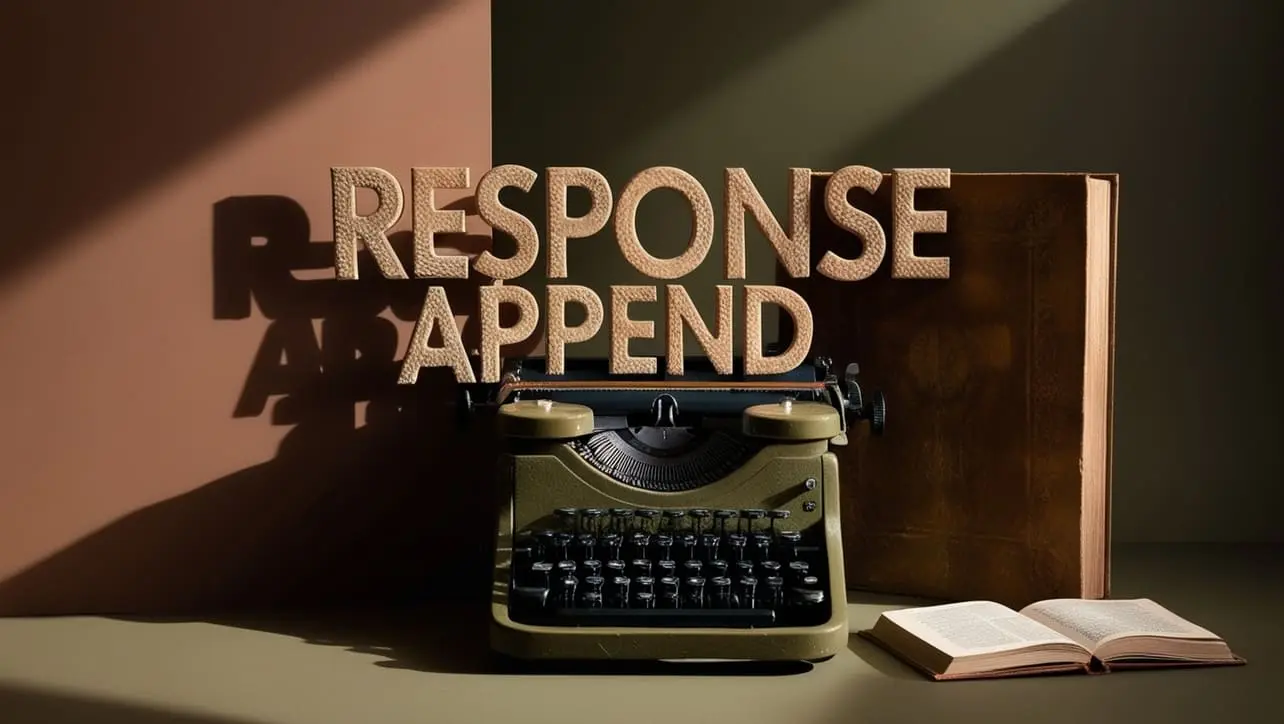
Express req.method Property
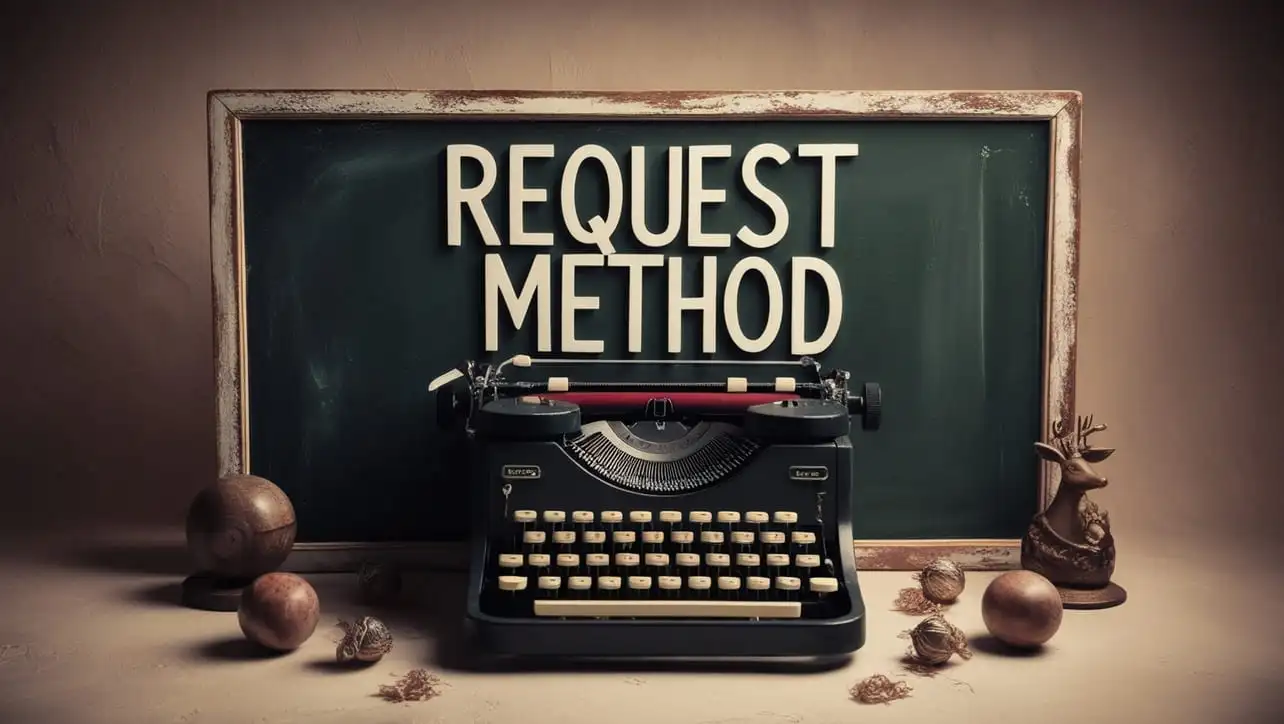
Photo Credit to CodeToFun
🙋 Introduction
In the world of web development, understanding the HTTP methods used in requests is crucial for building effective and secure applications.
Express.js, a powerful web application framework for Node.js, provides the req.method
property, allowing developers to access the HTTP request method.
In this guide, we'll explore the syntax, usage, and practical examples of req.method
in Express.js.
💡 Syntax
Accessing the HTTP request method using req.method
is straightforward:
const method = req.method;
- req: xyz.
- method: xyz.
❓ How req.method Works
The req.method
property provides insight into the HTTP method used in the incoming request. This information is valuable when you need to tailor your server's behavior based on the type of request, whether it's a GET, POST, PUT, DELETE, or another HTTP method.
app.get('/example', (req, res) => {
const requestMethod = req.method;
res.send(`Received a ${requestMethod} request at /example`);
});
In this example, the req.method
property is used to determine the HTTP method of the incoming request and respond accordingly.
📚 Use Cases
Route Handling Based on Method:
Use
req.method
to conditionally handle different HTTP methods for a specific route.example.jsCopiedapp.all('/api/data', (req, res) => { if (req.method === 'GET') { // Handle GET request res.send('Fetching data'); } else if (req.method === 'POST') { // Handle POST request res.send('Submitting data'); } else { // Handle other methods res.status(405).send('Method Not Allowed'); } });
Logging HTTP Methods:
Leverage
req.method
for logging purposes, gaining insights into the types of requests your server is receiving.example.jsCopiedapp.use((req, res, next) => { console.log(`Received a ${req.method} request at ${req.path}`); next(); });
🏆 Best Practices
Use Conditional Statements:
Employ conditional statements to check the value of
req.method
and execute different logic based on the HTTP method.example.jsCopiedif (req.method === 'PUT') { // Handle PUT request } else if (req.method === 'DELETE') { // Handle DELETE request }
Be Mindful of Security:
Consider security implications when using
req.method
. Ensure that sensitive operations are appropriately restricted to specific HTTP methods.example.jsCopiedapp.post('/delete-account', (req, res) => { // Ensure this route is only accessible via POST for security reasons // Handle account deletion logic });
🎉 Conclusion
The req.method
property in Express.js provides a valuable tool for developers to determine the HTTP method of incoming requests. By incorporating req.method
into your route handling and middleware, you can create more dynamic and secure Express.js applications.
Now, equipped with knowledge about req.method
, enhance your Express.js projects by tailoring your server's behavior to different HTTP request methods!
👨💻 Join our Community:
Author
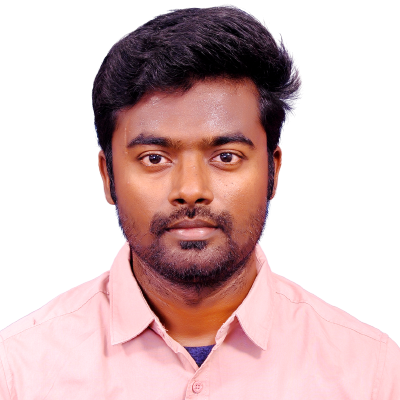
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.method Property), please comment here. I will help you immediately.