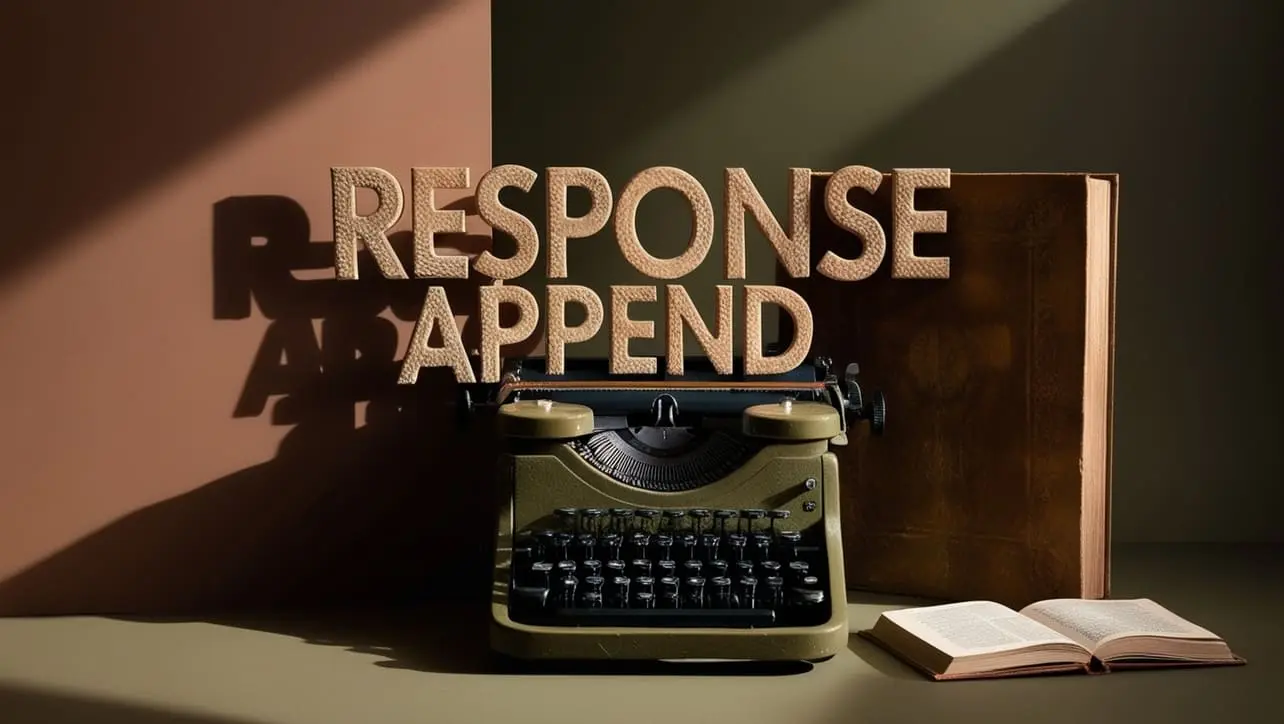
Express req.is() Method
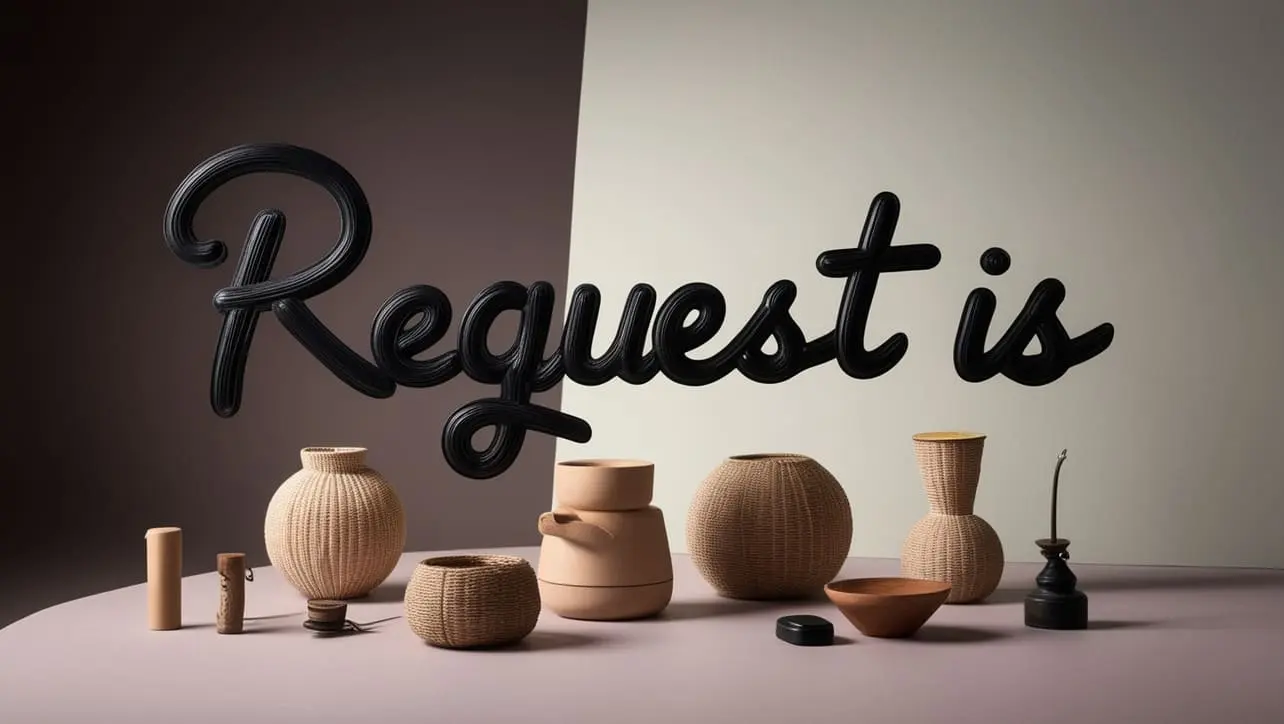
Photo Credit to CodeToFun
🙋 Introduction
When building web applications, handling different content types is crucial for processing incoming requests. Express.js provides the req.is()
method, which allows you to verify the content type of an incoming request.
In this guide, we'll explore the syntax, use cases, and best practices for using the req.is()
method in Express.js.
💡 Syntax
The syntax for the req.is()
method is straightforward:
req.is(type)
- type: A string or an array of strings representing the content type(s) to check against.
❓ How req.is() Works
The req.is()
method checks if the content type of the incoming request matches the specified type(s). It returns true if there is a match and false otherwise.
app.post('/upload', (req, res) => {
// Check if the content type is 'multipart/form-data'
if (req.is('multipart/form-data')) {
// Process the file upload
// ...
res.send('File uploaded successfully');
} else {
res.status(400).send('Invalid content type');
}
});
In this example, the route /upload uses req.is()
to verify that the incoming request has a content type of multipart/form-data before processing a file upload.
📚 Use Cases
File Upload Validation:
Use
req.is()
to ensure that file uploads are of the expected content type before processing them in your routes.example.jsCopiedapp.post('/upload', (req, res) => { // Check if the content type is 'multipart/form-data' if (req.is('multipart/form-data')) { // Process the file upload // ... res.send('File uploaded successfully'); } else { res.status(400).send('Invalid content type'); } });
API Endpoint Validation:
Verify the content type of incoming data in API endpoints to ensure it matches the expected type.
example.jsCopiedapp.post('/api/data', (req, res) => { // Check if the content type is 'application/json' if (req.is('application/json')) { // Process the JSON data // ... res.send('JSON data processed successfully'); } else { res.status(400).send('Invalid content type'); } });
🏆 Best Practices
Specify Content Types Carefully:
When using
req.is()
, be explicit about the expected content types to avoid unexpected matches.example.jsCopiedapp.post('/upload', (req, res) => { // Check if the content type is either 'image/jpeg' or 'image/png' if (req.is(['image/jpeg', 'image/png'])) { // Process the image upload // ... res.send('Image uploaded successfully'); } else { res.status(400).send('Invalid content type'); } });
Combine with Other Middleware:
Combine
req.is()
with other middleware functions to create more comprehensive request processing pipelines.example.jsCopied// Middleware for content type validation const validateContentType = (req, res, next) => { if (req.is('application/json')) { return next(); } else { res.status(400).send('Invalid content type'); } }; // Apply content type validation middleware to a route app.post('/api/data', validateContentType, (req, res) => { // Process the JSON data // ... res.send('JSON data processed successfully'); });
🎉 Conclusion
The req.is()
method in Express.js provides a simple yet effective way to verify the content type of incoming requests. By understanding its syntax, use cases, and best practices, you can enhance the reliability and security of your Express.js applications.
Now, armed with knowledge about the req.is()
method, go ahead and implement content type verification in your Express.js projects!
👨💻 Join our Community:
Author
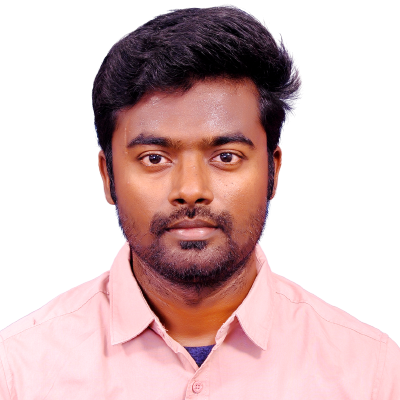
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.is() Method), please comment here. I will help you immediately.